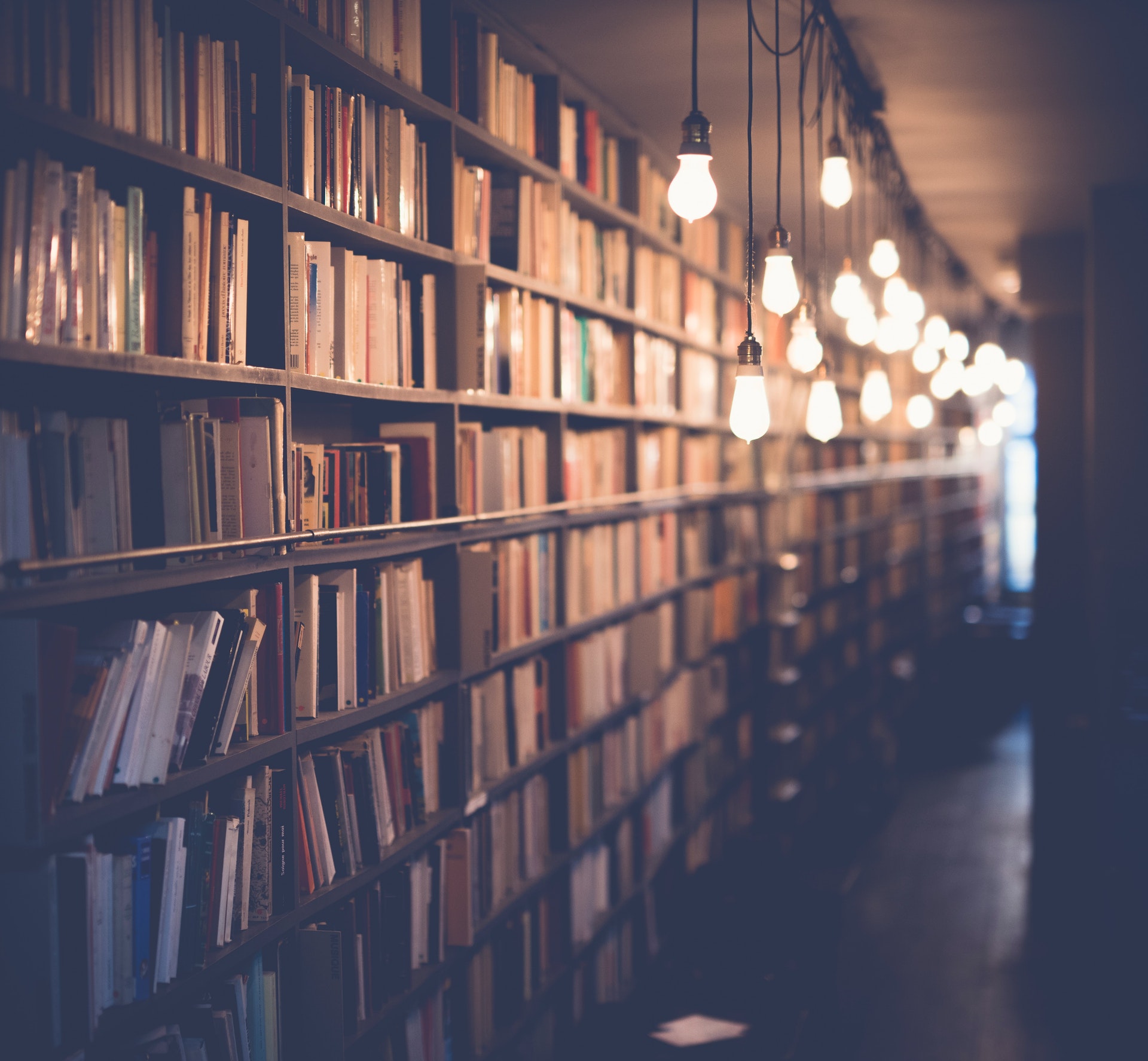
It is inevitable that when using the Inspect tool in a web browser, the values of certain HTML tags will be changed. While this may be desirable in some cases, there are also times when it is not. In order to prevent this from happening, there are a few steps that can be taken.
The first step is to simply avoid using the Inspect tool when working with values that should not be changed. This may seem like an obvious solution, but it is often overlooked. If the Inspect tool is not being used, then there is no risk of accidentally altering values.
Another solution is to use the Inspect tool in a read-only mode. This can be done by selecting the "Disable" button in the bottom-right corner of the Inspect window. This will prevent any changes from being made, even if the values are accidentally modified.
Finally, if the Inspect tool must be used, be very careful when making any changes. Always double-check the values before saving them. If possible, it is best to make a backup of the original values before making any changes. This way, if something does go wrong, it can be easily fixed.
Suggestion: Prevent Plantar Fasciitis
How do I prevent inspect from changing the value of my select tag?
It is a best practice to always declare your variables before using them, and to avoid using global variables. The following code will create a private variable inside the function, and will not change the value of the select tag:
var mySelect = document.getElementById('mySelect');
function preventInspectFromChangingSelectTag() { var selectTag = mySelect.options[mySelect.selectedIndex].value;
// Do something with selectTag
return selectTag; }
Executing the function will not change the value of the select tag.
Discover more: Carrier Pre Select
What is the best way to keep inspect from altering my select tag's value?
The best way to keep Inspect from altering your select tag's value is to use the disabled attribute.
The disabled attribute is a boolean attribute that, if present, will prevent the user from interacting with the element. The element will still be rendered, but the user will not be able to interact with it.
If you want to keep the user from changing the value of a select tag, you would use the disabled attribute like this:
One Two Three
If you want to keep the user from submitting a form that contains a select tag with a disabled attribute, you would use the form's submission event like this:
form.addEventListener('submit', function(event) { if (form.querySelector('select[disabled]')) { event.preventDefault(); } });
The disabled attribute is useful for keeping the user from changing the value of a select tag, but it doesn't provide any visual feedback that the element is disabled. If you want to provide users with a visual indication that an element is disabled, you can use the CSS property opacity.
.disabled { opacity: 0.5; }
The CSS property opacity is a number between 0 and 1 that specifies the opacity of an element. An element with an opacity of 0 is completely transparent, and an element with an opacity of 1 is completely opaque.
If you want to provide users with a visual indication that a select tag is disabled, you would use the CSS property opacity like this:
select[disabled] { opacity: 0.5; }
The disabled attribute is the best way to keep Inspect from altering your select tag's value. It will prevent the user from interacting with the element, and if you use the CSS property opacity, it will provide a visual indication that the element is disabled.
A different take: The Fed Can Change the Money Supply by Changing
Is there a way to keep inspect from automatically changing the value of select tags?
Yes, there is a way to keep inspect from automatically changing the values of select tags. You can do this by using the "disable triggers" option in the settings menu. This will prevent inspect from automatically changing the values of select tags, but you will still be able to manually change them if you need to.
A different take: Changing Money in Mexico
How can I stop inspect from automatically changing the value of select tags?
There are a few different ways that you can stop inspect from automatically changing the value of select tags. One way is to simply disable inspect from changing any values by going into the settings and disabling the option. Another way is to change the way that inspect works so that it only changes the value of select tags when you specifically tell it to. To do this, you can either add an "unless" statement to your code or change the default value for the "change" function in inspect.
Expand your knowledge: Delta Premium Select
How do I keep inspect from automatically updating the value of select tags?
When creating forms, one may want to prevent the browser from automatically updating the value of select tags. This can be done by specifying the autocomplete attribute on the form element with a value of "off". This will tell the browser not to update the value of select tags when the form is submitted.
There are other ways to prevent the browser from automatically updating the value of select tags. One way is to set the value of the form element's readonly attribute to "readonly". This will tell the browser not to update the value of select tags when the form is submitted.
Another way to prevent the browser from automatically updating the value of select tags is to set the form element's disabled attribute to "disabled". This will tell the browser not to update the value of select tags when the form is submitted.
The most effective way to prevent the browser from automatically updating the value of select tags is to use the autocomplete attribute on the form element with a value of "off". This will tell the browser not to update the value of select tags when the form is submitted.
Recommended read: Prevent Neighbors
What is the best way to keep inspect from automatically updating the value of select tags?
There are a number of ways to keep inspect from automatically updating the value of select tags. One way is to use the autocomplete="off" attribute on the select tag. This will prevent the browser from automatically updating the value of the select tag based on what is typed into the input field. Another way is to use the readonly attribute on the select tag. This will prevent the user from being able to type into the input field, but will still allow them to select a value from the drop-down list. Finally, you can use JavaScript to disable the input field. This will prevent the user from being able to type into the field, but will still allow them to select a value from the drop-down list.
Is there a way to keep inspect from automatically updating the value of my select tag?
This is a difficult question to answer definitively because it depends on a number of factors, including the specific implementation of Inspect and the structure of the HTML select tag. However, in general, it is possible to prevent Inspect from automatically updating the value of a select tag by using a 'readonly' or 'disabled' attribute on the tag.
When an HTML element is disabled, it cannot be updated by Inspect. The value of the disabled element will remain the same as when the element was first disabled. If the select tag is disabled, then Inspect will be unable to update the value of the tag.
It is also possible to make an HTML element read-only. A read-only element can be updated by Inspect, but the value of the element will not be changed when the user interacts with the page. This means that if the value of the select tag is set to 'readonly', Inspect will not be able to update the value of the tag.
In order to keep Inspect from automatically updating the value of a select tag, the best approach is to use a combination of the 'readonly' and 'disabled' attributes. This will ensure that the value of the tag cannot be changed by Inspect, even if the user tries to interact with the page.
How can I stop inspect from automatically updating the value of my select tag?
It's easy to disable automatic select tag value updates in Inspect. Simply right-click on the element in the HTML pane and select "Disable auto-update". This will prevent Inspect from automatically updating the value of your select tag, allowing you to manually update it as needed.
How do I keep inspect from automatically changing the value of my select tag when I inspect it?
If you're like most people, you probably use the browser's inspection tool (or "inspector") to peek at the HTML code behind a website. The inspector is a handy tool that lets you see how a webpage is put together, and it can be very useful for web developers and designers.
However, the inspector can also be a little bit annoying, because it can sometimes automatically change the value of your code when you inspect it. For example, if you have a element on your page, and you inspect it in the inspector, the inspector will often change the value of the element to "1".
The reason the inspector does this is because the inspector is trying to be helpful - it assumes that you want to see the value of the element, so it changes the value to "1" so that you can see it. But of course, this is not always what you want, and it can be very frustrating.
Fortunately, there is a way to prevent the inspector from automatically changing the value of your code. You can do this by adding a special attribute to the element called "data-inspector-value".
When you add this attribute to a element, the inspector will no longer automatically change the value of the element when you inspect it. Instead, the inspector will simply show the value that is already set in the "data-inspector-value" attribute.
This can be very useful if you want to make sure that the inspector doesn't accidentally change the value of your code. It can also be handy if you want to Inspect an element on a page but don't want to actually change the value of the element.
One thing to keep in mind is that the "data-inspector-value" attribute will only work if it is added to the element before the page is loaded. That means you'll need to add the attribute to the element in the HTML code, or else you'll need to use JavaScript to add the attribute after the page has been loaded.
Frequently Asked Questions
How to save changes done with Inspect Element?
Simply copy/paste the edited code into a text editor of your preference, and hit "save".
Can I inspect an element on the page and change its attribute?
Yes, in Chrome Developer Toolbar as well as Firefox and IE/Edge, you can inspect an element on the page and temporarily change its attribute in the markup displayed in the element tree.
How to change titles and images in Inspect Element?
1. In Inspect Element, open the Console tab. 2. Edit any element on your webpage. This includes titles and images. 3. Test different combinations of titles and images to see what looks best on your page.
What can I do with Inspect Element?
With Inspect Element, you can change any text on a webpage in a second. This is a handy tool if you need to quickly show developers what needs fixed on a site. Additionally, Inspect Element can be used to help agents better communicate with developers and to test changes before they go live.
How do I Live Edit a page using in Inspect Element?
In Inspect Element, click the "Live Edit" button in the top right corner of the window. In the live edit panel that opens, you can see a page preview and live editing buttons for the html and css files on the https server. If you want to make a change to an html or css file on a non-https server, use the link to Open in a New Tab or Window instead. To make a change to an html or css file on a https server, first locate the file(s) you want to edit using the Recent Files list at the top of the live edit panel. Then find and select the file you want to make your changes to. The highlighted area below shows where the chosen file will be opened in your web browser. Make your changes to the file as you would any other text document. When you're finished making your changes, save your edited file and close it by clicking on the "Ok" button.
Sources
- https://grabthiscode.com/javascript/javascript-prevent-value-change-in-select-option
- https://stackoverflow.com/questions/50356757/how-to-prevent-user-from-changing-the-value-of-a-select-without-using-disabled
- https://www.codeproject.com/questions/1032664/how-to-prevent-user-to-change-html-textbox-value-u
- https://www.quora.com/How-do-I-select-an-element-if-the-inspect-element-value-keeps-on-changing-for-Selenium-WebDriver
- https://learn.microsoft.com/en-us/microsoft-edge/devtools-guide-chromium/css/inspect
- https://www.quora.com/How-do-I-stop-people-changing-values-on-my-web-using-inspect-element
- https://www.quora.com/How-do-I-disable-the-inspect-element-in-all-browsers-from-an-administrator-that-the-local-user-cant-use-to-inspect-elements
- https://stackoverflow.com/questions/41905529/how-do-i-prevent-users-from-changing-form-values-or-removing-input-fields-elemen
- https://security.stackexchange.com/questions/88779/how-to-prevent-user-from-removing-disabled-attribute-and-manually-editing-role
- https://www.appsloveworld.com/bestanswer/jquery/442/how-to-prevent-user-from-altering-the-id-on-inspect-element-in-chrome
- https://www.folkstalk.com/2022/09/how-to-prevent-user-from-inspect-element-with-code-examples.html
- https://stackoverflow.com/questions/37716352/how-to-add-id-into-options-for-select-on-select-tag-rails
- https://www.youtube.com/watch
- https://stackoverflow.com/questions/40477397/is-it-possible-to-keep-inspect-mode-always-on-in-dev-tools
Featured Images: pexels.com