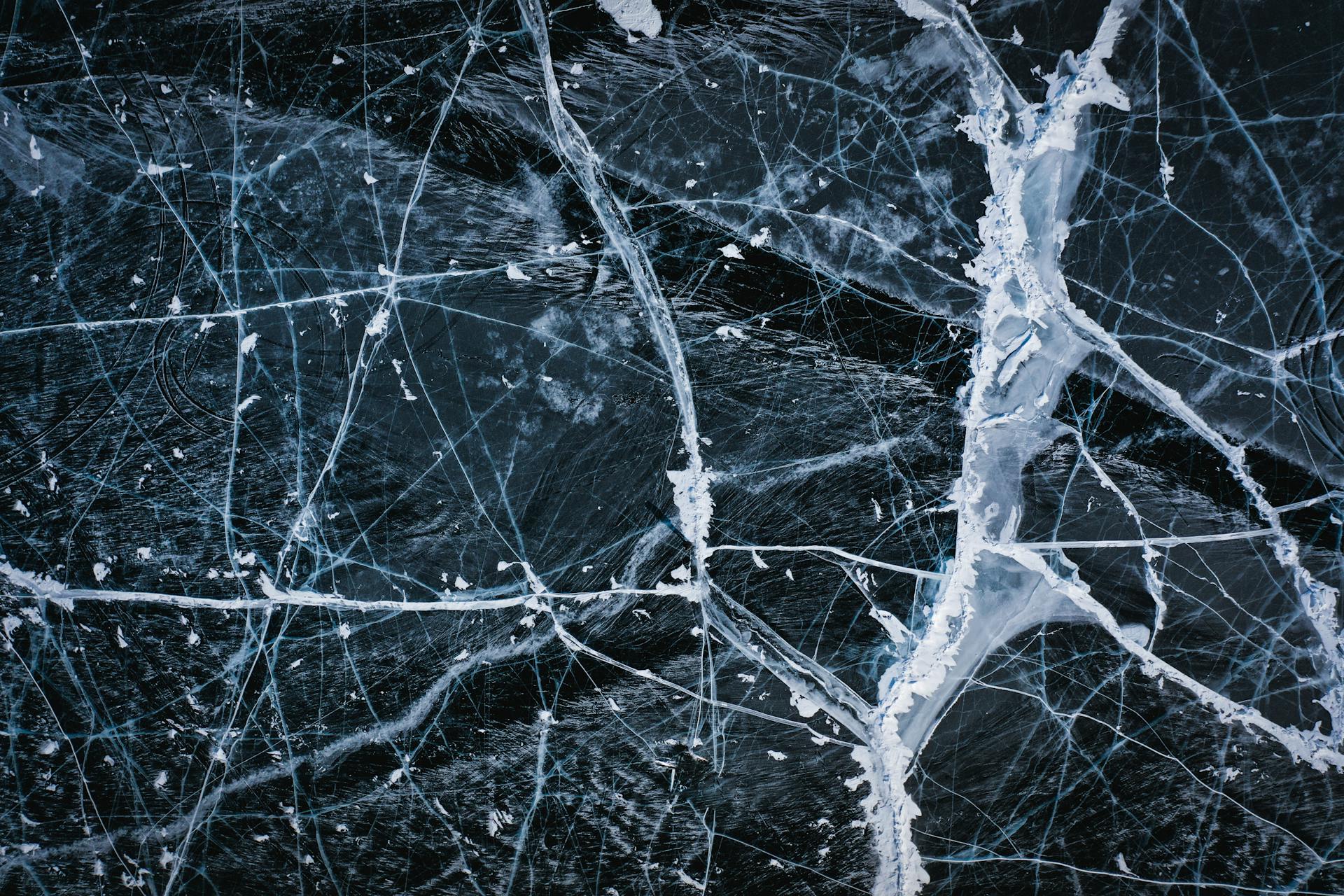
To create a smart contract with Ethereum and Solidity, you'll need to have a basic understanding of programming concepts and a text editor like Visual Studio Code.
Ethereum is an open-source blockchain platform that allows developers to build and deploy smart contracts.
Solidity is a contract-oriented programming language used for writing smart contracts on the Ethereum blockchain.
First, you'll need to install the Truffle Suite, a set of tools for building and deploying smart contracts on Ethereum.
Additional reading: Ethereum Smart Contracts
Solidity Language
The Solidity language is a high-level programming language designed specifically for smart contract development on the Ethereum platform. It's similar to JavaScript in terms of syntax, making it easy to learn for those with a background in programming.
Solidity is statically typed, meaning variables must be defined before they can be used. It also supports object-oriented programming concepts like inheritance and polymorphism. This makes it a powerful tool for building complex smart contracts.
Solidity includes features such as events, modifiers, and the ability to create custom data types. With these features, you can create smart contracts that are not only functional but also efficient and scalable.
Additional reading: Smart Contract Solidity
Supported Types
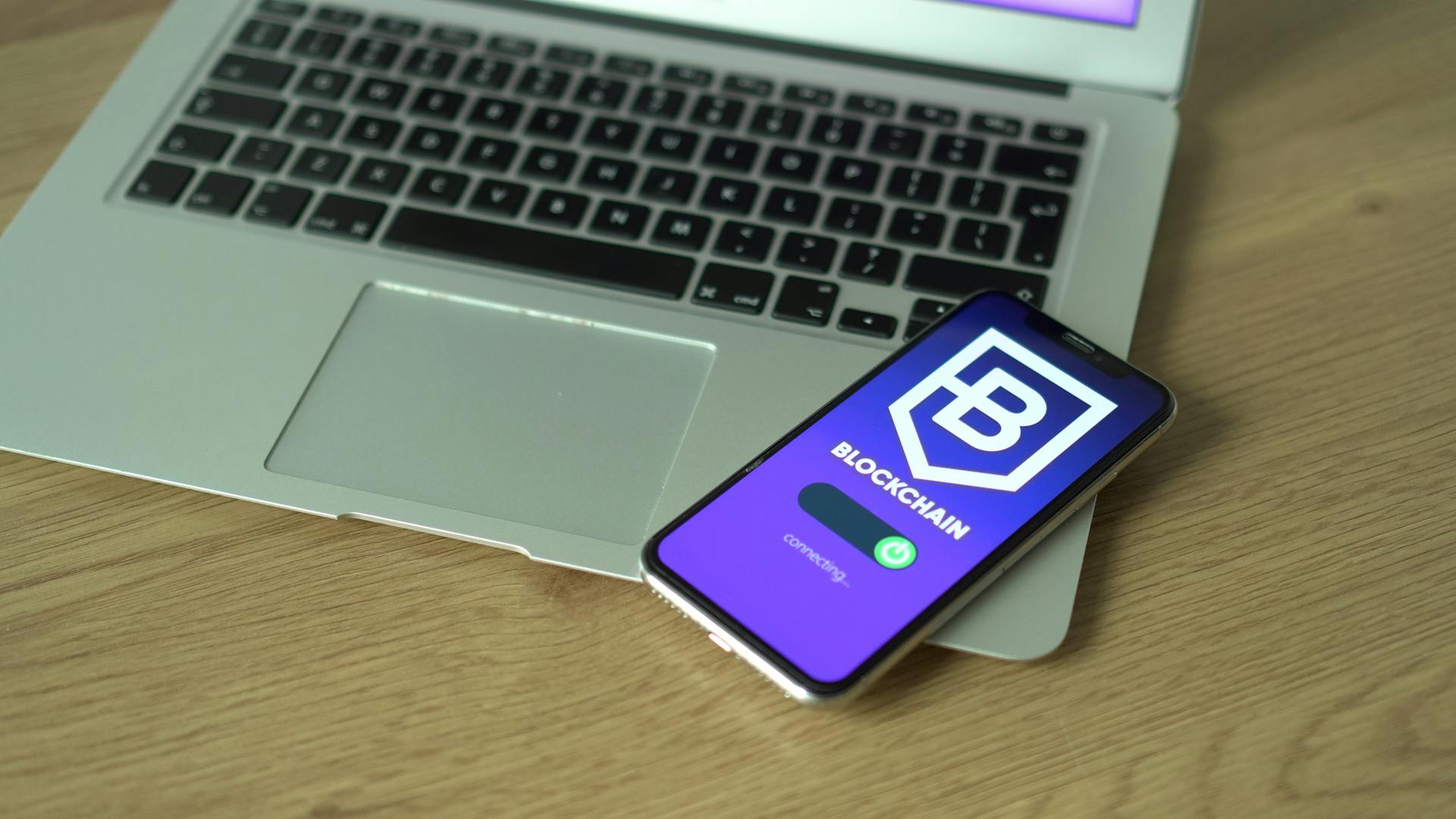
The Solidity language supports most Rust common data types, including booleans, unsigned and signed integers, strings, tuples, and arrays.
These data types are encoded and decoded using the Parity scale codec for efficient transmission over the network.
Substrate-specific types like AccountId, Balance, and Hash are also supported as if they were primitive types.
You can store an AccountId and Balance in your contract using the following code.
The scale codec allows for efficient transmission of data over the network, making it a key feature of the Solidity language.
Write Solidity Code
Writing Solidity Code is a crucial step in creating smart contracts on the Ethereum blockchain. You'll be utilizing the Solidity programming language, which is designed specifically for this purpose.
Solidity is statically typed, meaning variables must be defined before use, and it supports object-oriented programming concepts like inheritance and polymorphism. This makes it easier to write and maintain complex smart contracts.
To write Solidity code, you can start by customizing the basic contract example provided in Remix. This contract has a single public variable called "message" that is of type "string". You can also import libraries, other contract files, and call functions from other contracts.
For another approach, see: Justinians Code Created
The structure of a smart contract is similar to the one shown in the Remix example. It includes a constructor function that sets the initial value of the contract's variables and public functions that allow users to interact with the contract.
Here are the key elements to include in your Solidity code:
- License identifier: Specify the license under which the code is released (e.g., SPDX-License-Identifier: MIT)
- Compiler version: Specify the version of the Solidity compiler to use (e.g., pragma solidity 0.8.0;)
- Contract definition: Start the contract definition with the contract keyword (e.g., contract HelloWorld {)
- Variable declarations: Declare variables with their data types (e.g., string message;)
- Constructor function: Define a constructor function that initializes the contract's variables
- Public functions: Define public functions that allow users to interact with the contract
Remember to thoroughly test your code before deploying it to the mainnet. This will help you catch any errors or bugs that may arise during execution.
Integrated Development Environment (IDE)
Remix IDE is a web development environment created by the Ethereum Foundation, which offers features such as syntax highlighting, code autocompletion, and a built-in Solidity compiler.
For beginners, Remix IDE is the most appropriate and user-friendly tool for creating, testing, and deploying smart contracts. It's a great choice for those new to smart contract development.
To deploy a smart contract to the Ethereum test network, you'll need to have your own address on the Ethereum network, which can be obtained through the use of the Metamask browser extension.
You might enjoy: Ethereum App Development Company
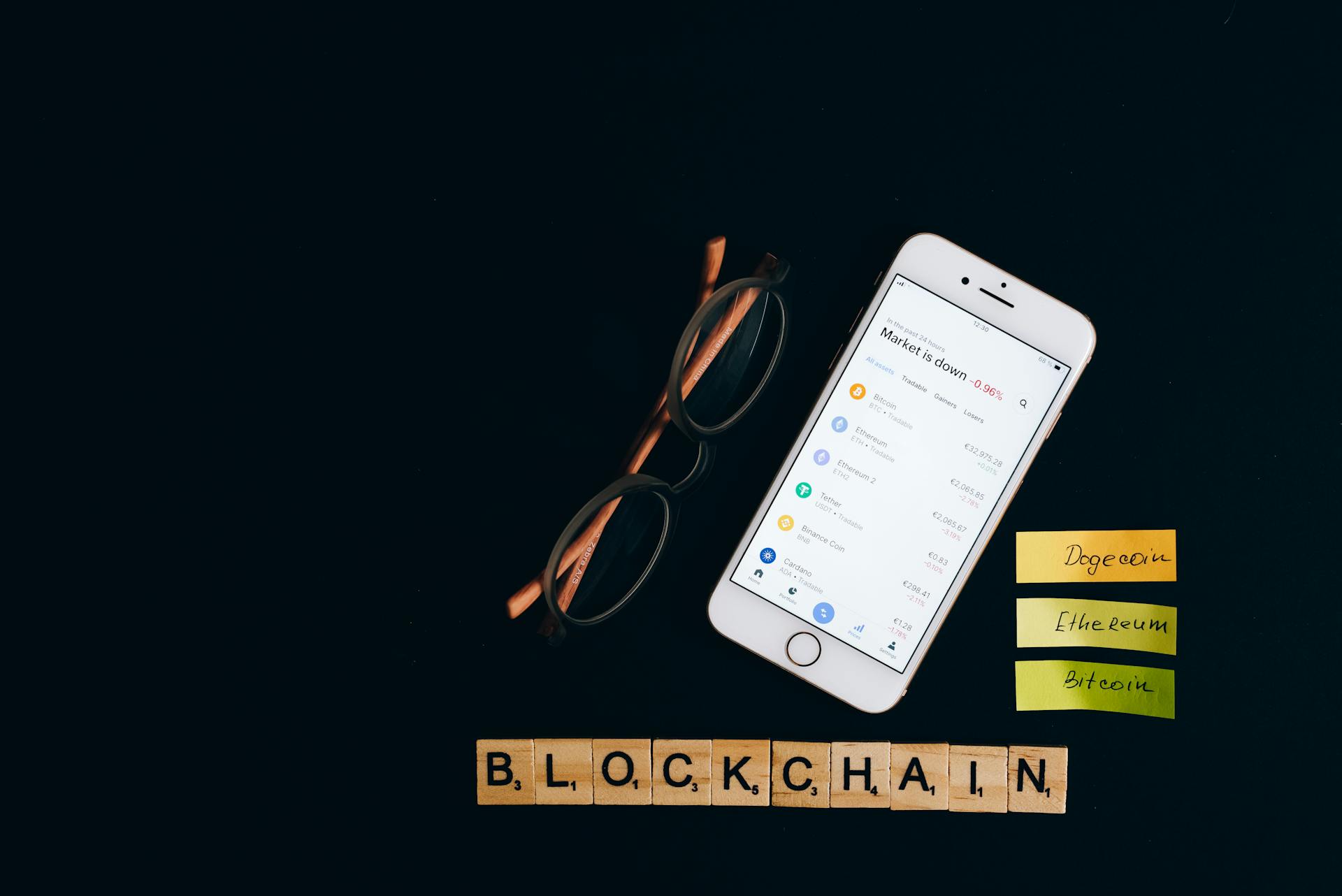
The Remix IDE has four main tools located at the top left of the screen for navigating and working within the environment: File Explorer, File explorer and search bar, Solidity Compiler, and Deploy & Run Transactions.
Remix IDE is a browser-based IDE for developing Ethereum smart contracts, and it doesn't require the installation of tools like frameworks, package managers, and libraries. All you may need is a crypto wallet and one of the blockchain simulators mentioned in the blockchain simulator section.
The Remix IDE interface is similar to other IDEs' interfaces, such as Visual Studio, and it auto-generates buttons for each function in your code. It features four sections: Main Panel, Terminal, Side Panel, and Icons Bar.
To get started with Remix, simply go to the website and open the IDE, then you can begin to set up your development environment, including selecting the compiler version and environment, as well as setting up any necessary plugins or libraries.
Here are the main features of the Remix IDE:
- Main Panel: Lists some useful shortcuts and opens up an editor whenever a file is selected or created in the File Explorer.
- Terminal: Allows you to run scripts and provides you with details about transactions.
- Side Panel: Acts as a graphical placeholder, showing text fields and dropdown lists from the highlighted plugin in the Icon Bar.
- Icons Bar: Located on the far left-hand side, it lists all the icons of the plugins you activated, the search icon, the Plugins Manager, and the Settings.
Development Environment
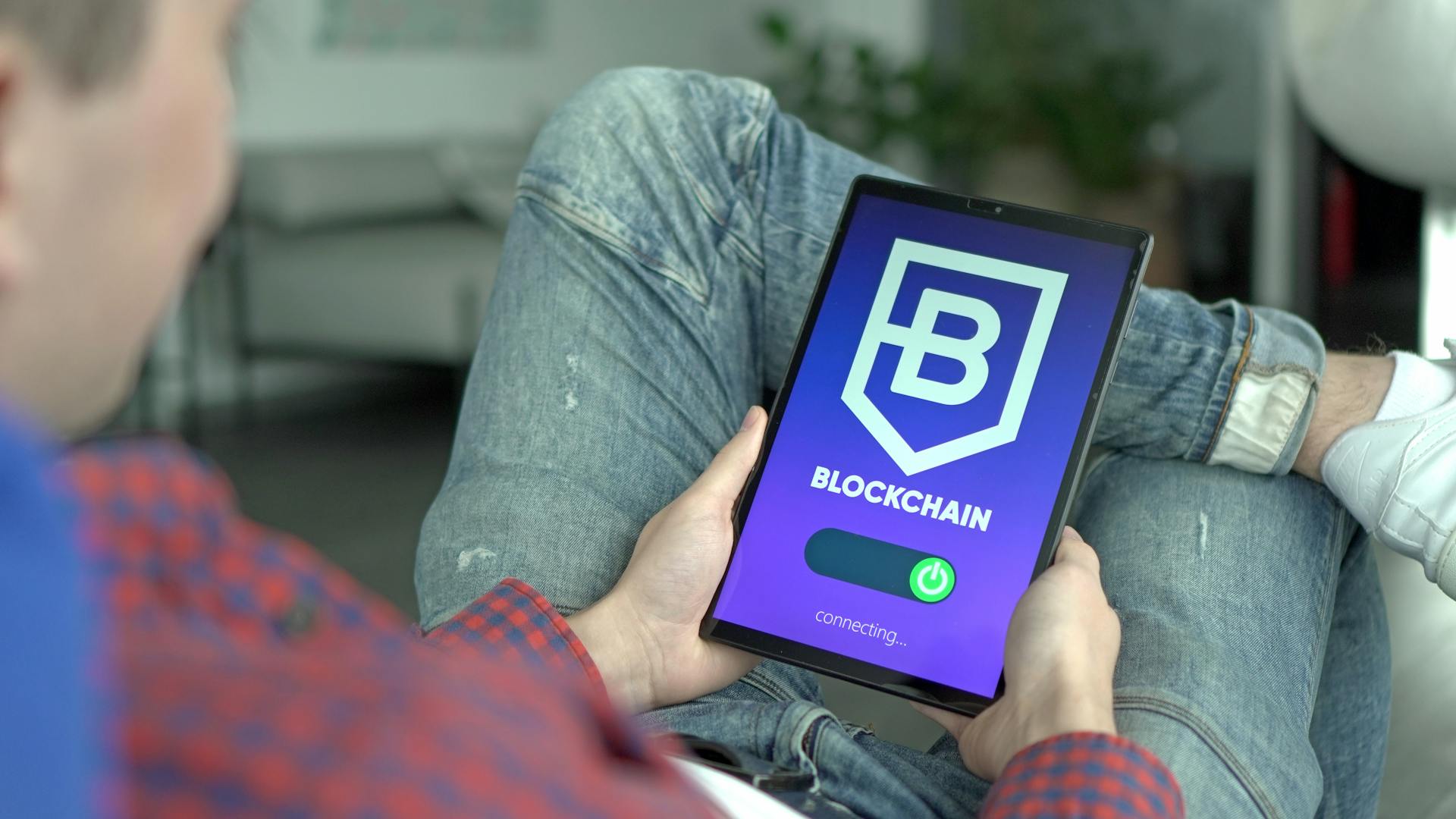
To create a smart contract, you'll need to set up a development environment. Remix is a web-based IDE that allows you to write, test, and deploy smart contracts on the Ethereum blockchain.
Remix has a user-friendly interface, making it a great choice for beginners. It's also beginner-friendly, so you don't need to install separate tools like frameworks, package managers, and libraries.
A coding setup for developing smart contracts includes a crypto wallet, an IDE, a package manager, a library, a framework, and a blockchain simulator. However, with Remix, you might only need an optional crypto wallet and a blockchain simulator.
To get started with Remix, simply go to the website and open the IDE. Once you're in the IDE, you can begin to set up your development environment.
The Remix interface features four main sections: the Main Panel, Terminal, Side Panel, and Icons Bar. The Main Panel lists useful shortcuts and opens up an editor when a file is selected or created in the File Explorer.
For your interest: How to Develop Blockchain Applications
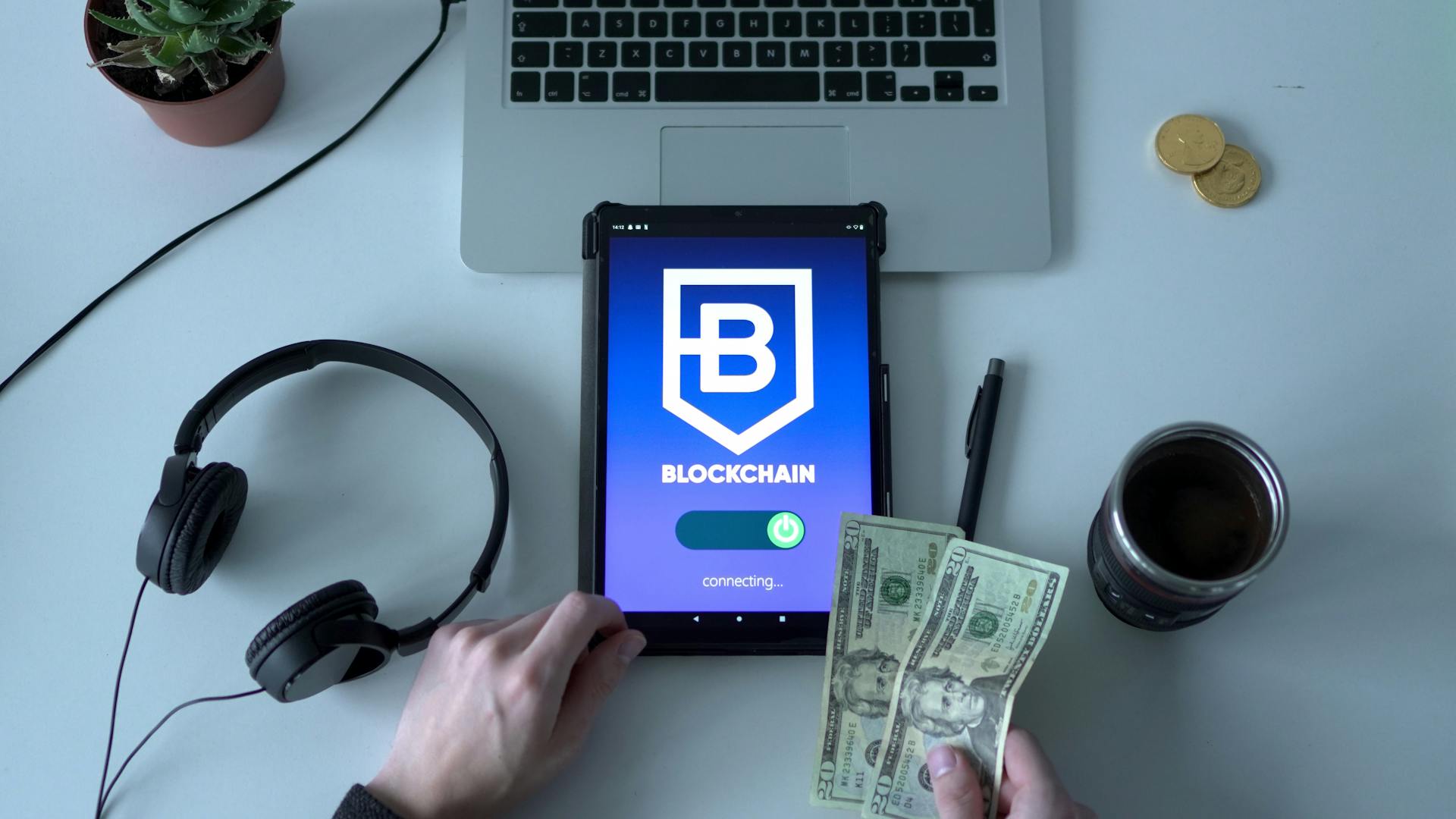
Here's a breakdown of the main sections of the Remix interface:
- Main Panel: Lists useful shortcuts and opens up an editor when a file is selected or created in the File Explorer.
- Terminal: Allows you to run scripts and provides details about transactions.
- Side Panel: Acts as a graphical placeholder, showing text fields and dropdown lists from the highlighted plugin.
- Icons Bar: Lists all the icons of the plugins you activated, the search icon, the Plugins Manager, and the Settings.
You can select the compiler version and environment, as well as set up any necessary plugins or libraries, to complete your development environment setup.
Ethereum Blockchain
Ethereum smart contracts are developed using Solidity, an object-oriented programming language that shares elements with other familiar languages.
The main programming language used for developing Ethereum smart contracts is Solidity, which uses Events to notify apps' front-ends about state changes on the blockchain.
Smart contracts' source code starts with a declaration of the version of the Solidity compiler to be used, which helps prevent issues with future compiler versions introducing changes that may hinder your code.
You'll often see License Identifiers added to smart Ethereum open-source projects, like in the sample smart contract code.
A simple Solidity contract, named WelcomeToWeb3, can be created to greet callers.
To develop a smart contract, you'll need a combination of software development tools.
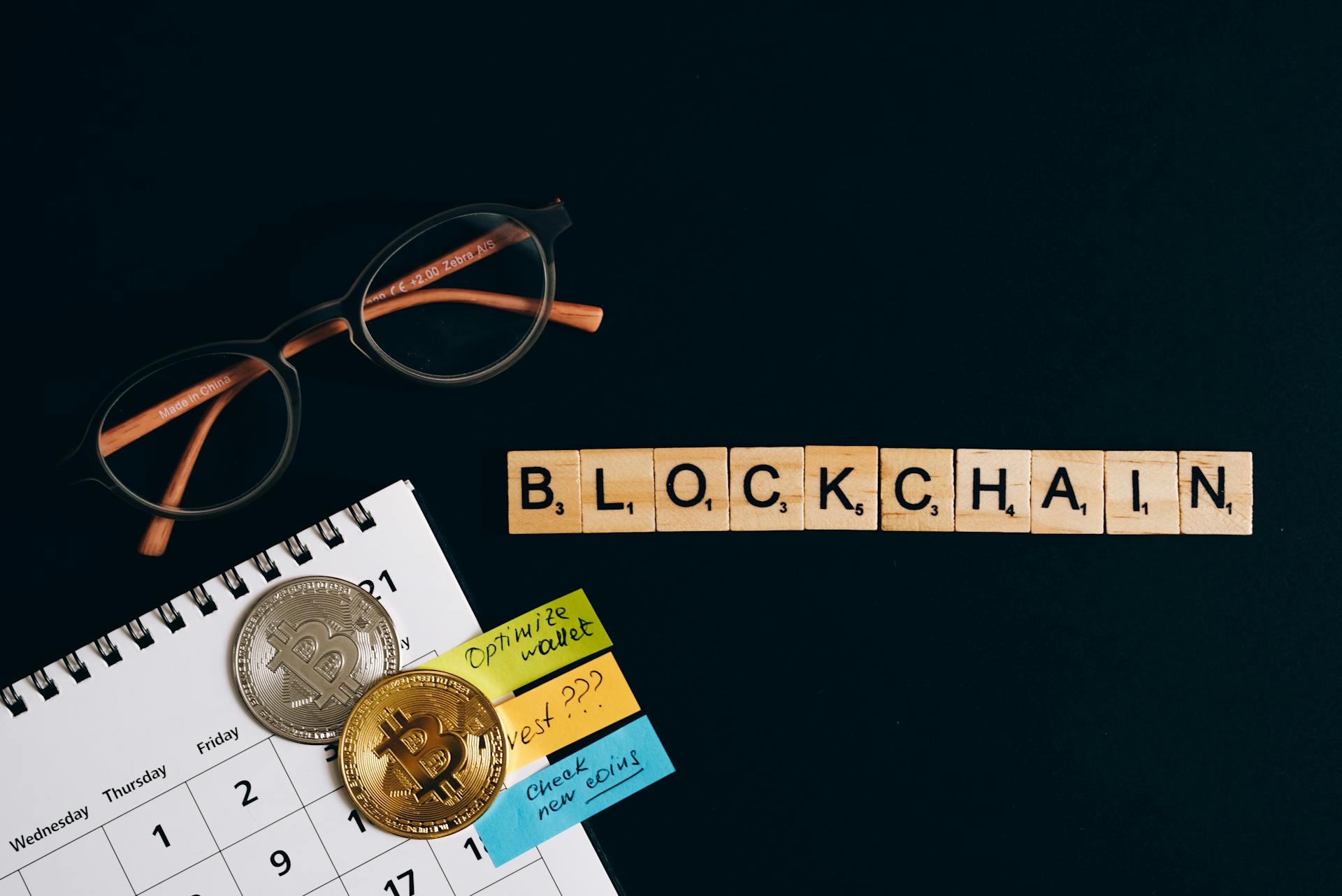
You can use Remix IDE, which doesn't require adding libraries and frameworks, but you'll need a blockchain simulator to produce realistic tests of your smart contract.
Here's a list of Ethereum blockchain simulators:
- Testnets: blockchain live test networks for testing smart contracts.
- Ganache: a local blockchain simulator installed on your computer.
- JVM: Remix's browser-based blockchain, which stands for JavaScript Virtual Machine.
Ethereum replaced its Proof-Of-Work (POW) consensus mechanism with Proof-Of-Stake (POS), which is used by blockchain systems to achieve agreement on data.
We'll use Goerli testnet because it upgraded to Proof-Of-Stake.
Deploy and Test
Deploying and testing a smart contract is a crucial step in the development process. You can deploy to a testnet using MetaMask and Remix, or deploy and test on a local blockchain node.
To deploy to a testnet, click on the "Deploy & Run Transactions" icon in Remix, then select the "Injected" option from the Environment dropdown menu. Next, click on the "Deploy" button to create a popup with deployment transaction details. Confirm the transaction to automatically execute the deployment.
You can also deploy and test on a local blockchain node using substrate-contracts-node and cargo-contract. Start the contracts node by running the command `substrate-contracts-node --log info,runtime::contracts=debug 2>&1`. Then, upload and instantiate the contract using the `cargo contract instantiate` command.
Intriguing read: What Are Smart Contracts Blockchain
Once your contract is deployed, you can interact with it by calling its functions and sending transactions. For example, to increment the value of a contract, use the `cargo contract call` command with the `inc` message and the `--args 42` option.
Here's a summary of the steps to deploy and test a smart contract:
Smart Contract Creation
To create a smart contract, you need to understand the basics of smart contract development, which includes knowing what a smart contract is and how it works on the Ethereum Virtual Machine (EVM). A smart contract is a self-executing program stored on a blockchain, designed to automate specific tasks without intermediaries.
For beginners, it's essential to have a solid foundation in programming before diving into Solidity and blockchain technology. Specifically, having a good understanding of object-oriented programming concepts in statically typed languages like C++, C#, Java, and Go can be extremely beneficial. Additionally, knowledge of JavaScript can also be helpful when working with Solidity.
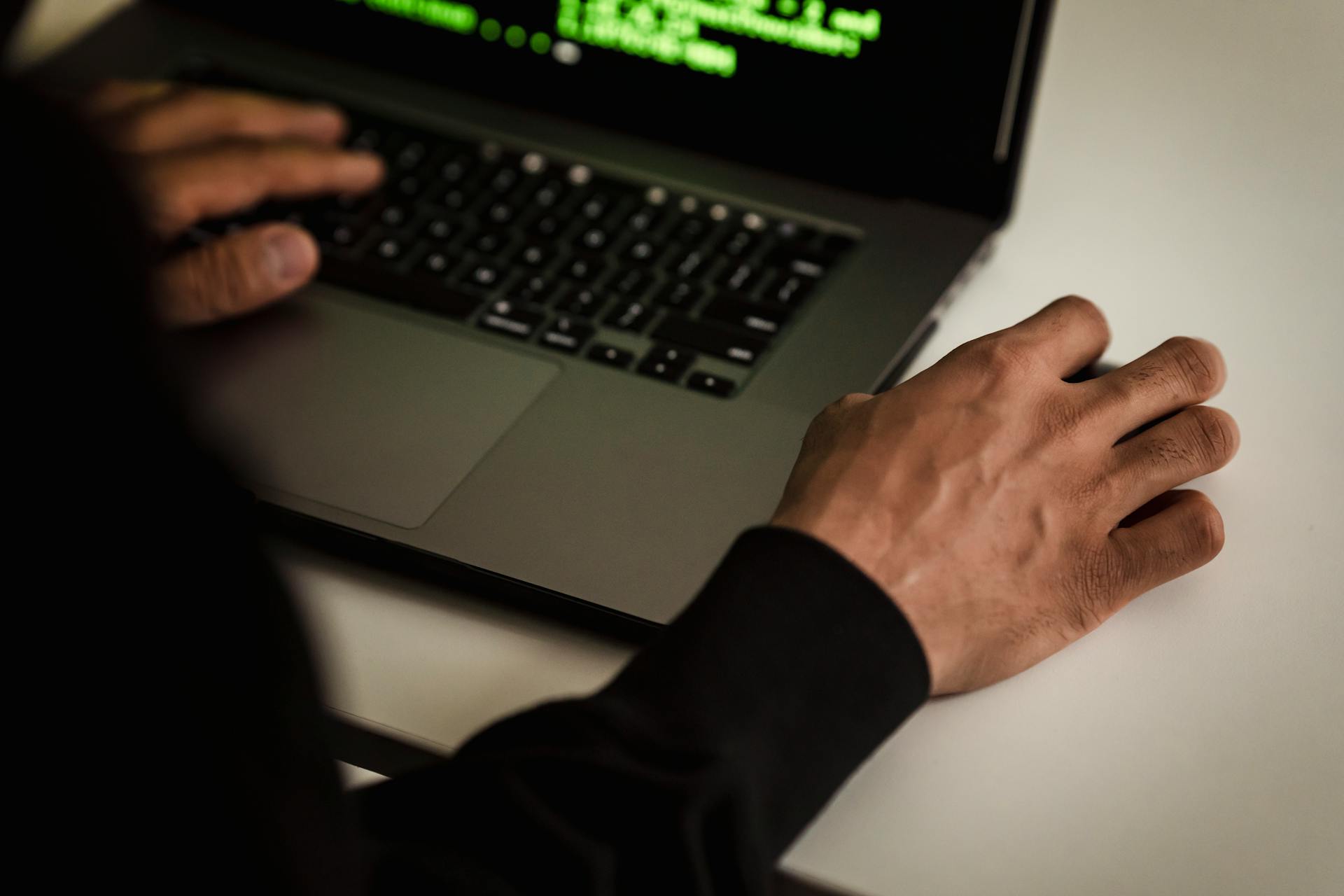
Here are some key concepts to keep in mind when creating a smart contract:
- The SPDX-License-Identifier comment specifies the license under which the code is released.
- The Solidity compiler version is specified using the pragma solidity statement.
- The contract definition starts with the contract keyword, followed by the contract name.
- Variables and functions are declared using the correct syntax, such as string message; and function getMessage() public view returns (string memory) {.
Having a good grasp of these concepts will help you create more secure and reliable smart contracts.
Intro
A smart contract is a self-executing program stored on a blockchain, designed to automate tasks without intermediaries. It's like a digital version of a traditional contract, but with added benefits of being secure, transparent, and decentralized.
Ethereum is a blockchain platform created to enable the development of smart contracts, using the Ethereum Virtual Machine (EVM) to execute code on the network. The EVM is a virtual machine that allows developers to create and test smart contracts before deploying them on the live network.
To become a smart contract developer, it's essential to have a solid foundation in programming, specifically object-oriented programming concepts in statically typed languages like C++, C#, Java, and Go. Having knowledge of JavaScript can also be helpful when working with Solidity.
If you're new to programming, start with the basics of computer programming first, and once you have a good grasp of the fundamentals, you can explore more complex concepts like smart contract development.
Constructors
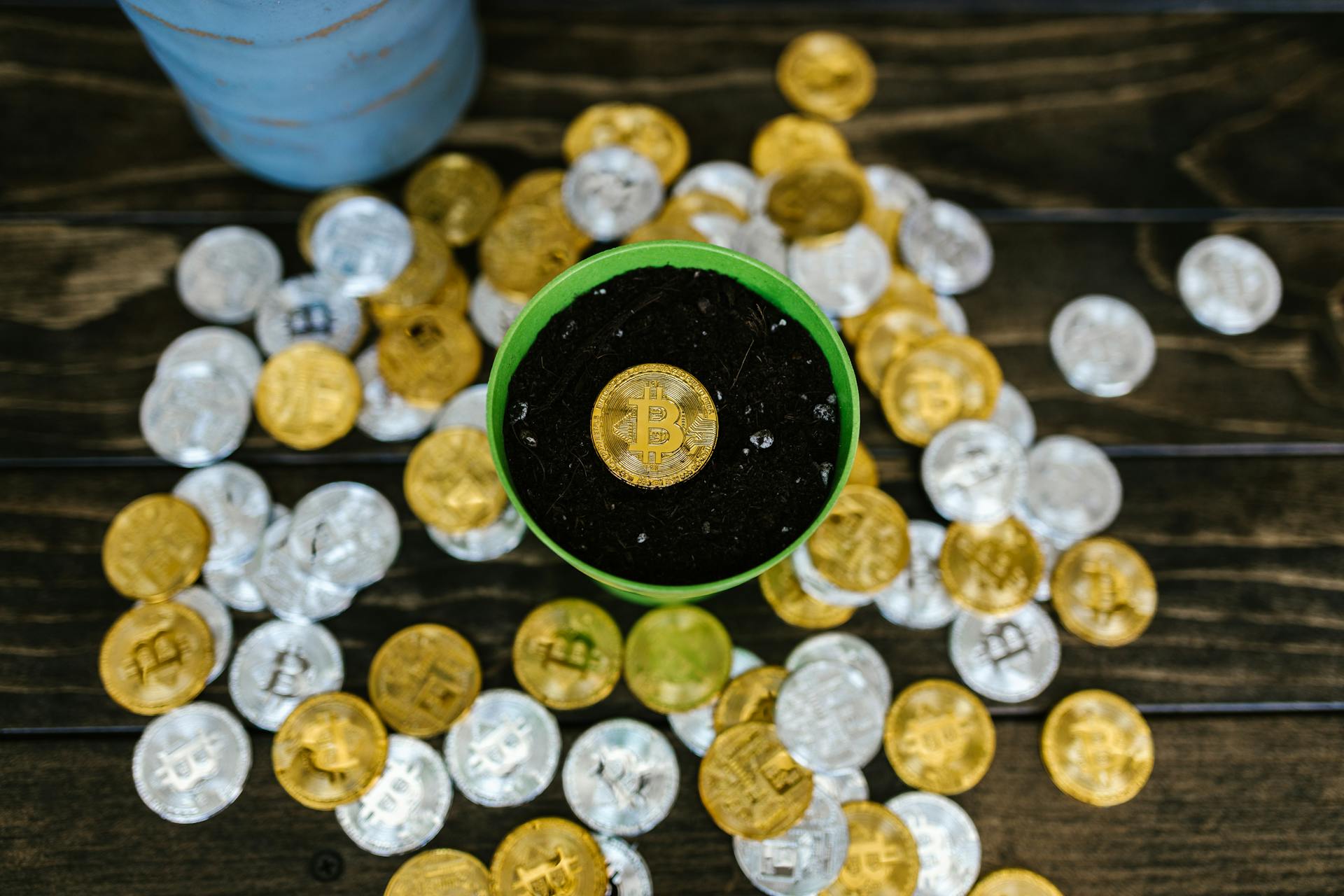
Constructors are a crucial part of smart contract creation, and every ink! smart contract must have at least one constructor that runs when the contract is created.
A constructor can be used to initialize variables and set up the contract's state, and it can also be used to validate inputs and ensure the contract is deployed correctly.
In some cases, a smart contract can have multiple constructors, if needed, as illustrated in the code example that shows using multiple constructors.
This flexibility allows developers to create contracts that are tailored to specific use cases and requirements.
Pre-Creation Checklist
To create a smart contract, you need to set up your coding environment with Remix IDE, MetaMask, and a testnet like Goerli. This setup allows you to test your contract without using real Ethereum.
Testnets are networks that mimic the main Ethereum network, so you can interact with them using test ETH. To do this, you'll need to provide the address of the smart contract, the function you want to call, and sometimes variables to pass to the function.
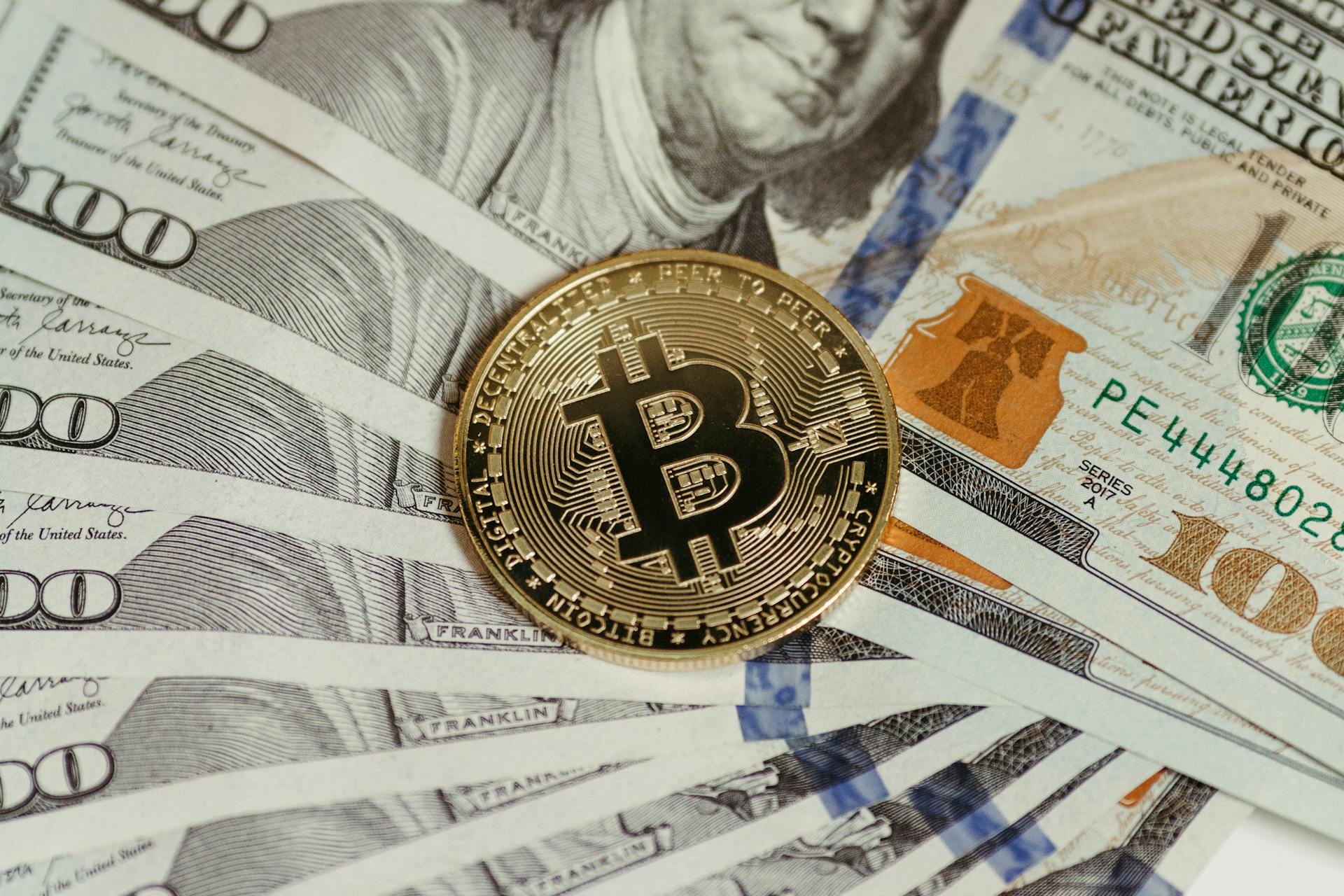
You can set up your own node to interact with the Ethereum blockchain, but it's more convenient to use a third-party service like Infura. Infura offers a node-ready infrastructure that you can use without setting up your own node.
MetaMask uses Infura's infrastructure as a Web3 provider, so you don't need to sign up for an Infura API key. This makes it easy to get started with smart contract creation.
To make it easy to access MetaMask, pin it to your browser toolbar. This way, you can quickly access it whenever you need to interact with your smart contract.
Here's a quick rundown of the tools you'll need to get started:
- Remix IDE
- MetaMask
- Goerli testnet
- Infura (or another third-party node provider)
How to
To create a smart contract, you need to understand the basics of programming. A smart contract is a self-executing program that automates the execution of specific tasks on a blockchain.
You can start by installing the Metamask extension for your browser, which will help you create a new wallet. This is a straightforward process, but if you encounter any difficulties, you can refer to the official guide for assistance.
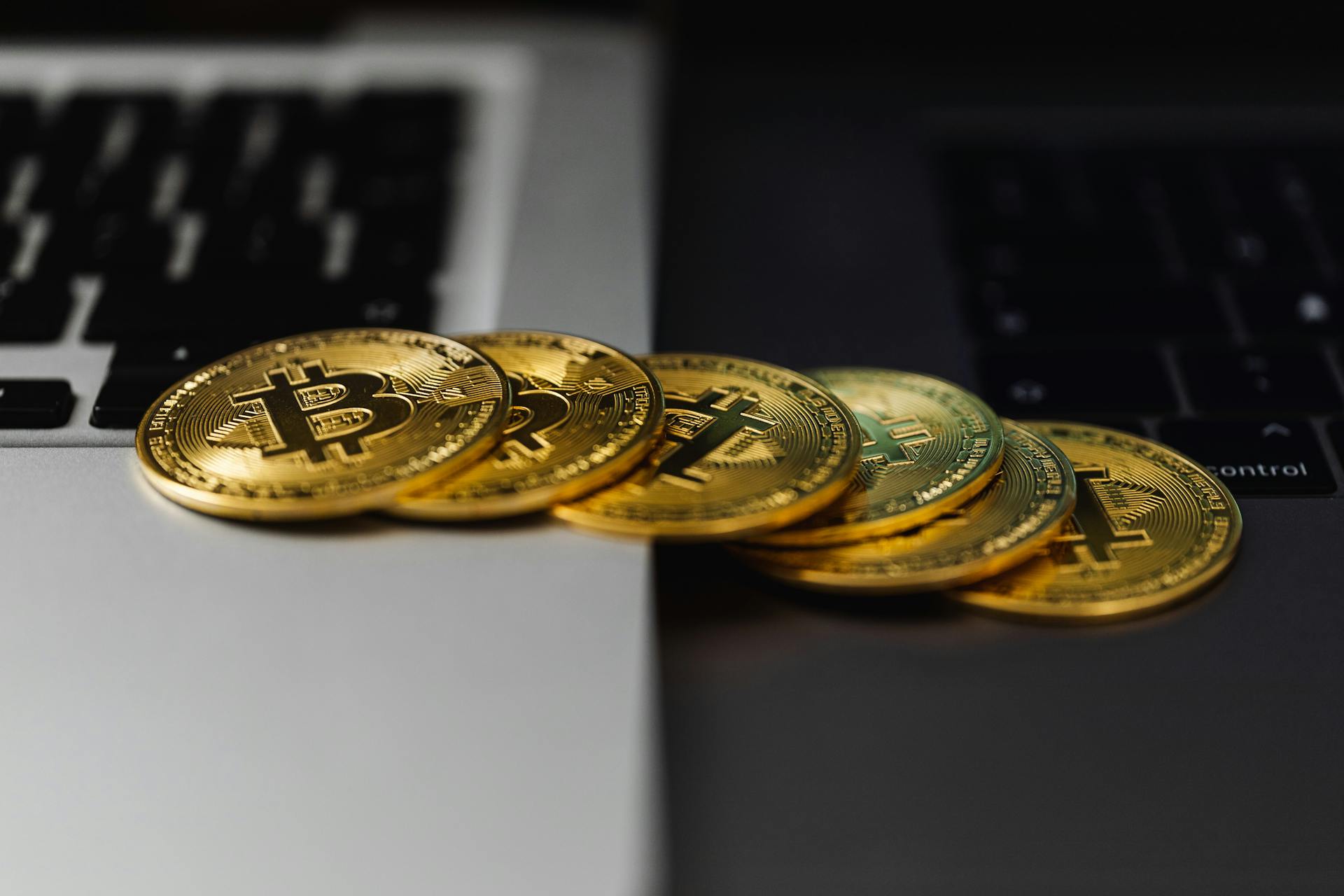
To write the smart contract code in Solidity, you can use a tool like Remix, which provides an editor where you can start writing your contract code. You can also import libraries and other contract files.
A smart contract typically has a constructor function that is executed when the contract is deployed, which sets the initial value of the contract's variables. For example, in the "MyVeryFirstContract" contract, the constructor function sets the initial value of the "message" variable to "MyVeryFirstContract".
To add a function to get a storage value, you need to update the contract code to include a public function that returns the data for the value storage item. This function should use the #[ink(message)] attribute macro.
Here's a step-by-step guide to adding a public function to get a storage value:
- Open the contract file in a text editor.
- Update the public function to return the data for the value storage item.
- Replace the test comment in the private default_works function with code to test the new public function.
- Save your changes and close the file.
Remember to thoroughly test your code before deploying it to the mainnet.
The 12 Benefits
Smart contracts offer numerous benefits to developers, artists, and Web3 enthusiasts. One major advantage is that they're not vulnerable to a single point of failure like centralized server apps, ensuring no restrictions or downtime.
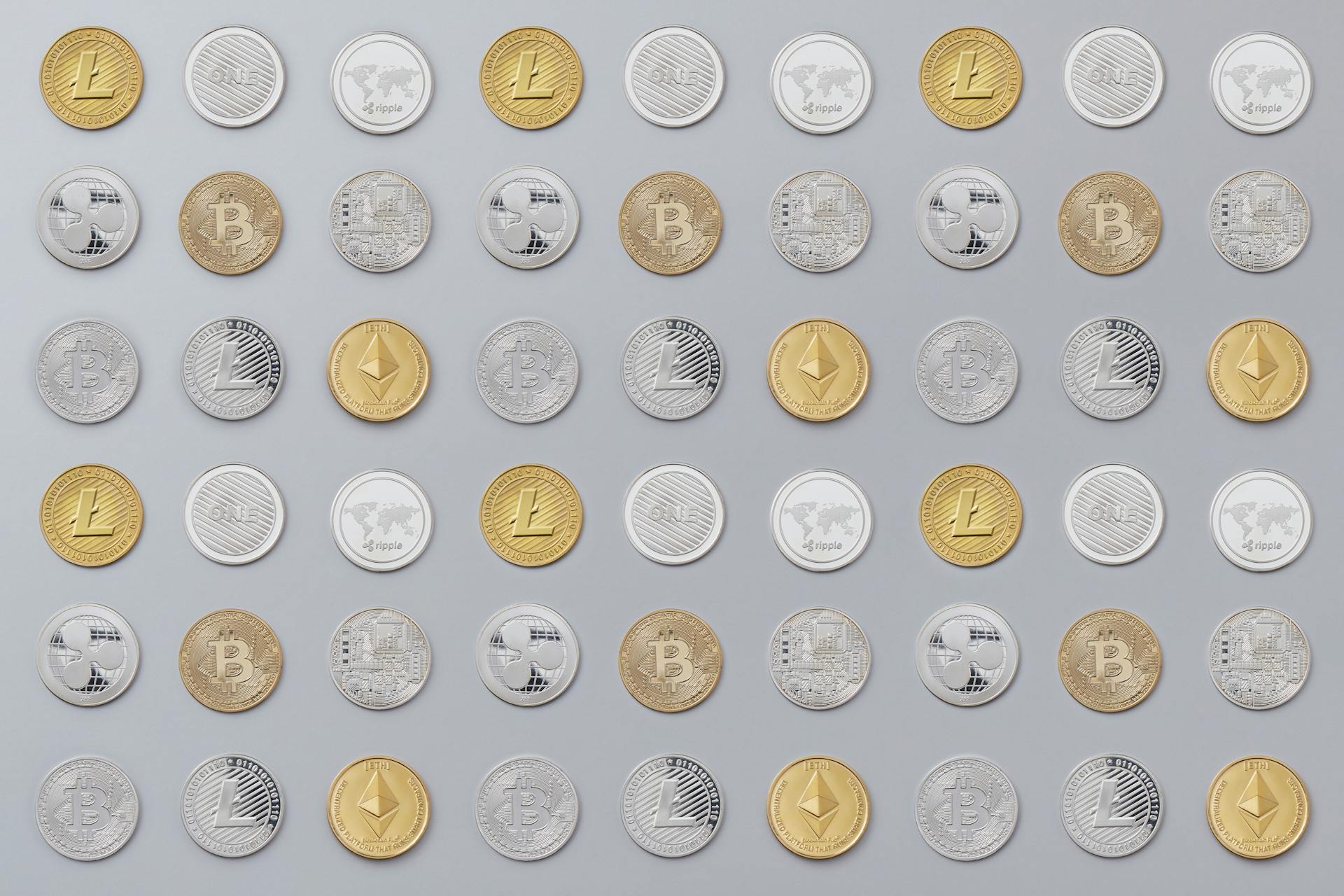
Smart contracts can be built as fully operational Dapp (Decentralized Application) that takes advantage of blockchain-based systems to store data in a decentralized manner. This allows for a high level of transparency and security.
With smart contracts, you can create proof of ownership for digital assets using ERC-721 smart contracts. This ensures that your digital assets are secure and cannot be tampered with.
Smart contracts are also censorship-resistant technology, meaning a central authority cannot temper with recorded data. This is especially important for those who value their online privacy.
Using smart contracts, you can monitor the whole activity of a specific Ethereum account, providing instant traceability. This can be useful for tracking transactions and identifying potential issues.
Smart contracts also offer great transparency, as blockchains' activities can be viewed on block explorers, including smart contracts' code. This allows for a high level of accountability and trust.
The identity behind Web3 activities is just a public address, inherently providing user privacy. This is a significant advantage for those who value their online anonymity.
Smart contracts can store cryptocurrencies such as Ether, providing a secure and decentralized way to store digital assets. This is especially useful for those who want to invest in cryptocurrencies.
Suggestion: Digital Profile
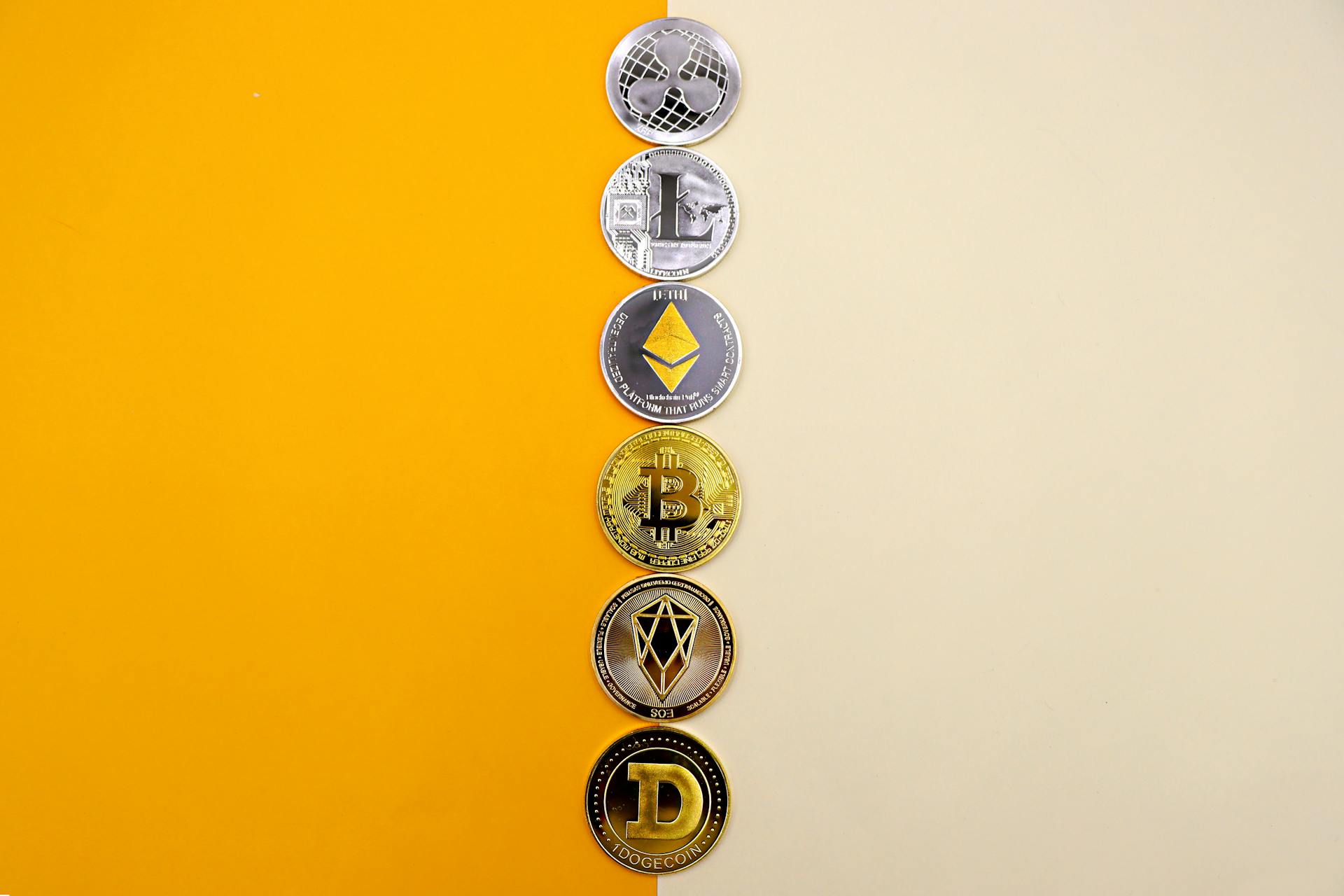
You can write code that triggers payment once specific conditions are met, allowing for conditional, automated payments. For example, you can store Ether in your smart contract and send it to an account 3 years later.
The blockchain is always running, allowing for 24/7 financial operations. This means that users can withdraw money in smart contracts virtually whenever they need to.
Smart contracts can be used as a more stable alternative to gold and traditional savings, providing a storage for inflation-resistant currency. This is especially useful for those who want to protect their assets from inflation.
You can create your own ERC-20 tokens using smart contracts, allowing for tokenization. This can be useful for creating custom tokens for specific use cases.
Most transaction fees associated with smart contracts are lower than those of traditional banking fees. This can help save money on financial transactions.
Consider reading: How to Make Money with Smart Contracts
A Module
To create a Move module, you need an account to publish it. This account's private key is used to create a module under its address and publish it.
See what others are reading: How to Create Your Own Amazon Account
You can create an account by running the command `aptos init --network devnet` in your move directory. This will create a `.aptos` directory with a `config.yaml` file that holds your profile information.
The `config.yaml` file contains your account's private key, public key, and account address. You can see this information by opening the `config.yaml` file.
You can access your account address by going to the Aptos Explorer Devnet network view and pasting the account address value from your configuration file into the search field.
A Move module is stored under an address, and its syntax is `account-address::module-name`. In our example, the account-address is `todolist_addr` and the module-name is `todolist`.
To import the `signer` and `table` modules, which are used in our `signer::` and `table::` statements, you need to add the following use statements at the top of your Move file:
```markdown
use signer::signer;
use table::table;
```
These use statements allow us to use the functions and types from the `signer` and `table` modules in our Move file.
Additional reading: How to Create Astropay Account
List Function
The create_list function is a crucial part of our smart contract, and it's essential to understand its components.
The create_list function accepts a signer, which is injected by the Move VM as the address who signed the transaction.
To create a new TodoList resource, we need to assign it to an account that only this account can have access to.
The signer argument is a key component of this function, and it's used to store the TodoList resource in the provided signer account.
Here's a breakdown of the create_list function's components:
- entry - an entry function is a function that can be called via transactions.
- &signer - The signer argument is injected by the Move VM as the address who signed that transaction.
The create_list function takes in a signer, creates a new TodoList resource, and uses move_to to have the resource stored in the provided signer account.
Task Function
The task function is a crucial part of our contract, allowing accounts to create new tasks. It's essentially a transaction, so we need to know the signer who signed and submitted it.
To create a task, we need to add a create_task function that accepts a signer and task content. This function should have the following logic.
First, we get the signer address to access the TodoList resource. Then, we retrieve the TodoList resource with the signer_address, which gives us access to its properties. We can now increment the task_counter property and create a new Task with the signer_address, counter, and provided content.
Here are the steps to create a task in more detail:
- Get the signer address to access the TodoList resource.
- Retrieve the TodoList resource with the signer_address.
- Increment the task_counter property.
- Create a new Task with the signer_address, counter, and provided content.
- Push the new Task to the todo_list.tasks table.
- Assign the global task_counter to the new incremented counter.
- Emit the task_created event with the new Task data.
The task_created event is emitted using the emit_event function, which accepts a reference to the event handle and a message. In this case, we're passing a reference to the account's TodoList resource and the new Task we just created.
Complete Task Function
The complete task function is a crucial part of our contract, allowing us to mark a task as completed.
We add this function by creating a new function called `complete_task` that accepts a signer and a task ID.
To implement this function, we retrieve the TodoList struct by the signer address, so we can access the tasks table that holds all the account tasks.
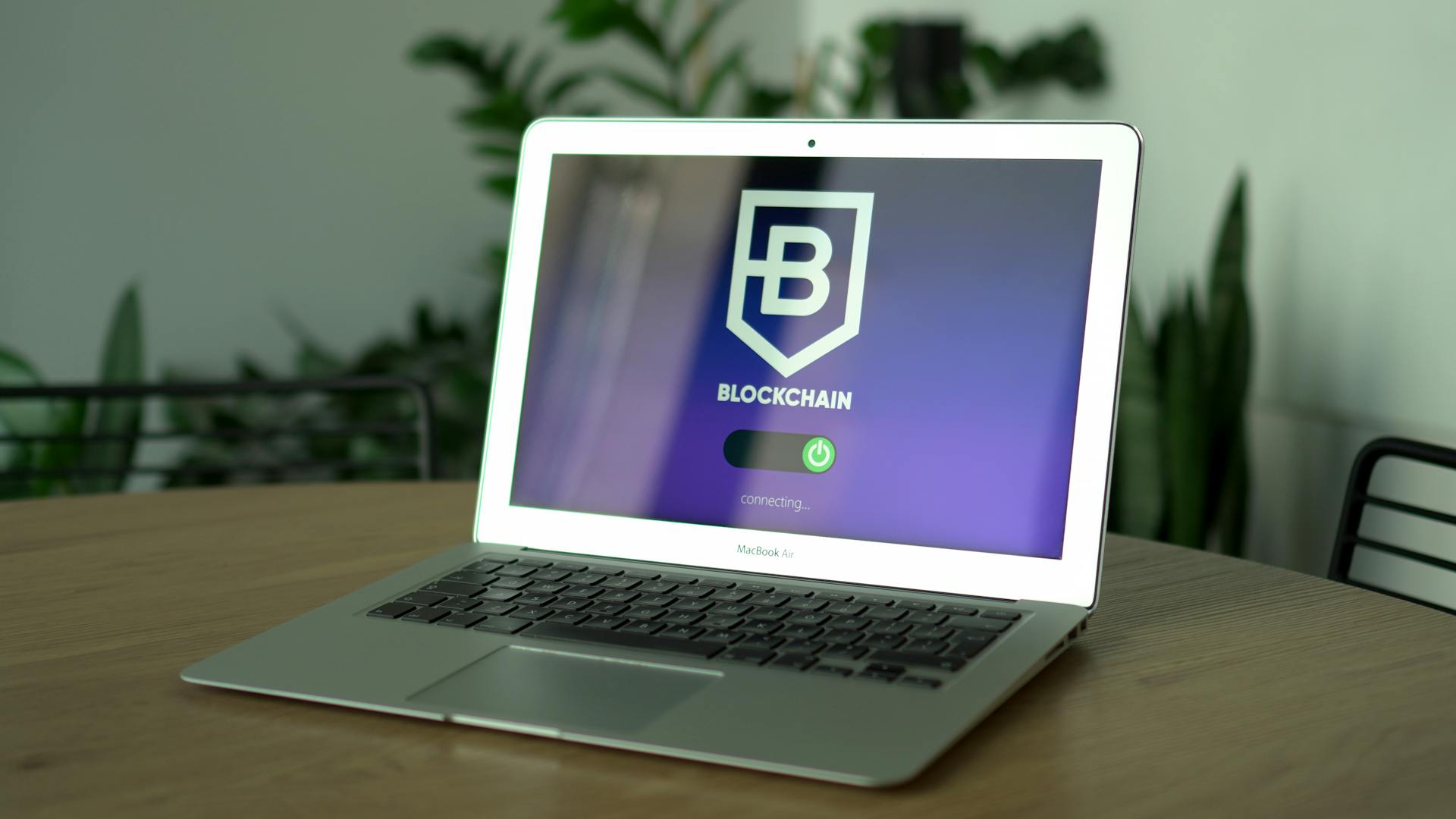
We then look for the task with the provided task ID on the todo_list.tasks table.
Next, we update that task's completed property to be true.
To compile this function, we run the command `aptos move compile`.
However, we might encounter an Unbound error, which we can fix by adding a `use` statement to use the account module.
To do this, we add the following line of code: `use account;`.
Now, we can update our asserts with these constants, which helps us to verify that our function is working correctly.
Here's a step-by-step guide to implementing the complete task function:
1. Add the `complete_task` function to your contract.
2. Retrieve the TodoList struct by the signer address.
3. Look for the task with the provided task ID on the todo_list.tasks table.
4. Update the task's completed property to be true.
5. Compile the function using `aptos move compile`.
6. Add a `use` statement to use the account module if necessary.
Frequently Asked Questions
Can anyone create a smart contract?
Yes, anyone can create a smart contract with the right resources and knowledge, regardless of prior coding experience. With accessible resources and learning opportunities, you can unlock the potential to write your own smart contracts.
Sources
- https://hacken.io/discover/create-smart-contract/
- https://www.bluelabellabs.com/blog/how-to-create-smart-contract/
- https://aptos.dev/en/build/guides/build-e2e-dapp/1-create-smart-contract
- https://docs.substrate.io/tutorials/smart-contracts/develop-a-smart-contract/
- https://wesoftyou.com/web3/how-to-write-a-smart-contract-on-ethereum-and-solidity/
Featured Images: pexels.com