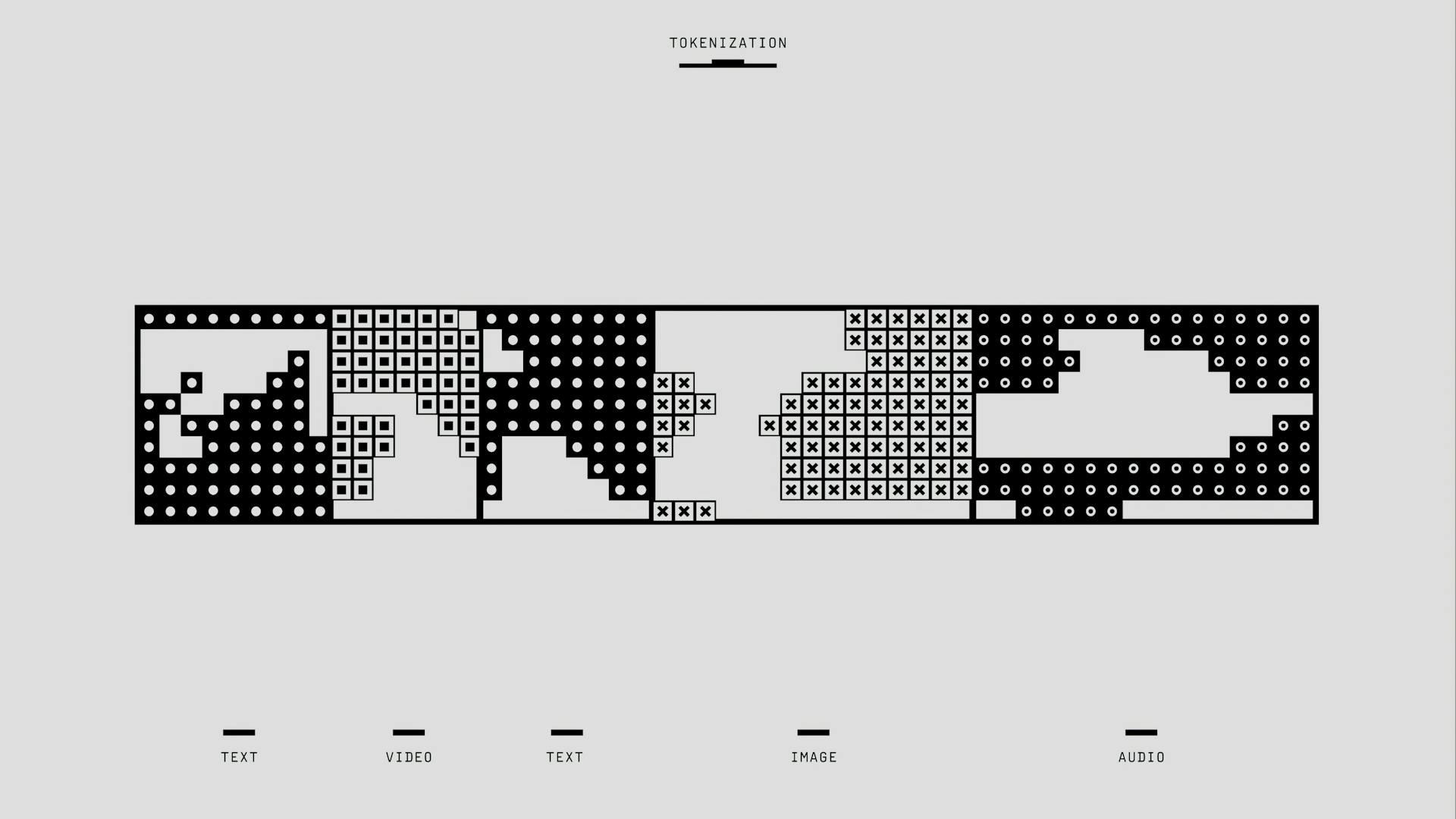
To create an ERC20 token, you'll need to write a smart contract in Solidity, a programming language used for Ethereum development.
Solidity is a contract-oriented, high-level language that's statically typed and supports object-oriented programming features.
The ERC20 token standard is an open standard that defines a set of rules for creating tokens on the Ethereum blockchain.
To deploy your ERC20 token on Ethereum, you'll need to use a development environment like Remix or Truffle Suite.
These tools provide a user-friendly interface for writing, deploying, and testing smart contracts.
A fresh viewpoint: Bitcoin Atm Tampa - Coinhub
Understanding ERC20 Tokens
ERC20 tokens are blockchain-based assets that can be sent and received, and have value. They are similar to Bitcoin and Litecoin in many aspects, but instead of running on their own blockchain network, ERC20 coins run on Ethereum's blockchain network and use gas as the transaction fee.
The ERC20 token standard ensures that all tokens have the same properties, including being fungible, meaning any one token is exactly equal to any other token. This standard also means that no tokens have special properties or rights associated with them.
Additional reading: Stellar Blockchain Token
Here are the API methods and events that a token must implement to follow the ERC20 token standard:
- totalSupply - a method that defines the total supply of your tokens, and stops creating new tokens when the total supply limit is reached.
- balanceOf - a method that returns the number of tokens a wallet address contains.
- transfer - a method that transfers a certain amount of tokens from the total supply and sends it to a user.
- transferFrom - a transfer method that transfers ERC20 tokens between users
- approve - verifies whether a smart contract is allowed to allocate a certain amount of tokens to a user, considering the total supply.
- allowance - checks if a user has enough balance to send a token to another user.
What is a Token?
A token is a blockchain-based asset that can be sent or received and has value.
ERC-20 tokens are similar to Bitcoin and Litecoin in many aspects, but instead of running on their own blockchain network, they run on Ethereum's blockchain network and use gas as the transaction fee.
ERC-20 tokens are standardized, which means they have a common list of rules and functions. This includes how the tokens can be transferred, how transactions are approved, how users can access data about a token, and the total supply of tokens.
The total supply of a token is defined by the totalSupply method, which stops creating new tokens when the total supply limit is reached. This method is a mandatory function of the ERC-20 standard.
The balanceOf method returns the number of tokens a wallet address contains. This information is essential for users to know their token balance.
Check this out: Bitcoin Atm Milwaukee - Coinhub
Tokens can be transferred from one account to another as payment, similar to cryptocurrencies like Bitcoin. The transfer method takes a certain amount of tokens from the total supply and gives it to a user.
Here are the mandatory functions of the ERC-20 standard:
- totalSupply: A method that defines the total supply of your tokens.
- balanceOf: A method that returns the number of tokens a wallet address has.
- transfer: A method that takes a certain amount of tokens from the total supply and gives it to a user.
- transferFrom: Another type of transfer method that is used to transfer tokens between users.
- approve: This method verifies whether a smart contract is allowed to allocate a certain amount of tokens to a user, considering the total supply.
- allowance: This method checks if one user has enough balance to send a certain amount of tokens to another.
Why Tokenize Your Business?
Tokenizing your business can bring numerous benefits. Creating an ERC20 token offers ease, interoperability with various platforms, and access to a well-established ecosystem of decentralized applications (DApps) and exchanges, which can enhance liquidity and utility.
The ERC20 token standard is highly popular in the market, making fundraising through an Initial Coin Offering (ICO) quite comfortable. This is a significant advantage for businesses looking to raise capital.
The Ethereum-based fungible token is also relatively easy and cost-effective to create, thanks to the use of smart contracts that are secure and difficult to break. This security feature gives investors confidence in the token.
A standardized token like ERC20 increases liquidity due to the trust factor and attracts more investors. This is because the token is easily recognizable and understood by the market.
See what others are reading: Bitcoins Biggest Investors
ERC20 tokens can be an excellent source of revenue generation for entrepreneurs and investors due to their extensive utility. This is one of the main reasons businesses choose to tokenize their business.
Here are some key benefits of tokenizing your business:
- Increased liquidity due to the trust factor and more investors
- Excellent source of revenue generation for entrepreneurs and investors
- Extensive utility, making it a valuable asset
Creating an ERC20 Token
Creating an ERC20 Token is a straightforward process that can be completed in a few simple steps. You can use a service like CoinFactory, which optimizes the contract deployment process and allows you to create your ERC20 token without requiring knowledge of the Solidity language.
To get started, you'll need to understand the basics of the ERC20 standard, which is the most popular way to create fungible cryptocurrencies on Ethereum and EVM-compatible blockchains. This standard allows builders and creators to develop digital assets for their protocol, marketplace, metaverse, or community.
There are three main templates to choose from: Standard Token, Essential Token, and Taxable Token. Each template comes with its own set of features, so you can choose the one that best fits your project's needs.
A fresh viewpoint: The Bitcoin Standard Goodreads
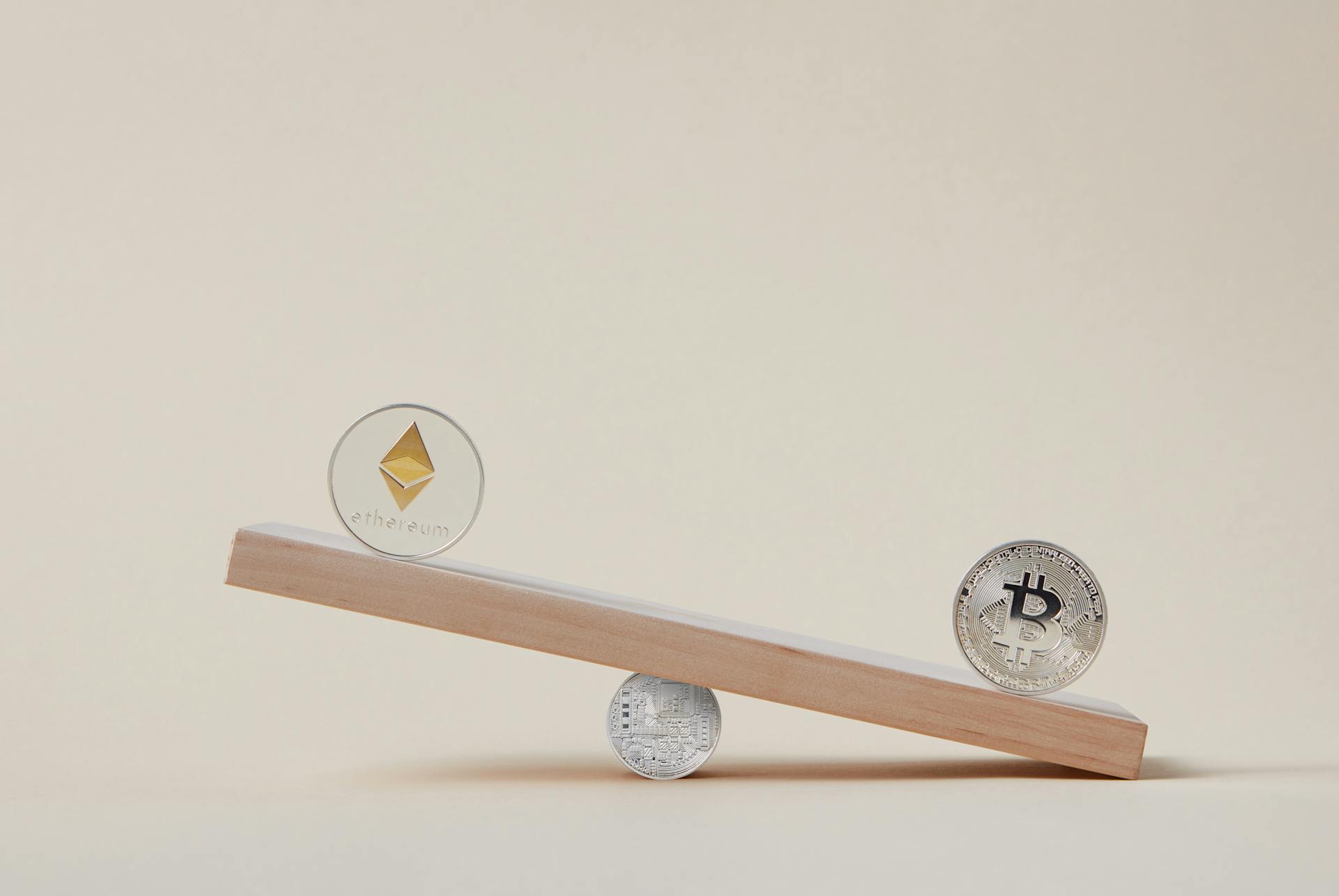
Here's a brief overview of each template:
Once you've chosen your template, you'll need to deploy your contract on the Ethereum network. This can be done using a service like CoinFactory, which allows you to deploy your contract with minimal network fees. You'll also need to verify your contract code on Etherscan, which is a quick and easy process.
With these simple steps, you can create your own ERC20 token and start building your digital assets. The estimated time to complete this guide is around 15 minutes, making it a quick and efficient process.
Worth a look: How to Create a Smart Contract
Preparation and Setup
To create an ERC20 token, you'll need to set up the environment with Remix. This involves navigating to remix.ethereum.org, creating a new file called "Token.sol", and adding the License-identifier and pragma to specify the Solidity version.
You'll also need to import the ERC20 token contract from OpenZeppelin, which is an essential step in understanding how to create your own cryptocurrency. To do this, you'll need to create a new folder and install Hardhat and its dependencies, which can be done by running `npx hardhat` in your terminal.
A unique perspective: An Airdrop of New Cryptocurrency following a Hard Fork Is
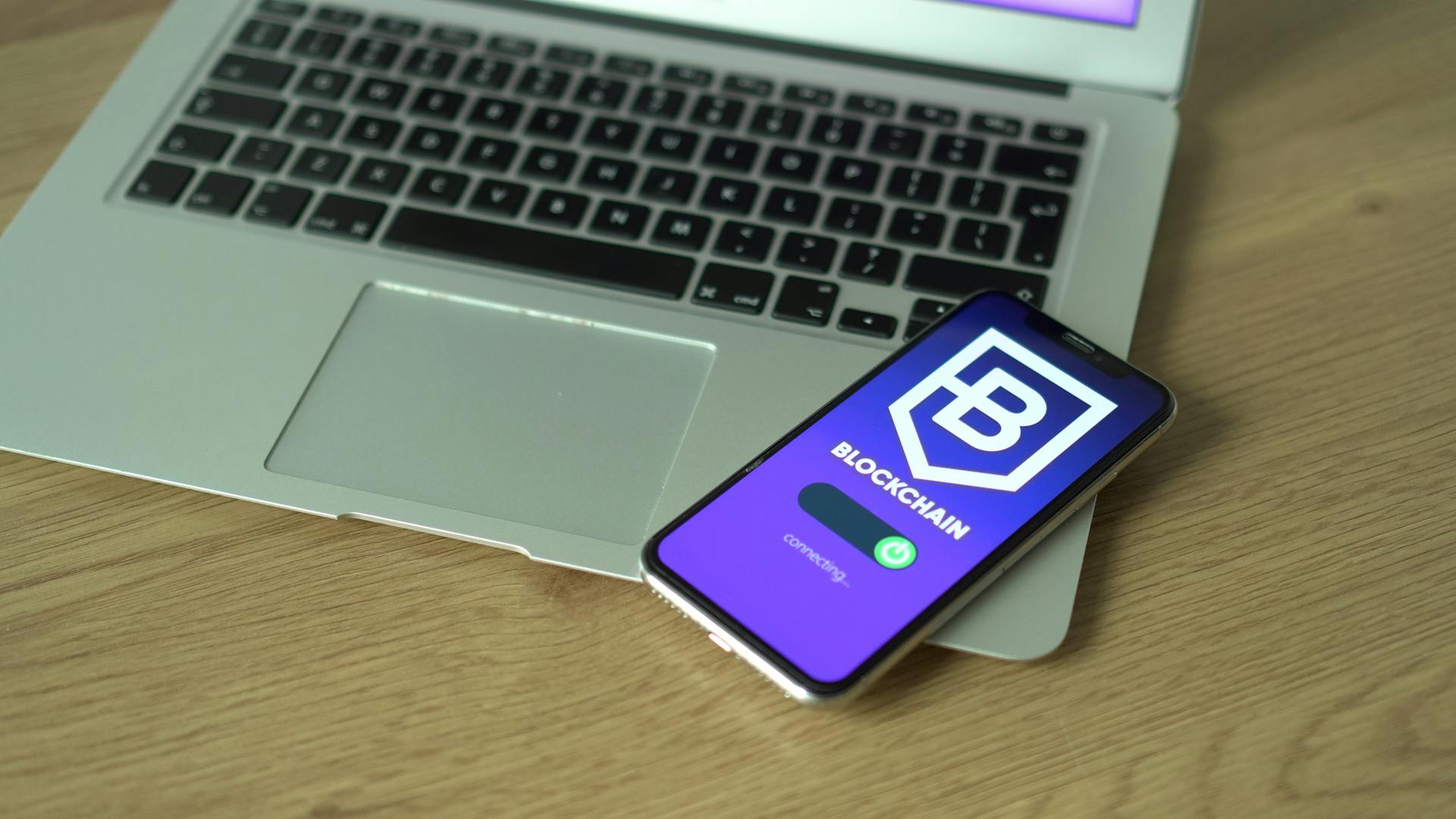
To connect your wallet, you'll need to start by connecting your wallet to the CoinFactory Generator page, where you'll need to connect the account that will be the owner of the smart-contract and where the initial token supply will be initiated. You can use popular options like Metamask and Trust Wallet if you don't have a wallet already.
You have two options to deploy your smart contract: spin up your own Ethereum node or use a third-party node provider like Alchemy or Infura. Using a third-party node provider is usually easier and more cost-effective, especially for development purposes.
On a similar theme: How to Make Money with Smart Contracts
Set Up Local Blockchain Dev Environment
To set up your local blockchain developer environment, you'll need to install Hardhat, a popular development environment for Ethereum smart contracts. Hardhat is available on the official website.
For this tutorial, we'll assume you have VSCode already installed on your device. Create a new folder to hold your project files.
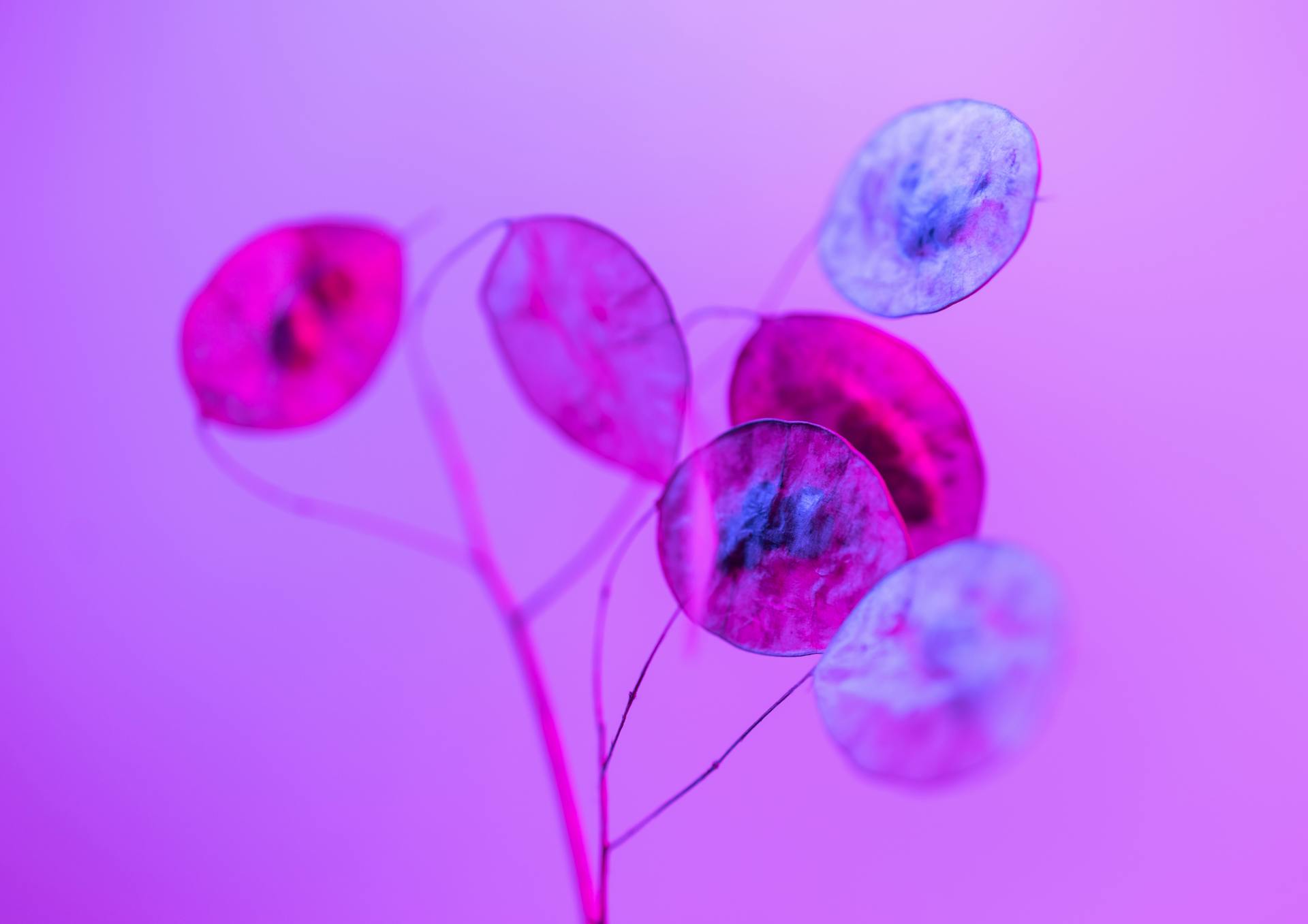
You'll need to install Hardhat and its dependencies to compile and test your smart contract. Check out the official Hardhat documentation to learn more about these dependencies.
To initialize the Hardhat setup wizard, run the command in your terminal or command prompt. Select "Create a basic sample project" and leave the default root. Add a .gitignore to your project.
This will populate your project directory with the default Hardhat files and folders. If you're new to some of these files, check out the create your first DApp with Solidity guide for more information.
You'll need to connect your smart contract to a running blockchain instance. There are two options: spin up your own Ethereum node or use a third-party node provider like Alchemy or Infura.
Here are the two options:
- Spin up your own Ethereum node.
- Use a third-party node provider like Alchemy or Infura.
Running your own node is usually discouraged due to the high maintenance and engineering effort required. In this case, we'll use Alchemy as a Mumbai Node provider, as it's completely free and easy to set up.
To use Alchemy, navigate to alchemy.com and create a new account. Once logged in, click on "Create App" and fill in the required fields, including Name, Description, Environment, Chain, and Network.
Connect Your Wallet
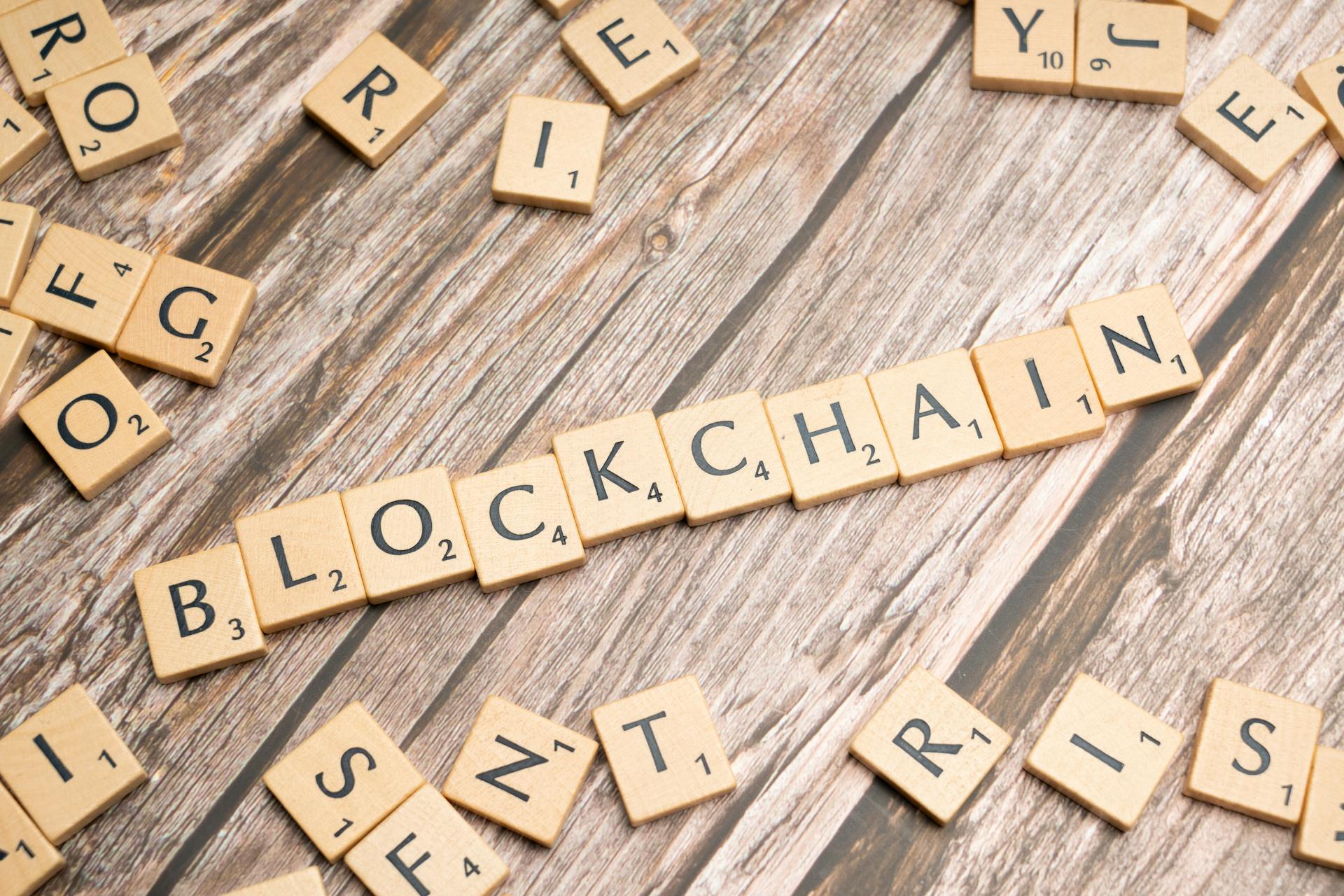
Connecting your wallet is a crucial step in the process. You need to connect the account that will be the owner of the smart-contract and where the initial token supply will be initiated.
To start, you'll need to connect your wallet to the CoinFactory Generator page. If you don't have a wallet, consider popular and secure options like Metamask and Trust Wallet.
Why to Approach a Development Company?
Approaching a development company to create an ERC20 token is a great idea. It ensures professional expertise and security, reducing the risk of errors and vulnerabilities in your token's smart contract.
With a development company, you tap into experience for customization and optimizing your token for specific use cases. This is especially helpful for aspiring startups developing ERC20 token ideas.
A renowned Ethereum token development services providing company like Coinsclone can help create ERC20 tokens with ease. We have enough hands-on experience in the industry to complete the token development process with ease.
Related reading: P2p Crypto Exchange Development
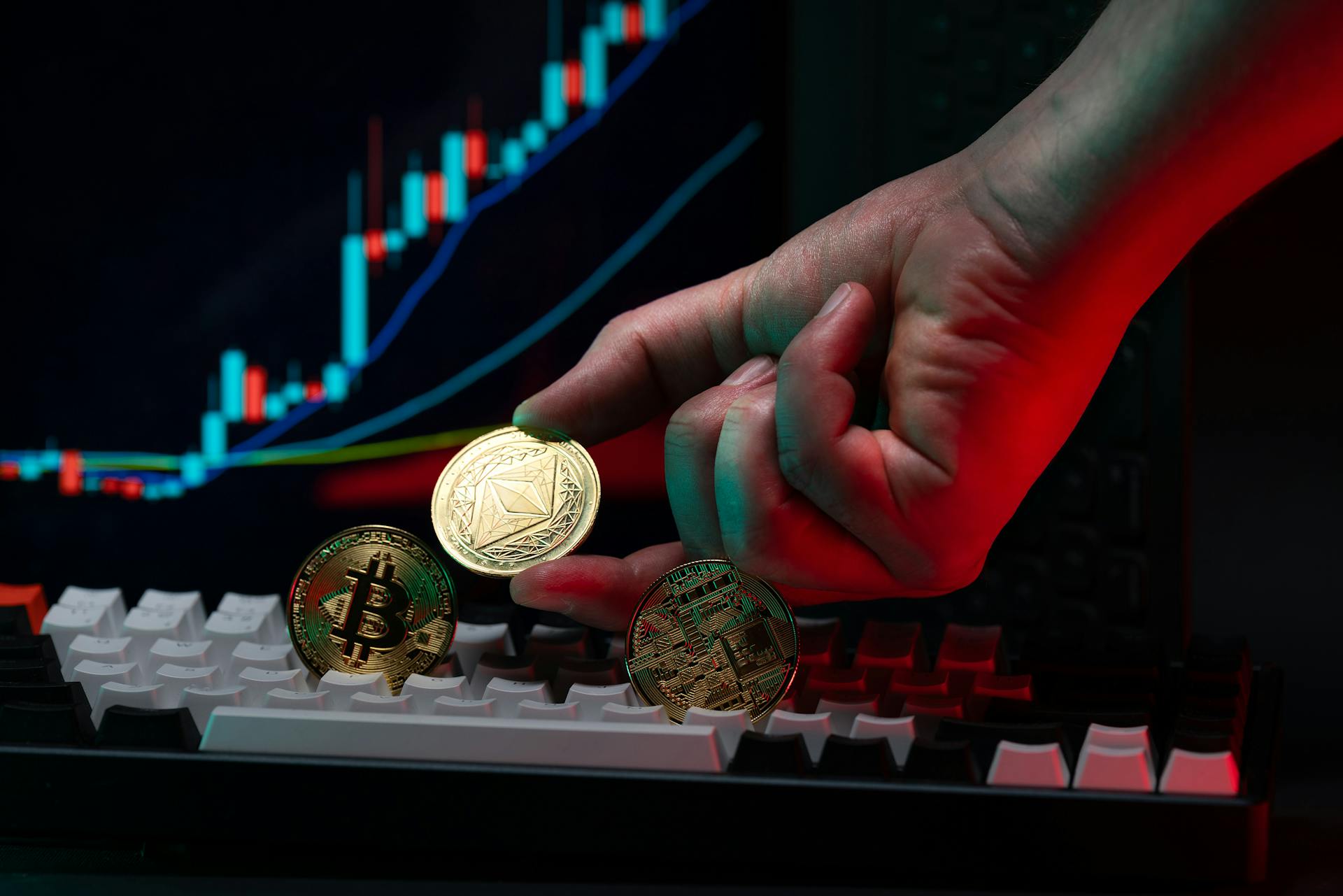
Approaching a Token development company also helps with different services apart from just the token creation. Here are some of the add-on works that are available:
- Setting Initial Supply
- No of Issues
- Setting Fractions
- Deploy Contracts
- Mintable
- Burnable
- Pausable
- Generate Tokens
- Validate Contract
- High-end security features
These services help reduce the risk involved in token development, giving startups or entrepreneurs with token development ideas peace of mind.
Smart Contract Development
Smart Contract Development involves creating a smart contract using a programming language like Solidity. The foremost need for creating a smart contract is Solidity language and core function integration.
Popular solidity languages used to frame smart contracts include Python, Javascript, and C++. Depending on your business needs and convenience, you can choose any of the languages.
To create a robust and secure implementation, many developers opt to use OpenZeppelin's ERC20 token standard, which provides a well-tested, community-audited library of reusable smart contracts.
Here are some core functions that are typically included in an ERC-20 token contract:
- Total Supply – The total Supply function signifies the overall number of tokens that can circulate.
- Balance of – The Balance of function reveals the token owner’s account balance, indicating the number of tokens held by a specific address.
- Transfer – The Transfer function automates the token transfer to a specified address.
- Transfer From – The Transfer From function facilitates receiving tokens from a specific address and permits transfers from an account not directly involved in the transaction.
- Approve – Approve function grants users permission to withdraw or spend tokens from an account.
Setting Up Remix Environment
To set up the Remix environment, navigate to remix.ethereum.org. This is the website where we'll be creating and deploying our ERC20 token.
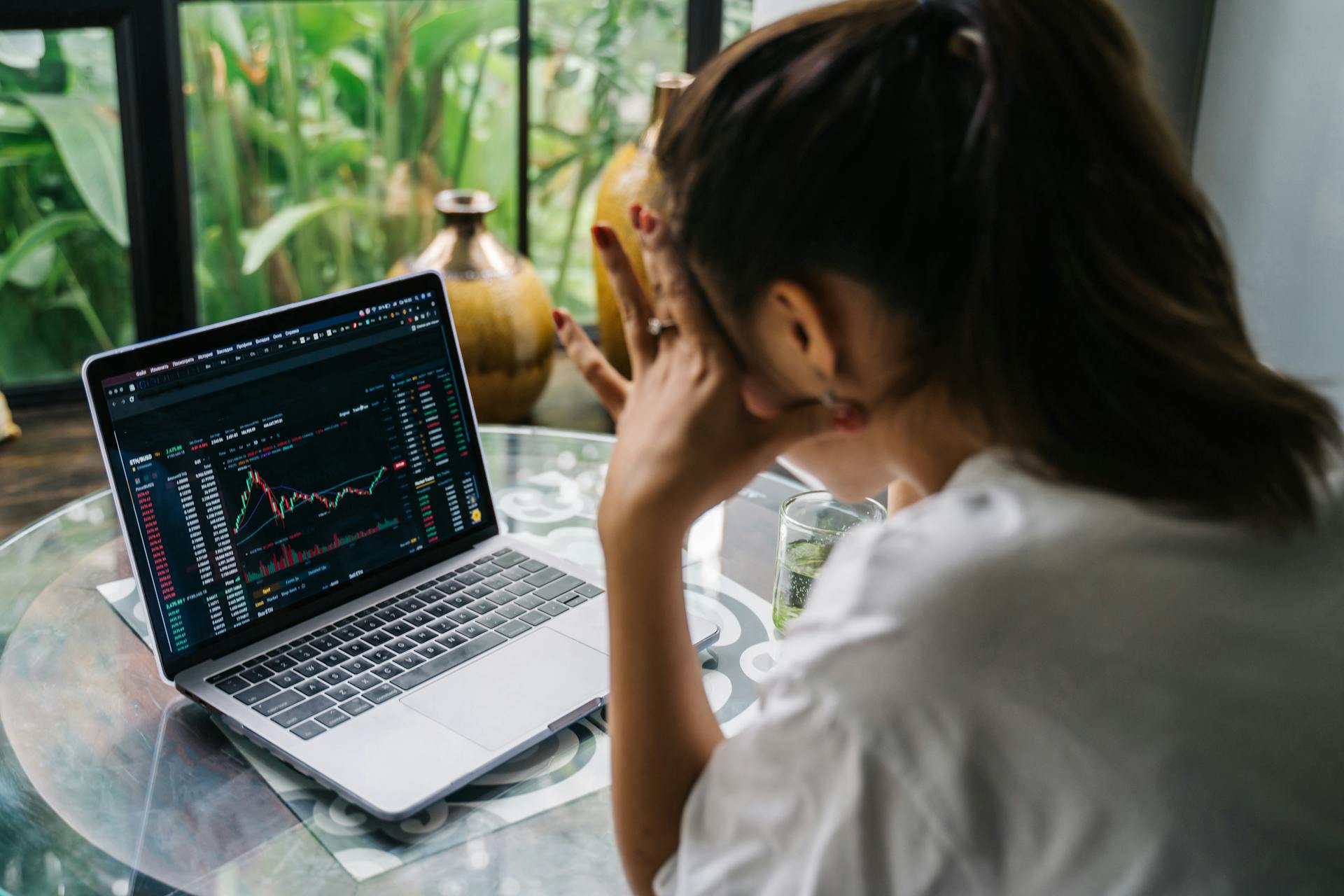
The first step is to open the contracts folder and create a new file called "Token.sol". This file is where we'll write the code for our token.
It's mandatory to add the license-identifier and the pragma to specify the Solidity version the compiler should use to build our code. This is a standard practice when creating a new Solidity file.
REMIX is an easy-to-use, free, and decently error-prone IDE, making it a great choice for creating and deploying smart contracts. Its Solidity compatible feature is particularly useful for building and compiling our code.
Check this out: How to Find New Crypto Coins Early
Write Smart Contract
To write a smart contract, you'll need to use a programming language like Solidity, which is similar to Java, JavaScript, or C and C++. Open up your code editor, navigate to the /contracts folder, and create a new .sol file with the same name as your token. For example, if your token is called Web3Token, your contract file should be named Web3Token.sol.
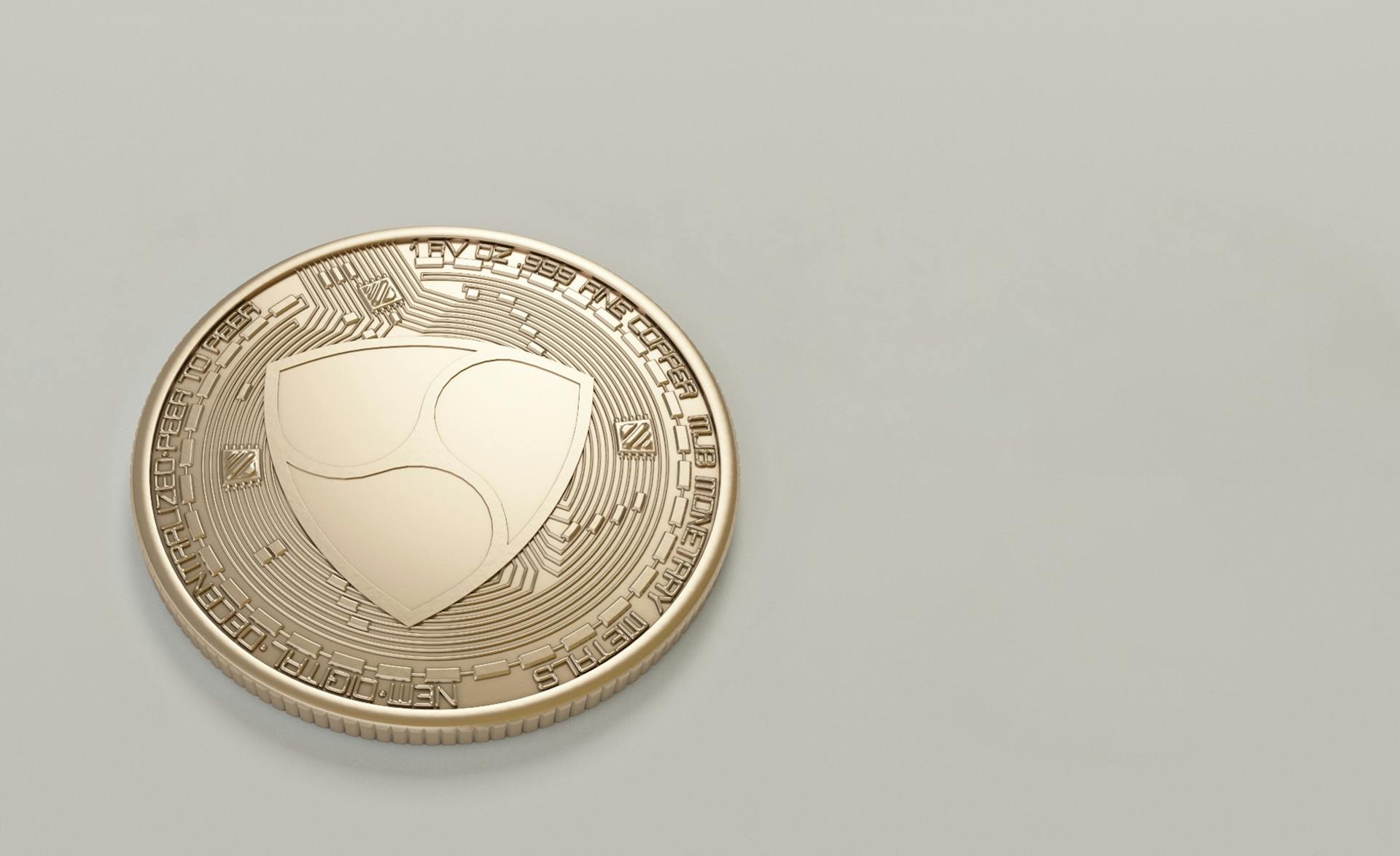
Solidity is a contract-oriented language, which means it's designed specifically for writing smart contracts. You can import pre-existing contracts, like the ERC20 contract from OpenZeppelin, to save time and ensure security.
To create a robust and secure implementation, many developers opt to use OpenZeppelin's ERC20 token standard. You can import the ERC20 contract and use its functions, eliminating the need to write the whole ERC20 interface yourself.
Here are some popular languages used for smart contract development:
- Python
- Javascript
- C++
- Solidity
Note that you can choose any language that suits your business needs and convenience.
The ERC20 contract from OpenZeppelin is a well-tested, community-audited library of reusable smart contracts. It provides a reliable and secure framework for ERC20 tokens, making it a preferred choice for ensuring compliance and security in token development.
To write a smart contract, you'll need to specify the SPDX-License-Identifier comment, which states the license under which the contract is released. You'll also need to use the pragma directive to specify the compiler version to use.
Here's an example of how to write a smart contract using the OpenZeppelin ERC20 contract:
Discover more: Openzeppelin Erc20
```
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/SafeERC20.sol";
contract MyToken is ERC20("MyToken", "MTK") {
constructor() ERC20("MyToken", "MTK") {
_mint(msg.sender, 1000000 * 10 ** 18);
}
}
```
This contract inherits the ERC20 contract and initializes the token with a name ("MyToken") and a symbol ("MTK"). The constructor function mints an initial supply of tokens, which is 1 million in this example.
Remember to customize the smart contract with your own details, such as updating the token name and symbol by modifying the ERC20("MyToken", "MTK") line.
Explore further: Crypto Coin Names
Core Functions
In smart contract development, the core functions of a token are crucial for its functionality. The total supply of tokens is set by the smart contract, allowing for a predetermined limit on the number of tokens that can circulate.
The balance of a token owner's account is easily accessible through the Balance of function, which displays the number of tokens held by a specific address. This information is vital for tracking token ownership and balances.
The Transfer function automates the process of sending tokens to a specified address, ensuring that the sender has sufficient tokens for the intended transfer. This function is a fundamental aspect of token transfer.
The Transfer From function allows users to receive tokens from a specific address and enables transfers from accounts not directly involved in the transaction. This feature enhances the flexibility of token transfer.
The Approve function grants users permission to withdraw or spend tokens from an account, giving them control over their token holdings.
Expand your knowledge: How to Transfer from Crypto Wallet to Fiat Wallet
Deployment and Testing
To deploy your ERC20 token, you'll need to compile the Token.sol code on REMIX and populate the artifacts folder with your contract's ABI and binary version. This will allow you to deploy it on the blockchain.
You can also use Hardhat to deploy your token, which involves renaming the sample-script.js file to "deploy.js" and replacing its content with the necessary code. This code will deploy the token, passing the total supply value to the Token constructor.
To test and audit your ERC20 token, it's crucial to identify and rectify any mistakes, errors, or bugs in the contract. This will ensure the hassle-free working of the tokens.
For another approach, see: Bitpay Qr Code
Deploy on Polygon Mumbai
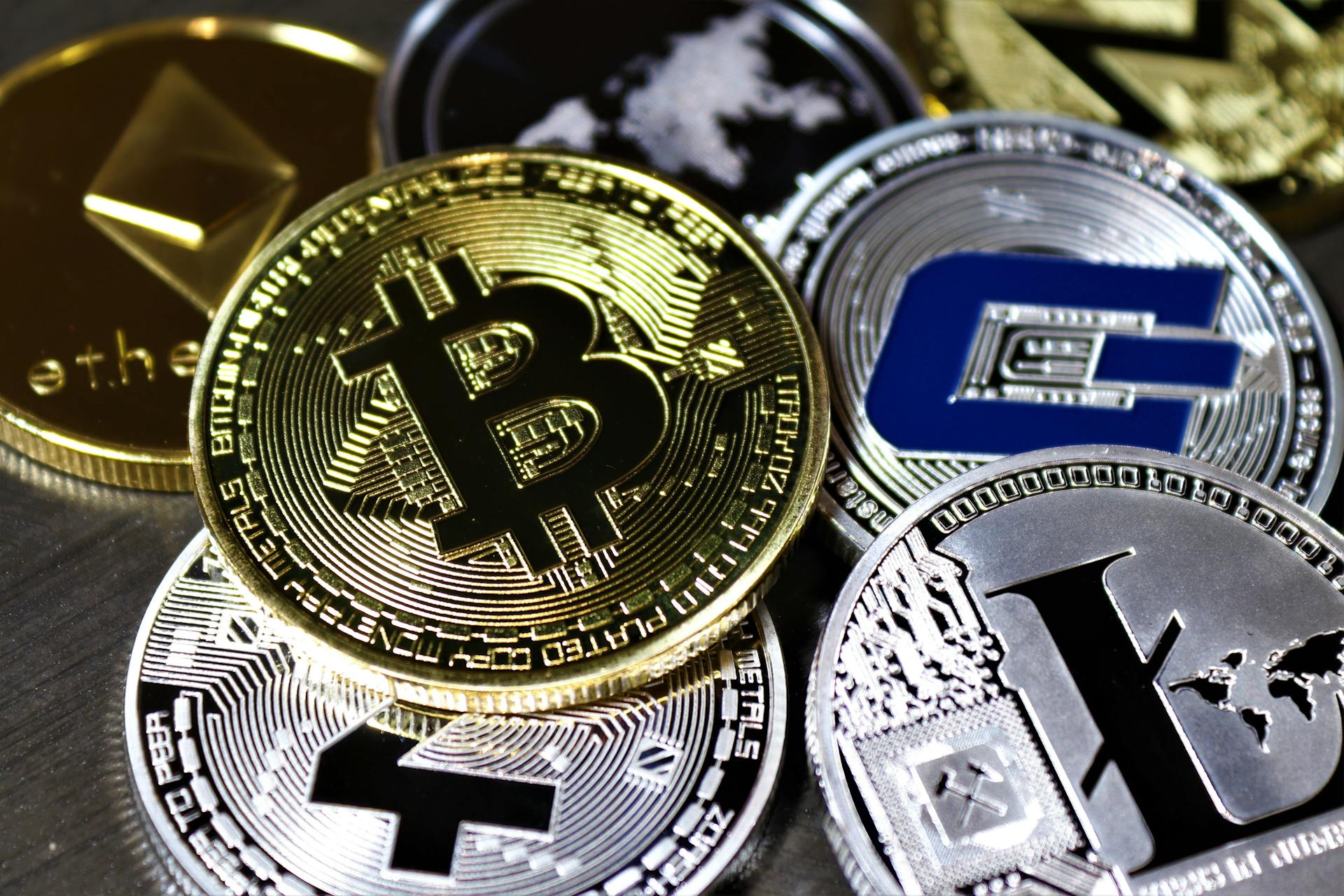
To deploy on Polygon Mumbai, you'll need to use the Mumbai network, which is specified in the hardhat.config.js file. This will allow you to deploy your token contract.
You'll need to run your deploy script using the Mumbai network, which should take a couple of seconds to complete. After that, you should see the Token Address on the Mumbai network logged in the terminal.
You've just deployed your first Token to the Blockchain! If everything worked as expected, you should now see your newly created cryptocurrency and the minted amount of tokens in your MetaMask wallet or interact with them in your DApp.
First, though, you'll need to tell MetaMask how to find your token. To do this, you'll need some MATIC to pay for the Gas fees, but don't worry, you won't spend a penny, as you're deploying on a testnet.
You can compile your Token Contract by running a specific command in the terminal, which will create a new folder called artifacts and populate it with the compiled version of your Token. This will allow the getContractFactory() function in deploy.js to grab your contract.
To deploy your contract, you'll need to deploy it on the Polygon Mumbai testnet, where you'll have enough MATIC to pay for the Gas fees without spending a penny.
If this caught your attention, see: Ethereum Gas
Write a Deployment Script
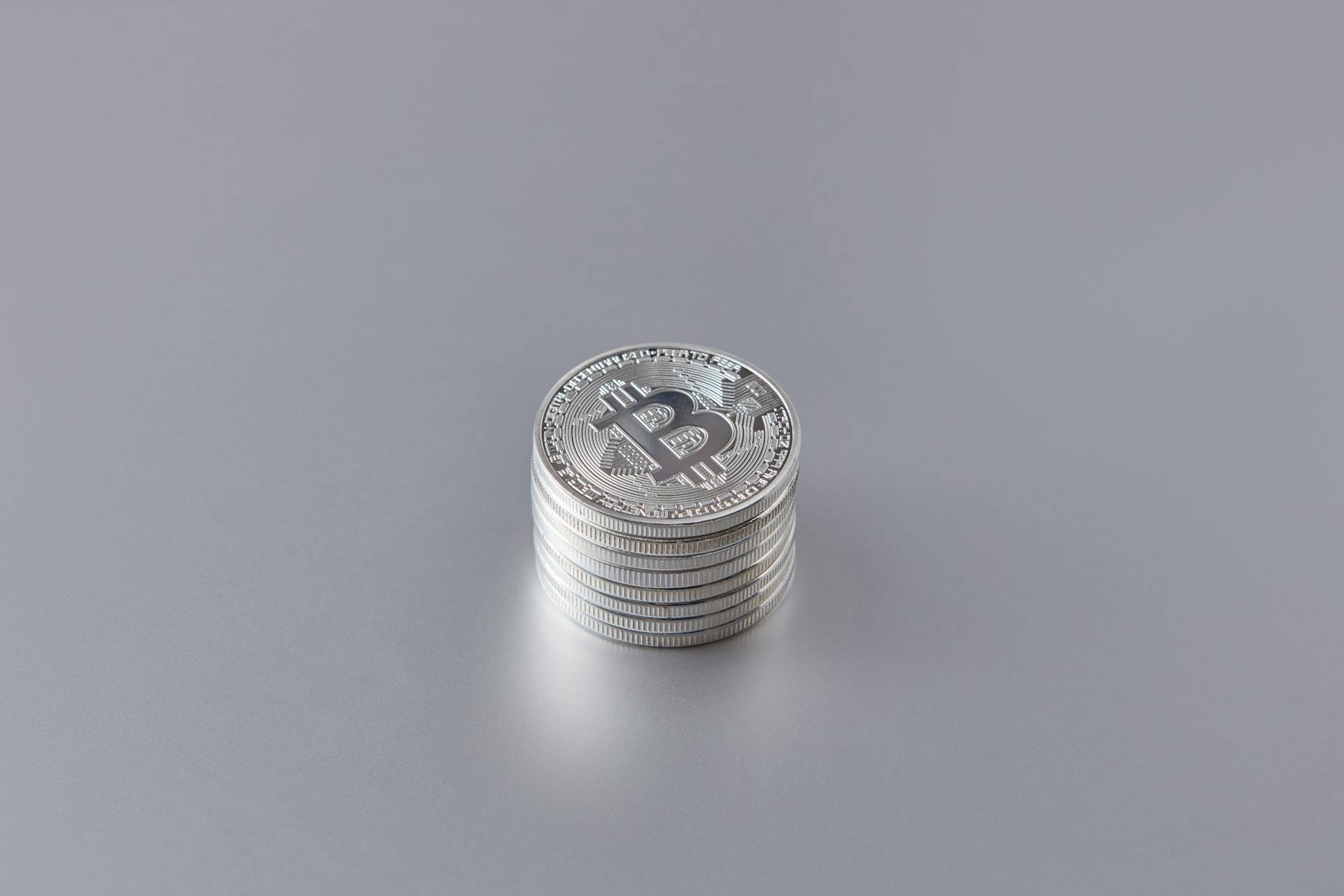
To write a deployment script for your ERC-20 token, navigate to the /scripts folder and create a new file called deploy.js. Then, open the deploy.js file and copy and paste the ERC-20 deployment code snippet into it.
You can use a library like Hardhat to give you access to utilities like getContractFactory(). To deploy your token using Hardhat, declare an async function and deploy the token passing as an argument the totalSupply value needed in the Token constructor.
Here's an example of what the deployment script might look like:
- Import the Hardhat library
- Declare an async function
- Deploy the token passing the totalSupply value as an argument
The totalSupply value should be passed as a string because it overflows the max int value allowed by JavaScript.
Here's an example of what the totalSupply value might look like:
* "1000000000000000000000000000"
You can read more about deploying with Hardhat in the official docs.
To deploy your contract, you'll need to run the following script in the terminal:
* npx hardhat run scripts/deploy.js --network polygon_mumbai
This will deploy your contract to the Polygon Mumbai testnet.
For your interest: What Happens When Bitcoins Run Out
Deploy to Goerli
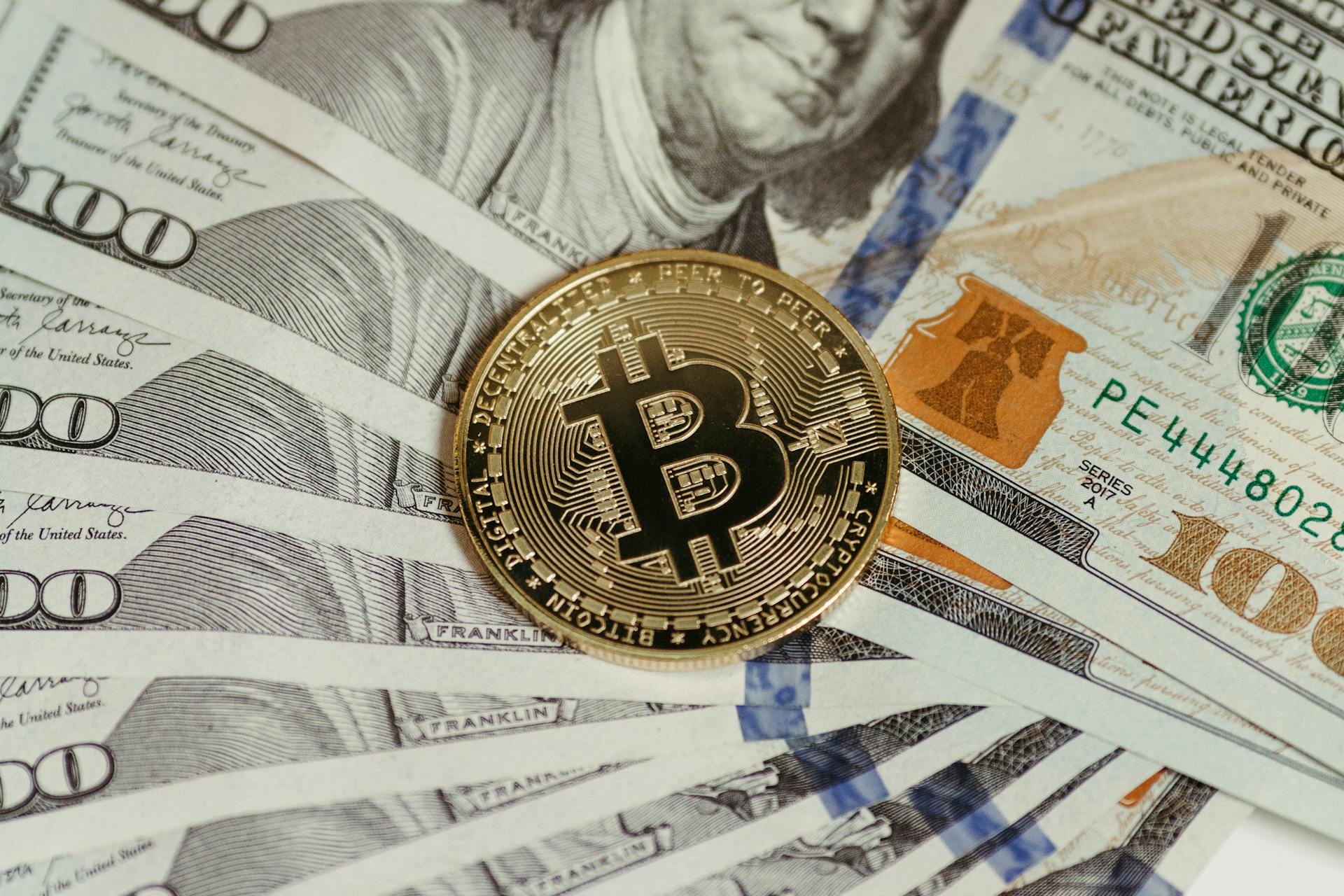
You should see a message appear with information about the smart contracts you are deploying, including your account address, account balance, and token address.
To verify your deployment, go to https://goerli.etherscan.io/ and input your outputted Token address to see your deployed ERC-20 contract on Goerli!
Here are the steps to deploy your ERC-20 token to Goerli:
- Navigate to your root directory
- Run the command to deploy your contract
- Verify your deployment on Goerli
You'll need to create a deployment script for your ERC-20 token before deployment. This script will be used to deploy your contract to the Goerli network.
Testing and Auditing
Testing and Auditing is a crucial step in the deployment process. It helps to rectify the malfunction of the ERC20 tokens and their functionalities.
You should thoroughly test your ERC20 token to ensure its hassle-free working. This includes identifying and fixing mistakes, errors, and bugs that may have been introduced during development.
Testing and auditing the ERC20 token is essential to prevent any issues that may arise after deployment.
Security and Access Control
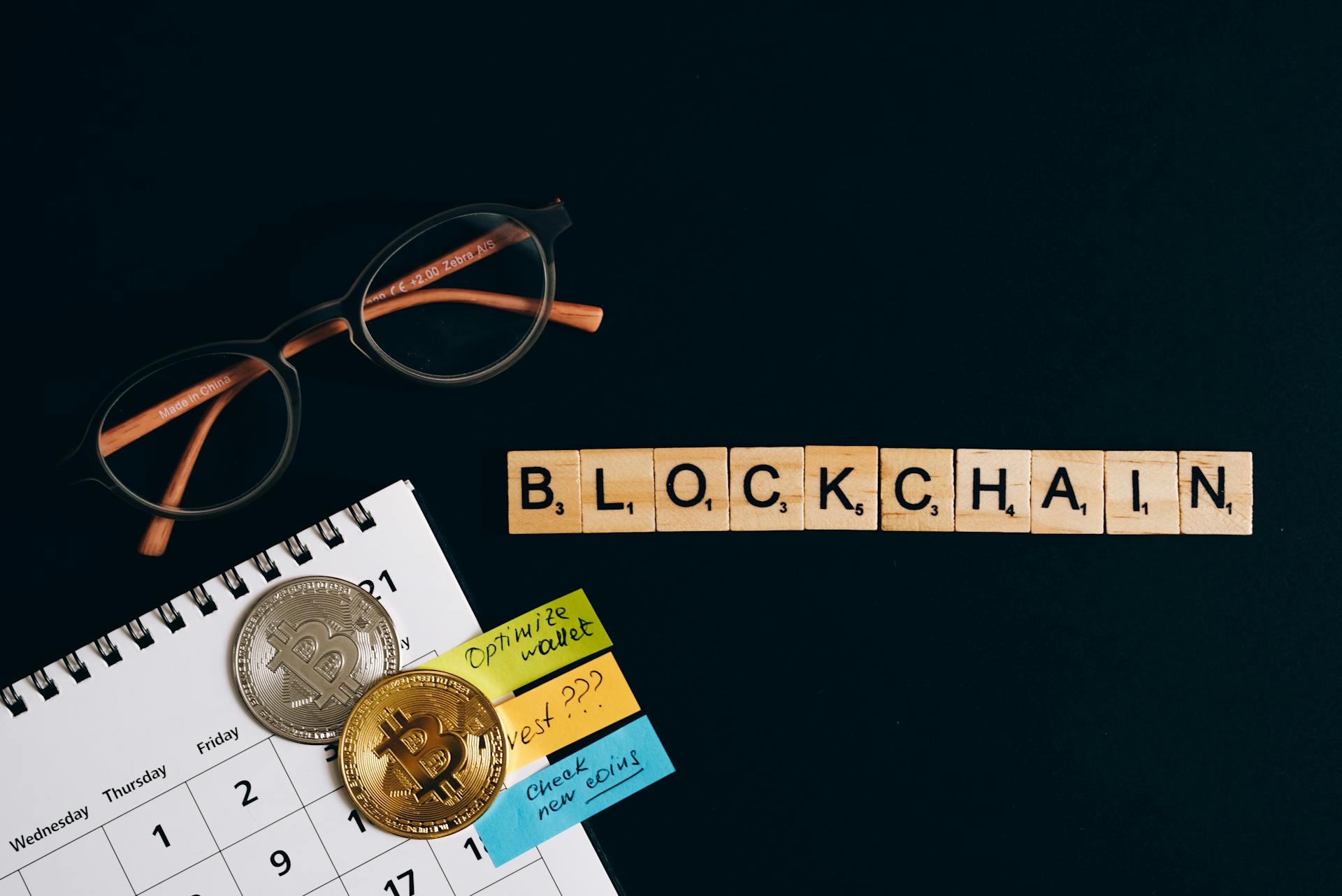
Access control is crucial when dealing with smart contracts, and it's used to determine who can perform sensitive tasks on a contract.
The most common form of access control is the concept of ownership, where only one account is allowed to perform sensitive tasks on a contract.
OpenZeppelin provides Ownable for implementing ownership in contracts, which makes it easy to add access control to your contract.
To use Ownable, you'll need to import the contract in your code and add it as a super-class of your Token contract.
The Token contract can now inherit from both ERC20 and Ownable, giving you access to the onlyOwner function modifier.
The onlyOwner function modifier will run every time issueToken() gets called, verifying if the caller is the owner of the contract.
By default, the owner of an Ownable contract is the account that deployed it, which is usually exactly what you want.
Understanding how to implement access control is critical to preventing someone else from stealing your system.
Token Details and Configuration
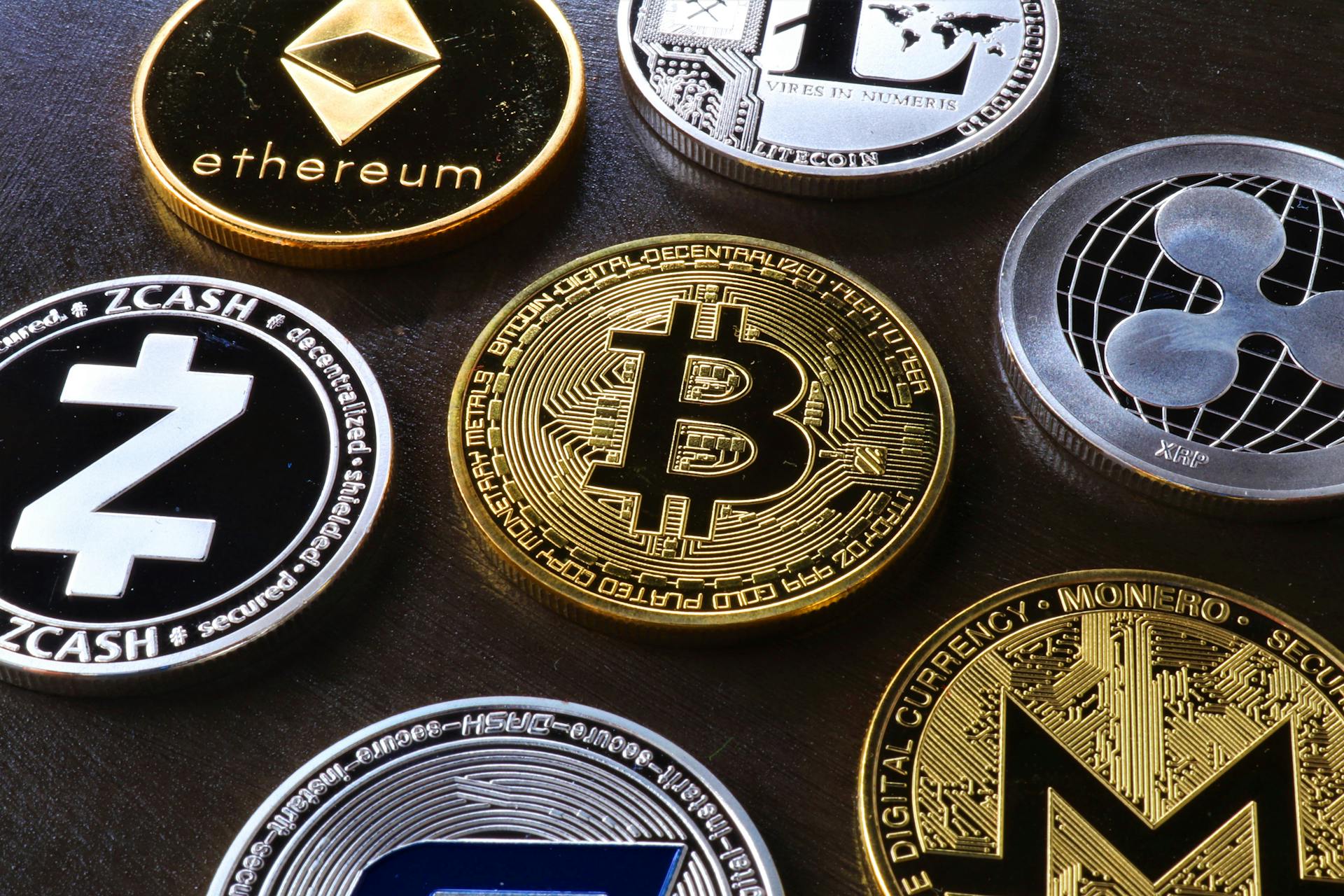
Defining your ERC20 token's details is a crucial step in its creation. This includes information such as the token name, symbol, total supply, and decimals.
You'll need to carefully consider these details as they will define the characteristics of your token. Take your time to fill out the form correctly, as changes become impossible once the contract is deployed on the blockchain.
The token details can be defined in the following fields: token name, symbol, total supply, and decimals. Here's a quick rundown of what each field means:
After filling out the form, click the "Create token" button, and you will see a confirmation form. Please double-check the data before confirming the transaction in your wallet.
Define Details
Defining the details of your ERC-20 token is a crucial step in the process.
Carefully consider the token name, symbol, total supply, and decimals, as they will define the characteristics of your token. Take your time to fill out the form, as changes become impossible once the contract is deployed on the blockchain.
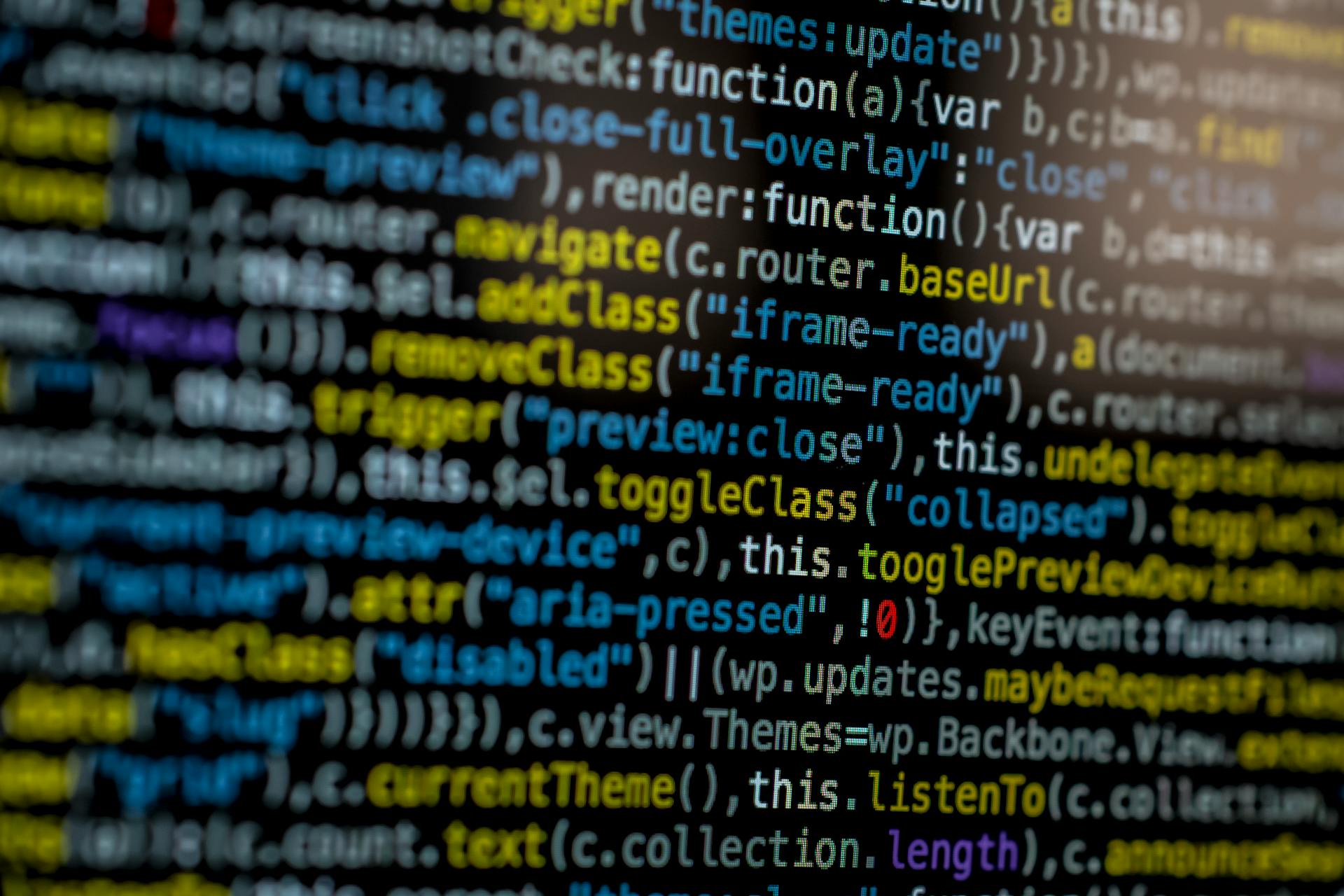
You'll want to double-check the data before confirming, as any mistakes will be irreversible. Click the "Create token" button and review the confirmation form before deploying the contract.
A token's decimals can be a bit tricky, but it's essential to understand the workaround. The Ethereum Virtual Machine supports 256-bit integers, so you can use larger integer values to represent decimal places.
For example, if you want a total max supply of 1.000.000.000 tokens with 18 decimal places, you'll need to pass 1000000000*10**18. This will ensure that your token can represent the desired amount of decimal places.
Be clear about the purpose of creating ERC20 tokens for your crypto business, and gather the necessary resources and tools to make the process easier. Finalizing the essential features needed for the token at the beginning will save you time and effort in the long run.
Capped Lazy
In the Capped Lazy Supply mechanism, tokens are issued in small quantities, with a max-supply decided beforehand and hardcoded in the smart contract. This approach allows for a more controlled release of tokens over time.
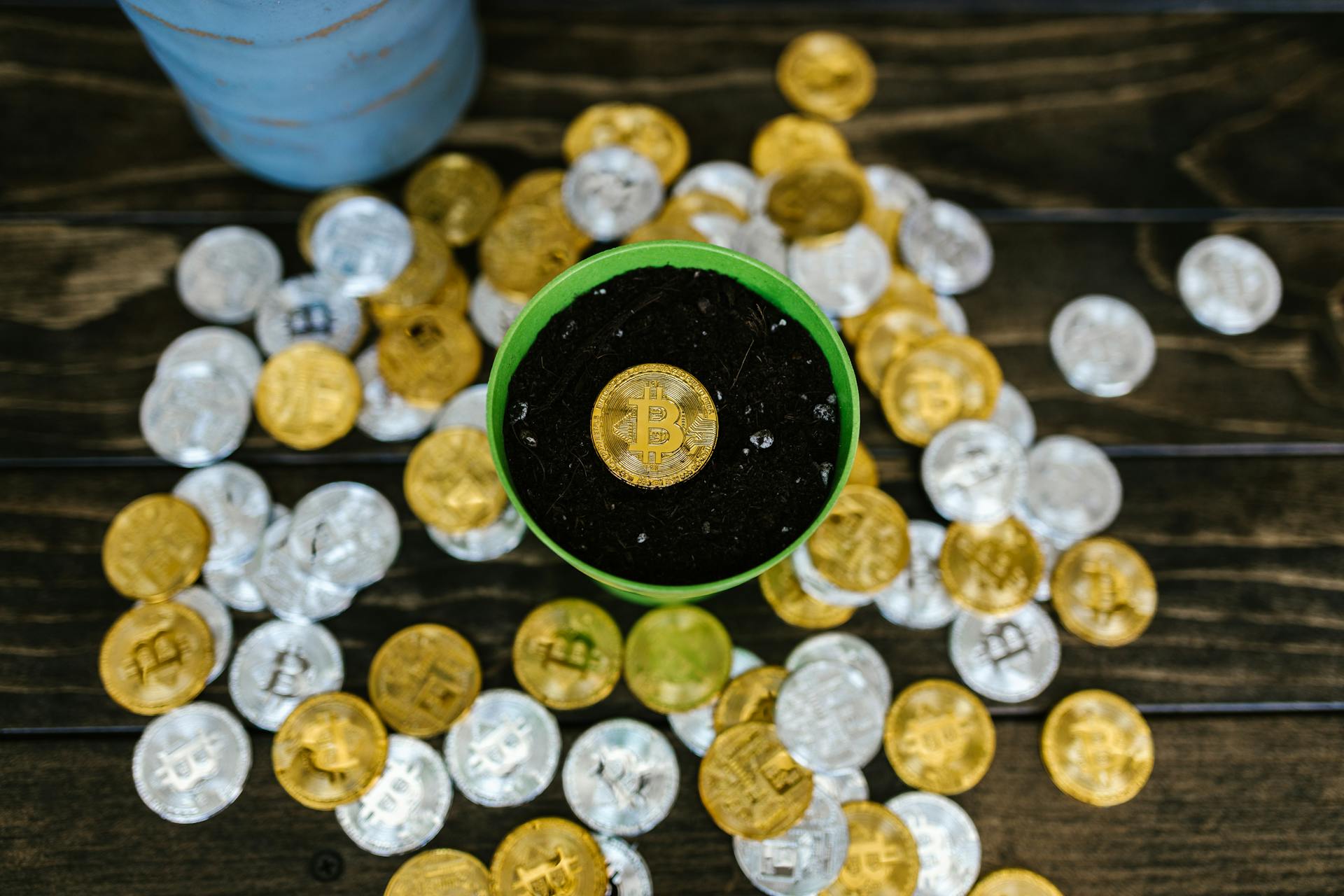
To create a token supply with Capped Lazy, you can check the token balance of the address that deployed the token by calling the balanceOf() method. This method is similar to the one used in the Uncapped Lazy Supply mechanism.
If you want to issue tokens over time and not in a unique batch, you can use the Capped Lazy Supply mechanism. It's a great way to manage your token supply and avoid flooding the market with tokens all at once.
Here are the key differences between Capped Lazy and Uncapped Lazy Supply:
In Capped Lazy Supply, the max-supply is decided beforehand and passed on deployment. This approach provides a more predictable and controlled release of tokens over time.
Add to Project
To add your ERC20 token to your project, you'll need to follow these steps. First, navigate to the /scripts folder in your project directory.
In this folder, you'll find a file called sample-script.js, which you'll need to rename to deploy.js. Then, open the deploy.js file and replace its content with the following code:
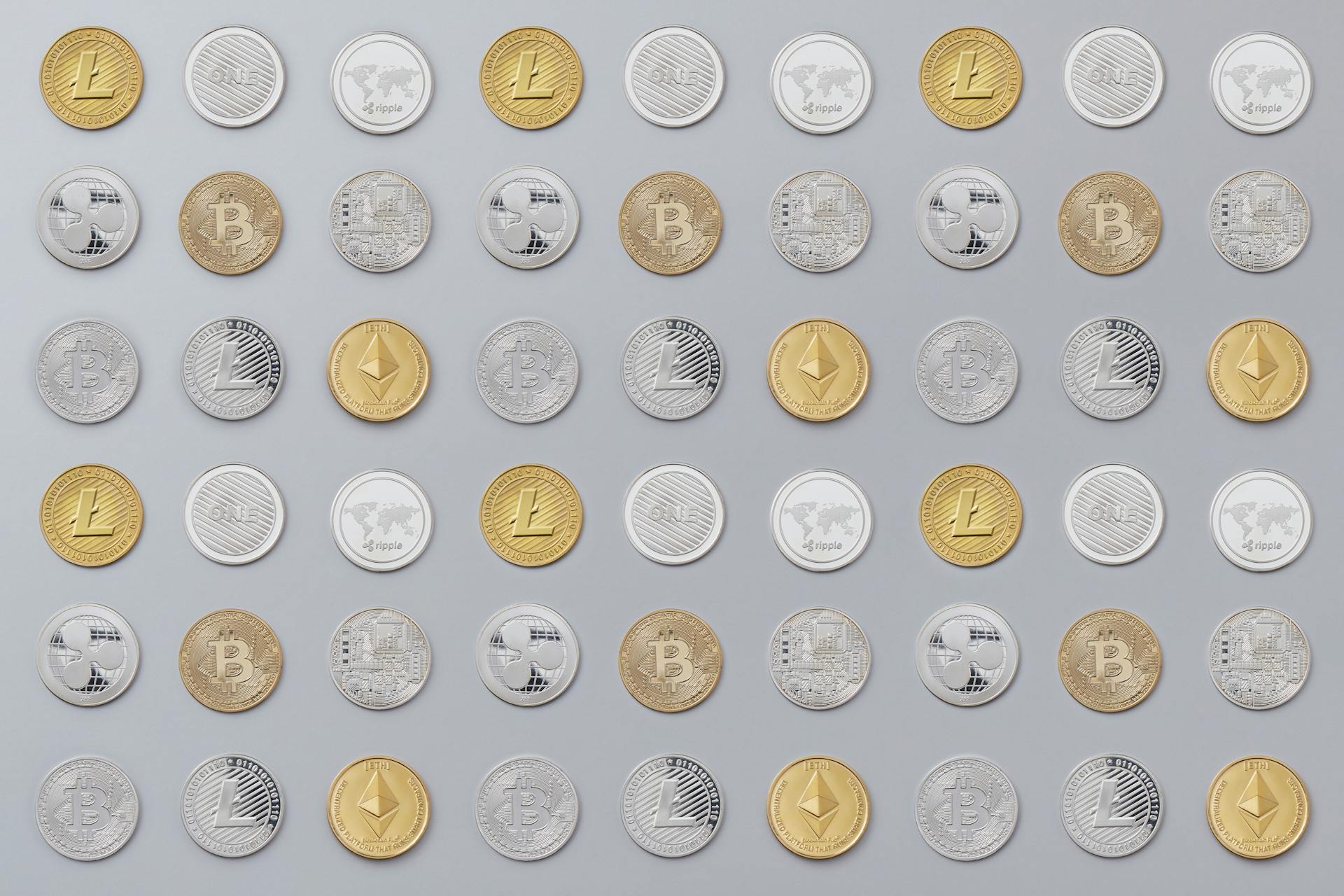
Here's a breakdown of what's happening in this code:
- We're importing the Hardhat library, which gives us access to utilities like getContractFactory().
- We declare an async function and deploy the token, passing as an argument the totalSupply value needed in the Token constructor.
- We await for the devToken to deploy and print its address.
To deploy your token, you'll need to run the following script in the terminal:
In the terminal, you can also run the command to compile your Token Contract and get the Artifacts. This is necessary to allow the getContractFactory() function in deploy.js to grab your contract.
Once you've deployed your token, you can view it on the Goerli network by inputting your token address on the Goerli Ethereum explorer.
Sources
- https://docs.alchemy.com/docs/how-to-create-an-erc-20-token-4-steps
- https://vitto.cc/how-to-create-and-deploy-an-erc20-token-in-20-minutes/
- https://medium.com/@coinfactory/how-to-create-an-erc-20-token-on-the-ethereum-3-steps-c077866d70cc
- https://www.coinsclone.com/create-erc20-token/
- https://www.quicknode.com/guides/ethereum-development/smart-contracts/how-to-create-and-deploy-an-erc20-token
Featured Images: pexels.com