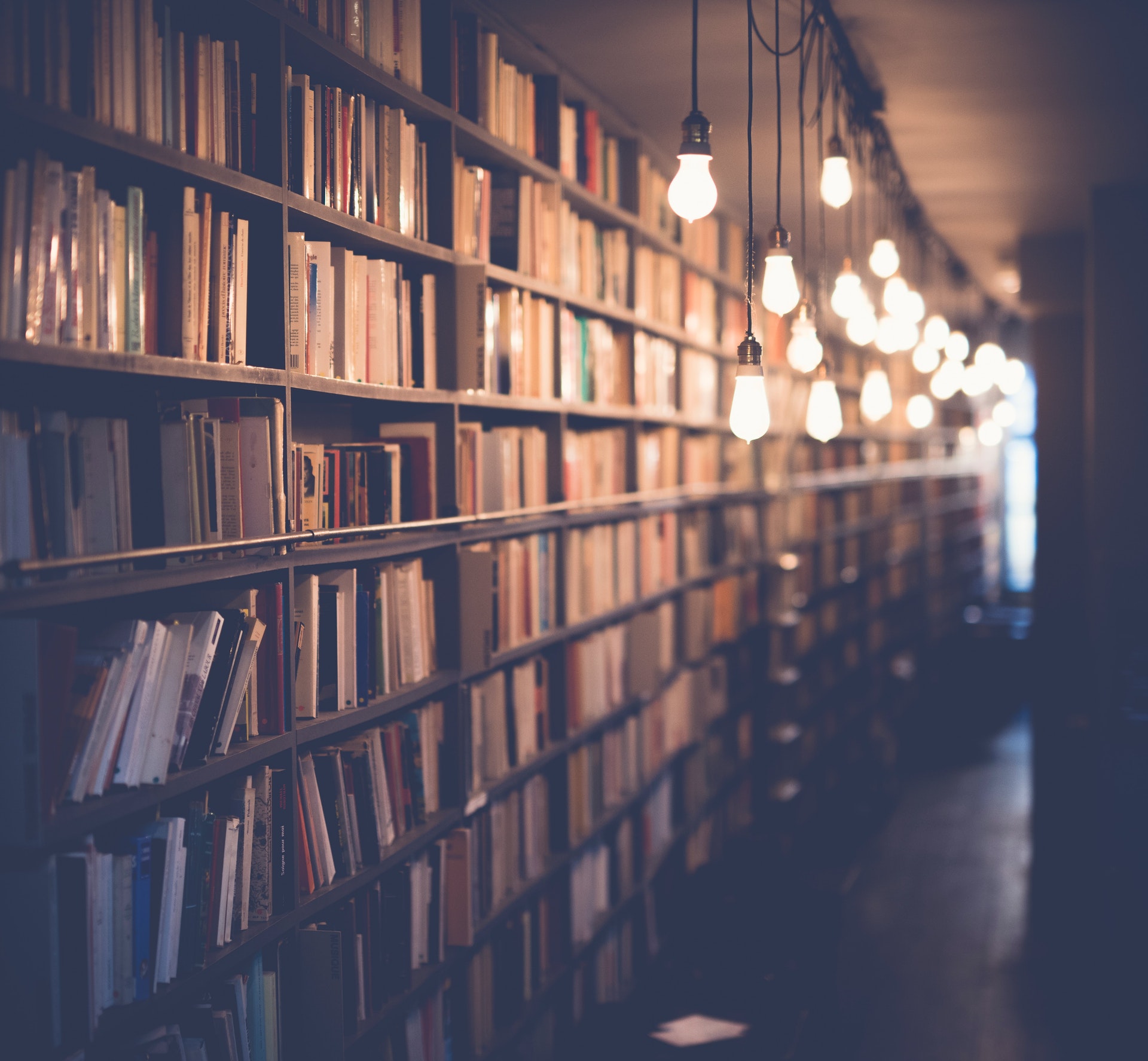
Users retrieve data stored in a database by issuing SQL statements. The SQL statements are processed by the database server, which accesses the data in the database and returns the results to the user.
The user can issue SQL statements to select, insert, update, and delete data in the database. The user can also issue SQL statements to create and drop tables and indexes, and to grant and revoke privileges on the database.
The user can issue SQL statements to select data from the database. The data is retrieved from the database and returned to the user. The user can issue SQL statements to insert data into the database. The data is inserted into the database and the user is notified of the success or failure of the operation.
The user can issue SQL statements to update data in the database. The data is updated in the database and the user is notified of the success or failure of the operation. The user can issue SQL statements to delete data from the database. The data is deleted from the database and the user is notified of the success or failure of the operation.
A unique perspective: Nen User
How do users access data in a database?
Databases are organized collections of data that can be accessed by computers. The data in a database can be accessed in many different ways.
One way to access data in a database is to use a query language. Query languages allow users to specify what data they want to retrieve from the database. Query languages are typically used by programmers and database administrators.
Another way to access data in a database is to use a programming language. Programming languages allow developers to write code that interacts with the database. Developers can write code to retrieve data from the database and to update the data in the database.
Databases can also be accessed using web-based application interfaces. These interfaces allow users to access the database using a web browser. Web-based application interfaces typically provide a simplified interface for users.
Finally, databases can be accessed using file-based interfaces. File-based interfaces allow users to access the database using a file system. File-based interfaces typically provide a more flexible way to access the data in a database.
Intriguing read: Can I Use Virtual Visa Card in Store
How do users query data in a database?
Users query data in a database by issuing SQL commands. SQL, or Structured Query Language, is a standard language for accessing and manipulating databases. SQL commands can be used to perform various operations on a database, such as retrieving data, inserting data, updating data, and deleting data.
There are four main types of SQL commands:
1. Data retrieval commands: these commands are used to retrieve data from a database. The most commonly used data retrieval command is the SELECT command. The SELECT command allows users to specify the columns of data that they want to retrieve, as well as the conditions under which the data should be retrieved. For example, a user could use the following SELECT command to retrieve all of the rows from a table that satisfy a certain condition:
SELECT * FROM table_name WHERE condition
2. Data insertion commands: these commands are used to insert data into a database. The most commonly used data insertion command is the INSERT command. The INSERT command allows users to specify the columns into which data should be inserted, as well as the values to be inserted. For example, a user could use the following INSERT command to insert a new row into a table:
INSERT INTO table_name (column1, column2, column3) VALUES (value1, value2, value3)
3. Data update commands: these commands are used to update data in a database. The most commonly used data update command is the UPDATE command. The UPDATE command allows users to specify the columns of data to be updated, as well as the new values for those columns. For example, a user could use the following UPDATE command to update a row in a table:
UPDATE table_name SET column1 = value1, column2 = value2, column3 = value3 WHERE condition
4. Data delete commands: these commands are used to delete data from a database. The most commonly used data delete command is the DELETE command. The DELETE command allows users to specify the conditions under which data should be deleted. For example, a user could use the following DELETE command to delete all of the rows from a table that satisfy a certain condition:
DELETE FROM table_name WHERE condition
Suggestion: Store Table Leaves
How do users update data in a database?
When a user wants to update data in a database, they first need to open the database. The user then needs to find the data they want to update. To update the data, the user needs to enter new information into the appropriate fields. The user can then save the updated data by clicking on the save button.
How do users delete data in a database?
There are a few ways users can delete data in a database. One way is to use the DELETE statement. This statement Deleting Data from a Database removes data from a database. It can delete one row at a time, or many rows at once. To delete all rows in a table, use the TRUNCATE TABLE statement. This statement can delete all rows from a table, or just those that match a condition. Another way to delete data is to use the DROP statement. This statement removes an entire table from a database. Be careful when using this statement, as it cannot be undone.
Here's an interesting read: Which Statement Is Supported by the Data in the Graph?
How do users create new databases?
Database creation is a process that started as manual entry into tables and has evolved into sophisticated tools that allow easy and rapid creation of databases. The process of creating a new database generally consists of the following steps:
Identifying the purpose of the database: What type of information will be stored in the database? What are the relationships between the different pieces of information?
Designing the structure of the database: How will the information be organized in the database? How will the different pieces of information be linked together?
Creating the database: This step involves actually creating the database using a database management system (DBMS).
Populating the database: This step involves adding the actual data to be stored in the database.
Testing the database: This step involves testing the database to ensure that it works as expected and that the data is accessible.
Making the database available to users: This final step involves making the database available to the users who will be accessing it.
Here's an interesting read: Vulnerable Road Users
How do users create new tables in a database?
Database tables are the foundation of any database and thus it is important for users to know how to create new tables. There are various ways to create new tables in a database, which vary depending on the database management system (DBMS) being used. In this essay, we will discuss how to create new tables in a database using the two most popular DBMSs: MySQL and Microsoft SQL Server.
MySQL is a free and open source relational database management system (RDBMS). It is one of the most popular DBMSs in use today and is supported by a large community of users. To create a new table in MySQL, the user must have the CREATE TABLE privilege. The syntax for creating a new table is as follows:
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
...
);
where table_name is the name of the new table, column1 and column2 are the names of the columns, and datatype is the data type of the column. The data type can be one of the following:
INTEGER
DECIMAL
CHAR
VARCHAR
DATE
TIME
DATETIME
BINARY
BLOB
For example, to create a table named "employees" with three columns (employee_id, name, and hire_date) of type INTEGER, VARCHAR, and DATE, respectively, the following SQL statement can be used:
CREATE TABLE employees (
employee_id INTEGER,
name VARCHAR,
hire_date DATE
);
Microsoft SQL Server is a relational database management system (RDBMS) developed by Microsoft. It is a commercial product and is not available for free. To create a new table in SQL Server, the user must have the CREATE TABLE permission. The syntax for creating a new table is as follows:
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
...
);
where table_name is the name of the new table, column1 and column2 are the names of the columns, and datatype is the data type of the column. The data type can be one of the following
Take a look at this: Which of the following Is an Example of a Database?
How do users add data to a database?
There are many ways to add data to a database. The most common way is to use an INSERT statement. This statement adds one or more records to a table.
Here is the syntax for an INSERT statement:
INSERT INTO table_name (column1, column2, column3, ...) VALUES (value1, value2, value3, ...);
The table_name is the name of the table where you want to add the data. The column names are the names of the columns in which you want to store the data. The values are the data you want to store.
Here is an example:
INSERT INTO customers (first_name, last_name, email) VALUES ('John', 'Doe', '[email protected]');
This statement adds a new row to the customers table. The first_name column is populated with the value 'John', the last_name column is populated with the value 'Doe', and the email column is populated with the value '[email protected]'.
You can also use the INSERT statement to insert multiple rows at a time. To do this, you use the following syntax:
INSERT INTO table_name (column1, column2, column3, ...) VALUES (value1, value2, value3, ...), (value4, value5, value6, ...), (value7, value8, value9, ...), ...;
For example:
INSERT INTO customers (first_name, last_name, email) VALUES ('John', 'Doe', '[email protected]'), ('Jane', 'Smith', '[email protected]'), ('Joe', 'Bloggs', '[email protected]');
This statement inserts three rows into the customers table.
Another way to add data to a database is to use the COPY command. This command copies data from a file into a table. The syntax for the COPY command is as follows:
COPY table_name FROM 'file_name';
The table_name is the name of the table where you want to copy the data. The file_
How do users modify data in a database?
Most databases allow users to modify data in a number of ways. The most common method is through an update query, which can change one or more pieces of information in a database table. To change data in a database, users typically need UPDATE privileges.
Another way to modify data in a database is to use the INSERT command. This command allows users to add new rows of data to a database table. To use the INSERT command, users typically need INSERT privileges.
Finally, users can also modify data in a database by using the DELETE command. This command allows users to remove rows of data from a database table. To use the DELETE command, users typically need DELETE privileges.
How do users remove data from a database?
Most databases allow users to delete data in specific ways. In order to delete data from a database, the user must first select the data they want to delete. The user can then use the delete command to remove the data.
Frequently Asked Questions
How to retrieve data from a database?
The simplest way to retrieve data from a database is to use the SELECT statement. This statement allows you to specify a subset of all the records in the database, or to select specific records based on their attributes. For example, the following SELECT statement will return all of the records in the salesdb database that have a price greater than 200: SELECT price FROM salesdb WHERE price > 200;
What is data retrieval?
Data retrieval is the process of identifying and extracting data from a database, based on a query provided by the user or application. It enables the fetching of data from a database in order to display it on a monitor and/or use within an application.
How is data stored inside a database?
Inside a database, data is stored into tables. A table is a collection of data that has been organized in a specific way. Tables are similar to folders in a file system. Each table contains information about one or more objects. Each row (or record) in a table is associated with one object. The columns in a table define the properties of the object. Each column stores data about the object in question, and each row refers to an instance of that object.
How do developers create data in a database?
In general, developers use a variety of techniques to create data in a database. One common technique is to use SQL commands to insert data into the database. For example, suppose you want to create a new row in the car table. You could use the SQL command INSERT INTO car (id, make, model) VALUES (1, “Toyota”, 1), (2, “Ford”, 2), (3, “Chevrolet”, 3).
How to retrieve data from a database with SQL?
You can retrieve data from a database either programatically or via a user interface. The way to retrieve data from your database with SQL is to use the SELECT statement. Using the SELECT statement, you can retrieve all records that match a certain condition. For example, you might use the SELECT statement to select all customer names in your database.
Sources
- https://stackoverflow.com/questions/18866881/how-to-get-the-list-of-all-database-users
- https://getanyanswer.net/how-do-users-retrieve-data-stored-in-a-database/
- https://www.indeed.com/career-advice/career-development/query-database
- https://quizlet.com/201126142/ais-exam-3-flash-cards/
- https://www.indeed.com/career-advice/career-development/what-is-database-query
- https://www.thinkautomation.com/features/sql-command-to-update-data-in-a-database/
- https://stackoverflow.com/questions/47596366/using-stored-procedure-to-retrieve-data-from-database
- https://www.chegg.com/homework-help/questions-and-answers/users-retrieve-data-stored-database-select-one--viewing-appropriate-data-tables-b-executin-q29611463
- https://www.simplilearn.com/tutorials/sql-tutorial/sql-update
- https://quizlet.com/206697570/chapter-4-exam-flash-cards/
- https://www.coursehero.com/file/p75o4jm/How-do-users-retrieve-data-stored-in-a-database-A-by-viewing-the-appropriate/
- https://satoricyber.com/access-control/access-control-101-a-comprehensive-guide-to-database-access-control/
- https://www.quackit.com/database/tutorial/querying_a_database.cfm
- https://openquery.com/what-is-a-query-in-database/
- https://blog.sqlbak.com/learn-sql-2-how-is-data-stored-in-a-database
Featured Images: pexels.com