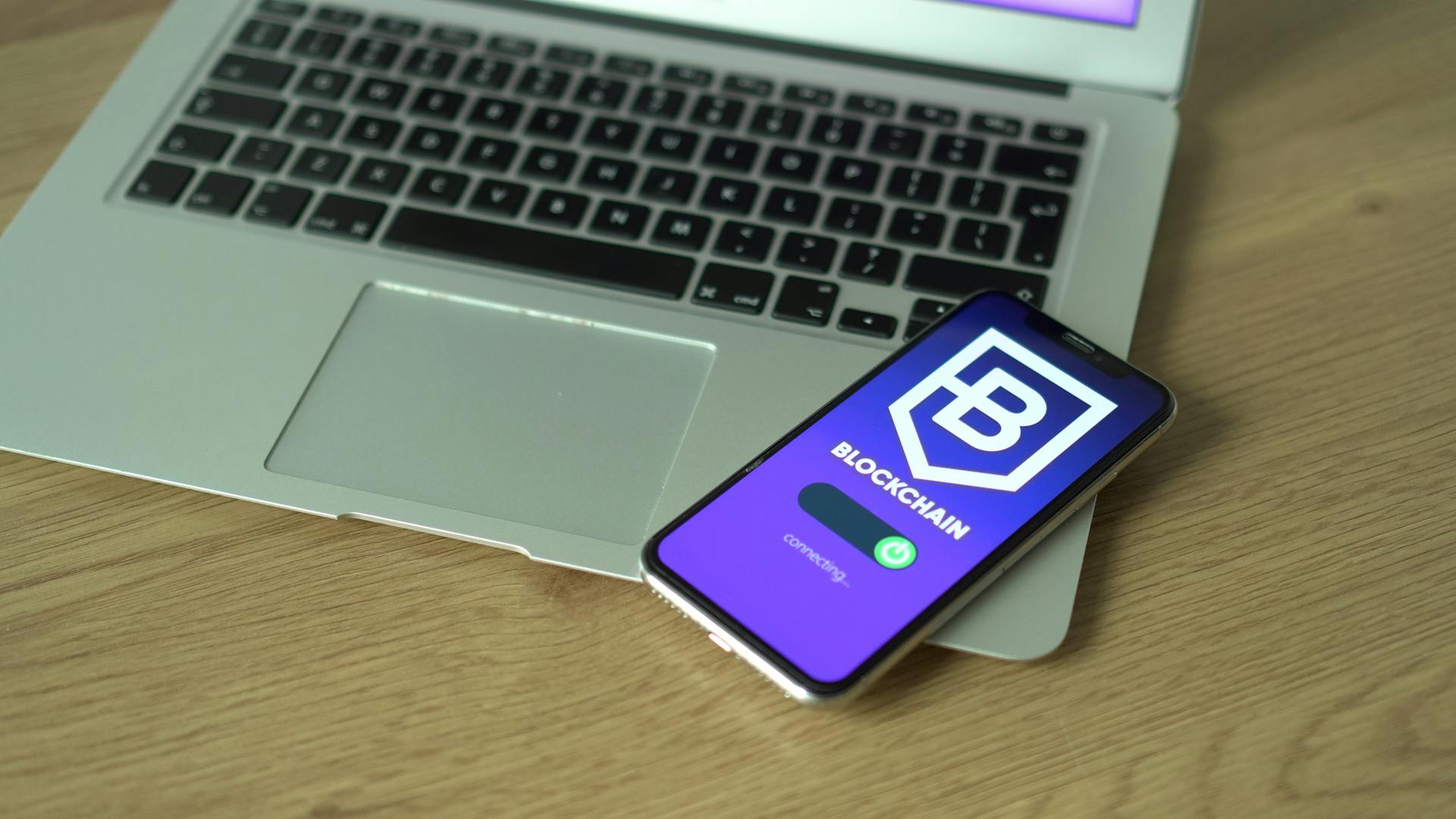
ERC20 ABI is a crucial component of working with smart contracts. It's a JSON file that contains the contract's interface, which is used by wallets and other Ethereum applications to interact with the contract.
The ABI file is generated by the compiler and contains information such as the contract's functions, events, and variables. This information is used to ensure that the contract is correctly deployed and interacted with.
To work with ERC20 ABI and smart contracts, you need to have a basic understanding of Ethereum and Solidity. You also need to have a development environment set up, such as Remix or Truffle.
Related reading: Marcus Smart Contract Grizzlies
ERC20 ABI/JSON Interface
To find the ABI/JSON Interface for an ETH/ERC20 contract, you can get it from Etherscan.
You can access the ABI by visiting etherscan.io/address/... and following the instructions.
The TUSD ABI can be found on Etherscan, as mentioned by Karthikeyan Thangavel in the comments.
IERC20
IERC20 is the interface of the ERC20 standard as defined in the EIP. It does not include the optional functions, and to access them, you should see ERC20Detailed.
The IERC20 interface includes six main functions: totalSupply(), balanceOf(account), transfer(recipient, amount), allowance(owner, spender), approve(spender, amount), and transferFrom(sender, recipient, amount). These functions are crucial for interacting with an ERC20 token contract.
Here are the functions included in the IERC20 interface:
- totalSupply()
- balanceOf(account)
- transfer(recipient, amount)
- allowance(owner, spender)
- approve(spender, amount)
- transferFrom(sender, recipient, amount)
IERC20 also includes two events: Transfer(from, to, value) and Approval(owner, spender, value). These events are used to track changes in the token balance and allowance.
Note that the IERC20 interface is a standard interface, and different implementations may have additional functions or variations. However, the core functions listed above are the minimum requirements for an ERC20 token contract.
Here's an interesting read: Erc20 Token List
Pausable
The Pausable feature is a crucial aspect of the ERC20 ABI/JSON interface. It allows you to temporarily halt all token transfers and allowances, giving you a safety net in case of emergencies or unexpected issues.
You can check if the contract is paused using the `paused()` function, which returns a boolean value indicating its status. This is useful for determining whether trades can proceed or not.
The `pause()` function is used to halt all token transfers and allowances, while the `unpause()` function is used to resume normal operations.
To add or remove pausers, you can use the `addPauser(account)` and `removePauser(account)` functions, respectively. These functions can be used to grant or revoke permission for specific accounts to pause or unpause the contract.
Here's a summary of the key functions related to pausing:
In addition to these functions, the Pausable feature also emits events when the contract is paused or unpaused, and when a pauser is added or removed.
On a similar theme: When Are Ethereum Gas Fees Lowest
Querying Token Metadata
Querying Token Metadata is a crucial aspect of working with ERC20 tokens. Each token has a total supply, which represents the total number of tokens in circulation.
The total supply is a key piece of information, and it's often used to determine the value of a token. In our example, we initialized the token contract to have 1 million tokens.
Intriguing read: Erc20 Token Creation
Token contracts can have varying numbers of decimal places, which affects the total supply. Our token contract has 18 decimal places, which means the raw total supply returned by the contract will have 18 additional decimal places.
This can sometimes lead to confusion, but it's essential to understand the implications of decimal places on token values.
Check this out: Ethereum Supply
ERC20 Functions
ERC20 Functions are a crucial part of any ERC20 token's functionality. They allow users to interact with the token in various ways.
The balanceOf function returns the amount of tokens owned by a specific account. This is a straightforward way to check if a user has the necessary tokens for a transaction.
There are three main functions for transferring tokens: transfer, transferFrom, and _burn. Each has its own set of requirements and outcomes.
Here are some key facts about these functions:
- transfer moves tokens from the caller's account to a recipient's account.
- transferFrom allows a sender to transfer tokens from their account to a recipient's account, using the sender's allowance.
- _burn destroys tokens from a specific account, reducing the total supply.
Each of these functions has its own set of requirements to ensure successful execution. For example, the transfer function requires the recipient to not be the zero address, while the transferFrom function requires the sender to have a balance of at least the amount being transferred.
Total Supply External
In ERC20, the total supply of tokens is a crucial aspect of the protocol. The totalSupply() function returns the amount of tokens in existence.
You can use this function to get the current total supply of tokens in your contract. This can be useful for tracking the overall supply of tokens over time.
The total supply is returned as a uint256, which is a 256-bit unsigned integer. This allows for a very large range of possible values.
BalanceOf Address account External
The balanceOf function is a crucial part of any ERC20 token contract. It allows you to retrieve the amount of tokens owned by a specific account.
The function signature is balanceOf(address account) → uint256 external, which means it takes an address as input and returns the token balance as a uint256 value.
You can use this function to query account balances, such as in the case of a token contract that starts with a single account, which we'll refer to as alice, holding all of the tokens.
If this caught your attention, see: Erc20 Wallet Address
Transfer(address recipient)
The transfer function is a crucial part of ERC20 smart contracts, allowing users to send tokens to each other.
To initiate a transfer, you'll need to specify the recipient's address and the amount of tokens to be sent.
The transfer function returns a boolean value indicating whether the operation was successful.
However, there are some important conditions that must be met for a transfer to occur.
If the recipient is the zero address, the transfer will not be executed.
Similarly, the caller must have a sufficient balance to cover the transfer amount.
Here are the specific conditions for a successful transfer:
- Recipient cannot be the zero address.
- Caller must have a balance of at least the transfer amount.
Allowance External
The ERC20 standard provides a way to manage token allowances using the allowance function, which returns the remaining number of tokens that spender will be allowed to spend on behalf of owner through transferFrom.
This value changes when approve or transferFrom are called, and it's zero by default. The allowance function is public, meaning it can be accessed by anyone.
Here are the parameters and return values of the allowance function:
The allowance function is used in conjunction with other ERC20 functions, such as transferFrom and approve, to manage token allowances.
Burnable
The Burnable function is an extension of the ERC20 standard that allows token holders to destroy their own tokens and those they have an allowance for. This can be done in a way that's recognizable off-chain through event analysis.
To burn tokens, you can use the burn(amount) or burnFrom(account, amount) functions. The burn(amount) function is straightforward, but it's worth noting that burnFrom(account, amount) also allows you to destroy tokens from an account that you have an allowance for.
The burnFrom(account, amount) function deducts the amount from the caller's allowance, making it a useful tool for managing token balances. However, it's worth noting that the account cannot be the zero address.
Here are the key differences between the _burn and burnFrom functions:
- The _burn function reduces the total supply, whereas burnFrom deducts from the caller's allowance.
- The _burn function requires the account to have at least the amount of tokens they're trying to burn.
It's worth noting that the _burn function has some additional requirements, such as ensuring the account cannot be the zero address.
ERC20 Events
ERC20 Events are a crucial aspect of the ERC20 protocol, allowing developers to track changes to a token's state. They are emitted by the contract when specific actions occur.
The Approval event is emitted when the allowance of a spender for an owner is set by a call to approve. This event includes the new allowance value, which can be used to track changes to the token's allowance.
The Approval event is triggered by a call to the approve function, which sets the new allowance for a spender. This event is essential for tracking changes to a token's allowance and ensuring that the token's state is accurately reflected.
Related reading: Batch Approve Transaction Ethereum
Approval Event
The Approval Event is a crucial part of the ERC20 standard, and it's triggered whenever the allowance of a spender for an owner is set by a call to approve.
The event is emitted with three parameters: the address of the owner, the address of the spender, and the new allowance value.
This event is a way for smart contracts to notify other contracts or external observers that the allowance has been updated. The new allowance value is also included in the event, providing a clear record of the change.
A different take: Ethereum Wallet Address
Transfer Event
The Transfer Event is a crucial part of the ERC20 standard. It's triggered whenever a user initiates a transfer of tokens from one account to another.
The Transfer Event is emitted by the contract, allowing developers to keep track of all token movements. This is essential for auditing and debugging purposes.
The Transfer Event includes the sender and recipient addresses, as well as the amount of tokens transferred. This information is stored on the blockchain, making it tamper-proof and transparent.
The Transfer Event is typically used in conjunction with the Approve Event to ensure that the sender has the necessary permissions to transfer the tokens. This is an important security measure to prevent unauthorized transactions.
In the context of the ERC20 standard, the Transfer Event is a fundamental building block for creating decentralized applications.
You might enjoy: E Interac Transfer Limit
ERC20 Properties
ERC20 tokens have a standard set of rules that define their behavior, including the total supply of tokens, the number of decimal places, and the token's symbol.
See what others are reading: Best Erc20 Tokens
The total supply of an ERC20 token is fixed and cannot be changed after deployment. This is determined by the contract's code and is a key property of ERC20 tokens.
ERC20 tokens also have a specific way of handling transfers, which involves a function called `transfer` that allows users to send tokens to another address. This function is a crucial part of the ERC20 standard.
Decimals Uint8
Decimals in ERC20 tokens are represented as uint8, a data type that can store numbers up to 255.
Tokens usually opt for a value of 18, imitating the relationship between Ether and Wei. This is a common choice, but not the only one.
The decimals() function returns the number of decimals used to get its user representation. This means you can use it to display token balances in a user-friendly format.
For example, if decimals equals 2, a balance of 505 tokens should be displayed to a user as 5,05 (505 / 10 ** 2).
Related reading: Ethereum Layer 2
Capped
The Capped property of ERC20 tokens is a crucial feature that adds a cap to the supply of tokens. This means that once the cap is reached, no more tokens can be minted.
The Capped property is an extension of the ERC20Mintable contract, which allows for the creation of new tokens. The constructor function for a Capped ERC20 token takes a single parameter, cap, which sets the maximum supply of tokens.
The Capped property also includes the cap() function, which returns the current cap.
Here are the functions related to the Capped property:
- constructor(cap)
- cap()
- _mint(account, value)
These functions work together to ensure that the supply of tokens does not exceed the set cap.
ERC20 Contract
You can find the ABI for an ERC20 contract on Etherscan, a block explorer. Etherscan is a great resource for getting the ABI and deployed address of a contract.
To interact with an existing ERC20 token contract, you'll need its deployed address and ABI. Both can be found using Etherscan or similar block explorers.
Consider reading: Usdc Address Ethereum
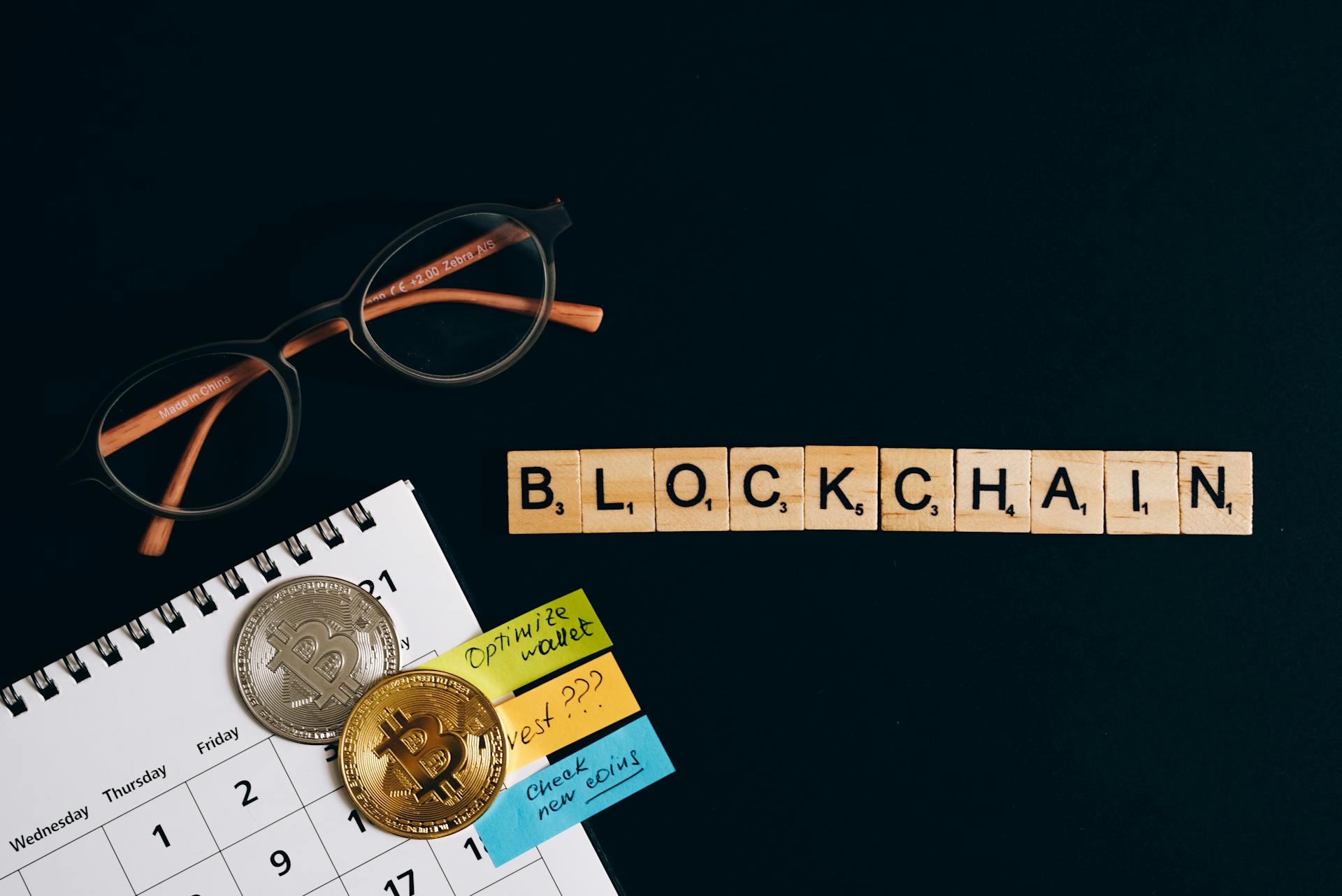
Here are the requirements for interacting with an existing ERC20 token contract:
- An existing token contract at a known address.
- Access to the proper ABI for the given contract.
- A web3.main.Web3 instance connected to a provider with an unlocked account which can send transactions.
The ERC20Contract inherits from Contract, and creating a new instance of a Contract connects to an existing contract by specifying its address on the blockchain, its abi, and a providerOrSigner. If a Provider is given, the contract has only read-only access, while a Signer offers access to state manipulating methods.
ERC20 Operations
ERC20 operations are relatively straightforward, and they're essential for managing your tokens. You can use the `balanceOf` function to query account balances, as seen in Example 3.
To transfer tokens, you'll need to use the `transfer` function, which requires the recipient's address and the amount of tokens to transfer. This is a common operation in ERC20 smart contracts.
The `allowance` function allows you to check the remaining allowance for a spender, while the `approve` function enables a spender to spend a certain amount of tokens on your behalf. These functions are useful for managing token permissions.
Here is a list of ERC20 operations:
- totalSupply()
- balanceOf(account)
- transfer(recipient, amount)
- allowance(owner, spender)
- approve(spender, amount)
- transferFrom(sender, recipient, amount)
- increaseAllowance(spender, addedValue)
- decreaseAllowance(spender, subtractedValue)
These operations are the foundation of ERC20 smart contracts, and understanding them is crucial for working with tokens on the Ethereum blockchain.
Mintable
The Mintable extension of ERC20 allows a set of accounts with the MinterRole to mint new tokens.
The deployer of the contract is automatically the only minter at construction.
Only the minter has permission to create new tokens.
Here are the key functions related to minting:
- onlyMinter(): checks if the deployer is the only minter
- constructor(): initializes the contract with the deployer as the only minter
- isMinter(account): checks if an account has the MinterRole
- addMinter(account): adds a new minter
- renounceMinter(): removes the deployer's minter role
- _addMinter(account): adds a new minter internally
- _removeMinter(account): removes a minter internally
Token Timelock
Token Timelock is a useful tool for managing token distributions, especially for advisors or team members who need to vest their tokens over time. It's like setting a timer for when the tokens will be released.
A Token Timelock contract can be created with a constructor that takes three parameters: the token, the beneficiary, and the release time. The beneficiary will receive the tokens after the specified release time.
The contract has several methods, including token(), beneficiary(), and releaseTime(), which return the corresponding values. The release() method is used to trigger the token release.
Here are the key methods of a Token Timelock contract:
- constructor(token, beneficiary, releaseTime)
- token()
- beneficiary()
- releaseTime()
- release()
This makes it easy to set up simple vesting schedules, like giving advisors all their tokens after a year.
Query Account Balances
The token contract we are using starts with a single account which we’ll refer to as alice holding all of the tokens.
To query account balances, you can use the contract’s balanceOf function. This function allows you to retrieve the balance of a specific account.
You can use the balanceOf function to check the balance of any account, not just the one that holds all the tokens.
Sending Tokens
Sending tokens is a fundamental operation in ERC20, and it's surprisingly straightforward. You can transfer tokens from one account to another using the contract's transfer function, which is available through the IERC20 interface.
To transfer tokens, you'll need to call the transfer function, passing in the recipient's address and the amount of tokens you want to send. This is exactly how we transfer some tokens from alice to bob in the guide, using the contract's transfer function.
The transfer function will revert if the sender's account balance is less than the amount being transferred, but this behavior is conventional and doesn't conflict with ERC20 applications. If the transfer is successful, the recipient's account balance will be increased by the transferred amount.
Here are the steps to transfer tokens:
- Call the transfer function, passing in the recipient's address and the amount of tokens to transfer.
- Verify that the sender's account balance is sufficient to cover the transfer.
- Check that the transfer is successful and the recipient's account balance has been updated.
ERC20 Security
ERC20 Security is a top priority for any cryptocurrency developer.
A common vulnerability in ERC20 tokens is the reentrancy attack, which can occur when a contract calls another contract that then calls the first contract again, causing an infinite loop.
To prevent this, developers can use the Checks-Effects-Interactions (CEI) pattern, which involves checking the balance of the contract before transferring funds.
The Solidity compiler also provides a built-in function to prevent reentrancy attacks, which can be used to replace the standard transfer function in ERC20 contracts.
Reentrancy attacks can result in significant financial losses, as seen in the Parity wallet hack in 2017, where $30 million was stolen.
Developers can also use libraries like OpenZeppelin to ensure their contracts are secure and up-to-date with the latest security best practices.
Regular audits and testing can also help identify potential vulnerabilities before they are exploited.
A different take: Ethereum Sec Security Status
Working with ERC20
You can interact with an existing token contract on the Ethereum blockchain if it conforms to the ERC-20 standard. Most fungible tokens on the Ethereum blockchain conform to this standard.
If this caught your attention, see: What Is a Smart Contract Blockchain
To interact with an existing token contract, you'll need three things: an existing token contract at a known address, access to the proper ABI for the given contract, and a web3.main.Web3 instance connected to a provider with an unlocked account which can send transactions.
You can find the ABI/JSON Interface for an ETH/ERC20 contract on block explorers like Etherscan. For example, you can get the TUSD ABI from Etherscan.
To use an existing contract, you'll need its deployed address and its ABI. Both can be found using block explorers like Etherscan.
Here are the steps to create a contract instance:
1. Create a contract instance with the address of your token contract and the ERC-20 ABI.
2. Connect to an existing contract by specifying its address on the blockchain, its abi, and a providerOrSigner.
3. If you give a Provider, the contract has only read-only access, while a Signer offers access to state manipulating methods.
Here are the requirements for interacting with an existing token contract:
- An existing token contract at a known address.
- Access to the proper ABI for the given contract.
- A web3.main.Web3 instance connected to a provider with an unlocked account which can send transactions.
Contract Creation
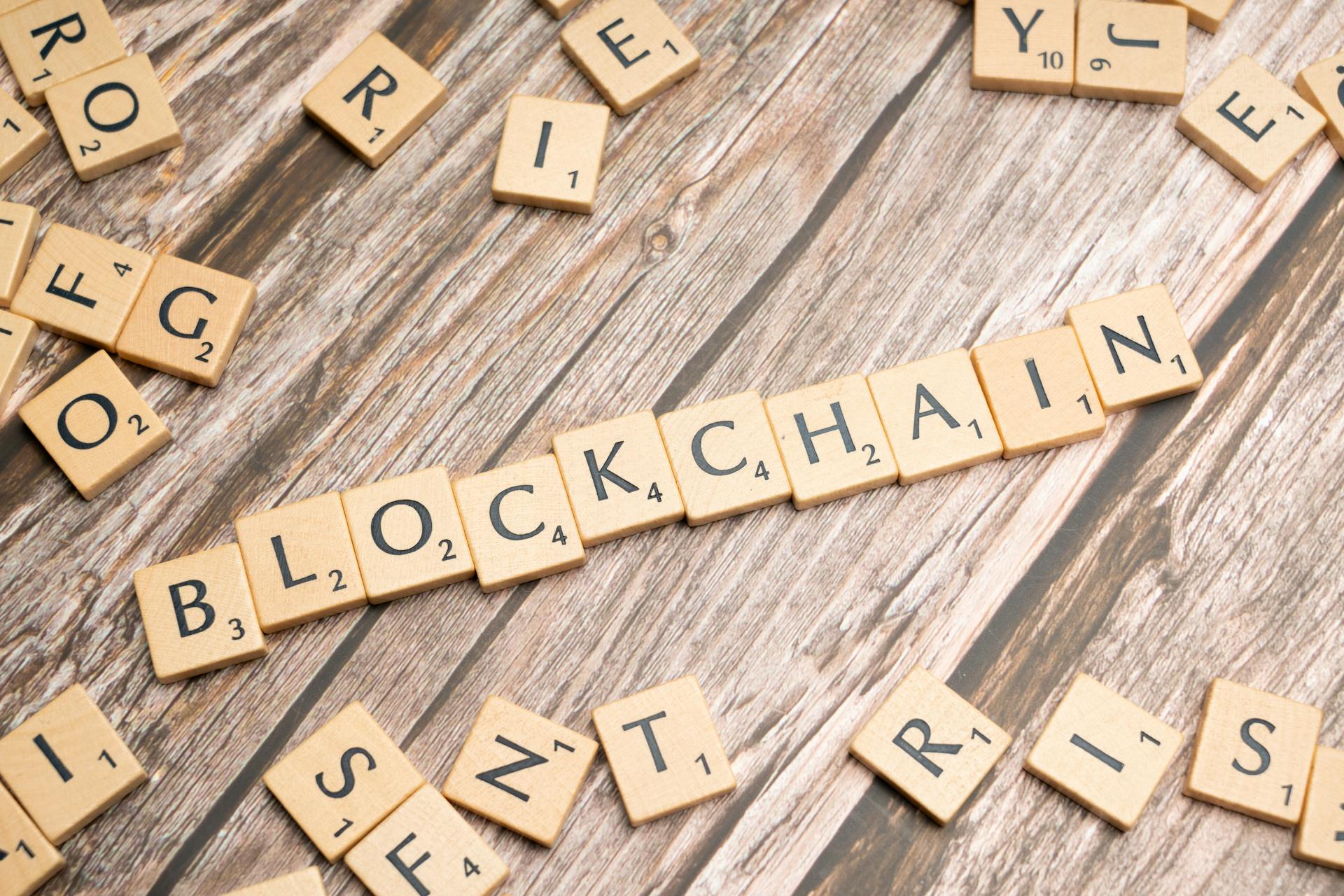
To create a contract, you need to create a contract factory by first creating a contract instance with the address of your token contract. This is where the magic happens.
The contract instance requires the address of your token contract, which is the foundation of your ERC-20 token. The ERC-20 ABI is also necessary for this step.
You'll need to get the address of your token contract, which will serve as the base for your contract factory. This address is crucial for creating the contract instance.
The ERC-20 ABI is a standard interface for interacting with ERC-20 tokens, and it's essential for creating a contract instance. It provides a blueprint for your token's functionality.
With the address of your token contract and the ERC-20 ABI in hand, you're ready to create the contract factory. This is a critical step in setting up your contract.
See what others are reading: How to Get Ethereum Wallet
Contract Connection
Connecting to a contract is a crucial step in working with an ERC-20 token contract. You'll need the contract's address and ABI, which can be found using block explorers like Etherscan.
To connect to a contract, you'll need to know its address, which is the address it was constructed with. You can find this address using a block explorer.
A Contract instance can be created by specifying the contract's address, ABI, and a provider or signer. If a Provider is given, the contract has only read-only access, while a Signer offers access to state manipulating methods.
You can use a web3.main.Web3 instance connected to a provider with an unlocked account to create a Contract instance. This instance will have access to the contract's methods and can send transactions.
Here's a summary of the requirements to connect to a contract:
Inherits Contract
ERC20Contract inherits Contract, which is the foundation for creating a new instance of a Contract.
ERC20Contract is built on top of Contract, allowing it to connect to an existing contract on the blockchain.
Creating a new instance of a Contract requires specifying its address on the blockchain, its abi, and a providerOrSigner.
If a Provider is given, the contract has only read-only access, limiting its capabilities.
A Signer, on the other hand, offers access to state manipulating methods, giving the contract more flexibility.
A unique perspective: New Ethereum Dapps
Sources
- https://ethereum.stackexchange.com/questions/83817/how-do-i-find-the-abi-json-interface-for-an-eth-erc20-contract
- https://docs.openzeppelin.com/contracts/2.x/api/token/ERC20
- https://web3py.readthedocs.io/en/stable/web3.contract.html
- https://medium.com/@0xCodeCharmer/introduction-to-tokens-and-erc20-633fddfb2b94
- https://docs.ethers.org/v5/api/contract/example/
Featured Images: pexels.com