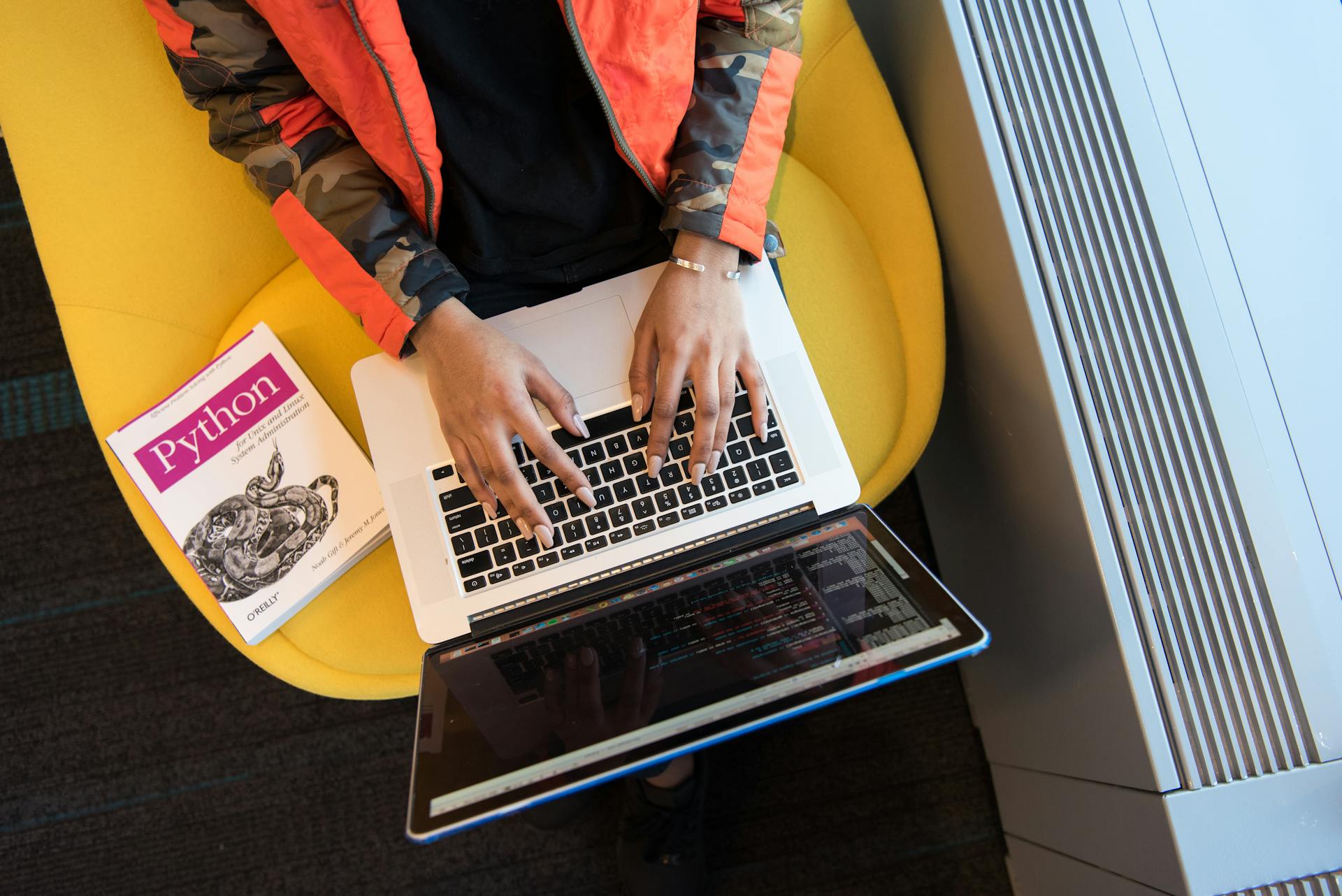
Getting started with the IBKR API in Python is a great way to automate your trading and investment strategies. The IBKR API allows you to access your trading account and perform various actions programmatically.
You can use the IBKR API to create a trading bot that automatically executes trades based on your predefined rules. This can be a huge time-saver and help you stay on top of your investments.
To get started, you'll need to install the IBKR API library for Python, which can be done using pip. The library is called ibapi and can be installed with the command pip install ibapi.
Setting Up IB API
To set up the IB API, you'll need to download the IBJts source from the install page, specifically the ZIP file under the Mac/Linux column.
Unzip the file and navigate to the IBJts/source/pythonclient directory. From there, run python3 setup.py install to complete the installation process.
For Windows users, the process is similar, but if you're using a Mac or Linux, this is the path you'll need to follow.
Setting Up IB Native Interface
Setting up the IB native interface is a crucial step in getting started with the IB API. You'll need to open an account with IB, which offers demo accounts perfect for testing. If you decide to connect to a live account, there's a read-only option for the API in TWS that's useful when testing and in the early stages of getting to know the API.
To download the IB Python native API, you'll need to get the script files written in Python that facilitate the connection and communication with IB's client. This client is connected to their server.
You'll also need to download your IB client, either TWS or IB Gateway, which is the default trading client provided by Interactive Brokers. TWS provides a visual confirmation of any activity in your account, making it a great choice for testing your script.
For coding your Python scripts, you'll need to choose an IDE that allows for a constant open connection. Not all IDEs are suitable, so be sure to pick one that works for you.
Here are the basic steps to setting up a connection to the IB API in Python:
- Open an account with IB
- Download the IB Python native API
- Download your IB client (TWS or IB Gateway)
- Choose your IDE
Establishing Connection
To establish a connection with IB's API, you need to be logged into TWS. Ensure you have a successful login session before proceeding.
Before running the code to connect your IDE with IB's API, you must be logged into TWS. A successful execution of the code will automatically confirm the connection.
You can begin developing your own analysis or trading logic after establishing the connection. This is a crucial step in setting up the IB API.
A successful execution of the code will automatically confirm the connection. This confirms that your IDE is now connected to IB's API.
Tread cautiously and rigorously test your code before using your live account.
Getting Started with IBPy
To get started with IBPy, you'll need to install git on your Ubuntu system, which can be handled by running a command.
IBPy is a Python wrapper around the Java-based Interactive Brokers API, making development of algorithmic trading systems in Python less problematic.
You'll create a subdirectory to store IBPy, such as under your home directory, to keep everything organized.
To download IBPy, use the git clone command within the IbPy directory.
Make sure to install IBPy using your preferred Python virtual environment once you've entered the IbPy directory.
After installation, you'll need to open up TWS, as described in the prior tutorial, to complete the setup process.
Finally, run the code to start using IBPy for your algorithmic trading systems.
IB API Components
The IB API components are the building blocks of any Python program that interacts with the TWS API. These components are essential for creating a connection to TWS and processing returned messages.
The API classes EClient and EWrapper are the core components of a TWS API Python program. EClient is responsible for creating a connection to TWS.
A function call to create a connection to TWS is also a crucial component of a TWS API Python program. This function call is necessary to establish a connection to the API.
A run loop for processing returned messages in the queue is another essential component of a TWS API Python program. This loop allows the program to receive and process messages from the API.
The "Hello World" example in the API documentation demonstrates how to implement these components in a simple Python program. This example sends a query for details about a financial instrument and prints the received details to the console.
The "Program.py" sample program, included with the API download, shows the syntax of all API functions.
Market Data and Orders
Requesting market and historical data is a fundamental aspect of using the IBKR API with Python. The TWS Python API allows you to request streaming and historical data, as well as display market data in the console.
You can use the TWS Python API to request market data, including streaming and historical data. This data can be displayed in the console, giving you a real-time view of market activity.
The IBKR API has limitations on requesting data, so it's essential to understand what types of data are included in the real-time feed versus the historical database.
Worth a look: Ibkr Pre Market
Defining Contracts
Defining a contract in the TWS API is a crucial step in accessing market data and executing trades. This process allows you to discover and define a contract using the TWS API.
To start, you need to explore contract discovery, which is covered in the TWS API documentation. Contract discovery is essential for defining a contract.
Defining a contract involves using the TWS API, as mentioned in the lesson on contract discovery. This allows you to access a wide range of contracts.
By defining a contract, you can access real-time market data, enabling you to make informed trading decisions.
Explore further: Ibkr Money Market Funds
Requesting Market Data
Requesting Market Data is a crucial step in trading, and the TWS Python API makes it relatively straightforward. You can request both real-time and historical market data using the API.
The API allows you to request streaming and historical data, which can be displayed in the console. This is particularly useful for traders who need to stay up-to-date with market movements in real-time.
IBKR's real-time feed includes various types of data, but there are limitations on requesting data. It's essential to understand what data is included in the feed and what's available in the historical database to make informed trading decisions.
The Python code examples provided demonstrate the minimum code necessary to request streaming and historical data. These examples are a great starting point for traders looking to integrate market data into their trading strategies.
Market Parameters & Scanners
You can request market scanner parameters using the TWS Python API, which is a powerful tool for retrieving market data.
The TWS Python API allows you to request market scanner parameters, giving you a detailed look at the market.
To request market scanner parameters, you'll use the TWS Python API, which is a valuable resource for traders.
The TWS market scanner is also accessible through the TWS Python API, providing you with real-time market data.
In this lesson, we'll walk through how to request market scanner parameters using the TWS Python API.
Portfolio and Parameters
The TWS API is a powerful tool for accessing portfolio data and account information. You can use it to receive portfolio and account data in real-time.
The API has various functions for receiving portfolio and account data, including reqAccountUpdates, which can be used to subscribe to both portfolio and account data for an individual account. This function is commonly used with a subscribe-and-publish model.
The reqAccountUpdates function allows you to continuously receive updates in real-time, making it an essential tool for tracking your portfolio and account activity.
Intriguing read: Ibkr Llc Account
Implementation and Examples
To get started with the IBKR API in Python, you'll need to install the ibapi library using pip.
The IBKR API supports both synchronous and asynchronous programming, allowing you to choose the approach that best fits your needs.
You can use the IB API to connect to the IBKR platform, but first, you need to create an account and obtain an API key.
The IBKR API provides a simple way to place trades, including market and limit orders, as well as stop-loss orders.
To place a trade, you'll need to create an Order object and specify the trade details, such as the symbol, quantity, and order type.
The IBKR API also supports real-time market data, including quotes, trades, and order book information.
You can use the IB API to get real-time quotes for a specific symbol, which can be useful for building your own trading strategies.
The IBKR API provides a range of examples in Python, including a simple example of placing a trade and another example of getting real-time quotes.
These examples can serve as a good starting point for your own projects, and can help you get up to speed with the IBKR API quickly.
If this caught your attention, see: Ibkr Futures Margin
Installation and Setup
To install the IB API on a Mac or Linux, navigate to the install page and download the ZIP file under the Mac/Linux column. Unzip the file, and then navigate to IBJts/source/pythonclient and run python3 setup.py install.
For Windows users, the process is similar. You can download the ZIP file from the install page and extract it to your desired location. The IB API is also available for download from GitHub, but be aware that this is the bleeding-edge source code.
The TWS API can be downloaded and installed by accessing the source code repository on GitHub. The repository includes the public download site and the private GitHub repository with the latest source code.
Download the Native
To download the Native API, head over to the Interactive Brokers website and navigate to Technology – Trading APIs – Get API Software, or simply click on this link – http://interactivebrokers.github.io/. Make sure to select API version 9.73 or higher, as anything prior to that won't have the Python source files needed.
You'll also want to ensure you're using Python version 3.1 or higher, as the API is compatible with this version and above. I recommend checking your Python version before proceeding to avoid any potential issues.
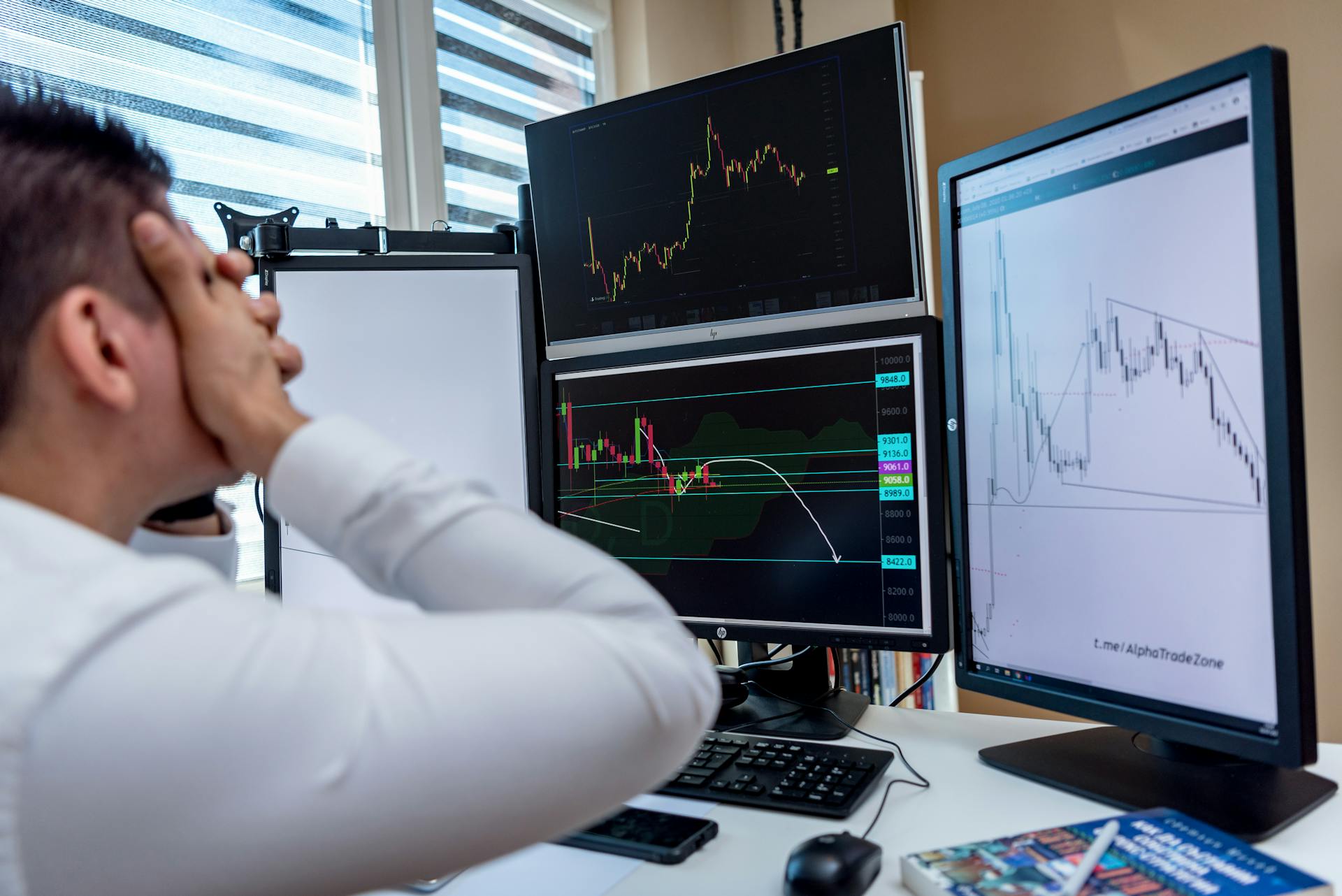
Run the downloaded msi file and follow the setup wizard to copy the required Python source files to your hard drive. Be sure to navigate to the directory you specified in the installer and drill down to the /TWS API/source/pythonclient folder.
In this folder, run the python3 setup.py install file to install the API as a package. This will complete the installation process.
Install IB on Mac or Linux
To install the IB API on a Mac or Linux, navigate to the install page and download the ZIP file under the Mac/Linux column.
The process is similar to the one described for Windows. You can find the ZIP file on the install page.
Unzip the file and navigate to IBJts/source/pythonclient.
Run python3 setup.py install to complete the installation.
Download IB Client
To download the IB Client, you'll need to visit the official website of Interactive Brokers, which can be accessed through the link provided in the "System Requirements" section.
The IB Client is compatible with Windows and macOS operating systems, as mentioned in the "System Requirements" section.
You'll need to create an account on the Interactive Brokers website to download the IB Client. This is a one-time process that only takes a few minutes.
The download process typically takes a few minutes to complete, depending on your internet connection speed.
Once the download is complete, you can launch the IB Client installer and follow the on-screen instructions to install the software.
Installing API Endpoint
Installing the API endpoint is a breeze. You can get started with just two lines of code.
The initial setup is in your IDE, which is a great advantage. This means you can begin working on your project right away.
There's no need to download anything extra from IB's TWS API website, unlike what their documentation suggests. This can save you time and effort.
Getting the API set up is straightforward and requires minimal effort.
Installing IBPy
Installing IBPy is a straightforward process. You'll need to install git first, which is handled by a simple command on a Ubuntu system.
To create a subdirectory for IBPy, you can place it underneath your home directory. This is what the author of the tutorial did on their system.
Next, you'll need to download IBPy via git clone. This is a crucial step in the installation process.
Once you've downloaded IBPy, you'll need to navigate to the IbPy directory. This is where the installation process will continue.
After navigating to the IbPy directory, you'll need to install it using a preferred Python virtual environment. This will ensure that your installation is isolated from other Python projects.
The final step is to run the code, but before that, you'll need to open up TWS as described in the prior tutorial.
Related reading: Tradingview Ibkr Tutorial
General Information
The IBKR API is a powerful tool that allows developers to access the Interactive Brokers trading platform programmatically. It's a Python library that makes it easy to connect to the IBKR API.
To get started with the IBKR API, you'll need to create an account with Interactive Brokers and obtain an API key. This key is required to authenticate and authorize API requests.
The IBKR API uses the RESTful API architecture, which is a widely adopted standard for building web services. This architecture allows for easy integration with other applications and services.
Overview
The Interactive Broker Client Portal Web API is a powerful tool that allows individuals with Interactive Broker accounts to manage their trades and accounts with ease. This is made possible through a Python API client library.
You can use this library to pull historical and real-time data, giving you a comprehensive view of your investments. This feature is especially useful for tracking market trends and making informed decisions.
The Python API client library also enables you to create and modify orders, giving you full control over your trades. This level of control is invaluable for investors who want to stay on top of their portfolios.
On a similar theme: Stock Broker Api
Join the Conversation
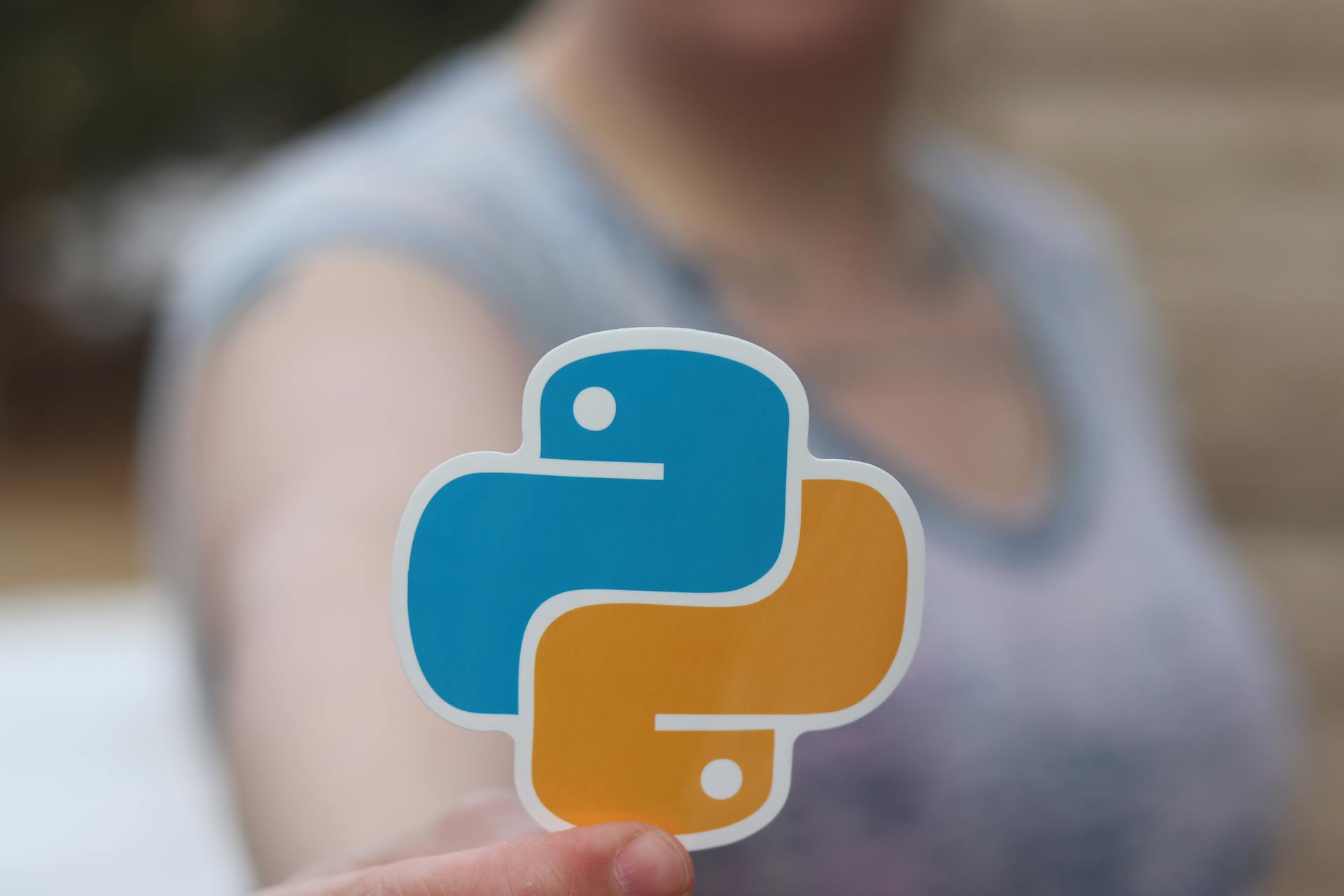
Before posting a question, it's a good idea to look through the FAQs to see if your question has already been covered. This will save you time and help you get a quicker answer.
You can also join the conversation by hearing about the latest tools and techniques from the IBKR API staff. They share their knowledge to help you stay up-to-date with the latest developments.
Looking through the FAQs is a great way to get started and make the most of your experience.
Frequently Asked Questions
What language is the IBKR API?
The IBKR API supports multiple programming languages, including Python, Java, C++, C#, and Visual Basic. Choose the language that best fits your development needs.
Sources
- https://www.interactivebrokers.com/campus/ibkr-quant-news/interactive-brokers-python-api-native-a-step-by-step-guide/
- https://www.interactivebrokers.com/campus/trading-course/python-tws-api/
- https://github.com/areed1192/interactive-broker-python-api
- https://www.quantstart.com/articles/Using-Python-IBPy-and-the-Interactive-Brokers-API-to-Automate-Trades/
- https://medium.com/@sauravchakers/integrating-interactive-brokers-api-with-python-in-your-ide-an-easy-implementation-guide-9e47cb87bf5e
Featured Images: pexels.com