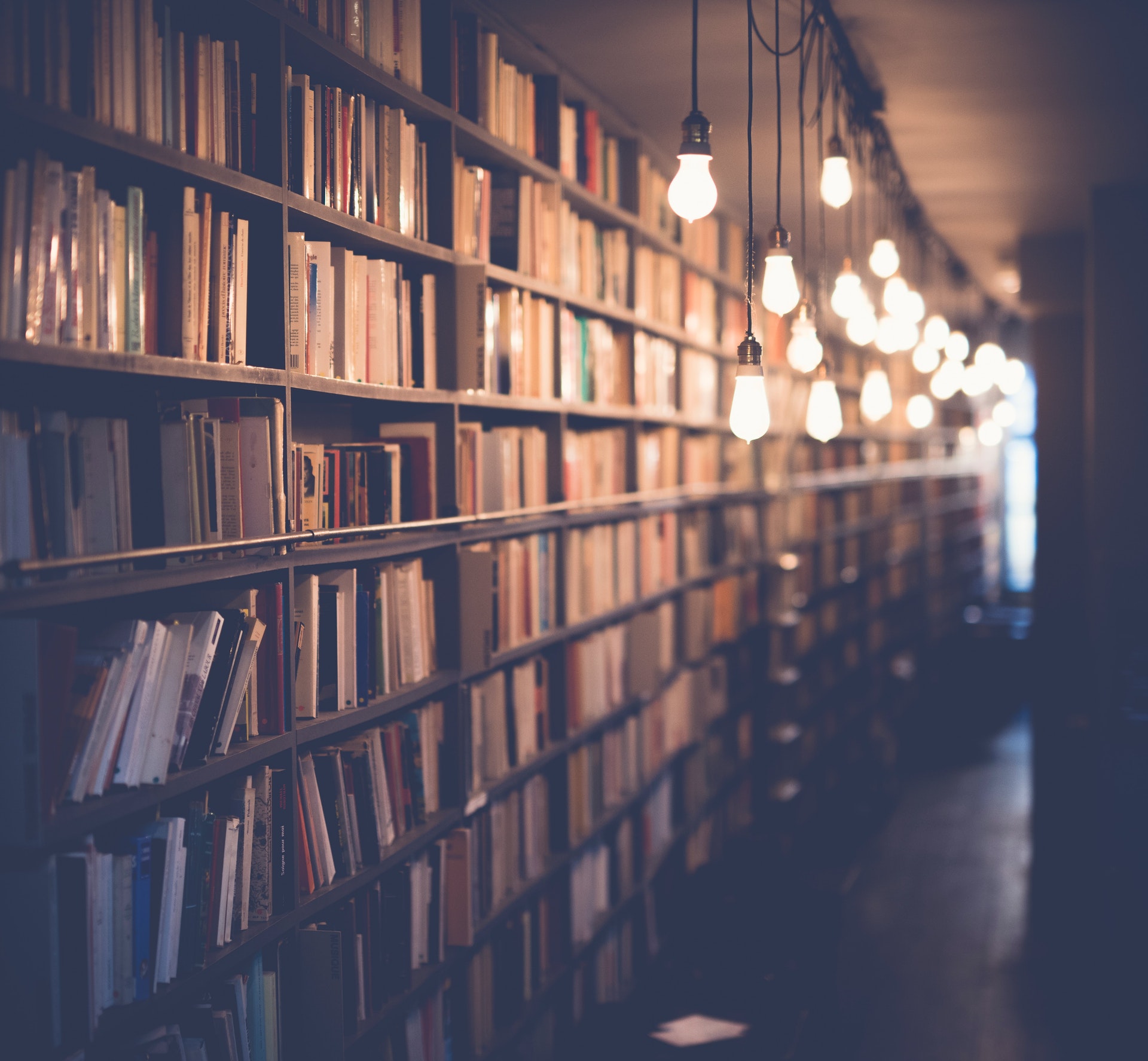
Visual Basic has a large number of built-in functions. These functions are categorized into the following types:
Arithmetic functions
Bit-level functions
Casting functions
Character functions
Date and time functions
File functions
Logical functions
Miscellaneous functions
String functions
Type conversion functions
Some of the more commonly used functions are described below.
Arithmetic Functions
These functions perform basic arithmetic operations.
Function name Description Abs Returns the absolute value of a number. Ceiling Returns the smallest integer greater than or equal to a number. Floor Returns the largest integer less than or equal to a number. Exp Returns e (the base of natural logarithms) raised to a power. Fix Returns the integer portion of a number. Log Returns the natural logarithm of a number. Mod Returns the remainder of a division operation. Power Returns a number raised to a power. Random Returns a random number between 0 and 1. Round Returns a number rounded to a specified number of decimal places. Sgn Returns a number that indicates the sign of a number.
Bit-Level Functions
These functions perform operations on the bit level.
Function name Description And Performs a bitwise AND operation. Eqv Performs a bitwise equivalence operation. Imp Performs a bitwise implication operation. Not Performs a bitwise negation operation. Or Performs a bitwise OR operation. Xor Performs a bitwise exclusive OR operation.
Casting Functions
These functions allow you to convert values from one data type to another.
Function name Description CBool Converts a value to a Boolean data type. CByte Converts a value to a byte data type. CCur Converts a value to a currency data type. CDate Converts a value to a date data type. CDbl Converts a value to a double data type. CDec Converts a value to a decimal data type. Char Converts a value to a character data type. CInt Converts a value to an integer data type. CLng Converts a value to a
What are some of the most commonly used built in functions in vb?
In Visual Basic, there are many built in functions that can be used to perform various tasks. Some of the most commonly used built in functions are the ones that allow you to manipulate strings, convert values between data types, and perform mathematical operations.
The String function is one of the most commonly used functions in Visual Basic. It allows you to manipulate strings in a variety of ways. For example, you can use the String function to concatenate two or more strings together, or to extract a sub string from a larger string. You can also use the String function to search for a specific string within another string, or to replace one string with another.
The Convert function is another commonly used function in Visual Basic. It allows you to convert values between different data types. For example, you can use the Convert function to convert a string to an integer, or to convert an integer to a string. You can also use the Convert function to convert a value from one data type to another data type that is compatible with it.
The Math function is a built in function that allows you to perform mathematical operations on values. For example, you can use the Math function to calculate the square root of a value, or to calculate the value of a number raised to a power. You can also use the Math function to perform trigonometric functions, such as calculating the sine or cosine of a value.
What do built in functions do in vb?
In Visual Basic, a built-in function is a function that is included in the Visual Basic language and can be called from your code. There are a variety of built-in functions, and they can be used to perform mathematical calculations, manipulate strings, and more.
Built-in functions can be called from your code using the following syntax:
FunctionName(arguments)
where FunctionName is the name of the function, and arguments are the values that are passed to the function.
Visual Basic includes a wide range of built-in functions. Some of the most commonly used functions are listed below:
Abs(number) - Returns the absolute value of a number
Asc(character) - Returns the ASCII code for a character
CDbl(expression) - Converts an expression to a Double data type
Chr(character code) - Returns the character that corresponds to a character code
CInt(expression) - Converts an expression to an Integer data type
CLng(expression) - Converts an expression to a Long data type
Cos(number) - Returns the cosine of a number
Exp(number) - Returns e (the natural logarithm base) raised to a power
Fix(number) - Returns the integer portion of a number
Hex(number) - Converts a number to hexadecimal
InStr(start position, string1, string2) - Searches for one string within another
LCase(string) - Converts a string to lowercase
Left(string, length) - Returns the leftmost characters of a string
Len(string) - Returns the length of a string
Log(number) - Returns the natural logarithm of a number
LTrim(string) - Removes leading spaces from a string
Mid(string, start, length) - Extracts a substring from a string
Oct(number) - Converts a number to octal
Replace(string, find, replacement) - Replaces one string with another
Right(string, length) - Returns the rightmost characters of a string
Rnd(number) - Returns a random number
Round(number, decimals) - Rounds a number to a specified number of decimal places
RTr
How do built in functions work in vb?
When working with Visual Basic, you may find yourself needing to use built in functions. These functions are already written and available for you to use in your programming. This can be extremely helpful, as you don't have to waste time writing your own functions. However, you may be wondering how these functions work.
In order to understand how built in functions work in Visual Basic, it is first important to understand what a function is. A function is a block of code that performs a specific task. Functions can take one or more parameters, and they will typically return a value. When you call a function, you are essentially asking the function to run the code and return a value.
Built in functions are functions that are written by Microsoft and included in Visual Basic. These functions are available for you to use in your programs without having to write your own code. This can be extremely helpful, as you don't have to waste time writing your own functions. However, you may be wondering how these functions work.
In order to understand how built in functions work in Visual Basic, it is first important to understand what a function is. A function is a block of code that performs a specific task. Functions can take one or more parameters, and they will typically return a value. When you call a function, you are essentially asking the function to run the code and return a value.
Built in functions are functions that are written by Microsoft and included in Visual Basic. These functions are available for you to use in your programs without having to write your own code. This can be extremely helpful, as you don't have to waste time writing your own functions. However, you may be wondering how these functions work.
In order to understand how built in functions work in Visual Basic, it is first important to understand what a function is. A function is a block of code that performs a specific task. Functions can take one or more parameters, and they will typically return a value. When you call a function, you are essentially asking the function to run the code and return a value.
Built in functions are functions that are written by Microsoft and included in Visual Basic.These functions are available for you to use in your programs without having to write your own code. This can be extremely helpful, as you don't have to waste time writing your own functions. However, you may be wondering how these functions work.
In order to understand how
What are the benefits of using built in functions in vb?
There are many benefits of using built in functions in vb. Built in functions make code more readable and prevent errors. They also make code more maintainable.
Some of the most popular built in functions are those that deal with strings. For example, the Left function returns the leftmost characters of a string, and the Right function returns the rightmost characters of a string. The Mid function returns a specific character or characters from a string. The Len function returns the length of a string.
The InStr function searches for a character or characters in a string, and returns the position of the character or characters within the string. The UCase and LCase functions convert a string to all uppercase or all lowercase letters, respectively. The StrComp function compares two strings and returns a value that indicates whether the first string is less than, equal to, or greater than the second string.
The Format function formats a value for output. The FormatCurrency function formats a value as a currency. The FormatNumber function formats a value as a number. The FormatPercent function formats a value as a percentage.
The CDate function converts a value to a date. The IsDate function determines whether a value can be converted to a date. The Date function returns the current system date. The Time function returns the current system time.
The Abs function returns the absolute value of a number. The Sgn function returns a value that indicates the sign of a number. The Min function returns the smaller of two values. The Max function returns the larger of two values.
The Randomize function seeds the random number generator. The Rnd function returns a random number.
The Int function rounds a number to the nearest integer. The Fix function rounds a number to the nearest integer less than or equal to the number. The Sqr function returns the square root of a number.
TheTan function returns the tangent of an angle. The Sin function returns the sine of an angle. The Cos function returns the cosine of an angle.
TheExp function returns e raised to a power. The Log function returns the natural logarithm of a number.
The Round function rounds a number to a specified number of decimal places. The Ceiling function rounds a number to the nearest integer greater than or equal to the number. The Floor function rounds a number to the nearest integer less than or equal to
What are some of the drawbacks of using built in functions in vb?
There are a few drawbacks to using built in functions in Visual Basic. First, if you're not careful, you can easily end up with code that is very difficult to read and maintain. Second, built in functions can sometimes be slower than hand-coded solutions. Finally, built in functions can sometimes be less reliable than hand-coded solutions.
How can built in functions be used to improve vb programs?
One of the great things about the Visual Basic programming language is the presence of built in functions. These functions can be used to improve the efficiency and quality of your programs.
The most basic built in function is the MsgBox function. This function allows you to display a message to the user. It is often used for debugging purposes.
Another built in function is the InputBox function. This function allows you to get input from the user. It is often used to get information that will be used in a calculation.
TheShell function is a built in function that allows you to open and close a Windows Shell. This can be used to launch other programs or to open and close files.
The Error function is a built in function that allows you to handle errors in your program. This is a very important function because it allows you to gracefully handle errors that might occur.
The Dir function is a built in function that allows you to get a list of files in a directory. This can be used to get a list of files to process or to verify that a file exists.
The Len function is a built in function that allows you to get the length of a string. This is often used to make sure that a string is the correct length.
The Asc function is a built in function that allows you to get the ASCII value of a character. This is often used to process text files.
The Val function is a built in function that allows you to convert a string to a number. This is often used when getting input from the user.
The FileLen function is a built in function that allows you to get the length of a file. This is often used to make sure that a file has the correct length.
TheFormat function is a built in function that allows you to format a string. This is often used to display numbers in a certain format or to display dates in a certain format.
The StrComp function is a built in function that allows you to compare two strings. This is often used to make sure that two strings are the same.
The InStr function is a built in function that allows you to find the position of a substring in a string. This is often used to find the position of a character in a string.
The Left function is a built in function that allows you to get the leftmost characters of a string. This is
What are some of the best practices for using built in functions in vb?
When working with built-in functions in Visual Basic, there are a few best practices to keep in mind. First, built-in functions should be used sparingly. Too much reliance on built-in functions can make code difficult to read and understand. Second, always use the most specific built-in function possible. For example, if you need to round a number, use the Round function rather than the Ceiling or Floor functions. This will make your code more readable and maintainable. Finally, be aware of the performance implications of using built-in functions. In general, built-in functions are faster than user-defined functions, but there are some exceptions. For example, the Array function is faster than the equivalent user-defined function. When performance is critical, always test your code with and without built-in functions to see which version is faster.
Are there any other tips or tricks for using built in functions in vb?
Yes, there are definitely other tips and tricks for using built in functions in vb! Here are a few that should help you get more out of them:
1. Use the right function for the job.
There are a lot of built in functions in vb, and they can all be tempting to use for every task. However, using the wrong function for the job can actually make your code more complicated and less efficient. So take a moment to think about which function is best suited for the task at hand before just picking one at random.
2. Be aware of side effects.
Some built in functions can have undesired side effects that you may not be aware of. For instance, the StrReverse function can actually reverse the order of letters in a string, which can cause problems if you're not expecting it. Understanding how each function works will help you avoid these kinds of issues.
3. Use built in functions sparingly.
While built in functions can be very useful, relying on them too heavily can make your code less readable and more difficult to maintain. So try to use them only when absolutely necessary, and consider other alternatives when possible.
following these tips should help you get more out of using built in functions in vb. Just remember to use them wisely and sparingly, and you should be able to avoid any potential problems.
Frequently Asked Questions
What are the built-in functions in Excel?
The main built-in functions in Excel are: The VBA functions have a similar set of features, as do most other programming languages. Functions can be found in the VBA Environment tab ( Insert > Module > Function ). The basic principles are the same, but some details may vary depending on which version of Excel you are using.
How to use built in functions in MS Excel?
To use a built-in function in Excel, we first need to identify which function we want to use. Functions are categorized by topic under the Formulas tab, as shown in the screenshot below. Clicking on the name of the function will display a dialog box with instructions on how to use the function. Once we have found the function we want, we can enter the necessary information into our formula. We can specify input values for our function, or provide an output value. If we want our formula to calculate multiple outputs, we can separate them with commas. We can also specify inputs for other functions that are called from our main formula. For example, if we wanted to sum the numeric values in cells A1 through D5, we could use the addition operator (+) followed by the SUM function, as shown in the following example: =SUM(A1:D5) If you want to undefined a particular range of data
What are the different types of functions in MS Excel?
There are three types of functions in MS Excel: built-in, custom, and VBA.
What is built-in function list in Excel?
This is a list of all the Excel functions that are available to be used in your worksheet. The list is organised into categories (text, logical, math, etc.), which can help you to quickly and easily locate the function you need from the Excel menu.
What is a function in Excel?
An Excel function is a predefined formula in Excel which does calculations in the order specified by its parameters. Functions can be called from other functions, or from your worksheet’s formulas. When you enter a function into Excel, you specify its name, input parameters (on the left side of the function), and return parameter (on the right side of the function). Functions are a valuable tool for automating routine tasks in Excel. For example, you might use a function to calculate sales tax on a sales invoice. You can also use functions to perform complex calculations quickly and easily.
Sources
- https://www.w3computing.com/vb2008/vb-built-in-functions/
- https://docs.microsoft.com/en-us/dotnet/visual-basic/language-reference/functions/
- http://www.universalteacherpublications.com/univ/free-asgn/2008/cs65/page2.htm
- https://www.gcreddy.com/2015/12/vbscript-built-in-functions.html
- http://www.functionx.com/vb6/Lesson09.htm
- https://onlinelibrary.wiley.com/doi/pdf/10.1002/9781119579366.ch9
- https://stackoverflow.com/questions/10283767/executing-build-in-functions-from-vba-excel
- https://social.msdn.microsoft.com/Forums/en-US/876a00ad-6bf7-47e5-ad09-0bdccbcada74/whats-the-benefits-of-using-built-in-function-app-connector-vs-
- http://lavape.sourceforge.net/doc/html/AdvantVB.htm
- https://www.dummies.com/article/technology/software/microsoft-products/excel/advantages-and-disadvantages-of-excel-vba-139800/
- https://stackoverflow.com/questions/10360011/drawbacks-of-combining-c-net-and-vb-net
- https://context.reverso.net/traduction/anglais-francais/Some+of+the+drawbacks
- https://www.quorak.com/qa/question/what-are-some-of-the-drawbacks-of-using-ink-cartridges
- https://www.guru99.com/vb-net-introduction-features.html
- https://datascopic.net/5-against-vba/
- https://www.vbtutor.net/lesson10.html
- https://www.ibm.com/docs/en/SSLTBW_2.2.0/com.ibm.zos.v2r2.cbcpx01/ceelib.htm
- https://idoc.pub/documents/built-in-functions-of-vb-k54609gqv948
- https://www.thevbprogrammer.com/Ch04/04-08-StringFunctions.htm
- http://www.functionx.com/vbasic/Lesson27.htm
- https://www.quora.com/What-are-the-best-practices-when-developing-vb
- https://stackoverflow.com/questions/13021036/best-practice-when-using-multiple-forms-vb-net
- https://stackoverflow.com/questions/33621594/what-is-the-built-in-function-precedence-in-visual-basic-net
- https://www.linguee.fr/anglais-francais/traduction/tips+and+tricks+for+using.html
Featured Images: pexels.com