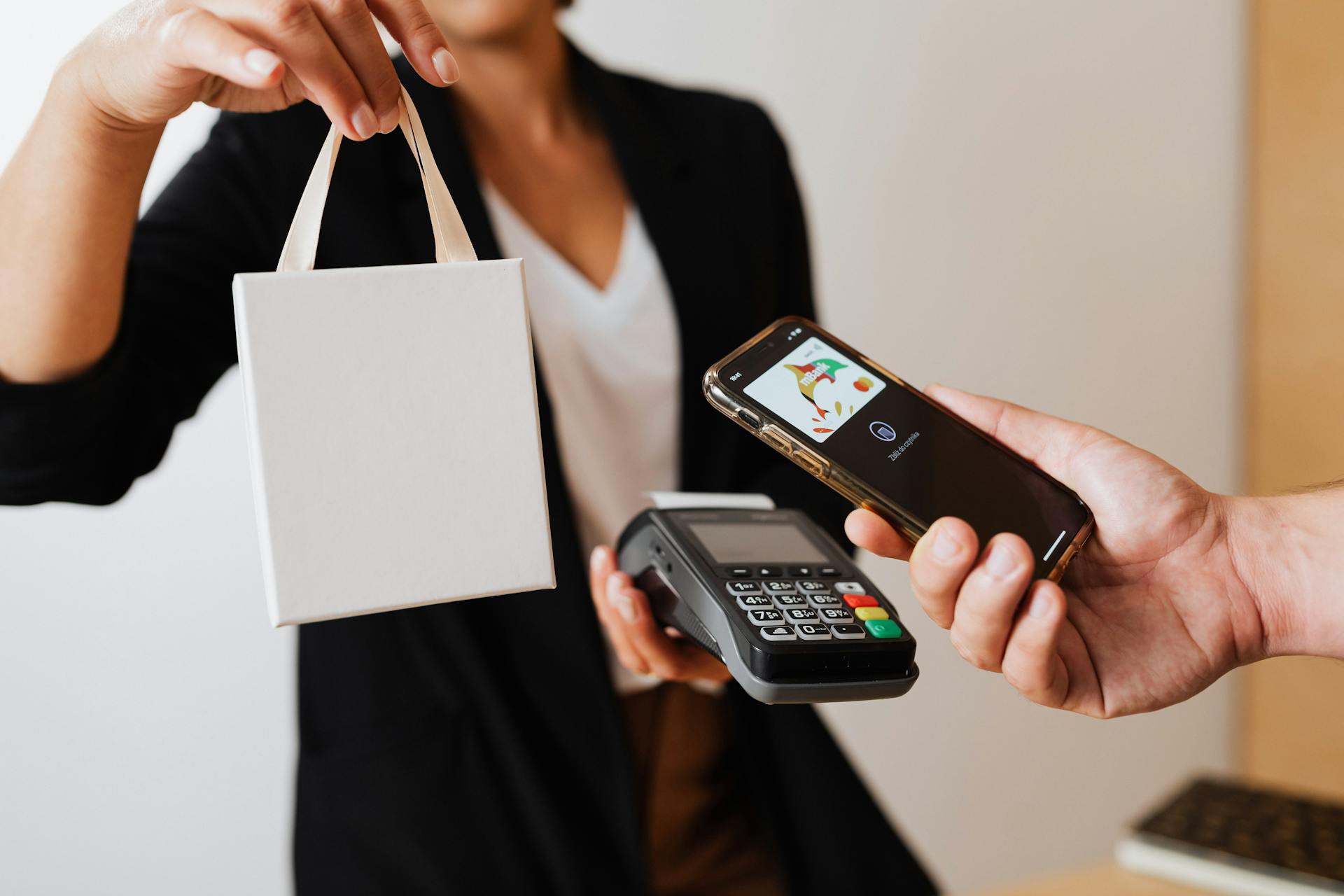
Implementing Apple Pay in SwiftUI requires a few key steps. First, you need to import the PassKit framework.
To use Apple Pay, your app needs to be configured as a payment processing app, which involves registering for a merchant ID and obtaining a payment processing certificate. This is a one-time process that you'll need to complete before you can start using Apple Pay.
Next, you'll need to create a PKPaymentButton in your SwiftUI view, which will handle the user's interactions with Apple Pay. The button's style and configuration will depend on your app's design and layout.
To add event handling to your PKPaymentButton, you'll need to use the .onAppear modifier to set up a payment session when the button appears on screen. This will allow your app to communicate with the user's device and process payments.
You might like: How to Pay with Applepay
Setting Up Apple Pay
Setting up Apple Pay involves several key steps. To begin, select the MoltinProducts project in the left navigation bar and change the Bundle Identifier to match the one you created previously.
Ensure that the Team selection box is pointing to the development team under which you created your App ID and merchant ID. This is crucial for Apple Pay setup.
Click the Capabilities tab, expand the Apple Pay section, and ensure that the switch on the right is set to ON. This enables Apple Pay functionality in your app.
To verify that your merchant ID is correctly set up, push the refresh button below the merchant ID list. You should see the list populate with the merchant ID you added on the developer portal.
Make sure that your merchant ID checkbox is selected. This confirms that your Apple Pay credentials are correctly set up.
Here's a quick checklist to ensure you've completed all the necessary steps:
- Select the MoltinProducts project and change the Bundle Identifier.
- Ensure the Team selection box is pointing to the correct development team.
- Enable Apple Pay in the Capabilities tab.
- Refresh the merchant ID list.
- Select the merchant ID checkbox.
At this point, you should have checkmarks next to all sections that deal with setting up a project, displaying a product, and setting up Apple Pay credentials.
Create Request
To create an Apple Pay request in SwiftUI, you'll need to import the PassKit framework. This is done by adding the following line to the top of your file: `import PassKit`.
You'll also need to locate the `applePayPressed(sender:)` function, which is executed when the user attempts to purchase an item. This is where you'll create a `PKPaymentRequest` and a `PKPaymentAuthorizationViewController`.
Here's a step-by-step guide to creating the request:
1. Add the following code to the body of `applePayPressed(sender:)`:
```swift
let request = PKPaymentRequest()
let applePayController = PKPaymentAuthorizationViewController(paymentRequest: request)
self.present(applePayController!, animated: true, completion: nil)
```
2. Add the following code just under the `IBOutlet` properties of your view controller:
```swift
let SupportedPaymentNetworks = [PKPaymentNetwork.visa, PKPaymentNetwork.masterCard, PKPaymentNetwork.amex]
let ApplePayMerchantID = "merchant.com.YOURDOMAIN.ApplePayMoltin"
```
Make sure to fill in your merchant ID and add any extra support payments as needed.
Here's a summary of the required code:
In the `viewDidLoad` method, check if the user can use Apple Pay by calling `PKPaymentAuthorizationViewController.canMakePaymentsUsingNetworks(SupportedPaymentNetworks)`. If the user can use Apple Pay, hide the Apple Pay button.
Remember to fill in the request details, such as the merchant ID and supported networks, in the `applePayPressed(sender:)` function.
Implementing Payment Methods
To implement Apple Pay in SwiftUI, you need to configure your environment by creating a merchant ID with Apple and a Payment Processing certificate. Make sure you're generating a certificate file and open it in your Keychain Access.
You can add Apple Pay Capability to your Xcode project by turning on the switch in the Apple Pay row and inputting the merchant IDs you want to use in the app. Ensure it matches the exact merchant ID you've created.
To enable Apple Pay for your app, you need to import the PassKit framework, which provides all the APIs for Apple Pay. Initialize a PKPaymentRequest object to specify relevant information, such as country code, currency code, supported payment networks, and merchant ID.
You can present the view controller using a PKPaymentAuthorizationViewController, which will display the Apple Pay UI and authorize the payment. This view controller manages all interactions with the user, so there's no extra work for you.
Here's a list of features you can specify in a PKPaymentRequest:
- Country Code
- Currency Code
- Supported Payment Networks (such as Visa, Master Card, American Express, etc)
- Merchant ID
- Required billing contact fields
- Required shipping contact fields
- Available shipping methods
You can also subscribe to payment events via PKPaymentAuthorizationViewControllerDelegate to get granular notifications for what the user is doing.
Implementing SwiftUI
Implementing SwiftUI for Apple Pay can be a bit tricky. You can't do it natively yet, so you'll need to wrap the PKPaymentAuthorizationViewController in a UIViewControllerRepresentable.
You can get a working view by binding the PKPaymentAuthorizationViewController to an isPresentingApplePay bool, but dismissing the view can be a challenge. Tapping on the cancel button or submitting the payment might not dismiss the view, and you might need to tap outside the Apple Pay window to get it to disappear.
The Apple Documentation Engineer suggests checking out the Fruta sample code project, specifically the PaymentButton.swift file, for a demonstration of how to present an Apple Pay button in your SwiftUI app. However, this only wraps the UI button and doesn't illustrate presenting or dismissing the authorization view or state management.
Set Up Project
To set up your project for implementing SwiftUI, you'll need to start by setting up your Apple Pay project. This involves selecting the MoltinProducts project in the left navigation bar and changing the Bundle Identifier to match the one you created previously.
You'll also need to ensure that the Team selection box is pointing to the development team under which you created your App ID and merchant ID. This is crucial for Apple Pay to work correctly.
Next, click on the Capabilities tab and expand the Apple Pay section. Make sure the switch on the right is set to ON, and then push the refresh button below the merchant ID list. This will populate the list with the merchant ID you added on the developer portal.
To verify that everything is set up correctly, check that you have checkmarks next to all sections that deal with setting up a project, displaying a product, and setting up Apple Pay credentials. If any of the sections is not marked as checked, retrace your steps and correct the details to make sure you’ve satisfied Apple’s requirements.
If this caught your attention, see: How to Set up Applepay
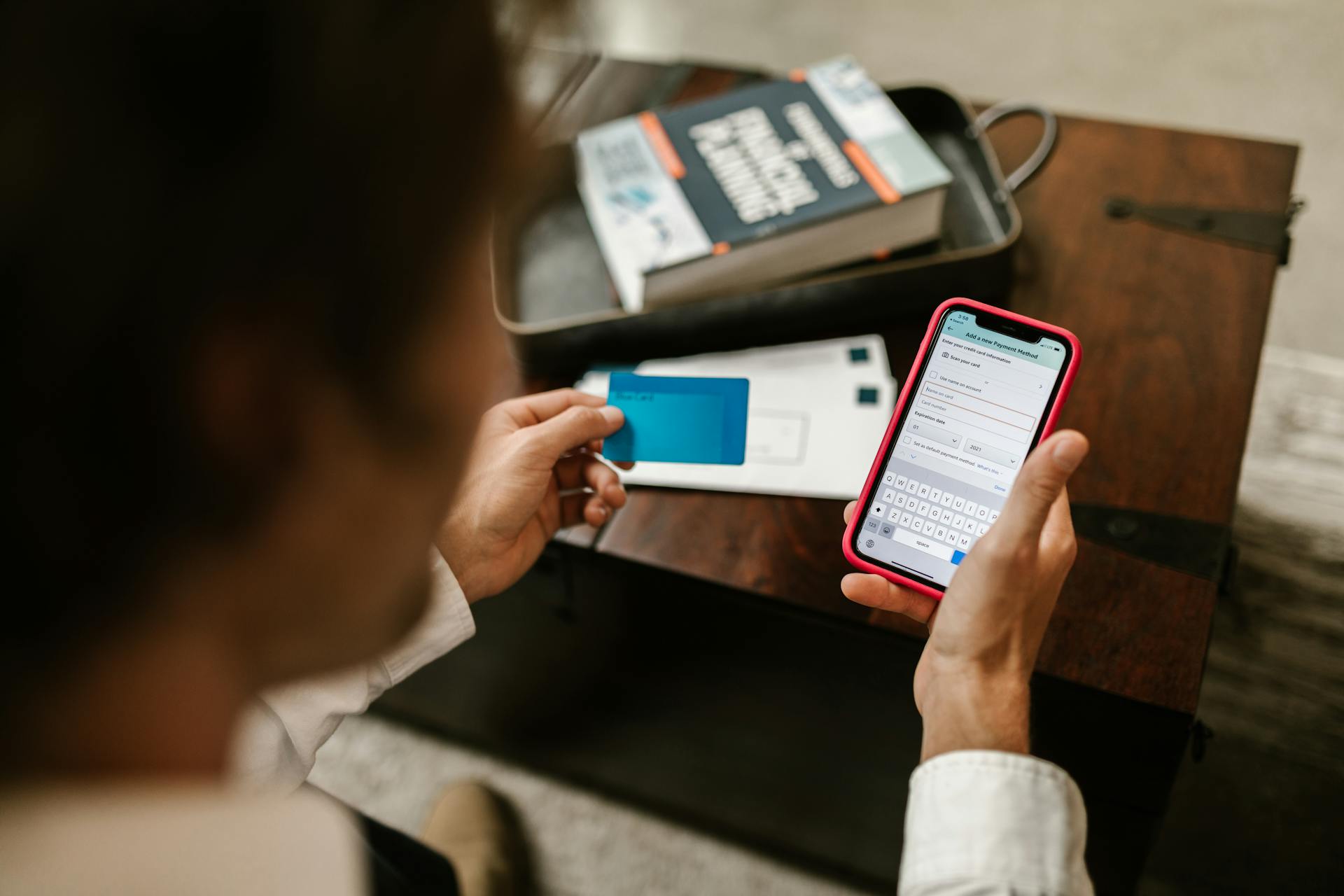
Here's a quick checklist to help you set up your project:
- Select the MoltinProducts project and change the Bundle Identifier
- Ensure the Team selection box is pointing to the correct development team
- Turn on the Apple Pay switch in the Capabilities pane
- Refresh the merchant ID list
- Make sure the merchant ID checkbox is selected
Implementing SwiftUI
Implementing SwiftUI can be a bit tricky, but don't worry, I've got you covered.
To get started, you'll need to import the PassKit framework, which is provided by Apple. This is a crucial step, as it allows you to access the APIs needed to implement Apple Pay.
One way to implement Apple Pay in SwiftUI is to use the PKPaymentAuthorizationViewController, but be aware that this can be a bit finicky. You may need to use a UIViewControllerRepresentable to wrap the view controller.
If you're using a SwiftUI app, you can display the Apple Pay UI by binding the view to an isPresentingApplePay boolean. However, be aware that the cancel button may not always dismiss the view, and sometimes the didFinish delegate function may not be called.
To handle this, you can try passing the binding variable to the didFinish delegate function and setting it to false. However, this may not always work, and the only way to get the view to disappear may be to tap on any part outside of the Apple Pay window.
Related reading: Implement Homomorphic Sorting
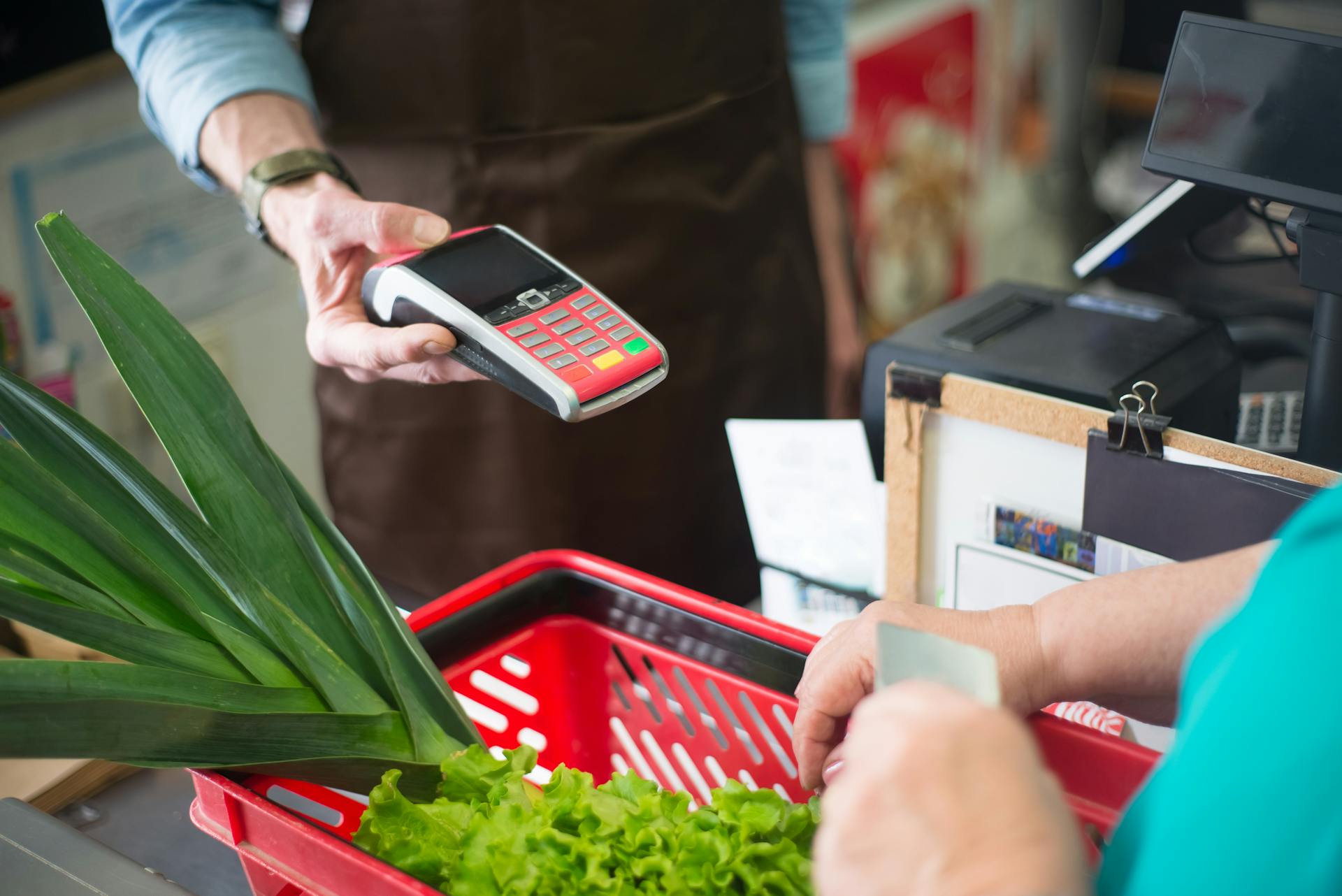
Here are some steps to help you implement Apple Pay in SwiftUI:
- Import the PassKit framework
- Use a UIViewControllerRepresentable to wrap the PKPaymentAuthorizationViewController
- Bind the view to an isPresentingApplePay boolean
- Handle the didFinish delegate function to dismiss the view
By following these steps, you should be able to implement Apple Pay in your SwiftUI app.
Add Button
To add a button in SwiftUI, review the Apple Pay guidelines. Apple has a set of specific guidelines for Apple Pay buttons.
You can find a zip file on the Apple Pay developer site that contains approved button resources for Apple Pay. This zip file is accessible through the Apple Pay Buttons and Resources link.
Select the Apple Pay button in the Buy Product Interface Builder and change the image to ApplePay. Give your button an empty title instead of the default "button" title.
Your scene should look similar to the one described in the guidelines. The Apple Pay images are also available in the starter project's Image.xcassets for your use.
Step-by-Step Guide
To implement Apple Pay in SwiftUI, you'll need to follow these steps. First, get the code from GitHub. This will give you a solid foundation to build upon.
Next, set up your iOS app project by following the instructions provided. This will ensure that everything is properly configured for Apple Pay integration.
Once you have your project set up, it's time to display a product. This can be done by adding the necessary code to your SwiftUI views.
After displaying a product, you'll need to update your store with the necessary information. This will allow users to make purchases using Apple Pay.
To set up Apple Pay credentials, you'll need to create an Apple Pay project. This involves setting up the necessary credentials and configuring your project accordingly.
Now that you have your Apple Pay project set up, it's time to add an Apple Pay button to your app. This will allow users to initiate the Apple Pay process.
To create an Apple Pay request, you'll need to handle shipping and implement Apple Pay delegates. This will ensure that the payment process is smooth and secure.
Finally, you'll need to create a checkout in Composable Commerce and create payment transactions. This will complete the Apple Pay integration process and allow users to make purchases using Apple Pay.
Here's a summary of the steps:
- Get the code from GitHub.
- Set up the iOS app project.
- Display a product.
- Update your store.
- Set up Apple Pay credentials.
- Add an Apple Pay button.
- Create an Apple Pay request.
- Handle shipping.
- Implement Apple Pay delegates.
- Create a checkout in Composable Commerce.
- Create payment transactions.
Sources
- https://elasticpath.dev/guides/How-To/paymentgateways/integrate-applepay-with-swift
- https://docs.moyasar.com/ios-sdk
- https://medium.com/@aserdah/integrating-apple-pay-and-stripe-payment-gateway-into-a-swiftui-app-400fefc1f4a
- https://stackoverflow.com/questions/60766310/implementing-apple-pay-with-swiftui
- https://iosapptemplates.com/blog/ios-programming/integrate-apple-pay-ios-app-swift-source-code
Featured Images: pexels.com