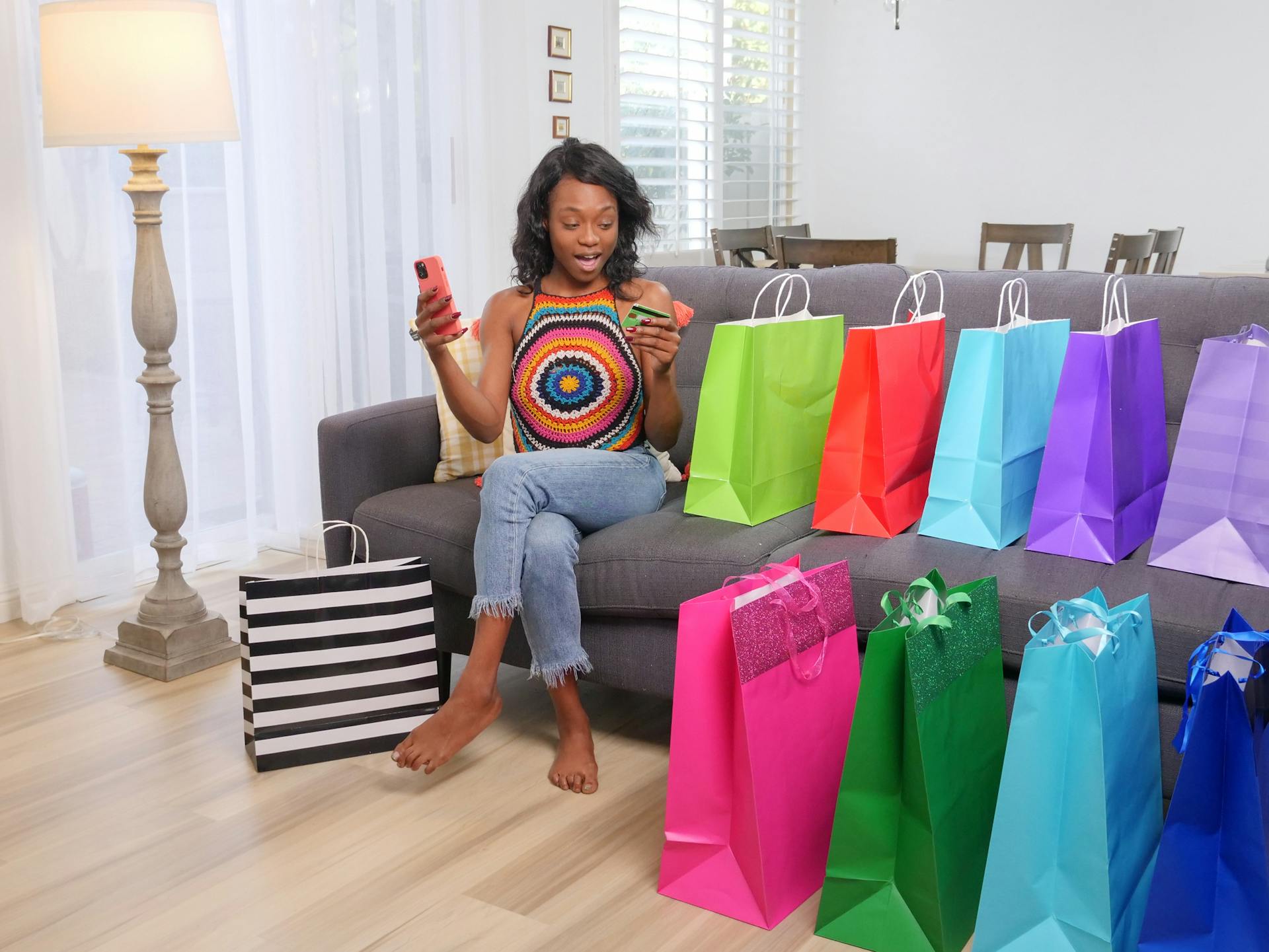
The Luhn algorithm is a widely used method for validating credit card numbers. It's a simple yet effective way to check if a card number is valid or not.
This algorithm works by summing up the digits of the card number, doubling the digits in the odd positions from right to left, and then subtracting the total from a multiple of 10.
The Luhn algorithm checks for the presence of invalid characters, such as letters, and also verifies the length of the card number. A valid card number should have a length of 13, 15, or 16 digits.
A unique perspective: Valid Credit Card Number with Csc
What Is the Luhn Algorithm?
The Luhn Algorithm is a simple yet effective check sum formula used to validate identification numbers, including credit card numbers. It was developed by German computer scientist Hans Peter Luhn in 1954.
The algorithm is also known as the "modulus 10 algorithm" and is used by most credit cards, as well as many government identification numbers. It's a simple method of distinguishing valid numbers from mistyped or otherwise incorrect numbers.
Expand your knowledge: Credit Card Bin Number
The Luhn Algorithm can quickly detect input errors, typos, or invalid numbers, making it a crucial step in processing credit card payments or identifying users. This is achieved by calculating a control digit, which is a single digit that makes it possible to check the other digits.
Here's a step-by-step breakdown of the Luhn Algorithm:
This algorithm is not intended to be a cryptographically secure hash function, but rather a simple method to detect accidental errors rather than defend against malicious attacks.
Introduction
The Luhn algorithm is a method used to verify the validity of credit card numbers. It's a widely used algorithm in the industry.
The algorithm was specifically designed to check if a credit card number is valid, and it's used by many companies to verify card numbers.
The purpose of this algorithm is to detect errors in card numbers, such as typos or incorrect digits.
Related reading: Verify Credit Card Number List
About Luhn's Method
The Luhn algorithm was developed by German computer scientist Hans Peter Luhn in 1954. It's a check sum formula used to validate identification numbers, including credit card numbers.
Most credit cards and many government identification numbers use the Luhn algorithm as a simple method to distinguish valid numbers from mistyped or incorrect numbers. It's not intended to be a cryptographically secure hash function, but rather to detect accidental errors.
The Luhn algorithm is primarily a safeguard against simple user errors, not malicious attacks. It's a simple method to distinguish valid numbers from random digits.
The algorithm is used to validate primary account numbers, including credit card numbers. It's a quick and efficient way to check numbers for errors before processing critical operations.
Here are the steps to verify a number with the Luhn algorithm:
- Start from the last right digit of the number
- Multiply every other digit by 2
- If the result of the doubling ends up with a two-digit number, add those two digits together
- Calculate the sum of all digits found
- The control digit is equal to (10 - (sum modulo 10)) modulo 10
The Luhn algorithm can detect almost any single-digit error, but it has some weaknesses. For example, it can't detect certain permutations of digits, such as 09 and 90, or double errors like 22 from/to 55.
How to Use the Luhn Algorithm
The Luhn algorithm is a simple yet effective way to validate credit card numbers. It's used by major credit card companies like MasterCard, American Express, and Visa to detect errors and invalid numbers.
To verify a number with Luhn, start from the end of the number and work your way backwards. Double every other digit, and if the result is a two-digit number, add those two digits together. For example, the number 8530 would be calculated as follows: 3*2=6, 5 stays the same, 8*2=16 becomes 1+6=7, and 0 stays the same. The sum is 6+5+7=18, which is not divisible by 10, so the number is invalid.
The Luhn algorithm can detect almost any single-digit error, making it a useful safeguard against simple user errors. However, it's not intended to protect against malicious attacks.
Here's a step-by-step guide to implementing the Luhn algorithm:
1. Write down the credit card number.
2. Double every other digit, starting from the first number.
3. If the result of the doubling ends up with a two-digit number, add those two digits together.
4. Check if the final sum is divisible by 10. If it is, the credit card is valid. If not, it's invalid or fake.
For example, the credit card number 4417 1234 5678 9113 would be calculated as follows:
4(x2) 4 1(x2) 7 1(x2) 2 3(x2) 4 5(x2) 6 7(x2) 8 9(x2) 1 1(x2) 3
The doubled numbers result in: 8 2 2 6 10 14 18 2
The final sum is 8+2+2+6+1+0+1+4+1+8+2=45, which is not divisible by 10, so the number is invalid.
Suggestion: 19 Digit Credit Card Number
In Python, you can implement the Luhn algorithm using the following code:
```python
def luhn_algorithm(credit_number):
# Calculate the Luhn key
sum = 0
for i, digit in enumerate(credit_number):
if i % 2 == 0:
digit = int(digit) * 2
if digit > 9:
digit -= 9
sum += digit
# Check if the final sum is divisible by 10
return sum % 10 == 0
```
Note that this code assumes the input is a string of digits.
If this caught your attention, see: 15 Digit Credit Card Number
Luhn Algorithm Limitations
The Luhn Algorithm isn't foolproof, and it has some limitations that you should be aware of.
One weakness is that it can't detect certain permutations of digits. For example, swapping a 0 with a 9 and a 9 with a 0 in a number will result in the same checksum.
The algorithm also fails to detect double errors, like swapping a 2 with a 5 and then back again, or swapping a 3 with a 6 and then back again.
Another issue is that the presence or absence of leading zeros doesn't affect the checksum. This can be both an advantage and a disadvantage.
For instance, the numbers 000123 and 123 have the same checksum, which can be useful in some cases, but also means that the algorithm can't always detect errors in leading zeros.
Consider reading: Numbers on Back of Credit Card
Credit Card Data Components
The credit card number is made up of several key components that work together to identify the card and its owner.
The bank identification number (BIN) is the first six digits of the credit card number and is used to identify the issuer of the card.
The account number is the string of numbers between the BIN and the check digit, and it's used to identify the individual account number.
The check digit is the last digit of the credit card number, and it's used to validate the authenticity of the card number based on the Luhn algorithm.
Here are the three components of a credit card number in a quick reference list:
- Bank Identification Number (BIN): The first six digits
- Account Number: The number between the BIN and the check digit
- Check Digit: The last digit, used for validation
Implementing the Luhn Algorithm
The Luhn algorithm is a simple yet effective way to validate credit card numbers, and it's widely used in the industry. It can detect almost any single-digit error, such as someone mistyping numbers when they put in their credit card.
To implement the Luhn algorithm, you'll need to follow a few steps. First, you'll need to write down the credit card number, and then you'll need to double every other digit starting from the first number. For example, if the credit card number is 4417 1234 5678 9113, you'll double every other digit to get 8 2 2 6 10 14 18 2.
The Luhn algorithm will then sum up all the digits, including the doubled ones, and check if the sum is divisible by 10. If it is, the credit card number is valid; otherwise, it's invalid. Most credit cards and many government identification numbers use this check as a simple method to distinguish valid numbers from random digits.
Here's a simple example of how you can implement the Luhn algorithm in Python:
```python
def check_luhn(card_no):
n_digits = len(card_no)
n_sum = 0
is_second = False
for i in range(n_digits - 1, -1, -1):
d = int(card_no[i])
if is_second:
d = d * 2
if d > 9:
d = d % 10 + d // 10
n_sum += d
is_second = not is_second
return (n_sum % 10 == 0)
card_no = "79927398713"
if check_luhn(card_no):
print("This is a valid card")
else:
print("This is not a valid card")
```
Note: This code is a simplified version of the Luhn algorithm and does not include error checking or handling.
Consider reading: 800 Number for Chase Credit Card
Implementing Luhn Algorithm in Python
Implementing Luhn Algorithm in Python is a straightforward process.
You can start by changing the string credit card number into a list datatype. This is because the algorithm requires you to work with individual digits.
To implement the Luhn algorithm, you can follow these steps: remove the last digit (check digit), reverse the remaining digits, double digits at even indices, subtract 9 if over 9, add the check digit back to the list, sum all digits, and check if the sum is divisible by 10.
Here's a step-by-step guide to implementing the Luhn algorithm in Python:
1. Change string to list datatype
2. Remove last digit (check digit)
3. Reverse remaining digits
4. Double digits at even indices
5. Subtract 9 if over 9
6. Add the check digit back to the list
7. Sum all digits
8. If the sum is divisible by 10, the number is valid; otherwise, it's invalid.
You can use Python libraries like luhn or fast-luhn to make the process easier.
Worth a look: How to Check Full Credit Card Number
C++
In the C++ implementation of the Luhn algorithm, the `checkLuhn` function takes a `const string&` as input, which is the card number to be checked.
The function initializes two variables, `nDigits` and `nSum`, to keep track of the number of digits in the card number and the sum of the digits, respectively.
The function uses a boolean variable `isSecond` to alternate between doubling and not doubling the digits in the card number.
The doubling of digits is handled by multiplying the digit by 2 and adding the two digits together if the result is greater than 9.
The function returns `true` if the sum of the digits is divisible by 10, indicating that the card number is valid.
Here is a step-by-step breakdown of the `checkLuhn` function:
- Initialize `nDigits` to the length of the card number
- Initialize `nSum` to 0
- Initialize `isSecond` to false
- Loop through the digits in the card number from right to left
- For each digit, subtract the ASCII value of '0' to get the actual digit value
- If `isSecond` is true, double the digit by multiplying it by 2
- Add the two digits together if the doubled digit is greater than 9
- Add the resulting sum to `nSum`
- Toggle `isSecond` to true or false
- After looping through all digits, return `true` if `nSum` is divisible by 10, indicating a valid card number.
Sources
- https://www.dcode.fr/luhn-algorithm
- https://www.groundlabs.com/blog/anatomy-of-a-credit-card/
- https://www.geeksforgeeks.org/luhn-algorithm/
- https://stackoverflow.com/questions/4514430/credit-card-checksums-and-validations-that-do-not-require-connection-to-the-fina
- https://dev.to/seraph776/validate-credit-card-numbers-using-python-37j9
Featured Images: pexels.com