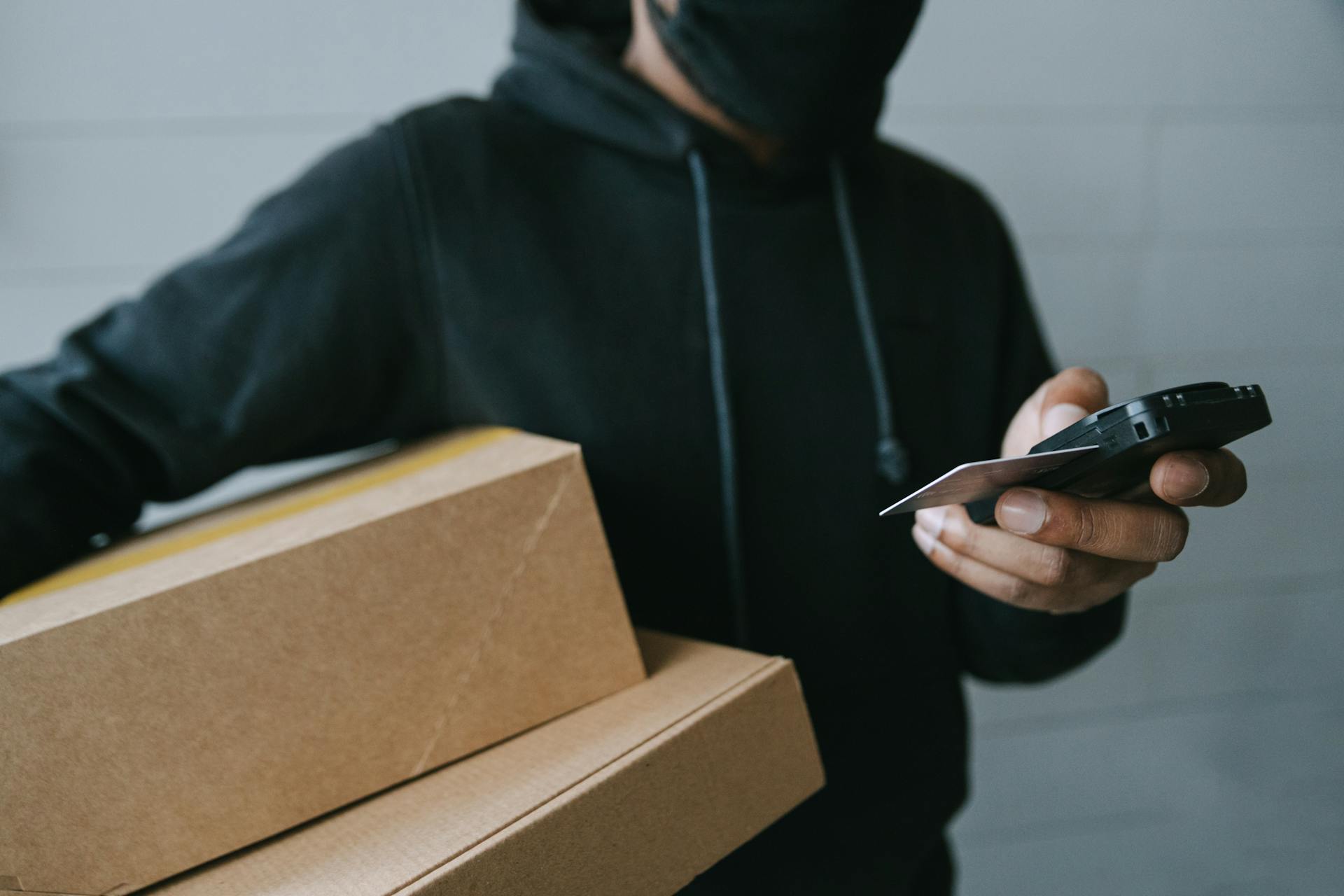
Algorithmic trading with Python can seem daunting at first, but with the right tools and knowledge, it's a powerful way to automate trading decisions.
Python's simplicity and flexibility make it an ideal language for algorithmic trading.
One of the key benefits of using Python for algorithmic trading is its extensive libraries, including Pandas and NumPy, which provide efficient data analysis and manipulation capabilities.
With these libraries, you can quickly and easily process large datasets, identify trends, and make informed trading decisions.
To get started with algorithmic trading in Python, you'll need to familiarize yourself with popular libraries like Zipline and Backtrader, which provide robust backtesting and trading capabilities.
These libraries allow you to create and test trading strategies, backtest their performance, and even execute trades automatically.
By leveraging these tools and libraries, you can streamline your trading process, reduce manual errors, and make more informed investment decisions.
For your interest: Algorithmic Trading System
Getting Started
To get started with algorithmic trading with Python, you'll need to install the required libraries. These include pandas, numpy, matplotlib, yfinance, and pandas_ta, which can be installed using pip like any other Python library.
Discover more: Algorithmic Stock Trading and Equity Investing with Python
You can install these libraries directly from the Python Package Index (PyPI) using pip. For example, you can install yfinance using the command `pip install yfinance`. Once you've installed the libraries, you can import them in your Python code.
Python's ease of use and extensive libraries make it a great language for algorithmic trading. However, it may not offer the same execution speed as lower-level languages like C++.
Discover more: Ball Python Burrowing
Getting Started
To get started with Python for algorithmic trading, you'll need to have a recent version of Python installed. You can use a custom build of ActivePython that includes a version of Python and just the packages the project requires, or install a recent version yourself.
Installing Python is a straightforward process. For this article, I'll be using a custom build of ActivePython, but you can follow the same steps to install a recent version on your own computer.
To follow along with the code in this article, you'll need to have the required libraries installed, including pandas, numpy, matplotlib, yfinance, and pandas_ta. These modules can be directly installed using pip like any other Python library.
Here are the top reasons why Python is the perfect choice for algorithmic trading:
- Ease of Use: Python's simple and readable syntax makes it accessible to both novice and experienced programmers.
- Extensive Libraries: Python offers a rich ecosystem of libraries and frameworks, such as NumPy, pandas, and scikit-learn, which are essential for data analysis and machine learning.
- Community Support: A robust and active community provides abundant resources, tutorials, and forums to assist developers.
- Integration Capabilities: Python easily integrates with other programming languages and platforms, making it versatile for various trading systems.
While Python's ease of use and extensive libraries come with a trade-off, offering slower execution speed compared to lower-level languages like C++, its benefits make it an ideal choice for algorithmic trading.
Online Courses
Getting started with trading and machine learning requires a solid foundation in both areas.
The Coursera course "Machine Learning for Trading" by Georgia Institute of Technology is a great resource for learning the fundamentals of trading and machine learning.
If you're looking for hands-on experience, Udemy courses like "Algorithmic Trading with Python and Pandas" can help you develop trading strategies using Python and Pandas.
Data Acquisition
Data Acquisition is a crucial step in algorithmic trading, and it's essential to get it right. High-quality data is the foundation of any algorithmic trading strategy.
You'll need to acquire historical price data, real-time market data, and other relevant information like news feeds and economic indicators. Python's libraries, like yfinance and pandas-datareader, can facilitate seamless data acquisition and manipulation.
On a similar theme: Algorithmic Trading and High Frequency Trading
However, the quality and integrity of data are paramount. Inaccurate or incomplete data can lead to flawed strategies. This is why it's essential to verify the accuracy of your data before using it in your trading strategy.
Here are some key considerations for data acquisition:
- Historical price data: This includes past prices of the assets you're interested in trading.
- Real-time market data: This includes current prices, volumes, and other market data.
- News feeds and economic indicators: These can provide valuable insights into market trends and sentiment.
By acquiring high-quality data and using the right tools, you can build a solid foundation for your algorithmic trading strategy.
Strategy Development
Strategy Development is a crucial step in algorithmic trading with Python. You can develop a trading strategy using simple moving averages, complex machine learning models, or a combination of both. Python's scientific libraries, such as NumPy and SciPy, provide the tools needed for statistical analysis and model development.
Start with simple strategies, such as moving average crossovers, and gradually progress to more complex models. Experiment with backtesting frameworks like Backtrader to refine your strategies. Try implementing a simple moving average crossover using historical stock data from Yahoo Finance.
A fresh viewpoint: Crypto Trading Bot Development Company
In this process, you can use indicators like SMA and MACD, which are two of the simplest yet extremely popular indicators for stock trading. You can calculate these values using the pandas_ta library and encode them to understand if there are any crossovers.
The next step is to develop a trading strategy that incorporates these indicators and other factors. This could range from simple moving averages to complex machine learning models. By using Python's scientific libraries, you can develop a strategy that is tailored to your specific needs and goals.
Backtesting and Optimization
Backtesting is crucial to evaluate a strategy's performance before deploying it in live markets. It involves simulating the strategy against historical data using libraries like Backtrader and PyAlgoTrade.
To backtest a trading strategy, you need to consider metrics such as profitability, drawdown, and Sharpe ratio. Trality provides a full suite of metrics to use when testing a strategy, categorized into performance, risk-return, and runs.
The Sharpe ratio is a popular risk-return measure that provides a better understanding of an investment's return compared to its risk. A high Sharpe ratio indicates that the investment managed to keep the portfolio safe while making a nice return.
Total return is a performance statistic that represents the accumulated net profit or loss for a given time horizon. It's calculated as the difference between the portfolio value at the start and end times.
Maximum drawdown is an indicator of downside risk over a specified period of time. It's the maximum observed loss from a peak to a trough of a portfolio before a new peak is attained.
Optimizing a trading strategy's parameters can be challenging and time-consuming. Trality's Optimizer can help with this process by finding the optimal parameters for the strategy.
The Optimizer uses @parameter annotations to optimize the parameters of the strategy. It can be activated under advanced settings to run the optimization process.
By running the Optimizer, you can find the optimal parameter values for the strategy. For example, the optimal parameter for ema_short is 6 and for ema_long is 21.25.
Walkforward Analysis is a robust algorithm that divides historical data into multiple time windows and walks forward in time, emulating the process of executing and retraining the strategy with new data in the real world.
For another approach, see: Bitcoins Trade Investment
Execution and Deployment
Execution involves sending orders to the market, managing positions, and monitoring performance, which can be done seamlessly with Python's integration capabilities with APIs from brokers and exchanges.
Python's integration with APIs from brokers and exchanges like Interactive Brokers and Alpaca enables this process.
To deploy your trading strategy, you can use Trality's Code Editor for FREE to tweak the settings and get a feel for the platform.
Trality's Code Editor allows you to tweak settings and get a better feel for the platform and what it can do for you.
Once you're happy with your Python trading bot, you can deploy it for virtual trading using Trality.
Virtual trading allows you to see how the strategy performs in real-world conditions without any risk to your finances.
Remember, virtual trading is just that—no real funds are used.
You can choose from Trality's constantly expanding list of exchanges for live trading, including Binance, Binance.US, Kraken, Bitpanda, FTX, and Coinbase Pro.
Intriguing read: Daytrading Platforms
Technical Analysis and Indicators
Technical analysis is a crucial aspect of algorithmic trading with Python. It involves analyzing charts and patterns to make informed trading decisions. By using technical indicators, you can identify trends and make predictions about future price movements.
Some popular technical indicators include the Simple Moving Average (SMA) and the Moving Average Convergence Divergence (MACD). The SMA is calculated by taking the average of a stock's price over a specific period, while the MACD is a trend-following momentum indicator that calculates the difference between two moving averages.
The pandas_ta library provides an easy way to calculate these indicators in Python. You can use the "sma()" function to calculate the SMA and the "macd()" function to calculate the MACD. For example, you can use the "sma()" function to calculate a 10-day, 30-day, 50-day, and 200-day moving average.
Here are some common technical indicators used in algorithmic trading:
- SMA (Simple Moving Average)
- MACD (Moving Average Convergence Divergence)
- EMA (Exponential Moving Average)
- QQE (Quantitative Qualitative Estimation)
- RSI (Relative Strength Index)
These indicators can be used to identify trends, predict price movements, and make informed trading decisions. By combining these indicators with other technical analysis tools, you can develop a robust algorithmic trading strategy with Python.
Building Strategies with Technical Indicators
Technical indicators are a crucial part of technical analysis, and they can be used to build effective trading strategies. We can use indicators like SMA and MACD to identify trends and generate buy and sell signals.
SMA stands for Simple Moving Average, and it's calculated by taking the average of a stock's price over a specified period. We can use the pandas_ta library to calculate SMA values.
MACD stands for Moving Average Convergence Divergence, and it's used to identify trends and generate buy and sell signals. We can use the macd() function from the pandas_ta library to calculate MACD values.
Some popular technical indicators include:
We can use these indicators to build trading strategies that are tailored to our individual needs. For example, we can use the SMA crossover strategy, which involves buying when the short-term SMA crosses above the long-term SMA, and selling when it crosses below.
Intriguing read: Short-term Trading
In addition to SMA and MACD, we can also use other indicators like EMA and QQE to build more complex trading strategies. EMA stands for Exponential Moving Average, and it's similar to SMA but gives more weight to recent prices. QQE stands for Quantitative Qualitative Estimation, and it's used to identify trends and generate buy and sell signals.
By combining multiple indicators and strategies, we can create a robust trading system that's able to adapt to changing market conditions.
Expand your knowledge: Currency Trading Strategies
Fetch Indicator Data and Asset Price
To fetch indicator data and asset price, you'll need to use an EMA (Exponential Moving Average) of 5 and 20 to identify the shorter trend. This involves fetching the indicator data first.
We'll use the QQE (Quantitative Quality Estimation) indicator with settings (20, 5, 4.2) to enter the trade at the correct moment. This requires fetching the asset price from data.
To enter a trade, the asset price should be below an EMA of 5. This means you'll need to fetch the asset price from data.
You can use the following indicators to identify trends: EMA of 5 and 20, and QQE with settings (20, 5, 4.2).
Here are the steps to fetch indicator data and asset price:
- Fetch the EMA of 5 and 20
- Fetch the QQE with settings (20, 5, 4.2)
- Fetch the asset price from data
By following these steps, you'll be able to fetch the necessary data to inform your trading decisions.
Frequently Asked Questions
Is Python or C++ better for algo trading?
For algo trading, Python is often preferred due to its faster development time, allowing you to get to market sooner. However, C++ may be a better choice if execution speed is your top priority.
Is Python good for trading bot?
Yes, Python is a popular choice for creating trading robots due to its simplicity and extensive libraries. It's an excellent choice for beginners and professionals alike in algorithmic trading and quantitative finance.
Is Python too slow for algorithmic trading?
Python's performance is generally sufficient for algorithmic trading, as the bottleneck is often the broker and API, not the programming language itself. However, the speed of your trading strategy may still be limited by external factors, making optimization and efficient coding crucial for success.
Sources
- https://www.activestate.com/blog/how-to-build-an-algorithmic-trading-bot/
- https://www.analyticsvidhya.com/blog/2023/10/building-and-validating-simple-stock-trading-algorithms-using-python/
- https://pyquantnews.com/topics/algorithmic-trading-with-python
- https://medium.com/@edtechre/algotrading-in-python-with-machine-learning-walkforward-analysis-1ebfb8a9fcf0
- https://www.trality.com/blog/algorithmic-trading
Featured Images: pexels.com