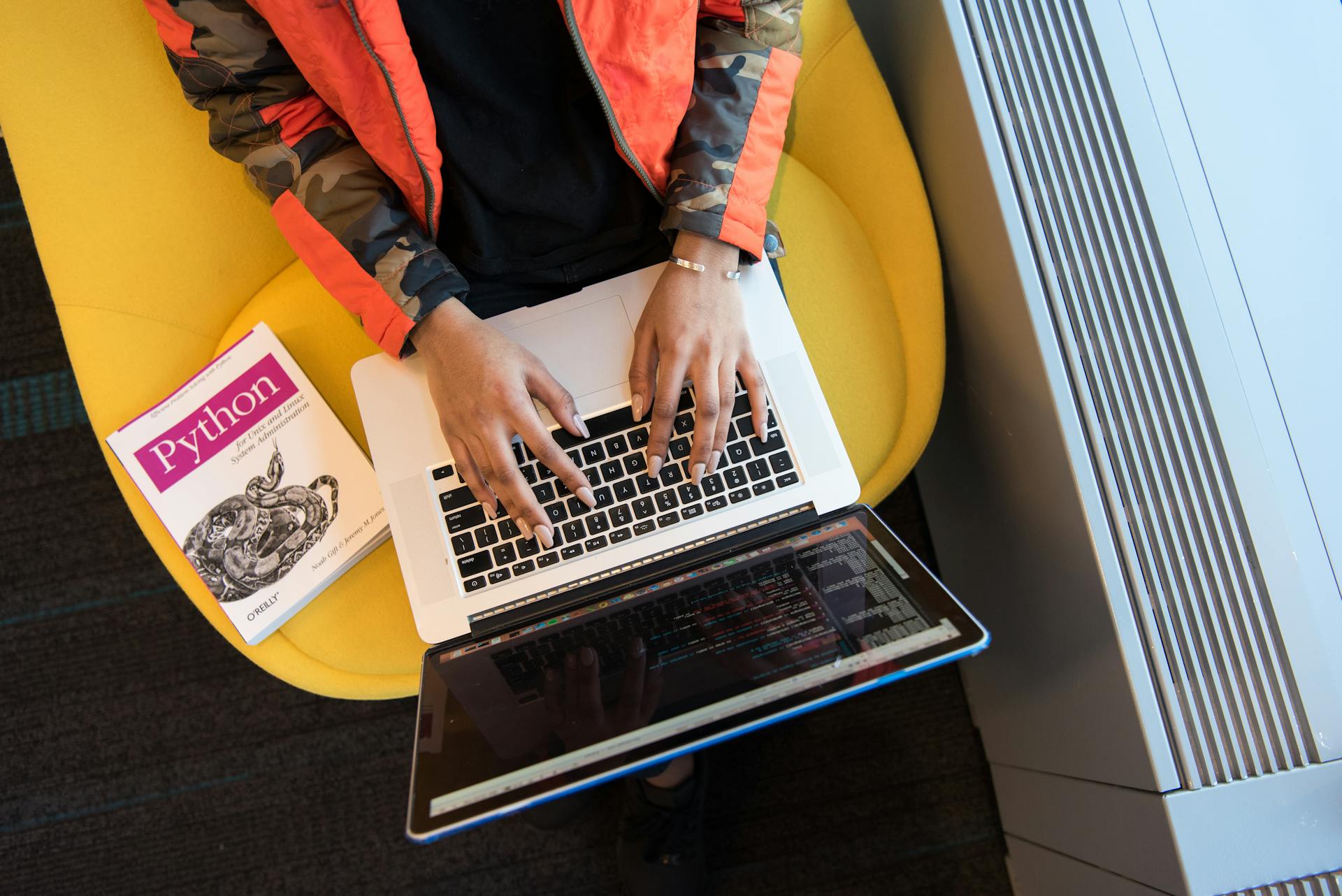
Building a Python trading bot requires a strong foundation in programming and a solid understanding of financial markets.
To get started, you'll need to install the necessary libraries, including backtrader and yfinance.
You can then use these libraries to connect to financial APIs and retrieve historical price data.
Here are the key steps to building a Python trading bot:
First, you'll need to define your trading strategy, including the indicators and rules you'll use to make trades.
Next, you'll need to implement the trading logic in your code, using libraries like backtrader to handle the execution of trades.
Finally, you'll need to backtest your strategy using historical price data to ensure it's effective.
Backtesting is a crucial step in building a successful trading bot, as it allows you to evaluate the performance of your strategy in different market conditions.
Getting Started
Python is a popular programming language for building trading bots due to its simplicity and extensive libraries.
To start building a Python trading bot, you'll need to install the necessary libraries, including backtrader and yfinance.
Choose a reliable data source for your trading bot, such as Yahoo Finance, which provides historical stock prices.
Configure Environment
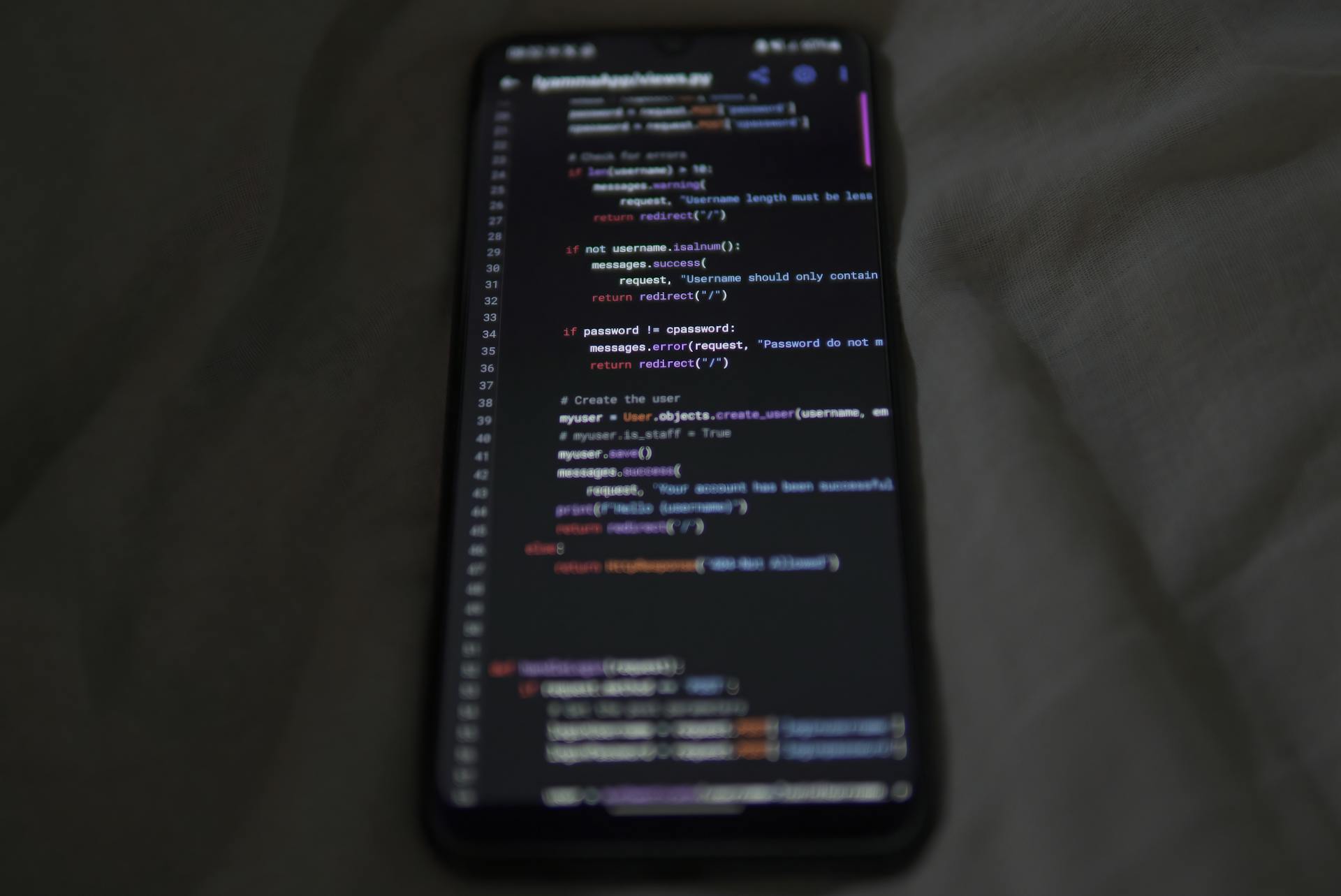
To get started with your Python trading bot, you need to set up your environment first. This means getting the right tools and libraries to help you connect with brokers and make trades smooth and fast.
Python libraries are like toolboxes full of ways to do jobs, and for trading bots, there are many. They help make it easy to talk to different broker APIs.
Some popular Python libraries for trading bots include Alpaca API, Interactive Brokers API, and TD Ameritrade API. These libraries allow you to connect your bot to sites like Alpaca, Interactive Brokers, or TD Ameritrade.
For example, you can use the Alpaca API to look at real market data, try trades with fake money, and trade for real. The Interactive Brokers API lets you trade in many markets, like stocks and futures, and get special orders and market news in real-time. The TD Ameritrade API helps with stocks, options, and ETFs trades, and gives you old and new market info, helps place orders, and manage your account.
Here's a quick rundown of some popular tools to consider:
These libraries and tools can make making and checking your bot easier, freeing you up to make better strategies.
Why Python Is Widely Used
Python is widely used for building trading bots due to its ease of use.
Python's clear language makes it easy for developers to write neat code, saving time and making the work easier.
Its extensive library ecosystem is a major advantage, with libraries like Alpaca, Interactive Brokers, and TD Ameritrade making connecting to brokers and trading simple.
Python's versatility allows it to be used for simple trading plans or complex algorithms, making it a top pick for traders who use algorithms.
Python has a big community and lots of help online, with developers and traders sharing tips and working on projects together.
Thanks to its simple use, extensive library ecosystem, and active community, Python is perfect for all levels of coders.
Understanding Basics
Before coding your Python trading bot, it's essential to understand the basics of your trading plan. This plan outlines when to enter and exit trades.
Know what market information to use, such as price and volume, to help your algorithm make informed decisions. Moving averages can be a great tool to spot trends, and your algorithm can buy or sell based on those trends.
A trading strategy could target trends and short-term prices, buying when the price is above its short-term average and selling when it drops below that average, suggesting a downtrend.
Installing for Financial Data
To get started with trading, you need to set up your algorithm with the right market info. This includes deciding what data to use, such as price and volume.
The S&P 500 is a good example of a stock market index that provides valuable data for trading.
For testing purposes, you can use paper trading with fake money to evaluate your algorithm's effectiveness.
To backtest your algorithm, you'll need to use past market data. This will help you understand how your algorithm would have performed in different market conditions.
Here's a list of some helpful terms to keep in mind:
- Paper Trading: The trading of securities with fake money for educational or testing purposes.
- Backtesting: Testing a trading algorithm against past market data in order to evaluate its effectiveness.
- Closing Price: The final price of a security during a unit of time
Remember, your trading plan should match your algorithm, and it should be based on the basics of trading.
What Is a
So, you're wondering what a basic is? Well, a basic is a fundamental element or principle that serves as a foundation for something.
In the context of understanding basics, a basic is often a simple concept or idea that is easy to grasp.
A basic can be a building block for more complex things, like a puzzle piece that fits together with other pieces to create a complete picture.
The concept of a basic is often used in various fields, such as science, technology, engineering, and math (STEM) education, where it's essential to understand the basics of a subject before moving on to more advanced topics.
Understanding the basics of a subject can make it easier to learn and retain new information, and it can also help you identify patterns and relationships between different concepts.
Building a Trading Bot
Building a trading bot is within your reach, and Python is the perfect tool for the job. You can now build your own trading bot using Python.
Using packages like pandas and robin-stocks, you can easily visualize the performance of individual holdings within your portfolio. This allows you to make informed decisions about your investments.
The buy and sell conditions set for the bot are relatively simplistic, but this code provides the building blocks for creating a more sophisticated algorithm.
Connecting to APIs
Connecting to APIs is a crucial step in building a Python trading bot. You'll need to use libraries to link your bot with a broker's API, allowing you to communicate with the broker's platform.
To connect to a broker's API, you'll need to understand their guide and rules. This includes obtaining an API key for access.
Using a broker's API lets your bot trade automatically, bringing faster execution and potentially more profit without manual intervention.
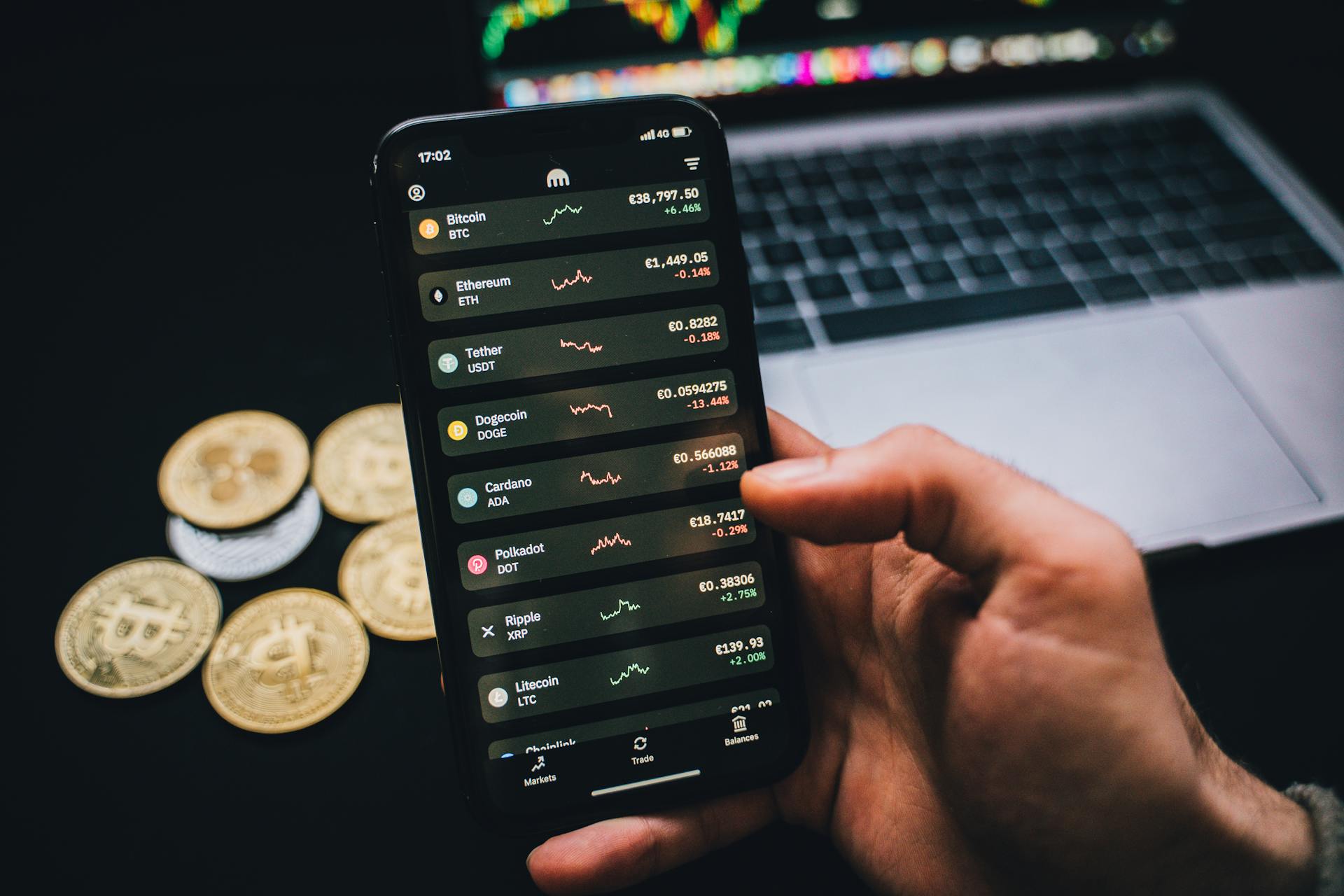
APIs provide real-time market data access, automated execution, portfolio management, customizable strategies, and risk management. These benefits make connecting to a broker's API essential for smart, automated trading.
Here are some well-known APIs for Python:
By connecting to a broker's API, you can execute trades without manual intervention, ensuring faster order execution and efficient portfolio management. This is a great way to automate your trading and try out different strategies with real market data.
Data and Indicators
To fetch data and create technical indicators, you'll need to have Python installed on your local computer or use Google Colab, which requires no setup and is free of charge.
Having access to a Python environment is outside the scope of this article, but using Google Colab is a great alternative. The yfinance library is also a must-have for retrieving financial information, including historical price data and financial statement data.
You can create a simple example snippet to check if the library is installed correctly and to show the basics, like fetching 1-minute bars for the most recent day for a stock like Amazon.
Fetching Data and Creating Indicators
To fetch data and create indicators, you'll need to have Python installed on your local computer or use a platform like Google Colab. The most popular Python library for retrieving financial information is YFinance, which provides access to historical price data and other datasets.
YFinance is a powerful tool that can be used to retrieve data from Yahoo Finance, but it requires some setup. You'll need to install it using pip, the Python package manager. Once installed, you can use it to fetch data for stocks like Amazon.
To get started with YFinance, create a simple example snippet to test if the library is installed correctly. This can be done by importing the library, creating a Ticker object for Amazon, and using it to fetch 1-minute bars for the most recent day. The history() function returns a pandas DataFrame with OHLC data that can be manipulated to get the desired information.
You can use the pandas_ta library to calculate technical indicators like moving averages without coding them yourself. This library provides a range of functionalities that can be leveraged to quickly implement dozens of technical indicators. To add two new columns to your DataFrame, use the pandas_ta library to calculate the fast and slow moving averages. The fast SMA uses the ten most recent price bars, while the slow one uses thirty minutes.
Reading Market Data
Reading market data is crucial for making informed decisions in trading. To do this, you can use APIs like Alpaca, which allows you to access real-time market data in Python.
To get started, you'll need to create an account with a trading platform that supports the API you're using, such as Robinhood, which offers a commission-free investing platform.
Once you have your account set up, you can use the API to extract information about your portfolio, including your holdings and profile information. For example, you can use the profiles module in the robin-stocks package to access your profile information.
Building a visualization tool is also essential for observing historical changes in a given stock. This can be done by creating a function that plots the opening price, high and low price over a specified time period.
When evaluating your bot's performance, it's essential to consider the market's stability and your bot's strategy. By regularly checking on your bot's performance and adjusting its strategy as needed, you can lower risks and earn more money.
You can customize the input ticker list when building a visualization tool, or use a function to extract tickers from your holdings. This allows you to focus on the specific stocks you're interested in.
Algorithm and Strategy
To create a Python trading bot, you need a solid trading strategy. This involves defining your trading plans, such as knowing when to buy or sell, and choosing a strategy that aligns with your risk comfort and time commitment.
Some popular strategies include momentum trading, mean reversion, and trend following. To test your trading plan, use past market data to see how your strategy would've performed. This can help you identify potential weaknesses and make adjustments before going live.
A good trading strategy is based on a well-researched and tested approach, removing emotions and uncertainties. This systematic method helps make informed trading decisions.
Here are some key considerations for your trading strategy:
- What's your goal with money and how much risk can you stand?
- What markets or items will you trade?
- What signals will you use to know when to buy or sell?
- How will you manage risks and set safety nets?
- What about big market news or new data?
What Is a Strategy?
A strategy is the foundation of any trading plan. To define a trading strategy, make rules for buying or selling.
Strategies can be based on various principles, such as trend following or mean reversion. Trend following involves identifying and following the direction of a market trend, while mean reversion involves buying when prices are low and selling when they're high.
Defining clear rules for buying or selling is essential to a well-structured trading strategy. This helps traders make informed decisions and avoid emotional impulse buys.
Writing Your Algorithm
Writing a trading algorithm is a crucial step in creating a successful trading bot. This algorithm will guide your bot to make smart choices by looking at market data and following your trading plan.
To write a trading algorithm, you'll need to mix your strategy with real market data. This includes prices, volume, and order book details.
Your algorithm should be based on a well-researched and tested approach that removes emotions and uncertainties. It should provide a systematic method for making informed trading decisions.
Here are some key elements to consider when writing your algorithm:
- Define your trading strategy: This could be momentum trading, mean reversion, or trend following.
- Choose your risk management techniques: These include setting stop-loss orders, determining position sizing, and establishing risk-reward ratios.
- Use backtesting to check your strategy: This involves simulating your algorithm using historical data to assess its performance.
By following these steps, you'll be well on your way to creating a robust and effective trading algorithm.
Some popular strategies include:
Remember to test your trading plan well and use past market data to see how your strategy would have done. This will help you fine-tune your plan and make adjustments as needed.
Risk Management
Risk management is key for any trading strategy's success, helping traders avoid big losses. Implementing risk management is essential to prevent significant financial losses.
Setting stop-loss orders is a crucial aspect of risk management. This action sells a security if its price drops too low, allowing traders to stop big losses. Traders need to pick the right stop-loss based on their risk level and the security's price changes.
Position sizing decides how much money goes into each trade, managing the size of trades vs. the risk. This keeps losses from being too big, and traders do this to avoid losing a lot of money on one trade.
Risk-reward ratios look at possible gains vs. risks in a trade, aiming for a good balance. Traders want the wins to be bigger than the losses, making their strategy likely to win overall.
Performance and Evaluation
Monitoring your Python trading bot's performance is crucial to its success. You want to make sure it's doing well at buying and selling and making money.
A big thing to watch is how many trades make a profit. This tells us if the bot is doing a good job at making money.
You should check how much money it makes compared to how much you put in. This helps you know if your bot is working.
It's key to track key stats like winning trades and investment returns. This checks your bot's success.
The following performance metrics should be looked at to know how your bot is doing:
Always checking on how well your Python trading bot is doing and fixing its strategy and code is key to making steady money.
Incorporating Advanced Techniques
Incorporating advanced techniques into your Python trading bot can make it a lot smarter.
Machine learning is a key advanced technique you can use.
This allows your bot to look at old data, spot trends, and make better guesses.
With a lot of market info to learn from, your bot can find trends and weird things in the market.
Using machine learning tricks makes your bot sharper and able to find good deals.
Advanced tools like machine learning can improve forecasting and choices in your bot.
These techniques can make your guesses more on point and help you understand the market more deeply.
Buying and Selling
Buying and selling stocks is a crucial part of a Python trading bot. You can use libraries like Alpaca Trading to buy and sell stocks in Python.
A simple strategy is to buy and sell whenever the 5 minute moving average crosses the price. This is not a good trading strategy, but it's a good way to focus on the general structure of a trading bot.
You can interface with a stock broker or a crypto exchange using libraries like Alpaca, Binance, or Interactive Brokers. These libraries are versatile and can perform requests without much refactoring.
Here are some popular libraries you can use for trading:
- Alpaca Trading
- Binance
- Interactive Brokers
Remember to manually create orders to ensure your bot behaves as expected, especially in the first versions.
Frequently Asked Questions
Are trading bots really profitable?
Profitability of trading bots depends on their configuration, but with proper setup and testing, they can be a lucrative investment opportunity
Sources
- https://kritjunsree.medium.com/building-a-trading-bot-in-python-a-step-by-step-guide-with-examples-6898244016cd
- https://www.activestate.com/blog/how-to-build-an-algorithmic-trading-bot/
- https://medium.com/@genedarocha/136-automated-trading-bots-with-python-creating-and-testing-your-bot-ffce2f4cbdeb
- https://mayerkrebs.com/create-a-trading-bot-in-python-and-yfinance/
- https://codesphere.com/articles/how-to-build-a-stock-trading-bot-with-python-2
Featured Images: pexels.com