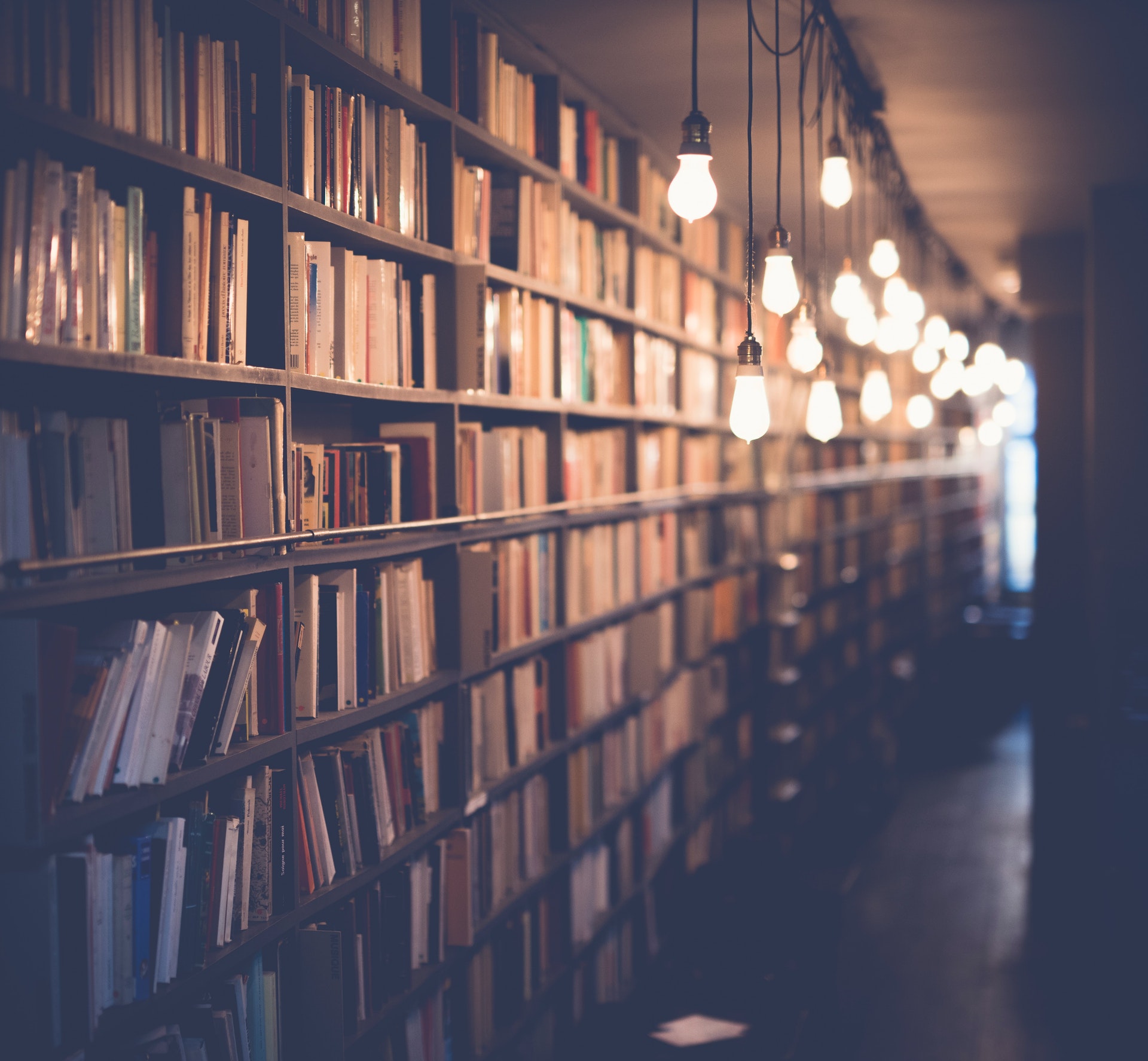
Python offers a library to send emails called smtplib. With this library, you can connect to a mail server and send mail. You will need the email address of the recipient and the server address of the email provider.
To start, let's import the smtplib library.
import smtplib
Now, we need to connect to the server. We will use the gmail mail server.
mail=smtplib.SMTP('smtp.gmail.com',587)
The first parameter is the server address and the second is the port. The port for gmail is 587. Now, we need to start the connection with the server.
mail.ehlo()
mail.starttls()
The ehlo() method is used to identify ourselves to the server. The starttls() method is used to upgrade the connection to a secure connection.
Now, we need to login to the account that will be sending the email.
mail.login('[email protected]','yourpassword')
Replace [email protected] with the email address of the account that will be sending the email. Replace yourpassword with the password for that account.
Now, we can start sending the email. The sendmail() method takes three parameters. The first is the email address of the sender, the second is the email address of the recipient, and the third is the message.
mail.sendmail('[email protected]','[email protected]','Happy birthday!')
Replace [email protected] with the email address of the account that will be sending the email. Replace [email protected] with the email address of the birthday person.
Finally, we need to end the connection with the server.
mail.close()
A unique perspective: Why Is Stamps Com Sending Me a Package?
What is the best way to send birthday wishes email with python?
In order to sending birthday wishes email with python, it is best to use a module called smtplib. This will allow you to connect to an email server and send your message. You will need to have a few things in order before you can use this module. First, you will need to have a working email account that you can access. Next, you will need to know the address of the email server that you will be connecting to. Finally, you will need to know the port number that the server uses for SMTP connections.
Once you have all of this information, you can start writing your Python code. The first step is to import the smtplib module. Next, you will need to create a variable that represents the server that you will be connecting to. This variable will be used later on in the code.
After you have created the server variable, you will need to create a message. This message will be what is sent to the person receiving the birthday wishes email. You will need to use the format string in order to insert the person's name into the message.
Finally, you will need to send the message using the sendmail() function. This function will take three arguments. The first argument is the server that you are connecting to. The second argument is the sender's email address. The third argument is the recipient's email address.
Once you have run this code, the email should be sent to the specified address. The person receiving the email will then be able to read your message.
Here's an interesting read: How to Send a Message to Someone Who Blocked You?
How can I make my birthday wishes email stand out?
When it comes to celebrating a birthday, email can be a great way to share wishes with friends and family. But how can you make your birthday wishes email stand out from the rest?
Here are a few tips:
1. Use an eye-catching template.
There are tons of birthday-themed email templates available online. Choose one that includes a festive design and includes all the information your recipients will need, such as the date, time, and location of the party.
2. Write a personalized message.
Take the time to write a thoughtful, personal message in your email. Your birthday wish should be specific to the recipient and include a gesture or memory that is meaningful to them.
3. Add photos or videos.
Include photos or videos of you celebrating your birthday. This is a great way to add a personal touch to your email and make it more fun for your recipients to read.
4. Send your email early.
Don't wait until the last minute to send your birthday wishes email. Send it a few days in advance so your recipients have time to read it and respond.
5. Follow up.
After you've sent your birthday wishes email, follow up with a phone call or text message. This is a great way to ensure that your message was received and to make sure your birthday wishes are truly memorable.
What are some tips for writing a great birthday wishes email?
When it comes to writing a great birthday wishes email, there are a few things you can do to make sure your message is received with enthusiasm. First, take into account the relationship you have with the birthday boy or girl. A close friend or family member will likely appreciate a more personal message, while a colleague or acquaintance will likely appreciate a more formal one. Second, consider what kind of birthday wish would be most appropriate for the person you are emailing. A funny wish may be appropriate for someone with a good sense of humor, while a more heartfelt wish may be best for someone who is more sentimental. Third, keep your email short and sweet - no one wants to read a long, drawn-out message on their birthday. And finally, make sure to proofread your email before sending it off - there's nothing worse than a birthday wish with a typo in it!
For more insights, see: Why Is Wish Not Working?
How can I make my birthday wishes email more personal?
The simplest answer is to ask the birthday person what he or she would like as a birthday wish. However, if you want to make your birthday wish email more personal, here are some tips:
1. Take the time to write a heartfelt message. A few sentences about what the birthday person means to you and why you are excited to celebrate his or her birthday will make the email feel more personal.
2. Share a memory. Whether it is something funny that happened when you were together or a time when the birthday person was there for you, a shared memory will make your email feel more personal.
3. Express your excitement for the upcoming birthday. Use enthusiastic language to let the birthday person know that you are excited to celebrate his or her special day.
4. Include a photo. A picture of you together, or even just a recent photo of the birthday person, will make your email more personal.
5. Use the birthday person's name throughout the email. Addressing the birthday person by name will make your email feel more personal than if you simply said "Happy birthday!" at the beginning and end.
By following these tips, you can write a birthday wish email that is more personal and heartfelt, and that will make the birthday person feel special on his or her big day.
Additional reading: When You Wish Events?
What are some common mistakes people make when sending birthday wishes email with python?
When sending birthday wishes email with python, some common mistakes people make include forgetting to import the email library, sending the email to the wrong address, and forgetting to attach the birthday card.
The email library is important because it contains the functions needed to send an email. Without it, the email cannot be sent. The most common mistake is forgetting to import the email library.
The next mistake is sending the email to the wrong address. This can happen if the person sending the email does not have the correct email address for the recipient. Another way this can happen is if the email address is misspelled.
The last mistake is forgetting to attach the birthday card. This can be a big problem because the recipient will not be able to see the birthday card. This mistake is usually made because the person sending the email forgets to click on the "attach" button.
A fresh viewpoint: Email Address for Discover Card
How can I avoid making mistakes when sending birthday wishes email with python?
Python is a powerful programming language that can be used for a wide range of tasks. One of these tasks is sending birthday wishes via email. While this may seem like a simple task, there are a few potential mistakes that can be made which can impact the success of your email.
One potential mistake is forgetting to add attachments. If you are sending a birthday wish via email, it is likely that you will want to include a card or otherbirthday-related attachment. If you forget to add this attachment, your email may seem incomplete or lacking in thoughtfulness.
Another potential mistake is forgetting to proofread your email before sending it. Even if you write a great birthday message, there may be typos or other errors that can make your message difficult to read. Taking the time to proofread your email before sending it can help ensure that your birthday wish comes across clearly.
Finally, you may also want to consider the format of your email. If you are sending a birthday wish to someone who does not use Python, they may not be able to read your email if it is written entirely in Python code. Including a brief message in plain text at the beginning of your email can help ensure that your birthday wish is received and understood.
Sending a birthday wish via email can be a great way to show someone you care. By taking the time to write a thoughtful message and avoid potential mistakes, you can ensure that your birthday wish is received and enjoyed.
What are some other things I can do to make my birthday wishes email more special?
There are many ways that you can make your birthday wishes email special. One way is to include thoughtful and personal messages for the birthday person. You can also include fun graphics or photos to make the email stand out. Additionally, you can include a link to a special birthday message or song. Finally, you can send the email to multiple people to spread the birthday cheer.
What are some common questions people have about sending birthday wishes email with python?
People often ask about sending birthday wishes via email using Python. There are a few things to keep in mind when doing this. First, make sure that you have the recipient's email addresses handy. It is also a good idea to check with the person beforehand to see if they would like to receive birthday wishes via email. Second, decide what message you would like to include in the email. You can either write your own message or use a pre-written message. If you choose to write your own message, be sure to keep it short and sweet. Third, select an appropriate email template. Some email templates are designed for birthday wishes, while others are more general. Fourth, include a link to a website where the recipient can find more information about Python. Finally, send your email and wait for a response.
Additional reading: How to Create Your Own Email Account
How can I make sure my birthday wishes email is received and read?
There are a few things you can do to make sure your birthday wishes email is received and read. First, avoid using any email addresses that are known to be overburdened or have a history of not delivering messages. Second, use a reliable email service such as Gmail or Yahoo, and include a personal message in the body of the email. Finally, send your birthday wishes email a day or two in advance to ensure it arrives on time.
Take a look at this: Birthday Wishes
Frequently Asked Questions
How do I send a birthday message to my subscribers?
Step 1: Firstly, open up Mailchimp and sign in. Step 2: Click on the Subscribers tab located at the top of your screen. Step 3: On the Subscribers tab, you will see all of your subscribers listed. Find the contact you want to send a birthday message to and click on their name. Step 4: Underneath their name, you will find a section titled "Birthday Messages." Select the appropriate option from the drop-down menu and enter your wish into the text field provided. Click Ket to save your changes. Tip: You can also add a custom promotional offer or expiration date to your birthday message if you wish. Simply click on the "Create Custom Message" button and enter in your information accordingly.
How to sort birthdays in Excel?
Sorting birthdays in Excel is an easy task if you know how to use the sorting features of Excel. The following steps will help you to achieve the desired result: 1. Open the document containing your data. 2. Increase the size of your column containing the dates so that they can be sorted easily. 3. Select your column and click on the Sort button situated at the bottom left corner of the Data window. 4. In the Sort A to Z dialog box, specify Month and Day as the sorting criteria and click on theSort button. Your data will be sorted in reverse chronological order according to day and month.
How to calculate the number of days until the birthday in Excel?
In order to calculate the number of days until the birthday in Excel, we first need to know the month and year. Next, use the TODAY() function to get the current date. Finally, use the DATEDIF function with "ym" as its parameter to calculate how many months and how many days remain until the birthday.
How to enter the birthday of your staff in Excel?
The following formula can help you enter the birthday of your staff in Excel. =IF (MONTH (B2)<>MONTH (TODAY ())," ",IF (DAY (B2)<>DAY (TODAY())," ","Happy Birthday"))
How to highlight the birthday dates of today in Excel?
1. Select all the dates in column B. 2. Then click Home > Conditional Formatting > New Rule, see screenshot: 3. In the Rule Type drop-down list, select Highlight Cells. 4. Under Highlights, select Birthday Dates, see screenshot:
Sources
- https://www.sendinblue.com/blog/birthday-email-guide/
- https://www.mailcharts.com/email-examples/birthdays
- https://www.geeksforgeeks.org/automatic-birthday-mail-sending-with-python/
- https://www.noobie.com/how-to-make-your-happy-birthday-wishes-really-stand-out/
- https://coderspacket.com/python-automated-birthday-email-sender-using-pandas-library
- https://blog.hubspot.com/marketing/happy-birthday-email
- https://www.pythonforbeginner.com/2022/09/how-to-send-birthday-wishes-email-with.html
- https://pythonscholar.com/blog/how-to-send-birthday-wishes-email-with-python/
- https://www.constantcontact.com/blog/how-to-automated-birthday-emails/
- https://www.birthdaywishes.expert/birthday-wishes/birthday-messages/
- https://appwrk.com/how-to-send-birthday-wishes-email-with-python
- https://pythonguides.com/python-program-for-birthday-wishes/
- https://mytemplets.com/happy-birthday-wishes-email-example/
- https://www.birthdaywishes.expert/birthday-wishes/clever-birthday-wishes-to-make-your-greetings-stand-out/
Featured Images: pexels.com