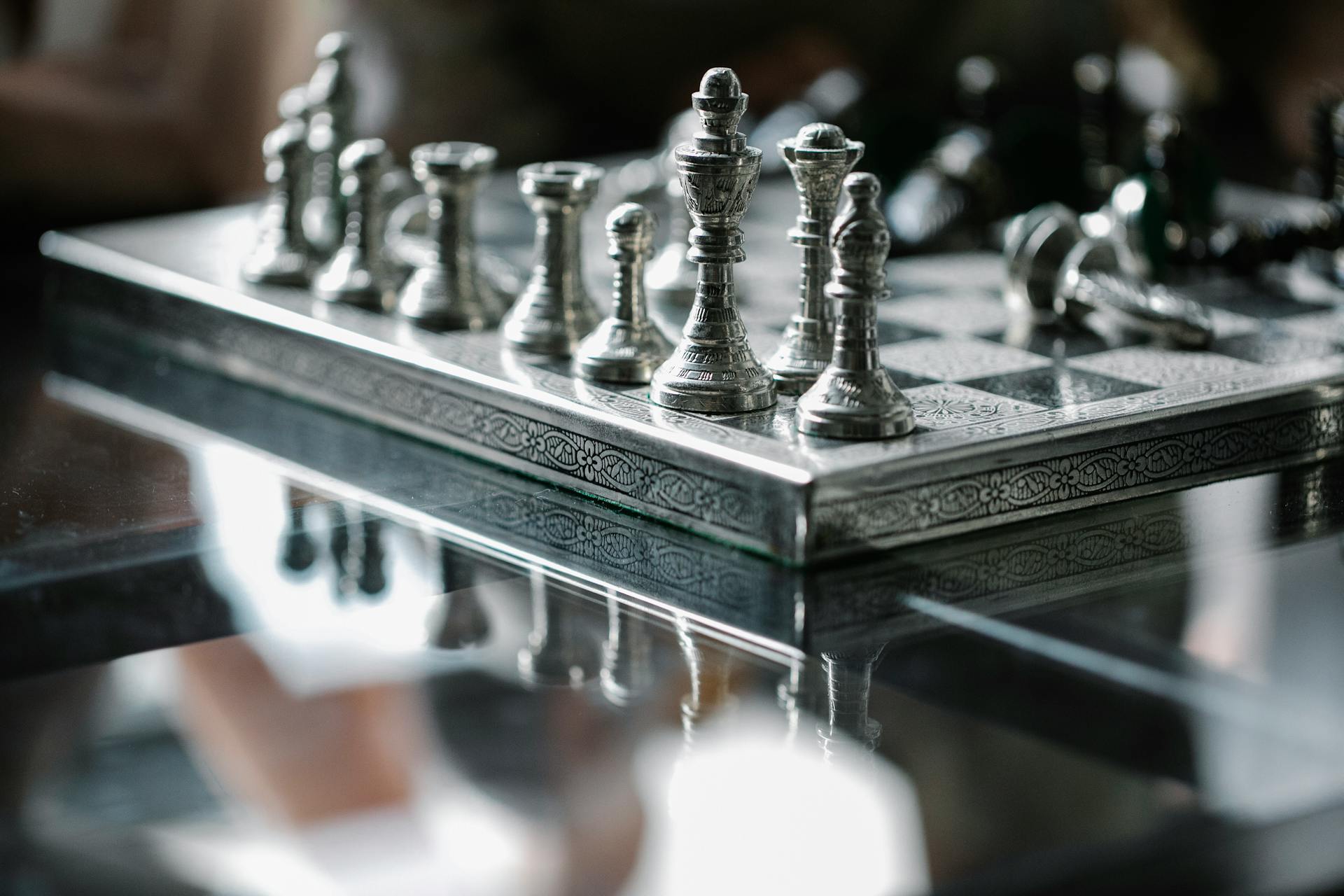
Convar Management and Control in Game Development is a crucial aspect of creating engaging and realistic game experiences. Convars are a type of variable used in game development, particularly in Source games.
Convars are used to store and manage game settings, allowing developers to easily modify and control game behavior. This is achieved through a unique syntax and naming convention.
A well-managed convar system enables developers to create complex game logic and dynamic gameplay mechanics. By controlling convars, developers can fine-tune game settings to achieve the desired outcome.
Convars can be used to implement various game features, such as customizable game modes, adjustable difficulty levels, and dynamic sound effects.
Setting and Managing Convars
Setting cvars is possible, even if they're closed-source.
You can set the value of an integer convar from a boolean using the SetBool method of the ConVar function. This method sets true to 1 and false to 0.
Setting cvars can be done regardless of their source code status.
The SetBool method is a convenient way to set integer convars from boolean values.
Convar Types and Flags
Convar types can be determined using the Type function, which returns the type of the convar.
ConVars can also have flags set, which specify powerful characteristics and must be handled with care. These flags are usually set in the constructor but may be modified with ConCommandBase::AddFlags().
The console system sets additional flags automatically depending on which module the command is defined in. These flags include FCVAR_LAUNCHER, FCVAR_GAMEDLL, FCVAR_CLIENTDLL, FCVAR_MATERIAL_SYSTEM, and FCVAR_STUDIORENDER.
These flags are mainly for internal engine use and shouldn't be set manually.
Here are the automatically set flags by the console system:
Convar Interface and Commands
The Convar Interface is a powerful tool that allows developers to register new commands and variables, making it easy to add new functionality to the game. This interface is available via the global CVAR in-game server/client code (cv in engine code).
The Convar Interface is accessible for all other modules via a public interface ICvar, which is defined in \public\icvar.h. This interface allows registering new commands and finding/iterating existing commands.
Developers can register new commands and variables by creating instances of the ConCommand and console_variables classes, which derive from the base class ConCommandBase. The constructor of these classes automatically registers the command at the console system.
The Convar Interface also provides a function called RegDefaultConVars, which registers an assortment of useful convars. This function is added in version inv0.6.0.
To get the value of a convar, you can simply type the convar name into the console, and the value will be printed to the console. If the convar has not been setup yet, typing the convar name into the console will return an error message.
The Convar Interface also provides a function called Interface, which returns the value of the convar as an interface. This function will never return an error.
Convar Flags and Implementation
Convar flags are powerful characteristics that must be handled with care. They're usually set in the constructor but can be modified with ConCommandBase::AddFlags().
Some flags are set automatically by the console system, depending on which module the command is defined in. These include FCVAR_LAUNCHER, FCVAR_GAMEDLL, FCVAR_CLIENTDLL, FCVAR_MATERIAL_SYSTEM, and FCVAR_STUDIORENDER.
These flags are mainly for internal engine use and shouldn't be set manually. They're not intended for user modification.
The Fcvar Flags
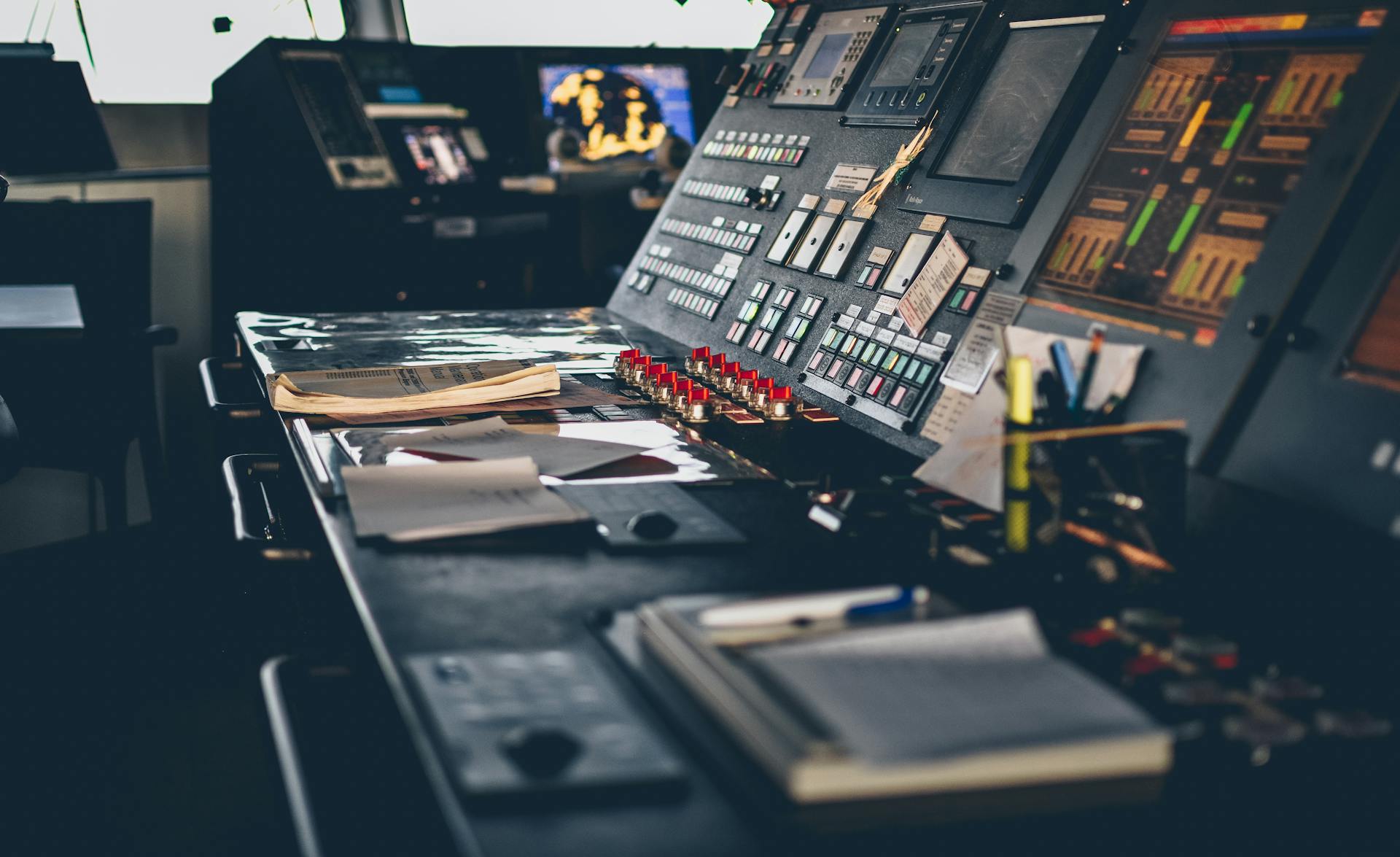
The Fcvar Flags are crucial to understand when working with console commands and variables. They can specify powerful characteristics, so it's essential to handle them with care.
Fcvar Flags are usually set in the constructor but can also be modified with ConCommandBase::AddFlags(). This function is not used very often, though.
Users typically don't have the ability to modify these flags, so it's best to leave them as they are.
The console system automatically sets certain Fcvar Flags depending on the module the command is defined in. These flags are mainly for internal engine use and shouldn't be set manually.
The following Fcvar Flags are set automatically by the console system:
- FCVAR_LAUNCHER
- FCVAR_GAMEDLL
- FCVAR_CLIENTDLL
- FCVAR_MATERIAL_SYSTEM
- FCVAR_STUDIORENDER
RegDefault Added Inv 0.6.0
With the introduction of RegDefaultConVars in Inv 0.6.0, developers can now easily access a set of useful convars.
This feature allows for streamlined console management, making it easier to work with convars in your projects.
RegDefaultConVars registers an assortment of useful convars, giving you a head start on setting up your console.
In addition to RegDefaultConVars, Inv 0.6.0 also introduced RegConVar, which registers a new convar to be used in the console.
This means you can now create and manage custom convars with ease, thanks to the improved functionality in Inv 0.6.0.
Convar Documentation and Resources
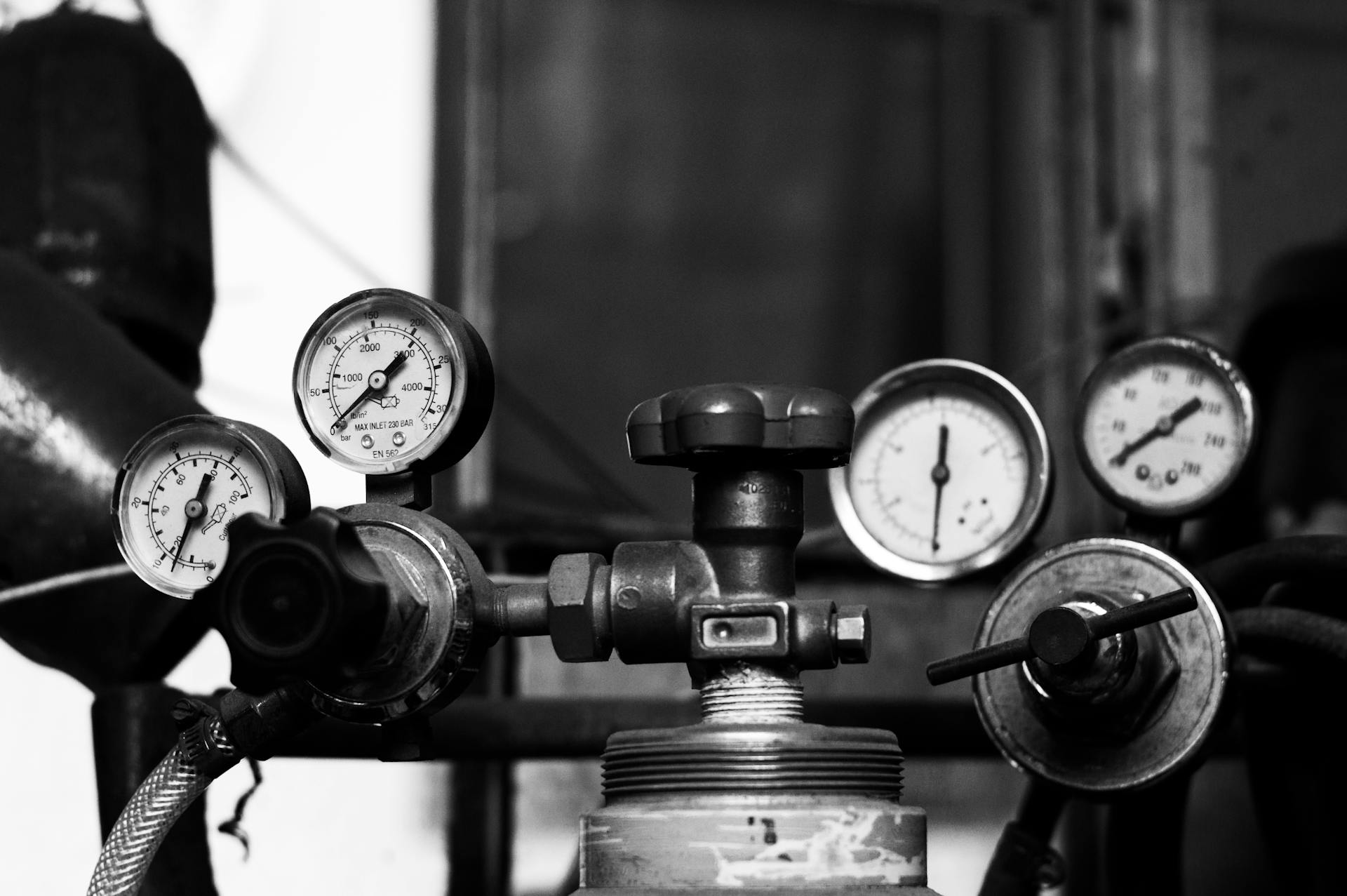
The official Convar documentation is available on their website, providing detailed information on how to use the Convar library.
You can find the Convar documentation by visiting their website and navigating to the "Documentation" section.
The documentation includes tutorials, guides, and reference materials to help you get started with Convar and troubleshoot any issues you may encounter.
Using Resources
You can set and get convar values using CFX API Set natives. This is an alternative to running ExecuteCommand() with the command as an argument, which requires the resource to have the command.set ace permission allowed.
To set a value, use the SetConvar() function, which works the same way in Lua, JS, or C# resources. You'll need to provide a default value, as GetConvar() will return this value if the convar hasn't been set yet.
GetConvarInt() is a variation of GetConvar() that's specifically for integer values, and it's a good option if you're sure your convar will only contain integers. The second parameter is the default return value.
Standard convars can only be used in server-side scripts, and they can't be obtained or set from a client script. This is an important thing to keep in mind when working with convars.
Documentation
Documentation is key to mastering ConVars. A ConVar's name should be a string with no spaces, and it's always given as a string even for ConVars with numerical values.
To create a ConVar, you'll need to use the ConVar class constructor, which takes several arguments, including the variable name, default value, flags, and a help string. The pName argument is the variable name, and the pDefaultValue is always given as a string.
Flags specify special characteristics of the variable, and all flag definitions start with a FCVAR_ prefix. You can find more information about these flags in the ConVar documentation.
The SetValue() function is used to set the value of a ConVar, and it allows all types of data. This means you can use it to set integer, float, or string values.
A ConVar can be reverted back to its original default value using the Revert() function. This is useful if you need to reset the ConVar to its original state.
The ICvar interface function FindVar() can be used to get a pointer to a ConVar object, if the variable name is known. However, this is an expensive search function and the pointer should be cached if reused often.
A range of valid values can be specified for numerical ConVars using a different constructor. This ensures that the ConVar value is always within a certain range.
Convar Server and Client Variables
You can create both server and client ConVars, but they're used in different ways. Server ConVars are created using the CreateConVar function, which is a serverside function that creates a server ConVar. Clients won't be able to access this ConVar unless the FCVAR_REPLICATED flag is set.
There are two types of ConVars: client ConVars and server ConVars. Client ConVars are created using the CreateClientConVar function, which is a clientside function used to create client ConVars. Only the client can see the ConVars you make, unless the 4th argument is set to true.
You can use the following functions to create ConVars:
What Is a Variable?
A variable is a fundamental concept in programming, and ConVars are a type of variable used in game development. ConVars store information that can be accessed using the developer console.
ConVars are short for "console variable" and can be accessed using the developer console. They're used to store information on the client and server.
There are two types of ConVars: client ConVars and server ConVars. Client ConVars are created using the CreateClientConVar function, which is a clientside function. Server ConVars are created using the CreateConVar function, which is a serverside function.
Client ConVars are only accessible to the client, unless the 4th argument is set to true. This means that only the client can see the ConVars you make using CreateClientConVar. Server ConVars, on the other hand, can be accessed by both the client and server, but only if the FCVAR_REPLICATED flag is set.
Here are some examples of what ConVars can store:
- ContentHeader
- ContentIcon
- ContentSidebar
- ContextBase
- ControlPanel
- ControlPresets
- DAdjustableModelPanel
- DAlphaBar
- DBinder
- DBubbleContainer
- DButton
- DCategoryList
- DCheckBox
- DCheckBoxLabel
- DCollapsibleCategory
- DColorButton
- DColorCombo
- DColorCube
- DColorMixer
- DColorPalette
- DColumnSheet
- DComboBox
- DDragBase
- DDrawer
- DEntityProperties
- DExpandButton
- DFileBrowser
- DForm
- DFrame
- DGrid
- DHorizontalDivider
- DHorizontalScroller
- DHScrollBar
- DHTML
- DHTMLControls
- DIconBrowser
- DIconLayout
- DImage
- DImageButton
- DKillIcon
- DLabel
- DLabelEditable
- DLabelURL
- DListBox
- DListBoxItem
- DListView
- DListView_Column
- DListView_Line
- DMenu
- DMenuBar
- DMenuOption
- DMenuOptionCVar
- DModelPanel
- DModelSelect
- DModelSelectMulti
- DNotify
- DNumberScratch
- DNumberWang
- DNumSlider
- DPanel
- DPanelList
- DPanelOverlay
- DPanelSelect
- DPanPanel
- DProgress
- DProperties
- DProperty_Combo
- DProperty_Float
- DProperty_Generic
- DProperty_VectorColor
- DPropertySheet
- DRGBPicker
- DScrollPanel
- DShape
- DSizeToContents
- DSlider
- DSprite
- DTab
- DTextEntry
- DTileLayout
- DTooltip
- DTree
- DTree_Node
- DVerticalDivider
- DVScrollBar
- IconEditor
- ImageCheckBox
- Material
- MatSelect
- PropSelect
Server Replicated Variables
Server replicated convars are a great way to share data between your server and client scripts. They allow you to make convar values available on the client side, but only allow changes to be made from the server side.
To make a convar server replicated, you'll need to use the "Use the following function to set a server replicated convar in a server script" command. This is a simple way to sync up your convar values.
You can also get server replicated convars from either a server or client script using the same function. This makes it easy to access the values you need.
Server information convars are another type of convar that can be displayed on the server connect details/info page and in the server list. They can also be exposed in the server info file.
To set a server information convar, use the "To set a server information convar, use the following command" command. This is a great way to share information about your server with players.
Convar Console and List Management
You can use the CFX API set functions to manage convars in the console and list them out.
There are a few key functions to know: GET_CONVAR, GET_CONVAR_INT, SET_CONVAR, SET_CONVAR_REPLICATED, and SET_CONVAR_SERVER_INFO. These functions allow you to get or set convar values, making it easy to customize and control your game.
Here's a quick rundown of the functions you can use:
- GET_CONVAR: Gets the value of a convar.
- GET_CONVAR_INT: Gets the integer value of a convar.
- SET_CONVAR: Sets the value of a convar.
- SET_CONVAR_REPLICATED: Sets a convar value that will be replicated to all clients.
- SET_CONVAR_SERVER_INFO: Sets a convar value that will be used as server info.
List of
Convar functions are used to get or set convars, and there are several types to choose from. You can use GET_CONVAR to retrieve a convar's value, GET_CONVAR_INT to get an integer convar, and SET_CONVAR to set a convar's value.
Here are some specific convar functions you can use:
- GET_CONVAR
- GET_CONVAR_INT
- SET_CONVAR
- SET_CONVAR_REPLICATED
- SET_CONVAR_SERVER_INFO
Resetting all convars to their default values is as simple as calling ResetAllVar. This function doesn't trigger the set/update callback.
Save
The Save function is a lifesaver for managing convars. It saves all non-default convar values to a given config file.
To use the Save function, you can call it on the Console object, like this: func (*Console) Save.
This is particularly useful when you want to preserve custom settings between sessions.
Quake-Like Console Implementation
The convar console implementation is a Quake-like console that offers a range of features for game development.
It's type-safe, which means it ensures the correct data type is used for each variable.
Variables can be of int, bool, float64, or string primitive types.
A special func type is also available, which accepts a single argument of any primitive type.
Variables can trigger a callback function when set or updated.
The implementation is concurrent safe, so you can use it in multi-threaded environments without worrying about data corruption.
Variable names are case insensitive, making it easier to use and manage them.
The convar console also includes helper functions, as well as the ability to save and load variables to and from a file.
You can even evaluate and execute simple commands, and get simple autocomplete functionality.
Console Variables
Console Variables are a crucial part of the Convar Console and List Management system. They can be used to store and retrieve values, and are essential for creating custom commands and variables.
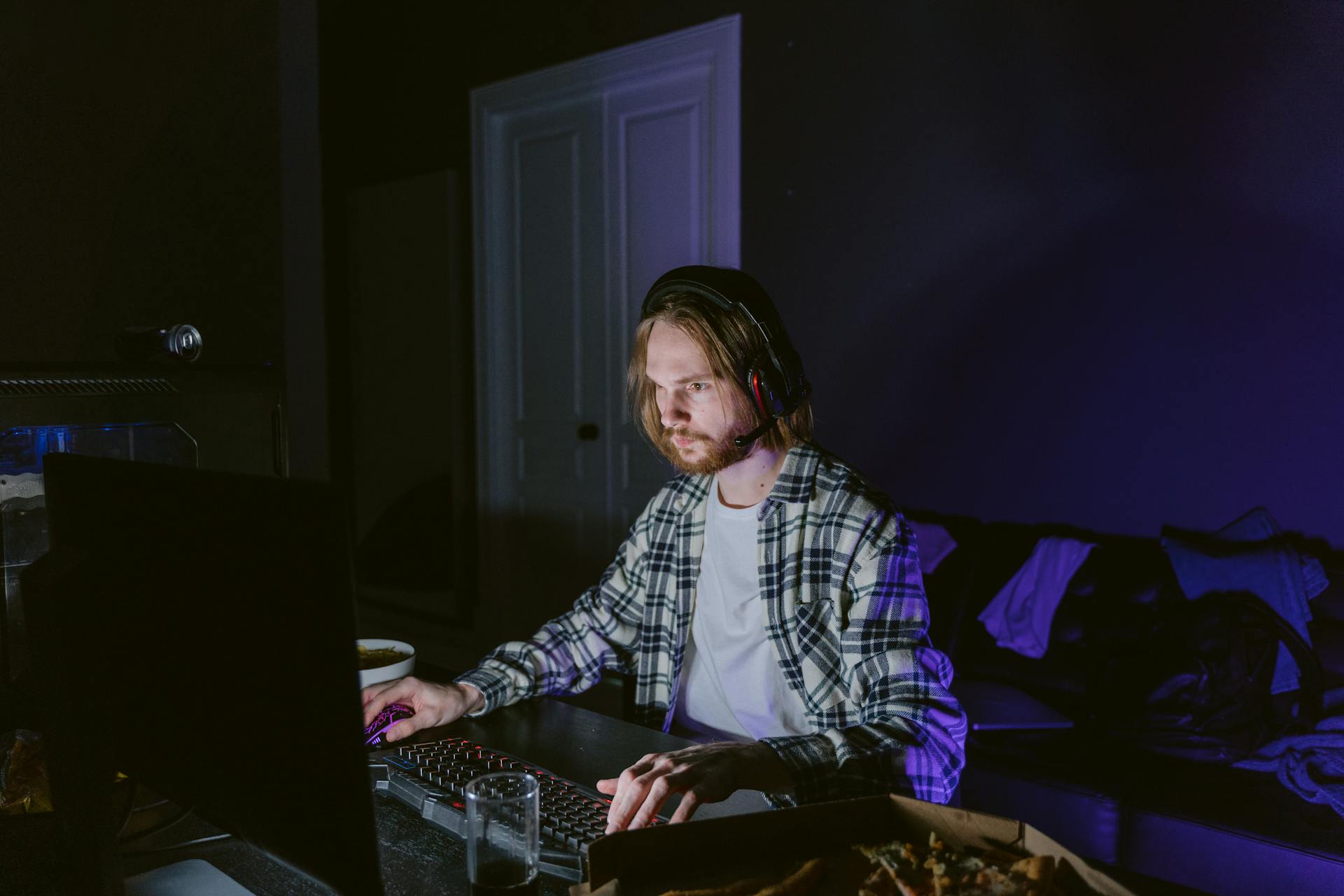
You can use the GET_CONVAR and GET_CONVAR_INT functions to retrieve the value of a convar. These functions are part of the CFX API set and can be used to get or set convars.
ConVars can be saved to a config file using the Save function of the Console class. Only non-default values are saved, so make sure to set the convar value before saving.
Adding new commands and variables is a straightforward process. You can use the ConCommand and console_variables classes to create a new command or variable. The constructor of these classes automatically registers the command at the console system.
Server replicated convars are a special type of convar that can be synced between the server and client scripts. To make a convar server replicated, use the SET_CONVAR_REPLICATED function. This will allow you to change the convar value from the server side, and get the value from both the server and client scripts.
Here are the different types of convars you can create:
- Server convars: These can be used to store values that are specific to the server.
- Client convars: These can be used to store values that are specific to the client.
- Server replicated convars: These can be used to store values that need to be synced between the server and client scripts.
Frequently Asked Questions
What is ConVar fivem?
A ConVar in FiveM is a customizable configuration variable that can be accessed and modified from various sources, including the server console and RCON clients. This allows for flexible and centralized management of game settings and configurations.
Featured Images: pexels.com