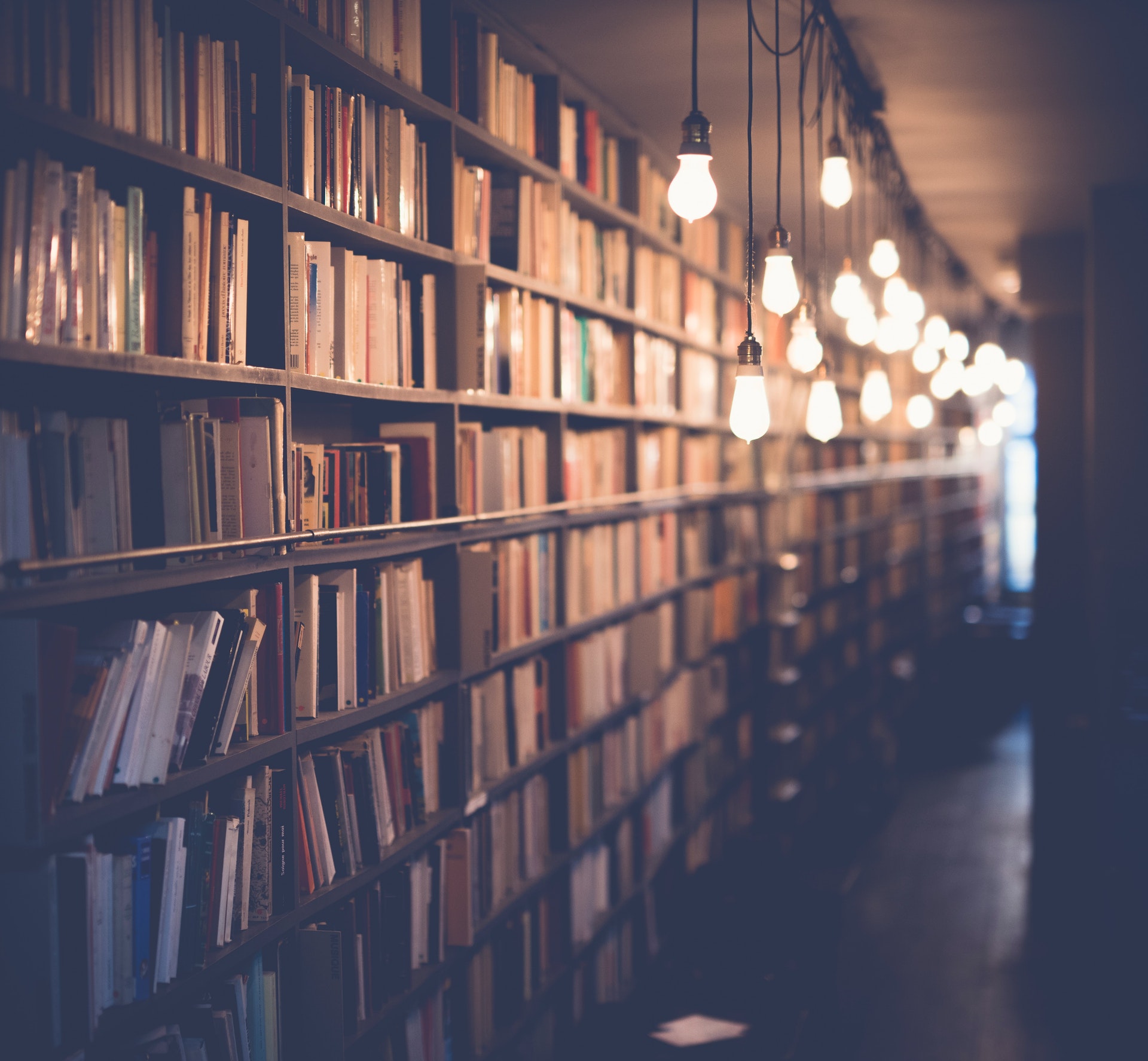
In computer science, homomorphic sorting is a sorting algorithm that can be applied to data that has already been sorted, without having to re-sort the data.
The algorithm works by taking advantage of the fact that the data is already sorted and using that to its advantage. The algorithm uses a comparison function that is able to determine the order of two elements in the sorted list. This comparison function is used to sorted list. This comparison function is used to compare the elements in the list and determine their order.
The algorithm then uses the comparison function to determine the order of the elements in the list. The comparison function is used to compare the elements in the list and determine their order. The algorithm then uses the comparison function to determine the order of the elements in the list. The comparison function is used to compare the elements in the list and determine their order.
The algorithm then uses the comparison function to determine the order of the elements in the list. The comparison function is used to compare the elements in the list and determine their order. The algorithm then sorts the data according to the comparison function.
Explore further: Data Governance
What is homomorphic sorting?
In computer science, homomorphic sorting is a sorting algorithm that can be used to sort data while preserving the structure of the data. That is, the algorithm preserves the order of the data while sorting it. Homomorphic sorting is a generalization of the bubble sort and insertion sort algorithms.
The idea behind homomorphic sorting is to represent the data to be sorted as a graph. The algorithm then sorts the graph by processing it topologically. That is, it sorts the vertices of the graph according to their in-degree, and then processes the edges of the graph in the order of the sorted vertices. The result is a topologically sorted graph, which is equivalent to a sorted list of data.
The time complexity of homomorphic sorting is O(n log n), where n is the number of items to be sorted. The space complexity is O(n), which is the same as the space complexity of the bubble sort and insertion sort algorithms.
The main advantage of homomorphic sorting is that it is a simple and elegant algorithm that can be used to sort data in a way that preserves the structure of the data. Additionally, the algorithm is relatively efficient, with a time complexity of O(n log n).
Discover more: Industry Sorting Code Directory
What are the benefits of homomorphic sorting?
Homomorphic sorting is a powerful tool that can be used to sort data in a way that preserves the structure of the data. This type of sorting is often used to sort data that has a great deal of structure, such as data that contains patterns or data that is highly connected.
There are many benefits to using homomorphic sorting. One benefit is that homomorphic sorting can be used to sort data in a way that is more efficient than traditional sorting algorithms. Homomorphic sorting can also sort data in a way that is more accurate than traditional sorting algorithms.
Another benefit of homomorphic sorting is that it can be used to preserve the structure of the data. This is important when the data contains patterns or is highly connected. By preserving the structure of the data, homomorphic sorting can help ensure that the data is correctly sorted.
Finally, homomorphic sorting can be used to sort data in a way that is more resilient to errors. This is because homomorphic sorting can sort data in a way that is more resistant to changes in the data. This is important when the data is being used for critical applications, such as data that is being used for medical applications.
Overall, homomorphic sorting is a powerful tool that can be used to sort data in a way that is more efficient, more accurate, and more resilient to errors. This makes homomorphic sorting an important tool for applications that require high-quality data.
On a similar theme: Pronounce Sorting
How can homomorphic sorting be implemented?
Homomorphic sorting is a type of sorting algorithm that can be used to sort data that is in a homomorphic form. This means that the data is in a form that can be mapped to a sorted form, without having to change the data itself.
There are many different ways that homomorphic sorting can be implemented, but one of the most common ways is to use a function that takes in the data and then outputs a sorted version of the data. This function can be written in many different programming languages, but it is typically written in C or assembly.
The function takes in the data and then uses a series of comparisons to determine how the data should be sorted. Once the comparisons are complete, the data is then output in the sorted form.
There are many benefits to using homomorphic sorting, but one of the biggest benefits is that it can be used to sort data that is in a very large scale. This is because the function can be written to run on data that is in a very large scale, and it will still output the data in the sorted form.
Another benefit of homomorphic sorting is that it is very efficient. This is because the function only has to run once on the data, and it can then output the data in the sorted form. This is in contrast to other sorting algorithms, which have to run multiple times on the data in order to sort it.
There are some drawbacks to using homomorphic sorting, but the biggest drawback is that it can only be used to sort data that is in a homomorphic form. This means that the data has to be in a form that can be mapped to a sorted form, without having to change the data itself.
Overall, homomorphic sorting is a very powerful sorting algorithm that has many benefits. It is most commonly used to sort data that is in a very large scale, but it can also be used to sort data that is in a homomorphic form.
What data structures are well suited for homomorphic sorting?
There are several data structures that are well suited for homomorphic sorting. One such data structure is the balanced binary tree. A balanced binary tree is a data structure that allows for the efficient retrieval and insertion of data. Furthermore, a balanced binary tree is well suited for homomorphic sorting because it maintains a balanced structure even when data is inserted in an unsorted fashion.
Another data structure that is well suited for homomorphic sorting is the heap. A heap is a data structure that allows for efficient retrieval and insertion of data. Furthermore, a heap is well suited for homomorphic sorting because it maintains its structure even when data is inserted in an unsorted fashion.
The final data structure that is well suited for homomorphic sorting is the suffix tree. A suffix tree is a data structure that allows for the efficient retrieval and insertion of data. Furthermore, a suffix tree is well suited for homomorphic sorting because it allows for the efficient retrieval of suffixes of data.
What are some of the challenges associated with homomorphic sorting?
There are several challenges associated with homomorphic sorting. One challenge is that it is difficult to evaluate the performance of homomorphic sorting algorithms. There are no well-defined benchmarks for these algorithms, so it is difficult to compare their performance against traditional sorting algorithms. Additionally, homomorphic sorting algorithms are often slower than traditional algorithms, so they are not always practical for large-scale sorting tasks. Another challenge is that homomorphic sorting algorithms can be hard to implement. These algorithms are often highly complex, and their implementation can be error-prone. Finally, homomorphic sorting can be vulnerable to security attacks. If an attacker can control the input to a homomorphic sorting algorithm, they can potentially influence the output of the algorithm in a way that is advantageous to them.
How can the efficiency of homomorphic sorting be improved?
There are a number of ways in which the efficiency of homomorphic sorting can be improved. One way is to use a more efficient sorting algorithm. For example, instead of using a quicksort algorithm, a more efficient sorting algorithm such as a mergesort algorithm could be used.
Another way to improve the efficiency of homomorphic sorting is to use a better heuristic for choosing the pivot element. For example, instead of choosing the pivot element to be the first element in the array, a better heuristic would be to choose the pivot element to be the median element in the array.
Finally, the efficiency of homomorphic sorting can be improved by using a more sophisticated data structure to represent the array. For example, instead of using an array of integers, a more sophisticated data structure such as a binary search tree could be used. This would allow the elements in the array to be sorted in logarithmic time instead of linear time.
What are some of the applications of homomorphic sorting?
Homomorphic sorting is a sorting algorithm that can be used to sort data based on similarity. It is a method of sorting that is well suited for data that has many-to-many relationships, such as people and their friends, or products and their reviews.
The algorithm works by first partitioning the data into groups based on similarity. Next, the groups are sorted based on their size. Finally, the data is output in order from the largest group to the smallest group.
The advantage of homomorphic sorting is that it is very efficient for large data sets. The disadvantage is that it can be difficult to understand the output.
Applications of homomorphic sorting include:
• Social networking: Friends can be sorted by how similar they are to the user.
• Product reviews: Products can be sorted by how similar they are to other products.
• Music: Songs can be sorted by how similar they are to other songs.
• Movies: Movies can be sorted by how similar they are to other movies.
Intriguing read: Ad Group
How does homomorphic sorting compare to other sorting algorithms?
Homomorphic sorting is a sorting algorithm that can be used to sort data that is in a homomorphic form. This means that the data can be represented as a set of values that can be mapped to a set of integers. Homomorphic sorting is similar to other sorting algorithms, but it has some advantages that make it more efficient.
One advantage of homomorphic sorting is that it can be used to sort data that is not in a uniform format. This is because the data can be represented as a set of values that can be mapped to a set of integers. This means that the data can be sorted without having to be converted into a uniform format.
Another advantage of homomorphic sorting is that it is not limited by the size of the data set. This is because the data can be mapped to a set of integers that can be sorted. This means that the algorithm can be used to sort data sets that are very large.
Homomorphic sorting is also more efficient than other sorting algorithms. This is because the data can be mapped to a set of integers that can be sorted. This means that the algorithm does not have to waste time on comparisons that are not needed.
Overall, homomorphic sorting is a more efficient sorting algorithm that can be used to sort data that is not in a uniform format. This algorithm has many advantages that make it more efficient than other sorting algorithms.
What are the limitations of homomorphic sorting?
There are many limitations to homomorphic sorting. One major limitation is its reliance on data that is already sorted. This can be a problem when sorting data that is not well-organized, or when the data is constantly changing. Additionally, homomorphic sorting is not very efficient when sorting large amounts of data. It can also be difficult to implement homomorphic sorting in distributed systems.
Frequently Asked Questions
What are the different types of sorting algorithms?
There are five main sorting algorithms: Selection Sort, Bubble Sort, Insertion Sort, Recursive Bubble Sort, and Recursive Insertion Sort. Each algorithm sorts a list of objects in a specific way.
What is homomorphic encryption and how does it work?
Homomorphic encryption is a method of representing and processing data that allows for an algorithm to be executed on encrypted data without decrypting it, allowing it to be treated as if it were data that is not encrypted. This is useful in privacy-critical situations where it is important to keep the data confidential. Homomorphic encryption has been used in IBM's Project Jasper, which has been developing tools that can process Massive Data Sets using Conjoint Analysis. Conjoint Analysis is a technique that uses large sets of data to identify patterns or correlations between variables. This could be used, for example, to understand how customers make purchases or how people vote.
What is seal homomorphic encryption library?
SEAL homomorphic encryption library is software that allows additions and multiplications on encrypted integers or real numbers. Encrypted comparison, sorting, or regular expressions aren't often feasible to evaluate on encrypted data using this technology.
What are the different types of sort algorithms?
Merge sort, heap sort, and quicksort are the three most popular sorting algorithms. Other algorithms include linear search (a fast algorithm to locate a particular item in a sorted list), bubble sort (a simple algorithm that compares adjacent items and swaps them if they are out of order), insertion Sort (you can insert an element into a sorted list at any position and the relocation will cause the list to become sorted), and selection Sort (the first compare operation selects the lowest value for one of the elements and then compares this value with all the other values).
What is a stable sort algorithm?
A stable sort algorithm sorts repeated elements in the same order that they appear in the input.
Sources
- https://learn.microsoft.com/en-us/azure/architecture/solution-ideas/articles/homomorphic-encryption-seal
- https://ieeexplore.ieee.org/document/9222337/
- https://www.researchgate.net/publication/265814080_Accelerating_Sorting_of_Fully_Homomorphic_Encrypted_Data
- https://www.quora.com/How-do-I-implement-a-homomorphic-encryption-scheme
- https://crypto.stackexchange.com/questions/30812/how-about-a-homomorphic-integer-sorting-in-a-mpc-context
- https://www.tutorialspoint.com/homomorphism
- https://eprint.iacr.org/2015/981.pdf
- https://blogs.mathworks.com/steve/2013/06/25/homomorphic-filtering-part-1/
- https://www.mailmech.co.za/article/3-benefits-sorting-machines/
- https://www.digitalreviewpro.com/post/how-does-homomorphic-encryption-work-and-what-are-its-benefits
- https://www.researchgate.net/publication/297758932_Updates_on_Sorting_of_Fully_Homomorphic_Encrypted_Data
- https://research.aimultiple.com/homomorphic-encryption/
- https://eprint.iacr.org/2022/1564
- https://eprint.iacr.org/2015/274.pdf
Featured Images: pexels.com