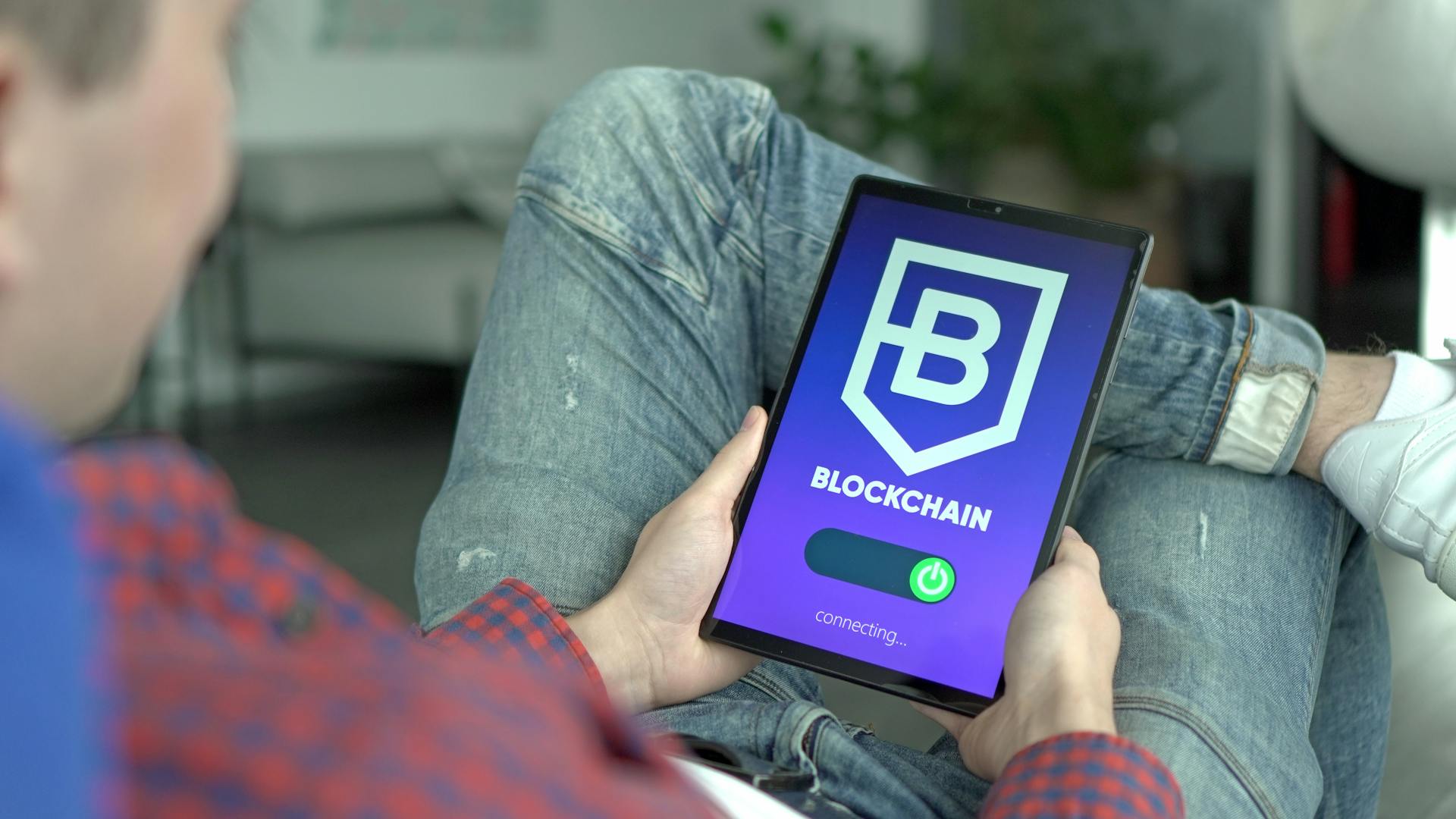
Smart contract solidity development is a complex process that requires a deep understanding of programming languages, blockchain technology, and smart contract protocols.
The most widely used programming language for smart contract solidity development is Solidity, which is designed specifically for Ethereum-based smart contracts.
To get started with smart contract solidity development, you'll need to have a good grasp of the Solidity language and its syntax.
In the Solidity language, variables are declared using the `var` keyword, and functions are declared using the `function` keyword.
Curious to learn more? Check out: Smart Contracts Development Uae
Setting Up the Environment
To set up the environment for smart contract development in Solidity, you'll need to install Node.js, which will serve as the foundation for your development process.
Install Truffle Suite, a widely recognized development framework for Ethereum, using npm install -g truffle. Truffle simplifies the process of building and deploying smart contracts.
Choose a code editor like Visual Studio Code or Atom, and install Solidity plugins/extensions for syntax highlighting and code completion. This will enhance your coding efficiency and make development a breeze.
Here's an interesting read: Crypto Token Development Services
Download Ganache, a personal Ethereum blockchain, from the Truffle Suite website. Ganache allows you to deploy contracts, develop applications, and run tests in a controlled environment.
In your terminal, navigate to the directory where you want to create your project and run truffle init. This command sets up a new Truffle project with the necessary directory structure.
Here are some popular development environments for Solidity:
You can also use a simple text editor like nano (Linux/Unix), TextEdit (macOS), or NotePad (Windows) to write and compile your Solidity programs. Save your program source code with a .sol extension and it will be recognized by the Solidity compiler.
Development
To develop smart contracts in Solidity, you'll need a development environment. There are several options available, including text editors like Emacs, Vim, and Atom, which offer features like syntax highlighting and macros to make development easier.
For a more web-based experience, you can use development environments like Remix IDE and EthFiddle. Alternatively, you can use a simple text editor like nano, TextEdit, or NotePad, as long as you save your program source code with a .sol extension.
Some popular development IDEs for smart contract development include Microsoft Visual Studio Code, JetBrains IntelliJ, Remix Online Editor, and EthFiddle Online Editor.
Additional reading: Crypto Wallet Development Cost
Basics
Developing smart contracts on the Ethereum blockchain requires a solid foundation in Solidity.
Solidity is a statically typed programming language designed for this purpose.
Understanding its basic concepts is crucial for effective development, and it's a must-know for any developer in this field.
Solidity's syntax is similar to JavaScript, making it easier for developers to learn and adapt.
The language is designed to be readable and maintainable, even for complex smart contracts.
The example of the Transfer event shows how Solidity is used to track transactions: "event Transfer(address indexed from, address indexed to, uint256 value);".
This event is triggered whenever a transfer occurs, and it provides valuable information for developers to work with.
The use of Solidity's indexing feature allows for efficient querying and indexing of data.
This is particularly useful in large-scale smart contract deployments.
The language's focus on security and modularity makes it an ideal choice for developing robust smart contracts.
Developers can leverage Solidity's features to create complex logic and rules within their contracts.
For more insights, see: Ethereum Contract Address
File Structure
When organizing your code, it's essential to understand the file structure of a Solidity project. A typical Truffle project includes a contracts directory where your smart contracts reside.
The contracts directory is a crucial part of any Solidity project. It's where you'll find your smart contract code.
A Truffle project also includes a migrations directory. This is where you'll find scripts to deploy your contracts to the blockchain.
The test directory is where you'll write unit tests for your smart contracts. Writing tests is a crucial part of ensuring your contracts work as expected.
In a Truffle project, you'll also find a truffle-config.js file. This file contains configuration settings for your project.
A package.json file is also a standard part of a Truffle project. This file lists dependencies and scripts for your project.
Here's a breakdown of the typical directories and files you'll find in a Truffle project:
- contracts/:
- migrations/:
- test/:
- truffle-config.js:
- package.json:
Writing Your First Smart Contract
Writing your first smart contract can be a daunting task, but with the right tools and knowledge, you'll be deploying contracts in no time. To start, you'll need to set up your development environment.
Create a new Truffle project, which will provide you with a solid foundation for building and deploying your contract. This involves setting up a new project directory and installing the necessary dependencies.
To write your smart contract, you'll need to create a new file in the contracts directory, for example, MyContract.sol. This is where you'll define the logic and functionality of your contract. Don't worry if this sounds overwhelming – with practice, you'll become more comfortable with the process.
Here are the basic steps to get started:
- Set up your development environment.
- Create a new Truffle project.
- Write your smart contract in the contracts directory.
Remember, the key to writing a successful smart contract is to take it one step at a time and focus on understanding the basics of Solidity. With patience and practice, you'll be writing contracts like a pro in no time.
Constructors
A constructor is an optional function declared with the constructor keyword, which is executed upon contract creation, and where you can run contract initialization code.
Before the constructor code is executed, state variables are initialized to their specified value if you initialize them inline, or their default value if you do not.
The deployment of the code costs additional gas linear to the length of the code, and it includes all functions that are part of the public interface and all functions that are reachable from there through function calls.
If there is no constructor, the contract will assume the default constructor, which is equivalent to constructor(){}.
You can use internal parameters in a constructor, but the contract has to be marked abstract, because these parameters cannot be assigned valid values from outside but only through the constructors of derived contracts.
Prior to version 0.4.22, constructors were defined as functions with the same name as the contract, but this syntax was deprecated and is not allowed anymore in version 0.5.0.
Prior to version 0.7.0, you had to specify the visibility of constructors as either internal or public.
Constructors can be called following the linearization rules, and if the base constructors have arguments, derived contracts need to specify all of them.
You can specify constructor arguments in two ways: directly in the inheritance list or in the way a modifier is invoked as part of the derived constructor.
Recommended read: Public Blockchain
Steps for Integration
To set up a testing environment for integration testing, you'll need to use Truffle and Ganache, as mentioned in Example 3. This will help you simulate real-world scenarios involving multiple contracts.
To write integration tests, you'll need to simulate real-world scenarios involving multiple contracts, such as the example provided in Example 3. This will ensure that your contracts interact correctly with each other.
Here's an example of how to write an integration test:
```javascript
const Token = artifacts.require("Token");
const Exchange = artifacts.require("Exchange");
contract("Exchange", accounts => {
let tokenInstance;
let exchangeInstance;
before(async () => {
tokenInstance = await Token.new();
exchangeInstance = await Exchange.new(tokenInstance.address);
});
it("should allow users to trade tokens", async () => {
await tokenInstance.mint(accounts[1], 100);
await tokenInstance.approve(exchangeInstance.address, 100, { from: accounts[1] });
await exchangeInstance.trade(100, { from: accounts[1] });
const balance = await tokenInstance.balanceOf(accounts[1]);
assert.equal(balance.toNumber(), 0, "Tokens were not traded correctly");
});
});
```
To run the integration tests, you'll need to execute the code as shown in Example 3. This will help you identify any issues with the interaction between your contracts.
By following these steps, you'll be able to write effective integration tests that ensure your contracts work together as intended.
You might enjoy: Smart Contracts Real Estate
Estimating Gas Cost
Estimating Gas Cost is crucial for smart contract development on the Ethereum network. To estimate the gas necessary to execute a contract method, use the gasEstimate function.
This function provides an estimate, as the Ethereum Virtual Machine (EVM) is Turing complete, making it possible to create functions with vastly different gas costs.
To obtain the gas price from the network, use the web3.eth.getGasPrice() function.
You can then estimate the gas cost by multiplying the gas estimate by the gas price.
Here's a simple example of how to do this:
It's essential to evaluate the gas cost of functions as part of your development workflow to avoid surprises when deploying contracts to the mainnet.
Life Cycle
The life cycle of development is a fascinating process. It starts with the initial idea, which can come from anywhere, even a simple conversation with a friend.
In the concept stage, ideas are explored and refined, often with the help of research and analysis. This stage can be lengthy, but it's crucial for laying the groundwork for future growth.
A good idea can come from anywhere, but it needs to be nurtured and developed to reach its full potential. This is where the concept stage comes in, where ideas are refined and made more concrete.
The design stage is where the concept becomes a tangible plan, with specific goals and objectives. This is where the idea starts to take shape and becomes more concrete.
Design is not just about aesthetics; it's also about functionality and usability. A well-designed product or service should be easy to use and understand.
The development stage is where the design becomes a reality, with coding, testing, and iteration. This is the most hands-on stage of the life cycle, where the idea is brought to life.
Development is a iterative process, with continuous testing and refinement. This is where the idea is refined and polished until it's ready for launch.
The launch stage is where the product or service is made available to the public. This is the culmination of all the hard work and planning that went into the development process.
Launch is just the beginning, as the product or service needs to be marketed and maintained to reach its full potential. This is where the real work begins, as the product or service needs to be updated and improved to stay relevant.
Recommended read: Smart Bulbs Work
Solidity Syntax
Solidity syntax is a crucial part of writing smart contracts. It's based on the JavaScript syntax and is used by the Solidity compiler to translate the code into bytecode that can be executed by the Ethereum Virtual Machine (EVM).
In Solidity, variables are declared using the `var` keyword, and their type is specified after the variable name. For example, `var x uint256;` declares a variable `x` of type `uint256`.
You can also use the `uint` keyword to declare unsigned integers. The `uint` keyword is a shorthand for `uint256`, which is the most common type of unsigned integer in Solidity.
Syntax and Data Types
Solidity supports generic value types, specifically booleans, integers, addresses, string literals, and modifiers. This is crucial for developing decentralized applications (DApps) that interact with smart contracts.
In Solidity, you can use boolean values, true or false, with logical operators like ! (not), && (and), || (or), == (equal), and != (not equal). This is useful for making decisions in your smart contract code.
Related reading: What Is Not a Smart Way to Negotiate?
Fixed-point numbers in Solidity are declared with (u)fixedMxN, where M is the size in bits (increments of 8 up to 256) and N is the number of decimals after the point (up to 18). For example, ufixed32x2.
Solidity also offers a variety of value literals, including seconds, minutes, hours, and days, which can be used to convert to multiples of the base unit seconds. Similarly, wei, finney, szabo, and ether can be used to convert to multiples of the base unit wei.
Here are the basic data types offered in Solidity:
Properly managing the data returned by smart contract functions is crucial for developing DApps that provide a good user experience. This involves understanding the data structure, using libraries like web3.js or ethers.js, and implementing error handling.
For your interest: Ethereum Data Dashboard
Base Constructors
Base constructors in Solidity are crucial for setting up a contract's behavior, and they must be called following specific rules.
If a base contract has a constructor with arguments, they must be specified in the derived contract. This can be done directly in the inheritance list or in a modifier-style in the derived constructor.
Specifying arguments in both places is an error. If a derived contract doesn't specify all the base contract's constructor arguments, it must be declared abstract.
The linearization rules for calling base constructors are essential to understand, and they can be complex. But don't worry, with practice, you'll get the hang of it.
In the example of the Faucet contract, the constructor sets the owner variable with the address from msg.sender. This is because the msg.sender in the constructor is the EOA or contract address that initiated contract creation.
Variables and Storage
In smart contract solidity, variables are used to store data. This data can be accessed and modified using functions.
A simple storage contract is an excellent way to understand how variables and storage work in solidity. This contract, as seen in the example, allows you to store and retrieve a single integer value.
Variables in solidity can be declared using the `uint256` data type, as shown in the example contract. This data type is used to store unsigned integers of 256 bits.
The contract also includes a `storedData` variable, which is used to store the integer value. This variable is updated using the `set` function and retrieved using the `get` function.
Integers
Integers are a fundamental data type in Solidity, and they're used to store whole numbers. They can be either signed or unsigned, and the default storage size is 256 bits.
You can allocate a specific storage size for integers by using keywords like uint8 and uint256. For example, uint8 allocates 8 bits of storage, while uint256 allocates 256 bits.
The operators you can use with integers include comparisons like <= and ==, as well as arithmetic operators like + and *. You can also use bit operators like & and |.
Here are some common arithmetic operators used with integers:
Note that the modulus operator (%) returns a Runtime Exception when applied to the zero of a "divide by zero" operation.
State Variable Visibility
State variables in smart contracts have different levels of visibility, which affect how they can be accessed and modified.
Public state variables have getter functions automatically generated by the compiler, allowing other contracts to read their values, but not modify them.
Internal state variables can only be accessed from within the contract they're defined in and its derived contracts. This is the default visibility level for state variables.
Private state variables are similar to internal ones but aren't visible in derived contracts. They're still visible to the world outside the blockchain.
Making something private or internal doesn't prevent it from being visible to the world outside the blockchain. It only prevents other contracts from reading or modifying the information.
Transient Storage
Transient storage is a temporary storage area in a computer's memory that stores data that's being processed or used by a program. This storage is volatile, meaning its contents are lost when the program ends or the computer is turned off.
The size of transient storage varies depending on the computer's architecture and operating system, but it's typically much smaller than primary storage. For example, in the article's discussion of "Memory Hierarchy", it was mentioned that the size of the cache memory, a type of transient storage, can range from a few kilobytes to several megabytes.
You might enjoy: Blockchain Database Storage
Transient storage is used to store data that's frequently accessed and modified, such as the values of variables. In the article's section on "Variables", it was explained that variables are used to store and manipulate data in a program, and transient storage is where their values are stored temporarily.
Transients storage is often faster and more efficient than primary storage, which makes it ideal for storing data that's being actively used by a program. This is why it's commonly used to store the values of variables, which are constantly being updated and accessed.
The use of transient storage can improve a program's performance and efficiency, but it also means that the data stored there is lost when the program ends or the computer is turned off.
Immutable State Variables
Immutable state variables are a powerful tool in smart contract development. They can be assigned a value at construction time and remain permanent, but with some restrictions.
Immutable variables can only be assigned to inside expressions for which there is no possibility of being executed after creation. This excludes all modifier definitions and functions other than constructors.
Immutables can be read before they're written to for the first time because variables in Solidity always have a well-defined initial value. You can even never explicitly assign a value to an immutable, and it will still work.
The initialization order of immutables at construction time is important to keep in mind. Even if you provide an explicit initializer, some expressions may end up being evaluated before that initializer, especially when they're at a different level in the inheritance hierarchy.
Prior to Solidity 0.8.21, initialization of immutable variables was more restrictive. Such variables had to be initialized exactly once at construction time and could not be read before then.
Here are some key differences between immutable and constant variables:
Immutables have a significant impact on the gas costs of your contract. They can be evaluated once at construction time and their value is copied to all the places in the code where they're accessed. This means that immutables can sometimes be cheaper than constants.
Return Variables
Return Variables are declared with the same syntax after the returns keyword.
You can omit the names of return variables, and they can be used as any other local variable. They are initialized with their default value until they are assigned.
Return variables can be explicitly assigned and then left alone, or you can provide return values directly with the return statement.
If you use an early return, you must provide return values together with the return statement.
Return variables can be used to return multiple values from a function, where the number of components must match the number of return variables, and their types must match.
Here are some examples of types that cannot be returned from non-internal functions:
- mappings,
- internal function types,
- reference types with location set to storage,
- multi-dimensional arrays (applies only to ABI coder v1),
- structs (applies only to ABI coder v1).
Agreements
In Ethereum, agreements are actually implemented through smart contracts, which are self-executing contracts with the terms of the agreement written directly into code.
Smart contracts are controlled by program code that is executed by the Ethereum Virtual Machine, and they have associated code and data storage.
Contract accounts, which are controlled by smart contracts, do not have private keys and instead "control themselves" in the predetermined way prescribed by their smart contract code.
Both externally owned accounts (EOAs) and contract accounts are identified by an Ethereum address.
EOAs, on the other hand, are controlled by users through transactions created and cryptographically signed with a private key in the "real world" external to and independent of the protocol.
Functions and Parameters
Functions in Solidity take typed parameters as input and can return an arbitrary number of values as output.
Function parameters are declared the same way as variables, and the name of unused parameters can be omitted.
You can assign function parameters to other variables, making them usable as any other local variable.
The ABI (Application Binary Interface) is used to interact with the contract, and it's an essential part of the contract deployment process.
Here's a brief overview of how to declare function parameters:
Note that the name of unused parameters can be omitted, as shown in the example.
Events and Errors
Events are a crucial part of Solidity, allowing you to notify other contracts or external entities about specific actions or changes in your contract's state. They are stored in the blockchain and can be accessed through the RPC interface of an Ethereum client.
You can define events at the file level or as inheritable members of contracts, and they can be indexed or non-indexed. Indexed events allow for faster searching and filtering, but they can only hold a single 32-byte value.
Events are especially useful for light clients and DApp services, which can "watch" for specific events and report them to the user interface, or make a change in the state of the application to reflect an event in an underlying contract.
Here's a quick rundown of the key differences between indexed and non-indexed events:
As for errors, Solidity provides four functions for handling them: assert, require, revert, and throw (now deprecated). The assert and require functions operate in the same way, evaluating a condition and stopping execution with an error if the condition is false.
Modifier
Modifiers are a crucial aspect of smart contracts, ensuring the coherence of conditions before executing code. They make sure conditions are properly defined to avoid inaccurate calculations and execution.
In Solidity, modifiers can be used to change the behavior of functions in a declarative way. They can be applied to functions to check conditions prior to execution.
Modifiers are inheritable properties of contracts and may be overridden by derived contracts, but only if they are marked virtual. They can also be defined in libraries, but their use is limited to functions of the same library.
Multiple modifiers can be applied to a function by specifying them in a whitespace-separated list and are evaluated in the order presented. They cannot implicitly access or change the arguments and return values of functions they modify.
A modifier's values can only be passed to them explicitly at the point of invocation. To specify when a function should be run, the placeholder statement (denoted by a single underscore character _) is used.
Explicit returns from a modifier or function body only leave the current modifier or function body. Return variables are assigned and control flow continues after the _ in the preceding modifier.
Function modifiers can override each other, working in the same way as function overriding. The virtual keyword must be used on the overridden modifier, and the override keyword must be used in the overriding modifier.
Events
Events are a powerful tool in the world of smart contracts. They allow you to store information about actions that occurred during the execution of a transaction, making it easier to debug and understand what's happening.
These log entries are stored in the blockchain and can be accessed through the RPC interface of an Ethereum client. You can even request a Merkle proof for logs, which can be used to verify the existence of a log within the blockchain.
Events can be defined at the file level or as inheritable members of contracts. They can take arguments, which are serialized and recorded in the transaction logs. You can also specify which arguments should be indexed, making it easier to search and filter for specific events.
There are a few key things to keep in mind when working with events. The hash of the signature of the event is one of the topics, unless you declared the event as anonymous. Anonymous events are cheaper to deploy and call, but they can also be used to "fake" the signature of another event.
Here are some key members of events:
- event.selector: This is a bytes32 value containing the keccak256 hash of the event signature, as used in the default topic.
By understanding how events work, you can create more robust and debuggable smart contracts. Events are especially useful for light clients and DApp services, which can "watch" for specific events and report them to the user interface.
Custom Errors
Custom errors are a crucial aspect of smart contract development, allowing you to provide specific feedback to users when something goes wrong. They can be used to return a custom error message, which is recorded in the transaction log.
In Solidity, the `revert` function can be used to explicitly revert the transaction and return a custom error message. This is useful in complex conditions where multiple checks are needed. For example, `revert("Error message");` can be used to return a custom error message.
The `require` function can also be used to return a custom error message, which is recorded in the transaction log. As of Solidity v0.4.22, `require` can include a helpful text message that can be used to show the reason for the error. For example, `require(condition, "Error message");` can be used to return a custom error message.
Here are some best practices for using custom errors:
- Use `revert` statements for explicit error handling.
- Use `require` statements for user input validation.
- Always provide a custom error message to help users understand what went wrong.
By following these best practices, you can create custom errors that provide valuable feedback to users and help prevent unintended consequences.
Inheritance and Polymorphism
Inheritance and Polymorphism is a powerful feature in Solidity that allows contracts to inherit functionality from other contracts. This means that a contract can use the code and variables of another contract, making it easier to create complex and modular smart contracts.
In Solidity, polymorphism is achieved through the use of virtual and override keywords. A virtual function is one that can be overridden by a derived contract, and the override keyword is used to indicate that a function is overriding a virtual function from a base contract.
To enable polymorphism, a function in a base contract must be marked as virtual, and the overriding function in the derived contract must use the override keyword. For example, in the example of the Final contract, the emitEvent function is marked as virtual and overridden by the Base2 contract, which in turn overrides the Base1 contract.
Functions with the private visibility cannot be virtual, and functions without implementation must be marked virtual outside of interfaces, where all functions are automatically considered virtual. Starting from Solidity 0.8.8, the override keyword is not required when overriding an interface function, except for the case where the function is defined in multiple bases.
Here are the possible mutability changes when overriding a function:
- nonpayable can be overridden by view and pure.
- view can be overridden by pure.
- payable is an exception and cannot be changed to any other mutability.
Inheritance
Inheritance is a powerful feature in Solidity that allows contracts to inherit functionality from other contracts. This is done using the `is` keyword, which specifies the parent contract.
Solidity supports multiple inheritance, which can be specified by comma-separated contract names after the `is` keyword. This allows a contract to inherit from multiple contracts at once.
A call to a function in a base contract can be made by explicitly specifying the contract using `ContractName.functionName()`, or by using `super.functionName()` to call the function one level higher up in the inheritance hierarchy.
State variable shadowing is considered an error in Solidity, and a derived contract can only declare a state variable `x` if there is no visible state variable with the same name in any of its bases.
The general inheritance system in Solidity is similar to Python's, but with some differences. For example, the order of base contracts in the `is` directive is important, and must be listed from "most base-like" to "most derived".
Solidity uses "C3 Linearization" to force a specific order in the directed acyclic graph (DAG) of base classes. This results in the desirable property of monotonicity, but disallows some inheritance graphs.
Here are the possible situations where a contract may contain multiple definitions sharing the same name due to inheritance:
- Overloading of functions.
- Overriding of virtual functions.
- Overriding of external virtual functions by state variable getters.
- Overriding of virtual modifiers.
- Overloading of events.
Contract inheritance allows us to write contracts in a modular way, with each contract focused on its specific functionality. This makes our code cleaner, easier to read, and easier to audit.
Overriding
Overriding is a powerful tool in Solidity that allows you to change the behavior of functions in derived contracts. You can override functions in base contracts to change their behavior.
To override a function, it must be marked as virtual in the base contract. The overriding function must then use the override keyword in the function header. You can change the visibility of the overridden function from external to public, but not the other way around.
The mutability of the function can also be changed, but with some restrictions. Nonpayable can be overridden by view and pure, while view can be overridden by pure. Payable, however, cannot be changed to any other mutability.
Here are the rules for overriding functions in multiple inheritance:
In the case of multiple inheritance, all direct base contracts must be specified explicitly. An explicit override specifier is not required if the function is defined in a common base contract or if there is a unique function in a common base contract that already overrides all other functions.
Functions without implementation must be marked virtual outside of interfaces. In interfaces, all functions are automatically considered virtual.
Modifier Overriding
Modifier overriding is a powerful feature in Solidity that allows you to change the behavior of modifiers in derived contracts.
To override a modifier, you must use the virtual keyword on the overridden modifier and the override keyword in the overriding modifier.
This means you can create a base contract with a modifier and then create a derived contract that overrides that modifier with its own behavior.
Modifiers are inheritable properties of contracts and may be overridden by derived contracts, but only if they are marked virtual.
This allows you to create a hierarchy of contracts where each contract can build upon and modify the behavior of the previous one.
By using modifier overriding, you can create a more flexible and reusable codebase that can adapt to different use cases.
Advanced Topics
Modifiers are a powerful tool in Solidity that can enhance the functionality and security of smart contracts. They allow you to reuse code and make your contracts more maintainable.
A modifier is essentially a function that can be applied to another function to modify its behavior. For example, the `add` function in the example code can be modified to check for overflow before performing the addition.
Modifiers can also be used to check for certain conditions before allowing a function to execute. This can help prevent errors and make your contracts more secure.
Here are some key areas to explore when it comes to modifiers:
- Using modifiers to check for overflow and underflow
- Applying modifiers to functions to modify their behavior
- Creating custom modifiers to reuse code and make contracts more maintainable
Composability
Composability is a crucial design principle for smart contracts, allowing multiple calls into individual contracts to be composed into more complex applications. This principle ensures self-contained behavior.
The EVM has largely guaranteed composable behavior, making multiple calls within a complex transaction virtually indistinguishable from separate calls over several transactions. However, transient storage can violate this principle.
Transient storage can break composability by resetting values when calls are not executed in the same transaction. For example, if a multiplier is set to 42, subsequent calls would return 0 if the calls are not in the same transaction.
Using transient storage as a replacement for in-memory mappings without clearing the mapping can lead to unexpected behavior in complex transactions. This is because values set by a previous call within the same transaction remain.
Clearing transient storage completely at the end of a call is recommended to avoid these kinds of issues and simplify the analysis of the contract's behavior.
Gas Optimization Techniques
Gas optimization is essential for reducing transaction costs and improving the efficiency of smart contracts on the Ethereum network. Here are some techniques to optimize gas usage.
Minimizing storage operations is crucial, as they are costly in terms of gas. To optimize, you should use efficient data types for your variables.
Avoiding redundant computations is also vital. Cache results of expensive computations to avoid recalculating them, which can save a significant amount of gas.
Batching operations is another technique that can save on gas fees. If possible, batch multiple operations into a single transaction to reduce the number of gas units needed.
Short-circuiting in logical operations can also prevent unnecessary computations and save gas. This technique is useful when dealing with complex logical operations.
Using libraries that are optimized for gas usage can also help. Leverage existing libraries that have already been optimized for gas usage to save time and resources.
Here are the gas optimization techniques summarized in a list:
- Minimize Storage Operations
- Use Efficient Data Types
- Avoid Redundant Computations
- Batch Operations
- Short-Circuiting
- Use Libraries
Security and Best Practices
To ensure the security and reliability of your smart contracts, it's essential to follow best practices.
Developers should use established libraries for common functionalities to reduce the risk of introducing vulnerabilities through custom code. This can be achieved by leveraging well-audited libraries, such as those for safe math operations.
Conducting thorough testing is also crucial, including unit tests and integration tests to ensure that all functionalities work as intended. This can be facilitated using testing frameworks.
To further enhance security, implement access control using modifiers to restrict access to sensitive functions. Limit external calls in your contracts and use checks-effects-interactions patterns to prevent reentrancy attacks.
Here are some key best practices to keep in mind:
- Use established libraries
- Conduct thorough testing
- Implement access control
- Limit external calls
- Use safe math libraries
Secure Best Practices
Adhering to best practices for smart contract security is crucial to mitigate risks and protect both assets and users.
Developers should leverage well-audited libraries for common functionalities to reduce the risk of introducing vulnerabilities through custom code.
Conducting thorough testing, including unit tests and integration tests, ensures that all functionalities work as intended.
Implementing security audits, such as a smart contract audit or a solidity audit, can help identify vulnerabilities that may have been overlooked.
Access control is key: use modifiers to restrict access to sensitive functions and ensure that only authorized users can execute critical operations.
Limiting external calls in your contracts minimizes the risk of reentrancy attacks and other potential exploits.
Safe math libraries can prevent overflow and underflow issues by handling arithmetic operations safely.
Avoid relying on block timestamps, as they can be manipulated by miners, and instead use block numbers or other mechanisms to ensure contract logic is secure.
Expand your knowledge: Security Contracts
Monitoring and upgrading contracts after deployment is essential to patch vulnerabilities as they are discovered.
Here are the best practices for secure smart contracts in a concise list:
- Use established libraries for common functionalities
- Conduct thorough testing
- Perform security audits
- Implement access control
- Limit external calls
- Use safe math libraries
- Avoid using block timestamps
- Monitor and upgrade contracts
- Educate users
Integration Best Practices
Integration best practices are crucial for ensuring the security and reliability of your smart contracts. Simulate real user scenarios by writing tests that mimic how users will interact with the application, including smart contract penetration testing.
To achieve this, you can use mock contracts to simulate external dependencies, if necessary. This approach allows you to isolate each test and avoid side effects from other tests.
Here are some key takeaways to keep in mind:
- Simulate real user scenarios, including smart contract penetration testing.
- Use mock contracts to simulate external dependencies, if necessary.
- Keep tests isolated to avoid side effects from other tests.
Integration testing is a vital step in the development process that catches interaction issues and validates overall functionality. It ensures that the entire system behaves as intended when all components are integrated.
By following these best practices, you can ensure that your smart contracts are robust, secure, and ready for deployment.
Deployment and Testing
Deploying a smart contract on the Ethereum blockchain involves several steps, including running a migration using Truffle or Hardhat, and checking the transaction on Etherscan or a local blockchain interface.
To deploy a contract, you can use the command `truffle migrate` or `hardhat deploy contract`, depending on your development environment. This makes your contract live and accessible to users.
You should also ensure that the contract address is recorded for future interactions, and verify the contract on Etherscan or similar services to make its source code publicly available and increase trust.
For unit testing, Truffle provides built-in support and allows developers to write automated tests in JavaScript or Solidity, making it flexible for developers familiar with either language.
Truffle Deployment
Truffle Deployment is a popular choice among developers for its simplicity and ease of use. It simplifies the process of building, testing, and deploying smart contracts on the Ethereum blockchain.
To set up Truffle, you'll need to create a new project directory and navigate to it in your terminal. You can do this by running the following commands: `mkdir myproject` and then `cd myproject`.
Once you've initialized Truffle, you can deploy your smart contract by running the `truffle migrate` command, specifying the network you want to deploy to.
Here are the steps to deploy a smart contract using Truffle:
- Run the migration to deploy the contract: `truffle migrate`
- Alternatively, if you're using Hardhat, you can use `hardhat deploy contract` to deploy your smart contract.
After deploying your contract, you should verify it on Etherscan or a similar service to make its source code publicly available and increase trust.
Using Truffle streamlines the deployment process, allowing developers to focus on building robust applications, including coding for blockchain and developing smart contracts.
Testnet vs Mainnet
Testnets and mainnets are crucial components in the blockchain ecosystem, serving different purposes in the development and deployment of decentralized applications (dApps).
To ensure that your applications are robust and secure before going live, it's essential to understand the differences between testnets and mainnets.
Testnets are used for configuring networks, which is a critical step in the development process. This allows developers to test and refine their applications in a controlled environment.
Mainnets, on the other hand, are used for writing and compiling smart contracts, which is a key aspect of deploying decentralized applications.
Developers can use testnets to write smart contracts, but it's not the best environment for this task. Mainnets are specifically designed for this purpose.
Here are some key activities that are typically done on testnets and mainnets:
- Configuring Networks
- Writing Smart Contracts
- Compiling Contracts
Tools and Frameworks
As a developer, you'll want to use tools and frameworks that make your life easier. Truffle is a popular development framework for Ethereum that provides built-in support for unit testing.
Truffle is specifically designed to help you write and deploy smart contracts, making it a great choice for working with Solidity.
You can use Truffle to create, test, and deploy your smart contracts with ease, saving you time and reducing the risk of errors.
Development IDEs
Development IDEs can be a game-changer for developers.
If you're looking for a powerful development environment, consider using Microsoft Visual Studio Code, JetBrains IntelliJ, Remix Online Editor, or EthFiddle Online Editor.
These IDEs offer features like syntax highlighting, auto-completion, and more to make your development process smoother.
Remix IDE, in particular, is a great choice for Ethereum smart contract development, as it provides a user-friendly interface for writing, testing, and debugging Solidity code.
Here are some popular development IDEs:
- Microsoft Visual Studio Code
- JetBrains IntelliJ
- Remix Online Editor
- EthFiddle Online Editor
Blockchain Platforms
Solidity is a versatile language that can be used on a variety of blockchain platforms.
You can run Solidity on Avalanche C-Chain, Binance Smart Chain, Counterparty, Ethereum, Ethereum Classic, Tron, Hedera Hashgraph, Polygon, Metis, Arbitrum, and Optimism.
Here are some of the notable platforms where Solidity is available:
- Avalanche C-Chain
- Binance Smart Chain
- Counterparty
- Ethereum
- Ethereum Classic
- Tron
- Hedera Hashgraph
- Polygon
- Metis
- Arbitrum
- Optimism
Solidity is also used in domain-specific programming languages and statically typed programming languages.
Debugging Techniques
Debugging Techniques are a crucial part of the development process, especially in smart contract development. They ensure that the code behaves as expected and is free from vulnerabilities.
To effectively debug your smart contracts, you can use print statements to output variable values at different stages of execution. This helps in understanding the flow of the program and identifying where things go wrong.
Assertions are also a valuable tool in debugging, as they allow you to check for conditions that must be true. If an assertion fails, it will revert the transaction, providing immediate feedback on where the issue lies.
Unit testing is another essential technique, allowing you to test individual components of your code in isolation. This makes it easier to identify bugs and ensure that your contract behaves correctly.
Here are some effective debugging techniques to consider:
- Print Statements: Use console.log or similar functions to output variable values at different stages of execution.
- Assertions: Implement assertions in your code to check for conditions that must be true.
- Unit Testing: Write unit tests for your smart contracts using frameworks like Truffle or Hardhat.
- Event Logging: Emit events at critical points in your contract to track state changes.
- Static Analysis Tools: Utilize tools like MythX or Slither to analyze your code for vulnerabilities and potential bugs before deployment.
- Testnet Deployment: Deploy your contracts on a test network (like Rinkeby or Ropsten) to observe their behavior in a live environment without risking real funds.
By incorporating these techniques into your development process, you can ensure that your smart contracts are thoroughly tested and debugged, reducing the risk of errors and vulnerabilities.
Emerging Features and Languages
Solidity, the primary programming language for Ethereum smart contracts, is constantly evolving. Recent updates have introduced several emerging features that can significantly benefit your projects.
User-defined value types allow developers to create custom value types, which can help optimize gas costs and improve code readability.
For more insights, see: Blockchain Types
Fixed-size arrays provide developers with more control over data structures, allowing for better memory management and enhanced performance.
Here are some notable emerging features in Solidity:
Custom error types allow developers to define specific error messages, improving debugging and providing clearer feedback during contract execution.
Try-catch statements enable developers to handle exceptions more gracefully, leading to improved contract reliability and user trust.
Inline assembly allows developers to use assembly code for critical sections of code, giving more granular control over gas consumption and performance optimization.
The Solidity compiler has been updated to produce more efficient bytecode, reducing gas costs for contract deployment and execution.
Frequently Asked Questions
What is a Solidity smart contract?
A Solidity smart contract is a self-executing program that automates rules and actions on the Ethereum network. It's a digital agreement that runs on the blockchain, ensuring transparent and secure interactions between parties.
What is the difference between smart contract and Solidity?
Smart contracts are self-executing contracts with specific rules, while Solidity is a programming language used to write and deploy these contracts on the Ethereum blockchain. In other words, Solidity is the tool used to create smart contracts, not the contracts themselves.
Is Solidity worth learning in 2024?
Solidity is a valuable skill to learn in 2024, with a gentle learning curve for beginners familiar with JavaScript. Start your journey to mastering Solidity today and unlock new possibilities in the world of blockchain development
Sources
- https://en.wikipedia.org/wiki/Solidity
- https://cryptoexchange.com/learning/what-is-solidity
- https://docs.soliditylang.org/en/latest/contracts.html
- https://www.rapidinnovation.io/post/how-to-create-a-smart-contract-using-solidity
- https://cypherpunks-core.github.io/ethereumbook/07smart-contracts-solidity.html
Featured Images: pexels.com