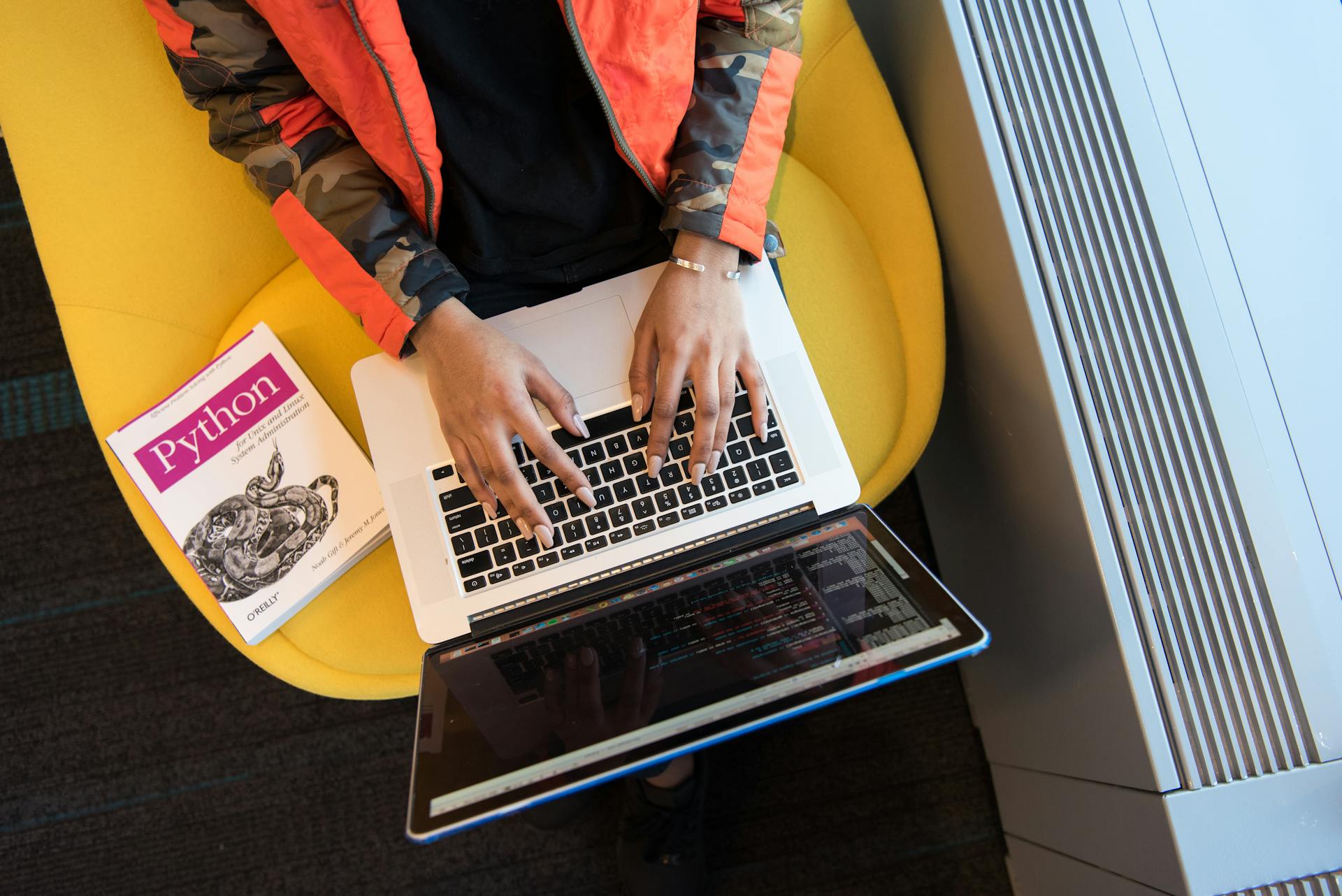
Getting a list of all stock ticker symbols from Yahoo Finance is a crucial step in any stock market analysis or trading project. You can use the yfinance library in Python to achieve this.
The yfinance library provides a function called tickers.info() that returns a dictionary containing information about a specific stock ticker symbol, including its name, industry, and sector. However, we're interested in getting a list of all stock ticker symbols.
To get a list of all stock ticker symbols from Yahoo Finance, you can use the yfinance library's tickers.tickers() function, which returns a list of all available stock ticker symbols. This list includes symbols for stocks, ETFs, and other financial instruments.
For your interest: The Dhandho Investor the Low-risk Value Method to High Returns
Importing Libraries and Data
To get started with importing the necessary libraries, you'll need to install them first. If you haven't installed them, you can copy and paste the required code to get them set up.
The libraries you'll need for this operation are BeautifulSoup, Requests, YahooFinance, and Datetime. Here's a list of the libraries and their purposes:
- BeautifulSoup: to pull data out of HTML files
- Requests: to grab the source code from Wikipedia's page
- YahooFinance: to get stock data
- Datetime: to deal with datetime objects
Once you've installed the libraries, you can import them to start working with your data.
Import the Libraries
To import the necessary libraries for our operation, we need four of them. BeautifulSoup is one of them, which we'll use to pull data out of HTML files.
We'll also need Requests to grab the source code from Wikipedia's page. This is a crucial step in getting the data we need.
Another library we'll need is YahooFinance, which will help us get stock data. This is a key component of our project.
Finally, we'll need Datetime to deal with datetime objects. This will come in handy when working with dates and times.
Here are the libraries we need to import, along with a brief description of what each one does:
- BeautifulSoup: pulls data out of HTML files
- Requests: grabs the source code from Wikipedia's page
- YahooFinance: gets stock data
- Datetime: deals with datetime objects
If you haven't installed these libraries before, you can copy and paste the installation code to get started.
Obtaining Pricing Data
To obtain historical pricing data for the S&P 500 constituents, we need to query the database for the list of all symbols, which will return the symbols along with their symbol IDs.
The next step is to use the Yahoo Finance API to download the historical pricing data for each symbol. This involves calling the API for each symbol individually.
We can optimize this procedure by using the Python ScraPy library, which is built on the event-driven Twisted framework and allows for high concurrency in downloads.
Each download will be carried out sequentially at the moment, but using ScraPy would enable us to download multiple symbols concurrently.
Get S&P 500 Tickers
You can get the S&P 500 tickers from Wikipedia by scraping the table containing the S&P 500 stocks data.
The table is under the class 'wikitable sortable', so you need to specify this to access it.
To extract the tickers, create an empty list and populate it using a for loop that iterates through the table, starting from the second row (since the first row is the header).
For each row, grab the ticker and append it to the list.
Recommended read: S&p 500 P/e Ratio Current
The resulting list will contain all the S&P 500 tickers, but they will be stored with the new line character (
) at the end of each symbol.
To remove this, you can use list comprehension.
Here's a simple example of how to do this:
The list of tickers would look like this: ['MSFT
', 'GOOG
', 'AMZN
']
Frequently Asked Questions
How to pull stock data in Python?
To pull stock data in Python, use the pandas_datareader or yfinance module to retrieve the data, and then store it in a CSV file using pandas' to_csv method. You can easily install yfinance in your Jupyter Notebook with a single line of code.
Sources
- https://www.quantstart.com/articles/Securities-Master-Database-with-MySQL-and-Python/
- https://robin-stocks.readthedocs.io/en/latest/robinhood.html
- https://wire.insiderfinance.io/how-to-get-all-stocks-from-the-s-p500-in-python-fbe5f9cb2b61
- https://quant.stackexchange.com/questions/26162/where-can-i-get-a-list-of-all-yahoo-finance-stocks-symbols
- https://pythonprogramming.net/sp500-company-list-python-programming-for-finance/
Featured Images: pexels.com