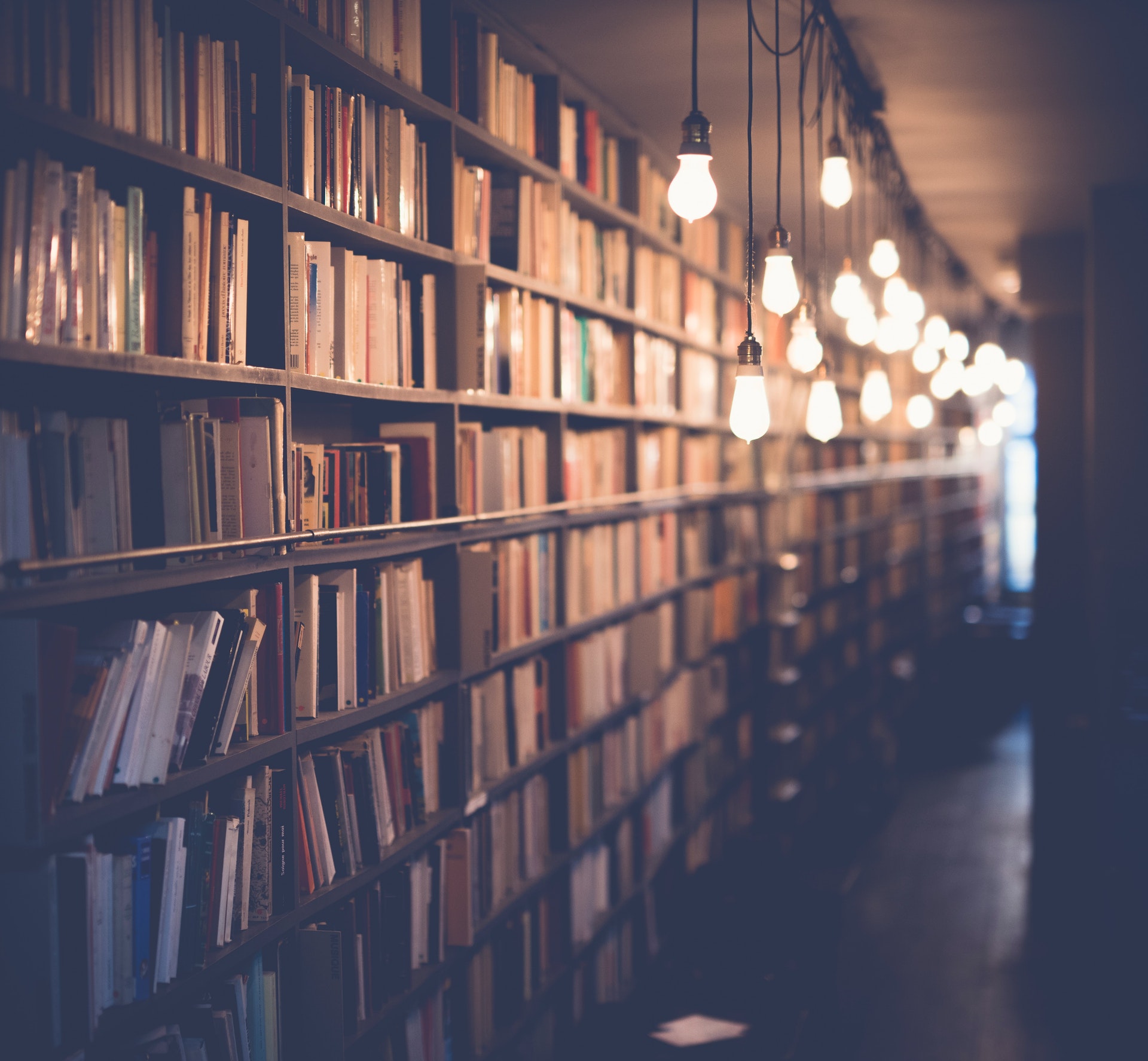
If you want to create an empty branch in Git, you can do so using the --orphan flag when you run git checkout. This will create a new branch that has no history, which can be useful for things like starting work on a new feature or fixing a bug in an isolated environment. To create an empty branch, simply run:
git checkout --orphan
This will create a new branch with the given name and no history. You can then start adding commits to this branch as normal. When you're ready to push your changes up to a remote repository, you can use the -u flag to set the upstream branch:
git push -u origin
This will push your changes to the remote repository and set the given branch as the upstream branch for the local one. From then on, you can simply use git push to push your changes up to the remote.
Curious to learn more? Check out: Honda Civic Run
What is a git branch?
A git branch is a logical entity that represents a separate line of development. Branches serve as an abstraction for the underlying Git repository and allow developers to work on different versions of a project simultaneously. By default, a Git repository has a single branch named "master" which is the primary development branch.
Git branches are lightweight and can be created and deleted easily. When a branch is created, it is simply a pointer to a commit. A commit is a snapshot of the changes made to a project. Creating a new branch just adds a new pointer to an existing commit.
Deleting a branch simply removes the pointer. Because branches are so cheap and easy to create, it is common for developers to create a new branch for every new feature or bug fix they work on. This allows them to isolate their changes from the rest of the codebase and makes it easy to integrate the changes when they are ready.
Git branches are also useful for creating prototypes or experimenting with new ideas without affecting the main codebase. This is because changes made on a branch do not affect the other branches in the repository.
In summary, a git branch is a separate line of development that is cheaper and easier to create than a traditional branch.
Intriguing read: Throw Neighbors Tree Branches Back
How do you create a new branch in git?
In git, creating a new branch is a simple three-step process.
First, you need to checkout the branch you want to create the new branch from. For example, if you want to create a new branch from the master branch, you would run the following command:
git checkout master
Next, you need to create the new branch. This is done with the git branch command. For example, to create a new branch called my-new-branch, you would run the following command:
git branch my-new-branch
Finally, you need to checkout the new branch. This is done with the git checkout command. For example, to checkout the my-new-branch branch, you would run the following command:
git checkout my-new-branch
Now, you can make changes to the new branch and when you're ready, you can merge it back into the master branch.
Additional reading: How to Create Your Own Ransomware?
How do you checkout a branch in git?
There are many ways to checkout a branch in git. The most common way is to use the 'git checkout' command followed by the name of the branch you want to checkout. For example, if you want to checkout the 'development' branch, you would run the following command:
git checkout development
This will switch you to the 'development' branch. If you want to create a new branch, you can use the 'git checkout -b' command followed by the name of the new branch. For example, if you want to create a new branch called 'feature-x', you would run the following command:
git checkout -b feature-x
This will create a new branch called 'feature-x' and switch you to that branch.
If you want to checkout a remote branch, you can use the 'git checkout' command followed by the name of the remote branch. For example, if you want to checkout the 'origin/development' branch, you would run the following command:
git checkout origin/development
This will switch you to the 'origin/development' branch.
You can also use the 'git branch' command to list all of the branches in a repository. To see all of the remote branches, you can use the '-r' flag. For example, to see all of the remote branches in a repository, you would run the following command:
git branch -r
This will list all of the remote branches in the repository.
A fresh viewpoint: How Can We Name a Love?
How do you merge two branches in git?
Merging two branches in git can be done in a few different ways. The most common way is to use the "git merge" command. This command will take two branches and combine them into one.
There are a few things to keep in mind when merging branches. The first is that you need to have a clean working directory. This means that all of your changes should be committed and there should be no outstanding changes.
The second thing to keep in mind is that the order of the branches matters. The branch that you merge into should be the branch that you want to keep. The branch that you are merging should be the branch that you want to delete.
The final thing to keep in mind is that you need to have a clear understanding of what changes are being made. The git merge command will show you a diff of the two branches. This diff will show you what changes are being made to each file. If there are conflicts, they will be highlighted in the diff.
Once you have a clean working directory and you understand the changes that are being made, you are ready to merge the branches. To do this, you will use the "git merge" command. The syntax for this command is:
git merge [branch to merge]
So, if you want to merge the "development" branch into the "master" branch, you would run the following command:
git merge development
This command will take the changes from the "development" branch and apply them to the "master" branch. If there are any conflicts, you will need to resolve them. Once the merge is complete, you will need to commit the changes.
git merge can also be used to merge multiple branches at once. To do this, you will use the "--no-ff" option. This option tells git to do a "Fast Forward" merge. This means that git will take all of the changes from the branch that you are merging and apply them to the branch that you are merging into.
git merge --no-ff [branch to merge]
So, if you want to merge the "development" branch into the "master" branch, you would run the following command:
git merge --no-ff development
This command will take the changes from the "development" branch and apply them to the "master" branch. If there are any conflicts
Explore further: How Empty of Me to Be so Full of You?
What is a fast-forward merge in git?
A fast-forward merge is a type of merge that is used in git when the commit history is linear. This type of merge is fast and easy to do because it doesn't require any special merge algorithms. The downside to fast-forward merges is that they can be less flexible than other types of merges, and they can make it harder to see what has changed in the history of a project.
How do you delete a branch in git?
Deleting a branch in git is a simple process that can be done in a few steps. First, you will need to checkout the branch that you want to delete. Next, you will need to run the git branch command with the -d (or --delete) option and the name of the branch you want to delete. Finally, you will need to push the changes to the remote repository.
You might like: Branch Mint
What is a remote branch in git?
For those not familiar with git, a remote branch is simply a branch that is located on a remote server. When working with a remote branch, you can push and pull changes to and from the server. Typically, you would use a remote branch when working with a team of developers, as it allows everyone to collaborate on the same codebase.
There are a few things to keep in mind when working with remote branches. First, you will need to have a remote repository set up. This is typically done by your team's lead developer. Secondly, you will need to be aware of the branch's push and pull URLs. These can be found in the repository's settings.
Finally, it is important to remember that remote branches are not always synchronized with the local branches. This means that if you make a change to a remote branch, you will need to push those changes in order for them to take effect. Similarly, if someone else makes a change to the remote branch, you will need to pull those changes in order to see them.
For more insights, see: When Can I Order a Maverick?
How do you push a branch to a remote in git?
When you want to push a branch to a remote in git, there are a couple different ways that you can do this. The first way is by using the git push command. This will push all of the local commits that you have made on the branch to the remote.
The second way that you can push a branch to a remote is by using the git push origin command. This will push the branch to the remote that is specified by the “origin” remote.
The third way to push a branch to a remote is by using the git push –u origin command. This will push the branch to the remote and set the upstream branch.
The fourth way to push a branch to a remote is by using the git push –f origin command. This will force push the branch to the remote.
The fifth and final way to push a branch to a remote is by using the git push –d origin command. This will delete the branch from the remote.
How do you pull a branch from a remote in git?
First, you'll need to find the remote's URL. To do this, open Git Bash and navigate to your repository. Then, type in the following command:
git remote -v
You should see something like this:
origin https://github.com/username/repository.git (fetch) origin https://github.com/username/repository.git (push)
The URL you're looking for is the one that comes after "origin." In this case, it would be "https://github.com/username/repository.git."
Next, you'll need to generate a personal access token. You can do this by going to your profile settings on GitHub. Once you're there, go to the "Developer settings" section and select "Personal access tokens." Then, click on "Generate new token."
You'll be prompted to give your token a name and select the scopes. For this application, you'll need the "repo" scope. Once you've done that, click on "Generate token."
Copy the token and head back to Git Bash. In the repository, type in the following command:
git remote set-url origin https://[token]@github.com/username/repository.git
Replace "[token]" with your personal access token.
Now, you should be able to pull the branch from the remote.
Frequently Asked Questions
How to create a new branch in Git without a commit?
If you want to create a new branch without committing anything, you can use the checkout/checkout --orphan flag.
How do I push an empty branch to GitHub?
First, make sure that you have a branch on your local machine that you want to push to GitHub. To create a new branch: git branch my-new-branch Next, commit the changes you want to push to the branch: git commit -am 'Added some content' Finally, push the branch to GitHub: git push origin my-new-branch
How do I create an empty branch with no history?
You can use git switch --orphan
How to create an orphan branch in Git?
1. In your local repository, create a new branch called "orphan" by running the following command: git branch -b orphan . 2. Remove everything from the branch by running the following command: git rm -rf . 3. Make some changes and commit them to the branch. 4. Push the commits made on the branch to the remote repository by running the following command: git push origin orphan .
How do I create a new branch from a commit?
To create a new branch from a commit, use the “git checkout” command with the “-b” option and specify the branch name as well as the commit to create your branch from. Alternatively, you can use the “git branch” command with the branch name and the commit SHA for the new branch.
Sources
- https://www.shellhacks.com/git-create-empty-branch/
- https://www.codingem.com/what-is-head-in-git/
- https://knowledgeburrow.com/how-do-i-create-a-new-branch-in-git/
- https://www.javatpoint.com/git-checkout
- https://gist.github.com/ozh/4734410
- https://www.reddit.com/r/git/comments/zcvrn9/you_have_divergent_branches_and_need_to_specify/
- https://nsnsearch.com/faq/can-we-create-empty-branch-in-git/
- https://www.educba.com/what-is-git-branch/
- https://www.git-tower.com/learn/git/faq/create-branch/
- https://tecadmin.net/git-create-empty-branch/
- https://arjunphp.com/git-create-empty-branch/
- https://www.freecodecamp.org/news/git-switch-branch/
- https://www.w3docs.com/snippets/git/checking-out-a-remote-branch-in-git.html
- https://bytenota.com/git-create-a-new-empty-branch/
- https://stackoverflow.com/questions/70589162/how-do-i-create-a-new-branch-in-git
Featured Images: pexels.com