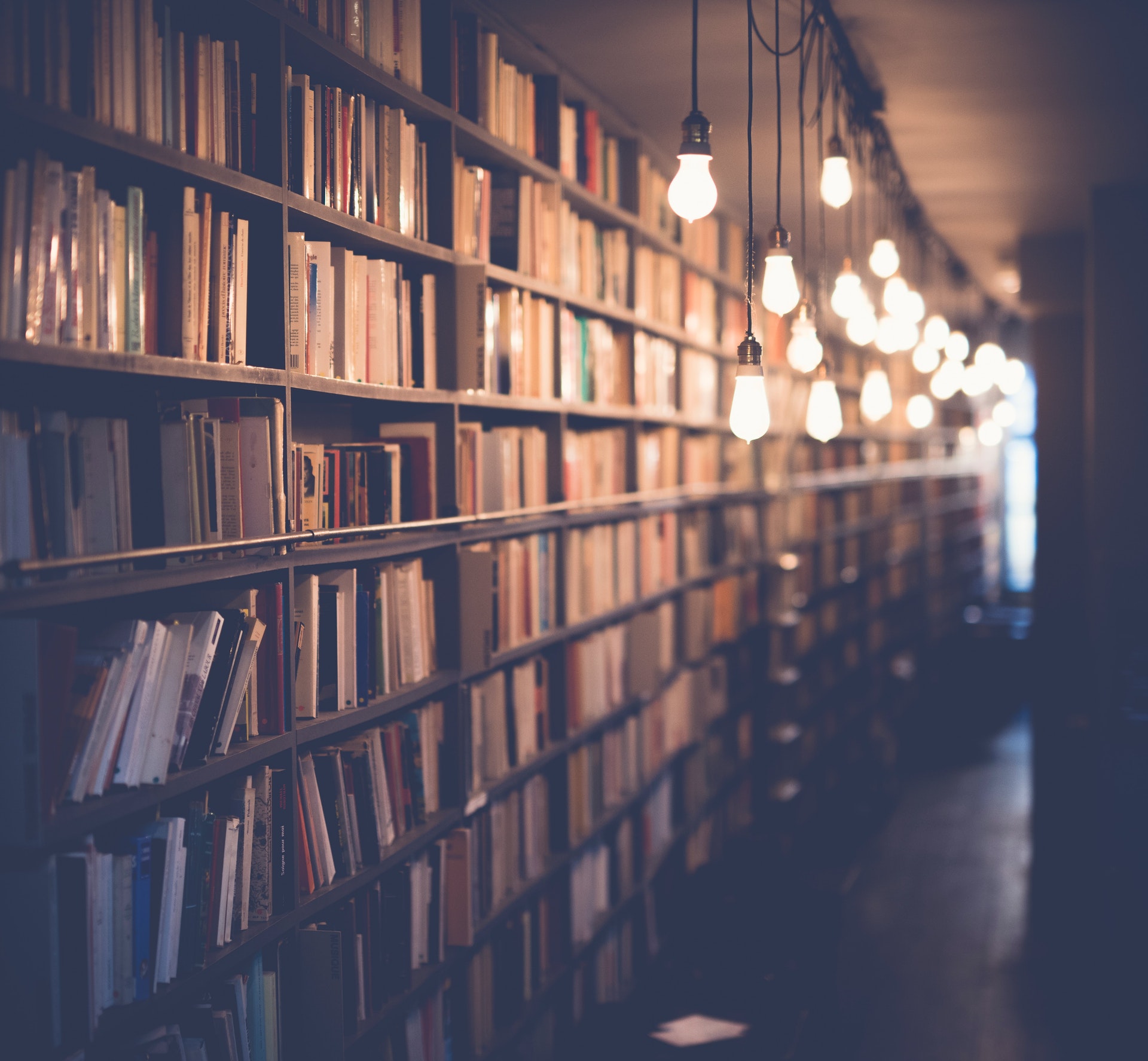
In order to select elements with a class name of "test", there are a few different methods that can be used. The first is to use the getElementsByClassName method, which is available on all major browsers. This method returns a live HTMLCollection of all elements with the specified class name.
Once you have the HTMLCollection, you can access any element within it by using array notation. For example, if the first element in the collection has a class name of "test", you would access it like this:
var firstEl = myCollection[0];
Another way to select elements with a class name of "test" is to use querySelectorAll. This method is available on all major browsers except IE8 and below. It works by returning a static NodeList of all elements that match the given CSS selector.
For example, to get all elements with a class name of "test", you would use the following CSS selector:
var myNodeList = document.querySelectorAll('.test');
Once you have the NodeList, you can access any element within it by using array notation. For example, if the first element in the NodeList has a class name of "test", you would access it like this:
var firstEl = myNodeList[0];
One final way to select elements with a class name of "test" is to use the className property. This property returns a string of all the class names of the element. You can then use the indexOf method to check if "test" is in the string.
For example, to get all elements with a class name of "test", you would use the following code:
var myElements = document.getElementsByTagName('*');
for (var i = 0; i -1) { // Element has a class name of "test" } }
There are a few different ways to select elements with a class name of "test". The most common methods are to use the getElementsByClassName method or the querySelectorAll method. You can also use the className property and the indexOf method to check if an element has the class name
You might like: Carrier Pre Select
How do you select elements with multiple class names?
Assuming you are using a CSS selector engine such as jQuery, the process for selecting elements with multiple class names is relatively straightforward. Simply provide the class names as a space-separated list when using the .className selector.
For example, given the following HTML:
Lorem ipsum
You could select this element using:
$('.foo.bar')
Or, if you had multiple elements with the same class names:
$('div.foo.bar')
Once you have a reference to the element (or elements) you can manipulate them as you please using the various jQuery methods.
See what others are reading: Initialize Class Orgcodehausgroovyvmpluginv7java7
Frequently Asked Questions
How do I select elements with a specific class?
.fooClass How do I select all elements with a specified HTML tag?
How do you use the Class Selector in HTML?
The following code selects all paragraphs that have the teachers class attribute: .teachers You can also target specific HTML elements by using the .include selector, which is similar to the Class Selector. The following code selects the input field with the id "name" and sets the text-area class to "myclass": .input_field { border: 1px solid #333; } .myclass input { width: 450px; height: 30px; }
How to get element through class name in CSS?
.activity { ... } /* Pass activity class */ By.css("activity", ".activities") /* Or use a selector */ .activity { ... }
How do you select elements with a class in HTML?
To select elements with a class in HTML, use the .class selector. This can be done either by writing the period character followed by the name of the class (.), or by using the class keyword followed by a space and the complete name of the class: .widget { } .box { }
How do I use the Class Selector?
To use the Class Selector, simply type a period (.), followed by the class name.
Sources
- https://bobbyhadz.com/blog/javascript-get-elements-by-multiple-class-names
- https://wonderdevelop.com/how-do-you-select-elements-with-class-name-test/
- https://mcqsbucket.com/mcq/how-do-you-select-elements-with-class-name-test
- https://a1meatsupply.com/how-do-you-select-elements-with-class-name-test/
- https://yamsoti.com/quiz/select-elements-class-name-test/
- https://stackoverflow.com/questions/1041344/how-can-i-select-an-element-with-multiple-classes-in-jquery
- https://www.slightbook.com/Subjects/Web-Development/CSS/Multiple-Choices/How-do-you-select-elements-with-class-name-test
- https://jeroenwiersma.com/javascript-for-optimizers-25-how-to-select-an-element-with-multiple-classes/
- https://brainly.in/question/3005552
- https://teamtreehouse.com/library/javascript-and-the-dom-3/select-elements-by-class-name
- https://www.javascripttutorial.net/dom/selecting/selecting-elements-by-class-name/
- https://stackoverflow.com/questions/58700051/select-element-with-class-name-that-starts-with-using-cypress
Featured Images: pexels.com