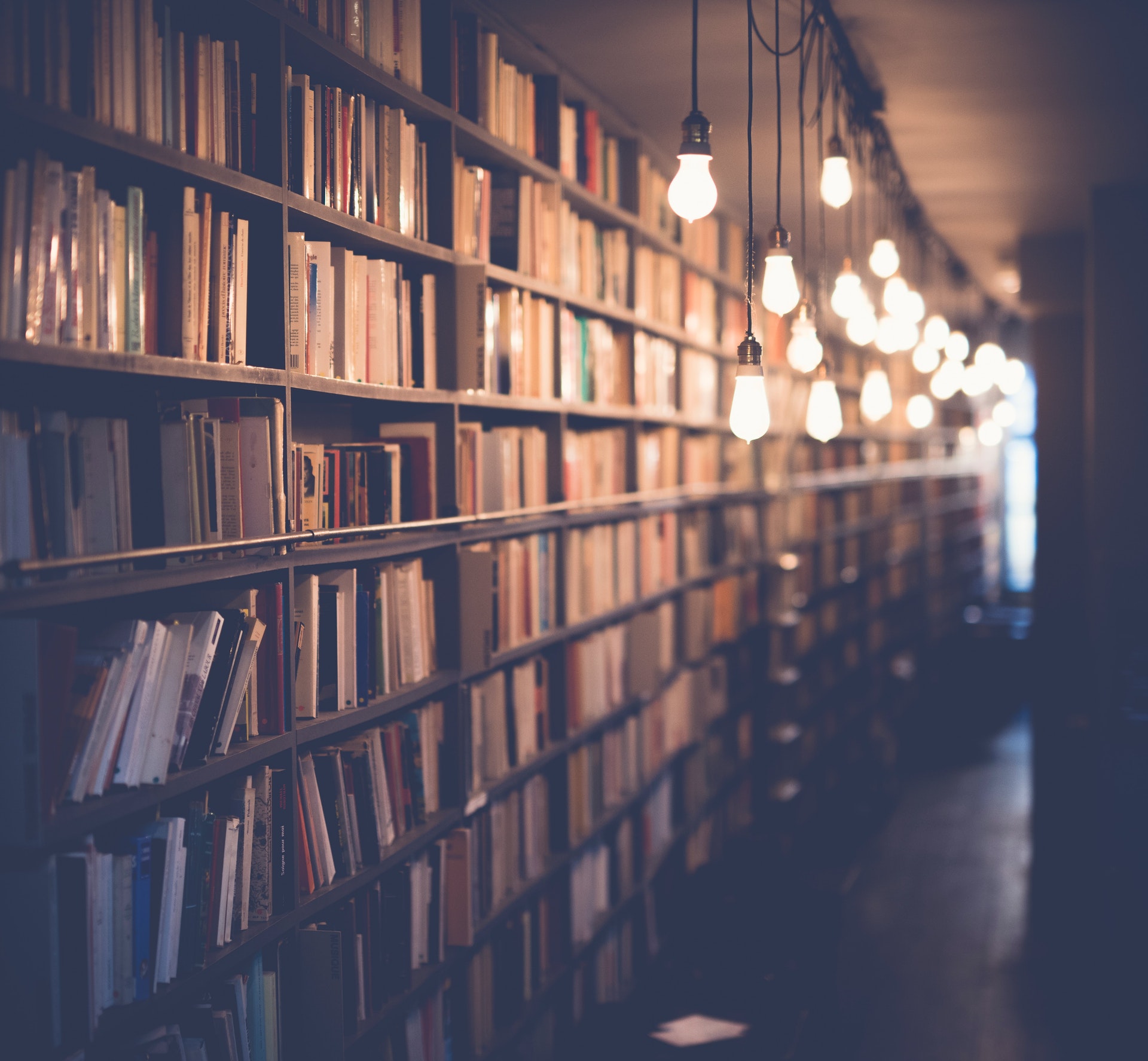
The error message "Can only concatenate str (not "int") to str" is caused by trying to concatenate a string with an integer. This can happen when trying to print a string and an integer together, or when trying to concatenate two strings together where one of the strings is an integer.
The error is caused by trying to use the "+" operator on an integer and a string. The "+" operator is only defined for strings, and cannot be used on other data types. In order to fix this error, you need to convert the integer into a string. This can be done by using the str() function.
str() takes an argument of any data type and returns a string representation of that data type. So, if we have an integer 5 and we want to concatenate it to a string, we can use str(5). This will return the string "5" which we can then concatenate to another string.
Here is an example of how to fix the error "Can only concatenate str (not "int") to str":
int i = 5 print("The value of i is " + str(i))
This will print the string "The value of i is 5".
What is the difference between concatenating a string and an integer?
Concatenating a string and an integer is a process of combining the two objects together so that they form a single entity. This can be done in a number of ways, but the most common method is to simply combine the two objects into a single string. There are a number of advantages to this approach, the most notable being that it is relatively simple and straightforward. Additionally, it can be used to produce a string that is more easily readable or understandable.
However, there are also a number of disadvantages to concatenating a string and an integer. One of the most significant is that the resulting string can be difficult to work with. This is because the string can end up being quite long and unwieldy, making it difficult to manipulate. Additionally, the string can end up being formatted in a way that makes it difficult to read or understand. Finally, if the string is not properly formatted, it can end up being interpreted incorrectly by software.
Why can you only concatenate a string to another string and not an integer?
There are a few reasons why you can only concatenate a string to another string and not an integer. The first reason is that, when you concatenate two strings, the resultant string is composed of the characters of the first string followed by the characters of the second string. However, when you concatenate an integer to a string, the characters of the string are intermingled with the digits of the integer, which can produce unexpected results.
Another reason is that, when you concatenate two strings, the resulting string is a new string that is different from both of the original strings. However, when you concatenate an integer to a string, the resulting string is simply a modification of the original string, with the integer being added to the end of it. This can cause problems if you later try to use the original string, without the integer, as it will now be different from what it originally was.
Lastly, strings are designed to represent human-readable text, whereas integers are designed to represent numbers. This means that it is more natural for a string to be concatenated to another string, as both are designed for human-readable text. However, it is less natural for an integer to be concatenated to a string, as the integer is not designed for human-readable text and so is not as easily converted into a string.
What happens if you try to concatenate a string and an integer?
If you try to concatenate a string and an integer, you will get an error. This is because you cannot concatenate a string and an integer together. You can only concatenate two strings together. If you try to concatenate a string and an integer, the integer will be converted to a string and the two strings will be concatenated together.
How can you concatenate two strings together?
You can concatenate two strings together by using the concatenation operator, which is two backslashes (\\). This operator will take the two strings and return a new string that is the concatenation of the two input strings. For example, if you have two strings, "Hello" and "World", you can concatenate them together using the concatenation operator like this:
"Hello" + "World" = "Hello\\World"
You can also use the concatenation operator to concatenate strings with other data types. For example, you can concatenate a string with an integer like this:
"Hello" + 42 = "Hello\\42"
The concatenation operator can be used on any data type, not just strings. When used on other data types, the operator will first convert the data type to a string before concatenating it.
What is the order of operations for concatenating strings and integers?
Order of operations is important when concatenating strings and integers. If two strings are being concatenated, the order in which they are concatenated does not matter. However, if an integer is being concatenated with a string, the order does matter. The integer must be converted to a string first, before it can be concatenated with the other string.
Can you concatenate multiple strings together?
Yes, you can concatenate multiple strings together. This is known as string concatenation. String concatenation is the process ofjoining two or more strings together. There are many ways to concatenate strings, and the most common is using the + operator. For example, if you have two strings, "Hello" and "World", you can concatenate them together using the following code:
var str1 = "Hello"; var str2 = "World";
var str3 = str1 + str2;
console.log(str3); // Will print "HelloWorld"
As you can see, the + operator simply takes two strings and concatenates them together. However, there are other ways to concatenate strings as well. For example, you can use the += operator to concatenate a string to the end of another string. For example:
var str1 = "Hello"; var str2 = "World";
str1 += str2;
console.log(str1); // Will print "HelloWorld"
You can also use the String.concat() method to concatenate strings. The String.concat() method takes one or more strings as arguments and concatenates them together. For example:
var str1 = "Hello"; var str2 = "World";
var str3 = str1.concat(" ", str2);
console.log(str3); // Will print "Hello World"
As you can see, the String.concat() method is very versatile and can be used to concatenate multiple strings together in a variety of ways.
What is the order of operations for concatenating multiple strings?
Assuming you are referring to the order of operations for string concatenation in programming, here is an outline of the steps involved:
1) Determine the number of strings that need to be concatenated. 2) Allocate memory for the new concatenated string. This is typically done using malloc() in C. 3) Loop through each string to be concatenated and copy it into the new string, one character at a time. 4) Add a null character at the end of the new string to mark the end of the string.
That is the basic process for concatenating strings. There are of course variations and optimizations that can be made depending on the specifics of the situation, but those are the basic steps.
How can you concatenate a string and an integer in Python?
You can concatenate a string and an integer in Python by using the + operator. For example:
>>> "1" + "2" '12'
You can also use the str() function to convert an integer to a string, and then concatenate the two:
>>> str(1) + "2" '12'
How can you concatenate a string and an integer in Java?
Java is a versatile language that allows for a number of different ways to concatenate a string and integer. The most common way to do this is by using the concatenation operator (+), which can be used to add two strings together, or to concatenate a string and an integer. For example, the following code will concatenate the string "Hello" with the integer 12:
String s = "Hello" + 12;
System.out.println(s); //prints "Hello12"
In this example, the concatenation operator is used to add the string "Hello" and the integer 12 together. The result is a new string that contains the characters from both the string and the integer.
Another way to concatenate a string and integer in Java is by using the String.valueOf() method. This method converts an integer (or other data type) into a string, which can then be concatenated with another string. For example, the following code will concatenate the string "Hello" with the integer 12:
String s = "Hello" + String.valueOf(12);
System.out.println(s); //prints "Hello12"
In this example, the String.valueOf() method is used to convert the integer 12 into a string. The resulting string is then concatenated with the string "Hello" using the concatenation operator. The result is a new string that contains the characters from both the string and the integer.
Finally, it is also possible to use the StringBuilder class to concatenate a string and integer. The StringBuilder class provides a number of methods for manipulating strings, including a method for concatenating strings (and other data types). For example, the following code will concatenate the string "Hello" with the integer 12:
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(12);
String s = sb.toString();
System.out.println(s); //prints "Hello12"
In this example, a new StringBuilder object is created. The append() method is then used to concatenate the string "Hello" and the integer 12. The resulting string is stored in a variable named s, and is then printed to the console.
Frequently Asked Questions
Can only concatenate STR(not int) to str in Python?
One way to fix this error is to convert the int to a string, for example: str (my_int) . Another way to solve this problem is to use the int() function and pass the integer value as an argument.
Why can't I concatenate a string and an integer in Python?
Integer and string can't be concatenated because they are of different types.
Why can't I concatenate an employee variable to a string?
The error is coming from the employee variable being an integer, which Python can't handle as a string. You'll need to convert it to a string using the str() function.
Why do I get error when adding a float to string?
When you try to add a float to a string, Python's built-in str.format method will not allow you to concatenate floating point values together. To do this, you'll need to use the format string "%f" instead. This can be done like so: str = "The value of %s is %f" % (name, value) Note that when printing out strings, you might want to use the print Statement Formatter if you are wanting precision or multiple lines of output.
What are F-strings in Python?
F-strings are lines in a string that contain interpolated expressions. They look like this: "Python f-string". The expression that is interpolated will be evaluated within the context of the string and the results will be assigned to the variable that is specified as the value for the f variable. There are a few important things to keep in mind when working with F-strings in Python: They can be used anywhere that strings can be used. This includes withinitute strings, function arguments, and even comments. They support most of the standard string operations, including concatenation, substitution, and iteration. They have their own special evaluation context which means they can access values stored in variables that are defined inside of them.
Sources
- https://www.pythonpool.com/solved-typeerror-can-only-concatenate-str-not-int-to-str/
- https://bobbyhadz.com/blog/python-typeerror-can-only-concatenate-str-not-int-to-str
- https://blog.csdn.net/qq_46620129/article/details/115017140
- https://blog.csdn.net/df0128/article/details/88245607
- https://itsmycode.com/typeerror-can-only-concatenate-str-not-int-to-str/
- https://pyquestions.com/concatenating-string-and-integer-in-python
- https://www.geeksforgeeks.org/difference-between-concatenation-of-strings-using-str-s-and-str-str-s/
- https://www.c-sharpcorner.com/blogs/difference-between-concat-and-string-concatenation-operator-in-java
- https://www.quora.com/What-is-the-difference-between-an-integer-and-a-string
- https://www.geeksforgeeks.org/difference-between-concat-and-operator-in-java/
- https://www.reddit.com/r/learnpython/comments/uzkkgf/why_does_python_only_concatenate_string_to_string/
- https://pytutorial.com/typeerror-can-only-concatenate-str-not-int
- https://www.c-sharpcorner.com/article/6-effective-ways-to-concatenate-strings-in-c-sharp-and-net-core/
- https://www.geeksforgeeks.org/happen-concatenate-two-string-literals-c/
- https://technical-qa.com/can-you-concatenate-int-and-string/
- https://www.digitalocean.com/community/tutorials/python-concatenate-string-and-int
- https://stackoverflow.com/questions/32984737/what-will-happen-if-int-concatenate-with-string-in-php
- https://communities.sas.com/t5/New-SAS-User/SAS-concatenate-string-and-integer/td-p/627834
- https://www.tutorialspoint.com/concatenate-string-to-an-int-value-in-java
- https://forum.freecodecamp.org/t/python-combine-strings-to-integer/336827
- https://www.programiz.com/c-programming/examples/concatenate-string
- https://www.educative.io/blog/concatenate-string-c
- https://www.ablebits.com/office-addins-blog/excel-concatenate-strings-cells-columns/
- https://stackoverflow.com/questions/8465006/how-do-i-concatenate-two-strings-in-c
- https://stackoverflow.com/questions/668032/what-is-the-order-of-operations-with-this-concatenation
Featured Images: pexels.com