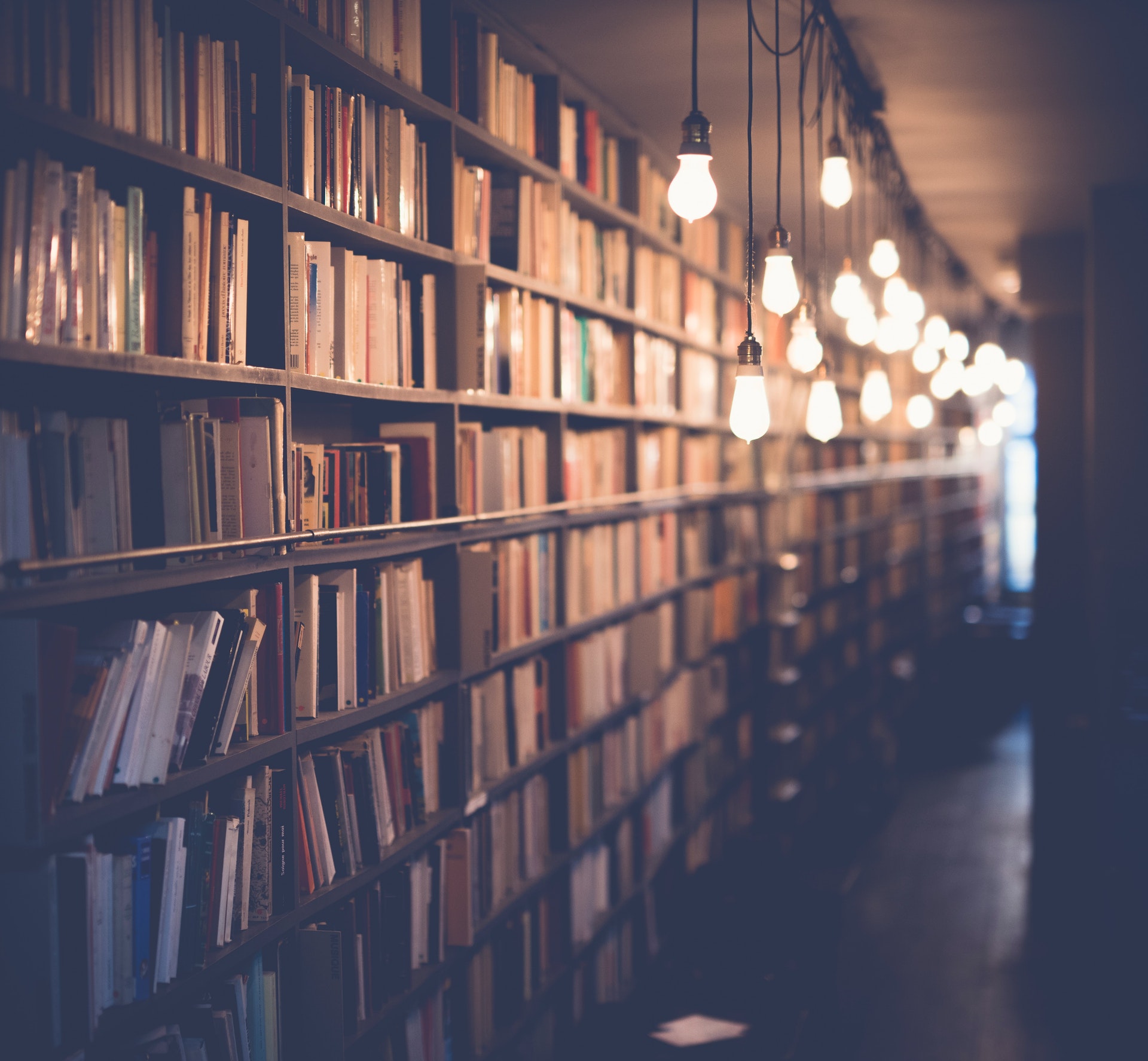
There are a number of JavaScript module bundlers, such as webpack and Browserify, that allow you to bundle your code into a single file. These bundlers typically expect that your code will use a CommonJS module system, which means that each file is a module and you use the require function to import other modules.
However, there are a number of popular JavaScript libraries that are not written using the CommonJS module system. Instead, they use a different module system, called AMD. AMD modules are designed to be used with a module loader, such as RequireJS.
One problem that can occur when using a bundler with AMD modules is that the bundler may not be able to find the module's dependencies. This can happen if the module is not written in a format that the bundler understands, or if the module doesn't provide an explicit list of its dependencies.
In some cases, you may be able to use a tool to convert AMD modules to a format that the bundler understands. But in other cases, you may need to find a different way to bundle your code.
See what others are reading: Should I Use My Real Name on Tinder?
What are the consequences of a module not providing an export named 'default'?
module.exports is the object that's returned as the result of a require call.
If a module doesn't provide a property named exports, then the module system will create an empty object, and the module will be returned as the result of require.
If the module does provide an exports property, then the module system will use that property as the result of require.
So, if a module doesn't provide a property named exports that's an object, then require will return an empty object.
A different take: What Many a Us State Is Named After?
How can you work around a module not providing an export named 'default'?
There are many ways to work around a module not providing an export named 'default'. One common way is to use a tool like webpack which can perform module analysiys and rewrite the code to add a default export. Another way is to use a tool like babel which can compile the code to add a default export. Another way is to simply create a file which performs the require statement for the module and exports the module.default property.
Consider reading: Watch Code
What is the difference between a module not providing an export named 'default' and a module providing an export named 'default' with a value of 'undefined'?
When a module does not provide an export named 'default', the import statement will look for an 'index' property on the module's default export. If there is no index property, the entire default export is returned. If the module provides an export named 'default' with a value of 'undefined', the import statement will return 'undefined' when used.
For your interest: What Disappears When You Say Its Name?
What problems can arise from using a module that does not provide an export named 'default'?
There are a few potential problems that could arise from using a module that does not provide an export named 'default'. First, if the module is only designed to work with a default export, then importing the module into a project that does not have a default export could cause errors. Secondly, if the module is frequently updated, and the default export is changed, projects that imported the module could break if they were not expecting the change. Finally, if the module is intended to be used as a library, not providing a default export could make it more difficult for users to consume.
Related reading: What Is Not an Alternate Name for Wolfsbane?
Frequently Asked Questions
How to solve SyntaxError does not provide an export named ‘default’?
We can only have a single export default in a JavaScript file. To make the default export the keyword exports should be changed to default and all exports must be preceded by the keyword import.
Why does the requested module does not provide an export named “default”?
The requested module does not provide an export named “default”.
What is the difference between a default export and a named Import?
A default export is a value that is exported by default in a CSV or Excel file. A named export is a value that has been given a name and can be imported into your report.
Does not provide an export name default error in Node JS?
If you run the file2.js file using the browser, you will get the following error. This code will generate a ‘module not found’ error because the module file is missing. Module not found error in browsers?
How to fix ‘does not provide an export name default’ in JavaScript?
Default keyword can fix the error.
Sources
- https://stackoverflow.com/questions/72142661/module-does-not-provide-an-export-named-default-compiled-typescript-module
- https://stackoverflow.com/questions/71022803/the-requested-module-does-not-provide-an-export-named-default-error-but
- https://bobbyhadz.com/blog/javascript-requested-module-not-provide-export-named-default
- https://www.appsloveworld.com/vuejs/100/12/how-do-i-fix-the-requested-module-does-not-provide-an-export-named-default
- https://bobbyhadz.com/blog/javascript-requested-module-not-provide-export-named
- https://errorsandanswers.com/uncaught-syntaxerror-the-requested-module-add-js-does-not-provide-an-export-named-add/
- https://stackoverflow.com/questions/57333522/module-doesnt-provide-an-export-named-default
- https://bobbyhadz.com/blog/javascript-does-not-contain-default-export
- https://www.reddit.com/r/Deno/comments/gwht72/the_requested_module_does_not_provide_an_export/
- https://github.com/Polymer/tools/issues/608
- https://www.roelvanlisdonk.nl/2017/11/08/fix-typescript-error-module-has-no-default-export/
- https://errorsandanswers.com/why-and-when-to-use-default-export-over-named-exports-in-es6-modules/
- https://solveforum.com/forums/threads/solved-why-does-fullcalendar-give-the-error-that-it-does-not-provide-an-export-called-default.273525/
- https://github.com/standard-things/esm/issues/182
- https://github.com/vitejs/vite/issues/2679
- https://bignerdranch.com/blog/default-exports-or-named-exports-why-not-both/
- https://github.com/nuxt/framework/issues/5666
- https://stackoverflow.com/questions/69602633/whats-the-difference-between-import-name-from-module-and-import-as-name
- https://www.tutorialsinfo.com/es6-modules.html
- https://www.reddit.com/r/node/comments/6d2jyc/moduleexports_best_practices/
- https://blog.neufund.org/why-we-have-banned-default-exports-and-you-should-do-the-same-d51fdc2cf2ad
- https://stackify.com/node-js-module-exports/
- https://stackoverflow.com/questions/41666130/export-default-vs-module-exports-differences
- https://technical-qa.com/what-is-the-difference-between-module-exports-and-export-default/
- https://stackoverflow.com/questions/56938798/what-is-the-difference-between-export-default-and-module-exports
Featured Images: pexels.com